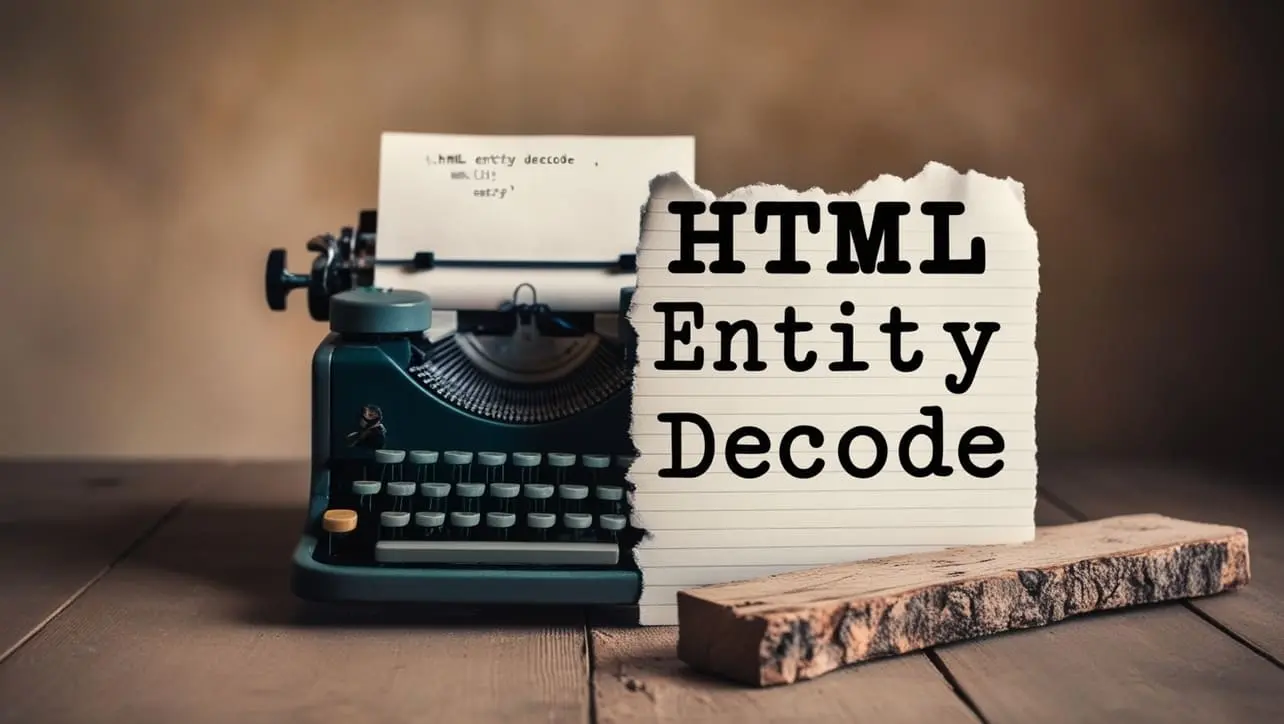
PHP Topics
- PHP Intro
- PHP String Functions
- addcslashes()
- addslashes()
- bin2hex()
- chop()
- chr()
- chunk_split()
- convert_cyr_string()
- convert_uudecode()
- convert_uuencode()
- count_chars()
- crc32()
- crypt()
- explode()
- fprintf()
- get_html_translation_table()
- hebrev()
- hebrevc()
- hex2bin()
- html_entity_decode()
- htmlentities()
- htmlspecialchars_decode()
- htmlspecialchars()
- implode()
- join()
- PHP Interview Programs
- PHP Star Pattern
- PHP Number Pattern
- PHP Alphabet Pattern
PHP String fprintf() Function
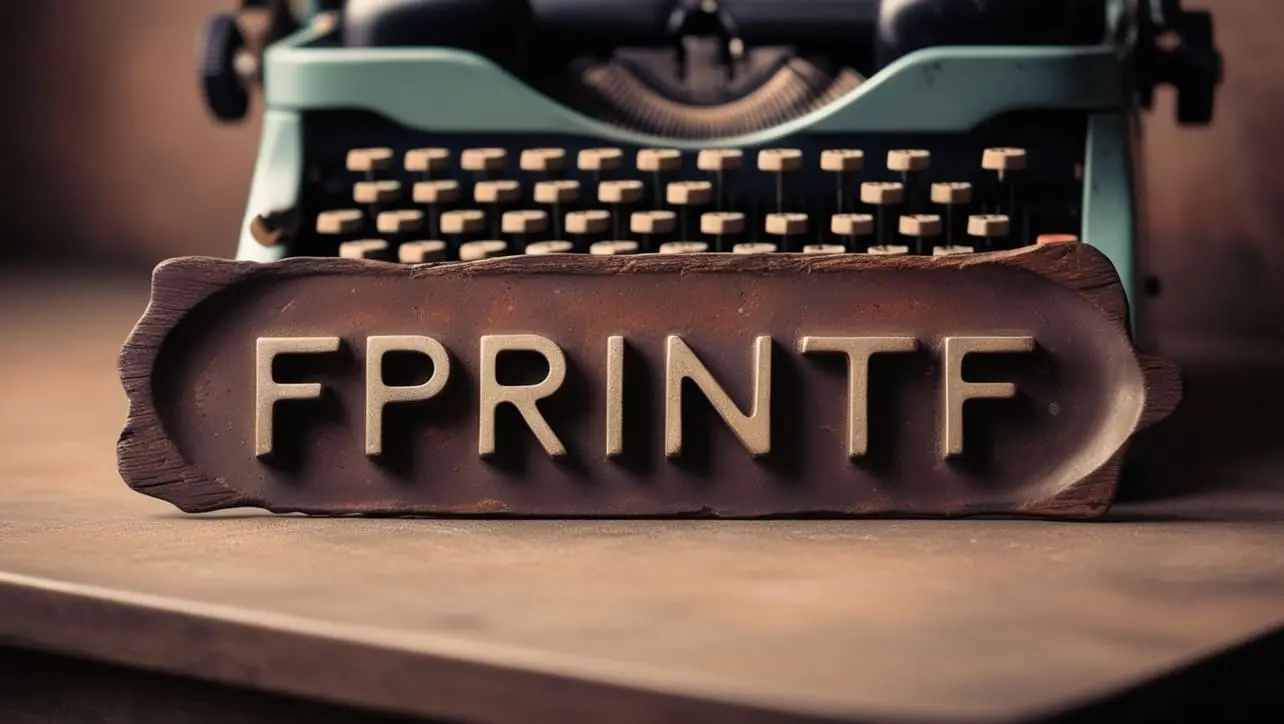
Photo Credit to CodeToFun
Introduction
In PHP programming, working with strings is a common task, and the fprintf()
function provides a way to format strings and write them to a file or, in this case, a string.
This function is similar to sprintf(), but it allows you to specify a file handle or a string as the output destination.
In this tutorial, we'll explore the usage and functionality of the fprintf()
function for formatting strings.
Syntax
The signature of the fprintf()
function is as follows:
fprintf($handle, $format, $arg1, $arg2, ...);
- $handle: The file handle or string where the formatted output will be written.
- $format: The format string, similar to the one used in sprintf().
- $arg1, $arg2, ...: The values to be formatted and inserted into the format string.
Example
Let's delve into an example to illustrate how the fprintf()
function works with a string as the output destination.
<?php
// Open a temporary memory stream
$memoryStream = fopen('php://memory', 'rw+');
// Check if the stream was opened successfully
if ($memoryStream) {
// Format and write to the memory stream
fprintf($memoryStream, "Hello, %s!", "PHP");
// Rewind the memory stream
rewind($memoryStream);
// Read the contents of the memory stream
$output = stream_get_contents($memoryStream);
// Close the memory stream
fclose($memoryStream);
// Output the result
echo $output;
} else {
echo "Failed to open the memory stream.";
}
?>
Output
Hello, PHP!
How the Program Works
In this example, the fprintf()
function is used to format the string "Hello, %s!" with the value "PHP" and write it to a temporary memory stream. The contents of the memory stream are then read and output.
Return Value
The fprintf()
function returns the length of the output string or false on failure.
Common Use Cases
The fprintf()
function is particularly useful when you need to format strings and write them to a specific destination, such as a file or a string buffer. It allows you to control the output format and insert dynamic values seamlessly.
Notes
- Ensure that the file or string handle passed to
fprintf()
is opened in a mode that allows writing.
Optimization
The fprintf()
function is generally optimized for its purpose. If you're dealing with a large amount of data, consider optimizing the string formatting and concatenation for better performance.
Conclusion
The fprintf()
function in PHP is a versatile tool for formatting strings and writing them to a specified destination. Whether you're working with files or in-memory strings, fprintf()
provides a convenient way to control the output format dynamically.
Feel free to experiment with different format strings and destinations to see the flexibility of the fprintf()
function. Happy coding!
Join our Community:
Author
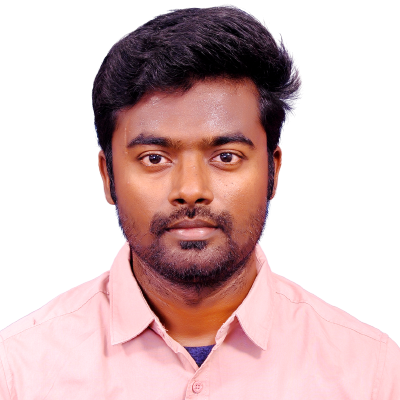
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in PHP
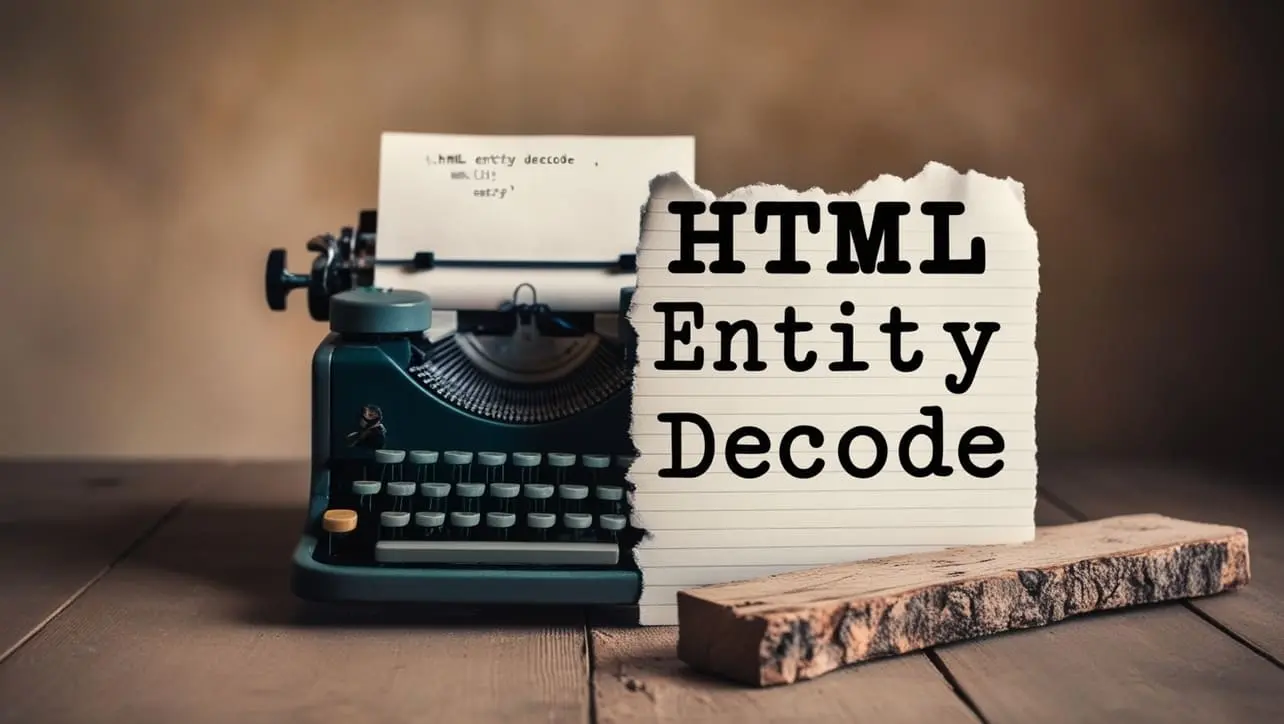
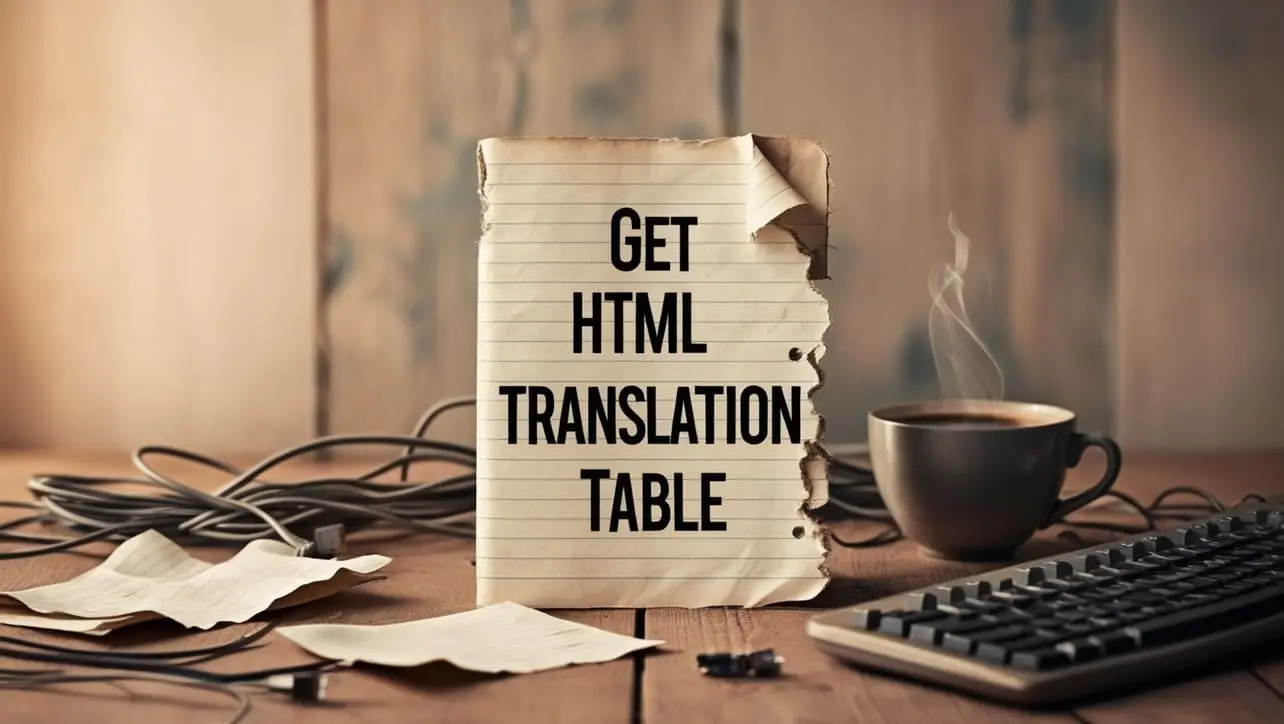
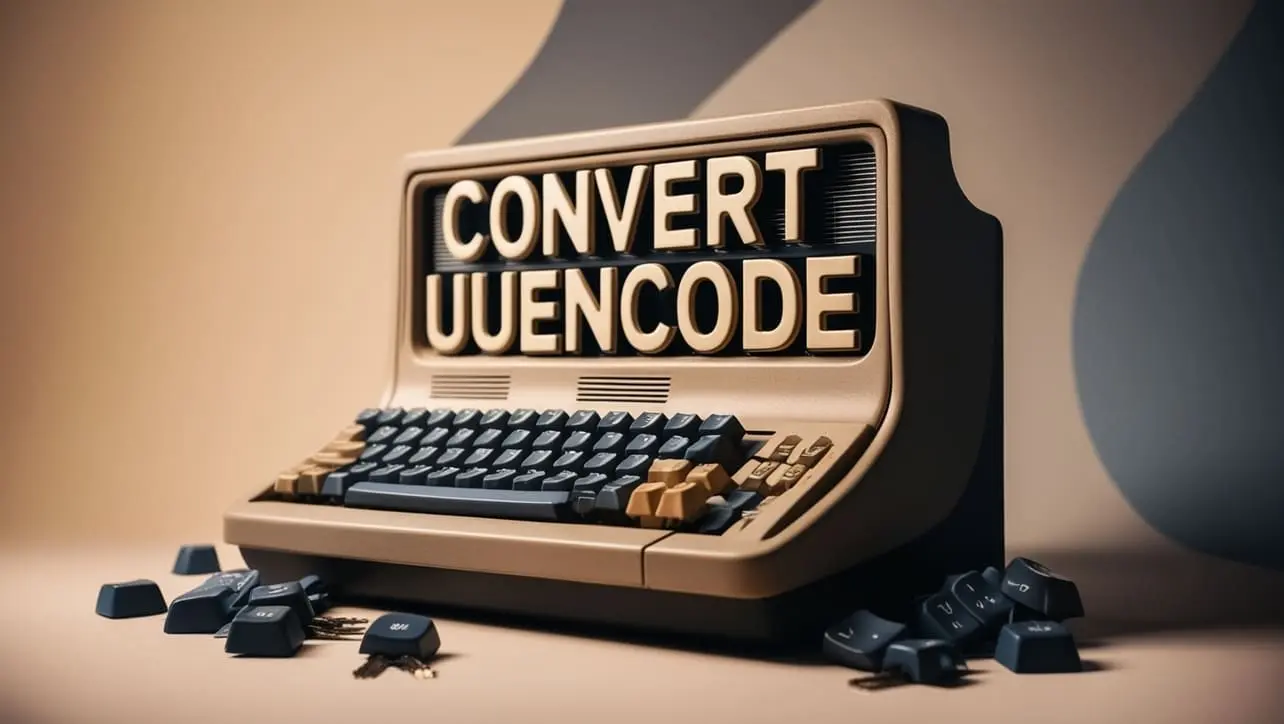
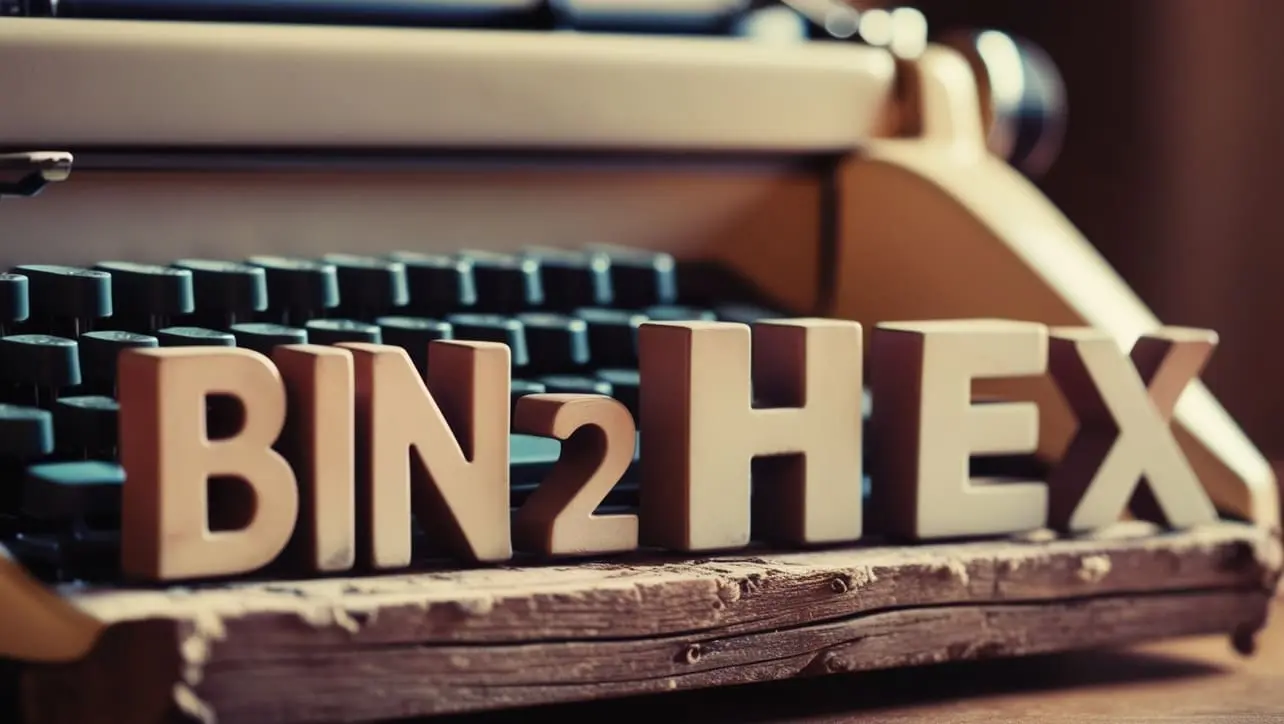
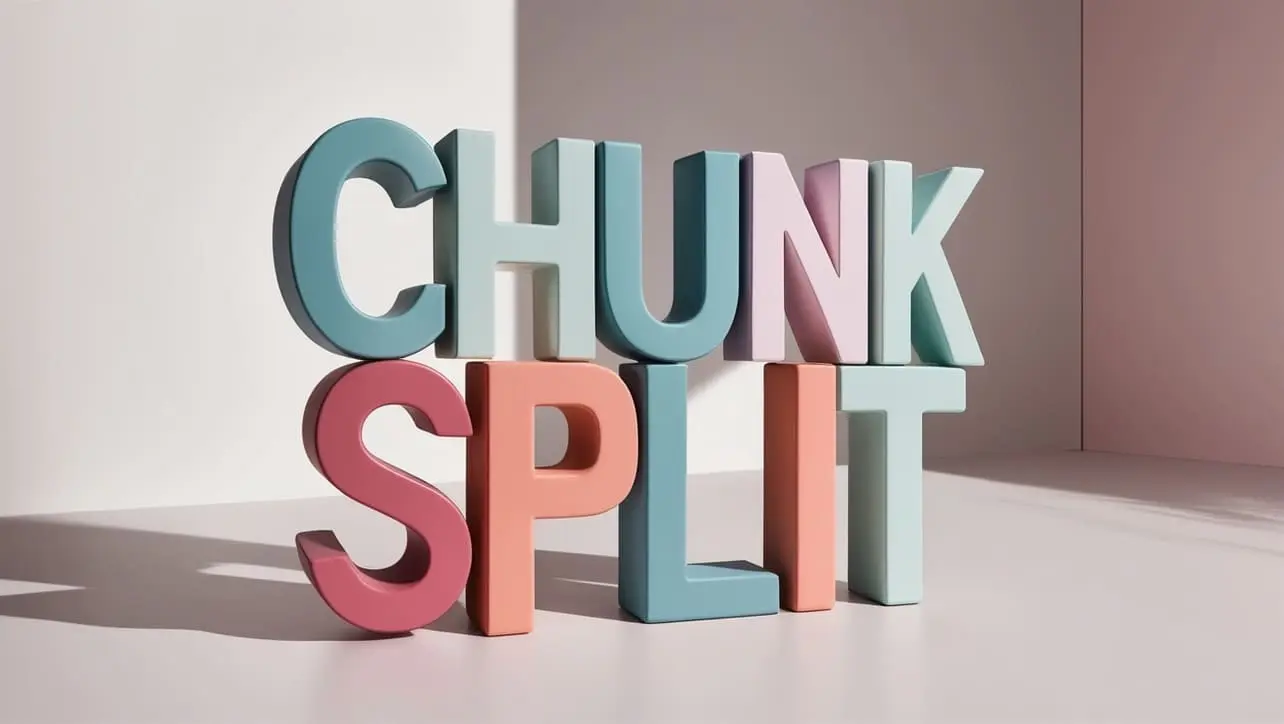

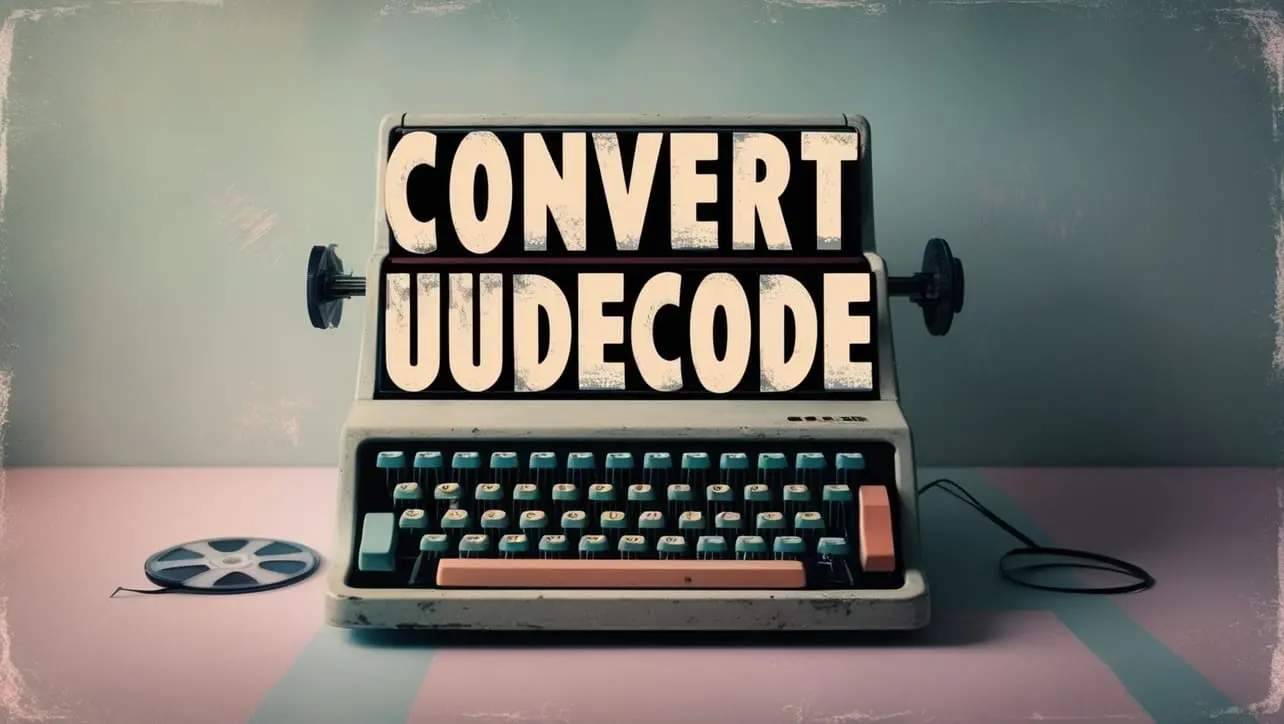
If you have any doubts regarding this article (PHP String fprintf() Function), please comment here. I will help you immediately.