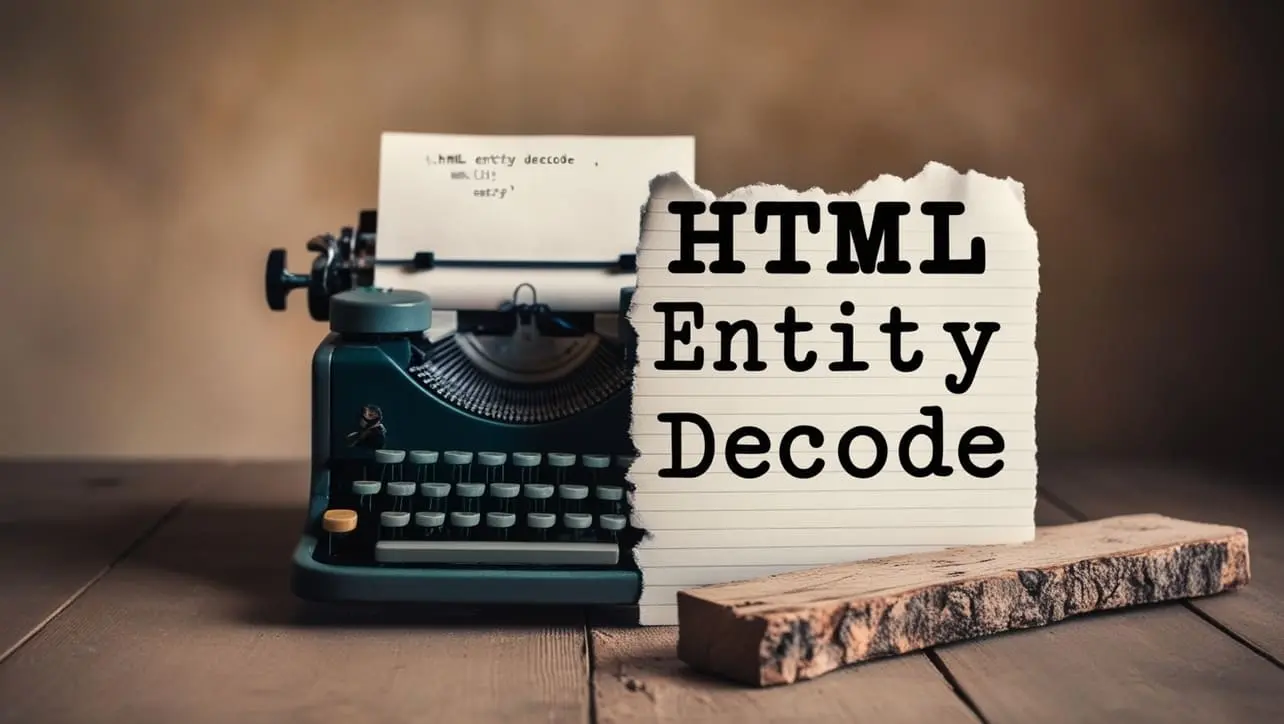
PHP Basic
- PHP Intro
- PHP Star Pattern
- PHP Number Pattern
- PHP Alphabet Pattern
- PHP String Functions
- PHP addcslashes()
- PHP addslashes()
- PHP bin2hex()
- PHP chop()
- PHP chr()
- PHP chunk_split()
- PHP convert_cyr_string()
- PHP convert_uudecode()
- PHP convert_uuencode()
- PHP count_chars()
- PHP crc32()
- PHP crypt()
- PHP explode()
- PHP fprintf()
- PHP get_html_translation_table()
- PHP hebrev()
- PHP hebrevc()
- PHP hex2bin()
- PHP html_entity_decode()
- PHP htmlentities()
- PHP htmlspecialchars_decode()
- PHP htmlspecialchars()
- PHP implode()
- PHP join()
PHP String chr() Function
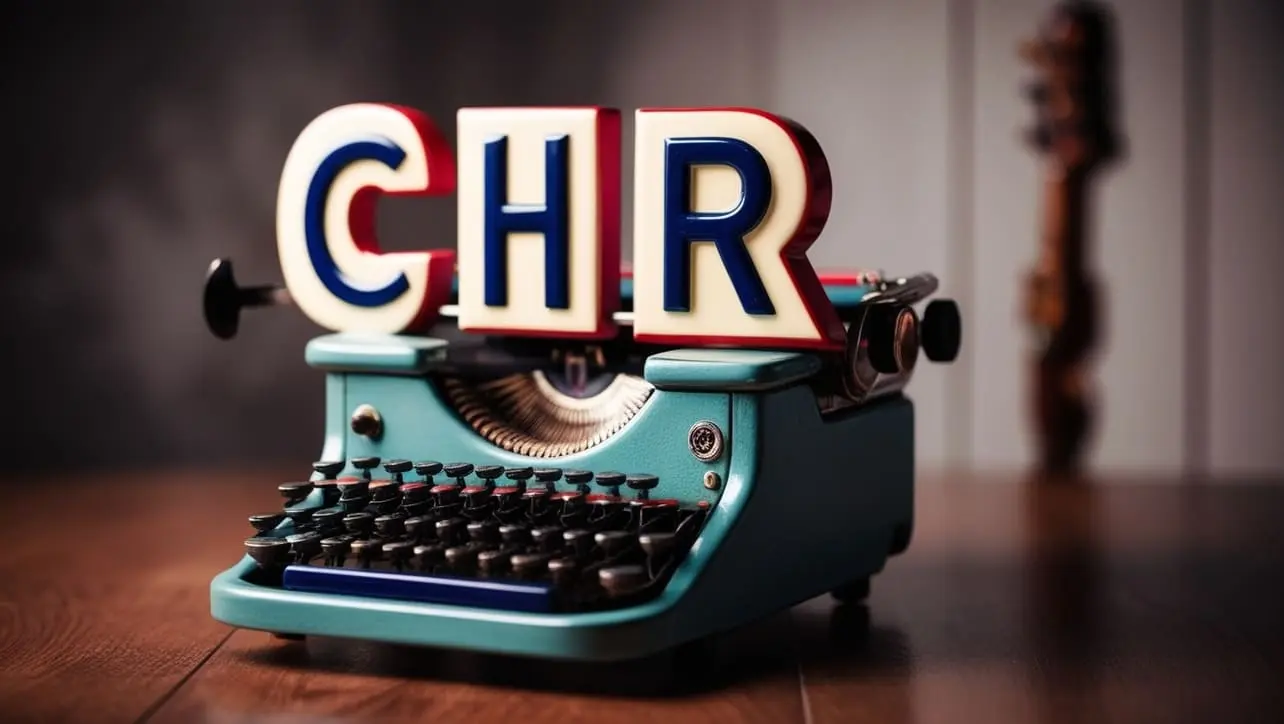
Photo Credit to CodeToFun
đ Introduction
In PHP, strings play a crucial role in handling textual data, and various functions are available to manipulate and work with strings.
The chr()
function is one such function that converts an ASCII code to its corresponding character.
In this tutorial, we'll explore the usage and functionality of the chr()
function in PHP.
đĄ Syntax
The signature of the chr()
function is as follows:
string chr(int $ascii);
- $ascii: An integer representing the ASCII code of the desired character.
đ Example
Let's delve into an example to illustrate how the chr()
function works.
<?php
// ASCII code for the letter 'A'
$asciiCode = 65;
// Convert ASCII code to character using chr()
$character = chr($asciiCode);
// Output the result
echo "Character: $character\n";
?>
đģ Output
Character: A
đ§ How the Program Works
In this example, the chr()
function converts the ASCII code 65 (which corresponds to the letter 'A') to the actual character and prints the result.
âŠī¸ Return Value
The chr()
function returns a string containing the character represented by the ASCII code.
đ Common Use Cases
The chr()
function is particularly useful when you need to convert ASCII codes to characters dynamically. It is commonly used in scenarios where you want to generate strings based on ASCII values or manipulate individual characters.
đ Notes
- Ensure that the provided ASCII code is within the valid range (0 to 127) to get meaningful results.
- The
chr()
function is the inverse of ord(), which converts a character to its ASCII code.
đĸ Optimization
The chr()
function is optimized for converting ASCII codes to characters. However, for performance-critical applications, optimization is usually not necessary due to the function's lightweight nature.
đ Conclusion
The chr()
function in PHP is a handy tool for converting ASCII codes to characters. It provides a straightforward and efficient way to work with individual characters, enhancing the versatility of your PHP scripts.
Feel free to experiment with different ASCII codes and explore the behavior of the chr()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
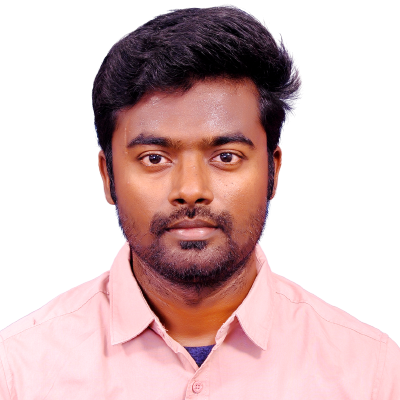
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP String chr() Function), please comment here. I will help you immediately.