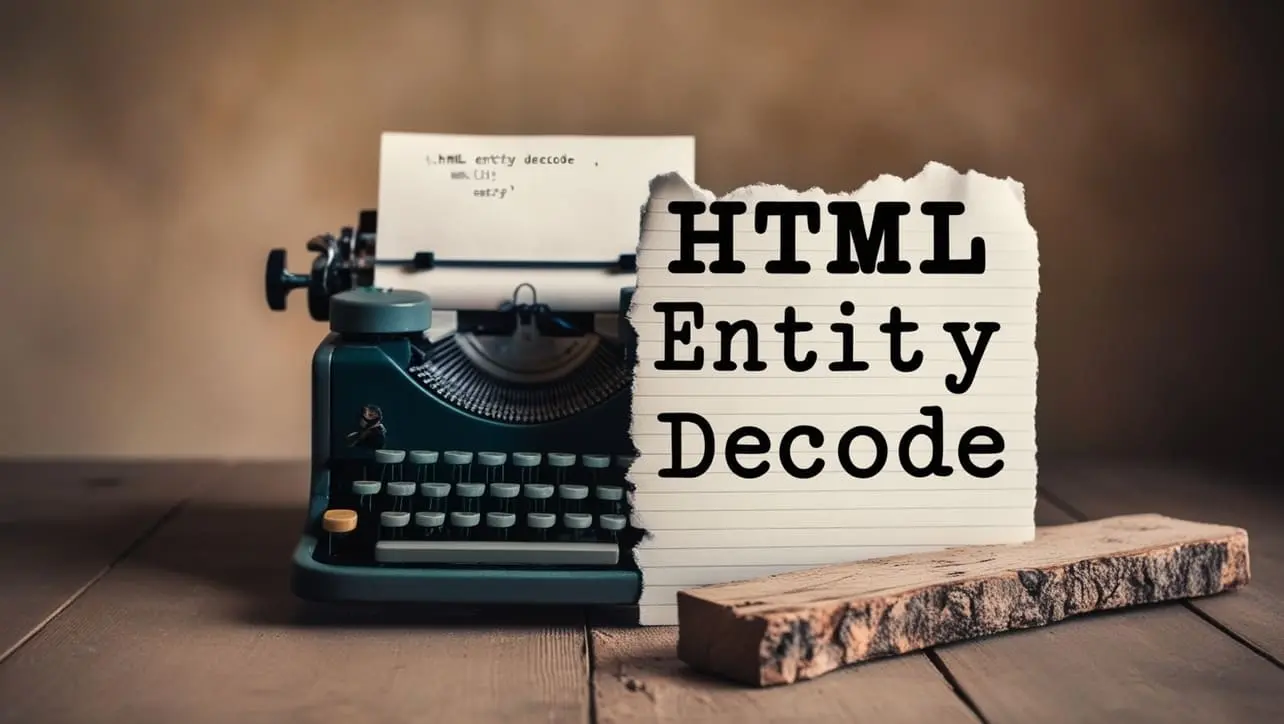
PHP Topics
- PHP Intro
- PHP String Functions
- addcslashes()
- addslashes()
- bin2hex()
- chop()
- chr()
- chunk_split()
- convert_cyr_string()
- convert_uudecode()
- convert_uuencode()
- count_chars()
- crc32()
- crypt()
- explode()
- fprintf()
- get_html_translation_table()
- hebrev()
- hebrevc()
- hex2bin()
- html_entity_decode()
- htmlentities()
- htmlspecialchars_decode()
- htmlspecialchars()
- implode()
- join()
- PHP Interview Programs
- PHP Star Pattern
- PHP Number Pattern
- PHP Alphabet Pattern
PHP String chop() Function
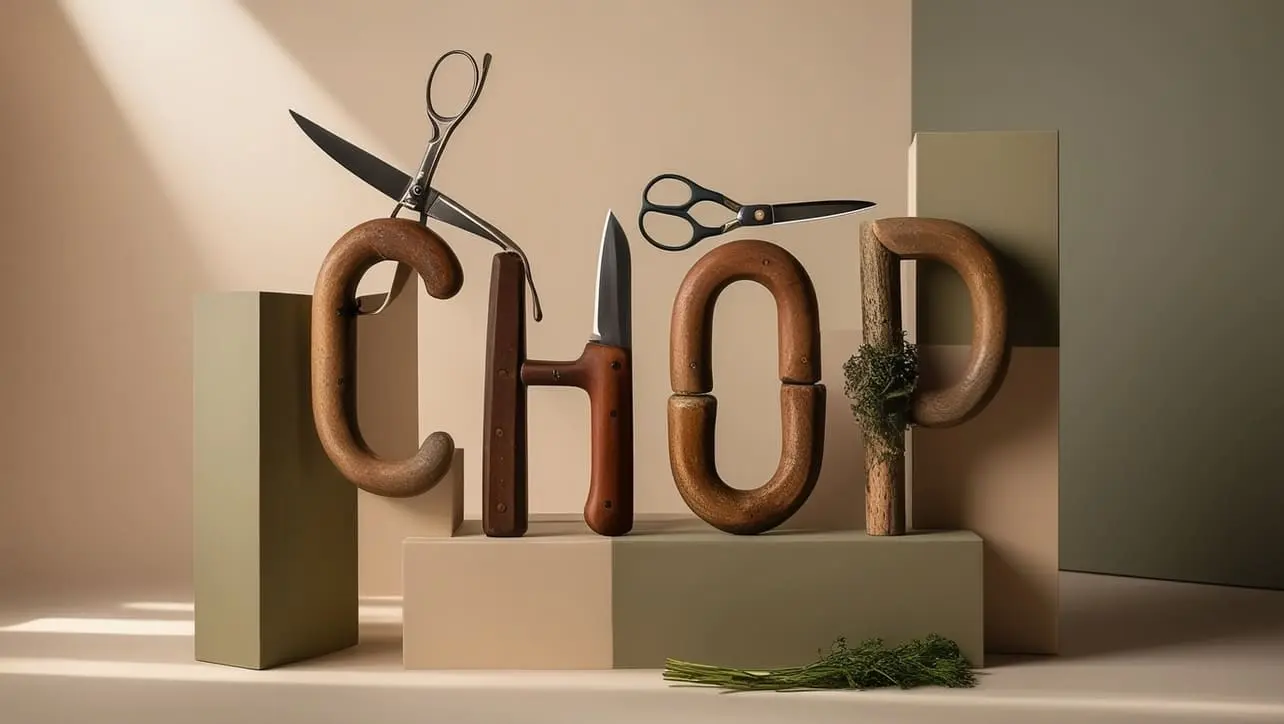
Photo Credit to CodeToFun
đ Introduction
In PHP, manipulating strings is a common task, and various functions are available for string operations.
The chop()
function is one such function that removes whitespace or other specified characters from the end of a string. It is essentially an alias for the rtrim() function.
In this tutorial, we'll explore the usage and functionality of the chop()
function in PHP.
đĄ Syntax
The syntax for the chop()
function is as follows:
string chop(string $string, string $characters = " \t\n\r\0\x0B" )
- $string: The input string to be trimmed.
- $characters (optional): A list of characters to be removed. If not specified, it removes whitespaces.
đ Example
Let's delve into an example to illustrate how the chop()
function works.
<?php
// Example 1
$text1 = "Hello, World! ";
$trimmed1 = chop($text1);
echo "Example 1: '$trimmed1'\n"; // Output: 'Hello, World!'
// Example 2
$text2 = " PHP is awesome \t";
$trimmed2 = chop($text2, " \t");
echo "Example 2: '$trimmed2'\n"; // Output: ' PHP is awesome'
?>
đģ Output
Example 1: 'Hello, World!' Example 2: ' PHP is awesome'
đ§ How the Program Works
In Example 1, the chop()
function removes trailing whitespaces from the string "Hello, World!".
In Example 2, it removes leading and trailing whitespaces and tabs from the string "PHP is awesome".
âŠī¸ Return Value
The chop()
function returns the trimmed string.
đ Common Use Cases
The chop()
function is useful when you want to remove trailing whitespaces or specific characters from the end of a string. It's commonly used to clean up user input or prepare data for further processing.
đ Notes
chop()
is essentially an alias for the rtrim() function. They behave identically.- The default list of characters to remove includes space, tab, newline, carriage return, null byte, and vertical tab.
đĸ Optimization
The chop()
function is optimized for string trimming. If you have specific character removal needs, consider using rtrim() with custom character lists for better control.
đ Conclusion
The chop()
function in PHP is a convenient tool for removing trailing whitespaces or specified characters from the end of a string. It provides a clean and readable way to sanitize and manipulate strings in your PHP applications.
Feel free to experiment with different strings and explore the behavior of the chop()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
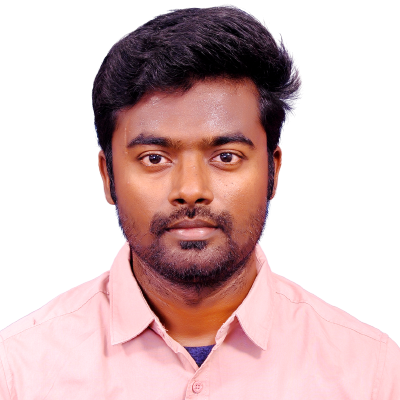
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in PHP
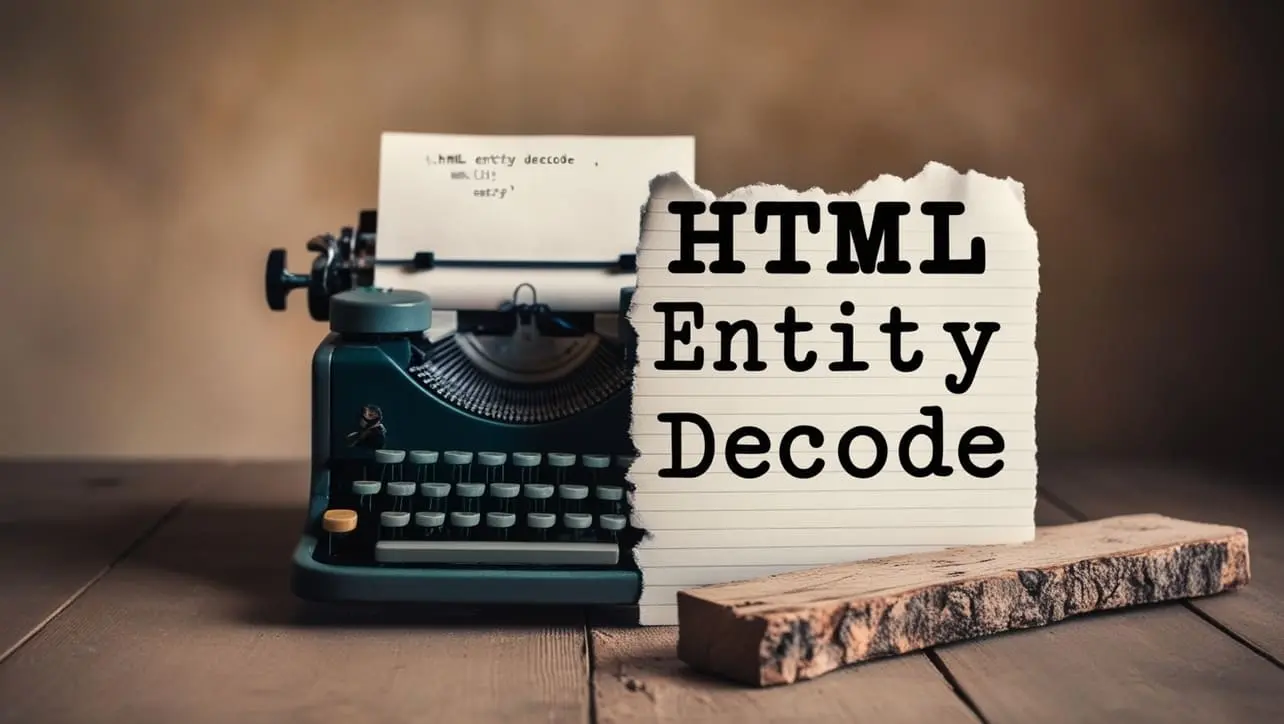
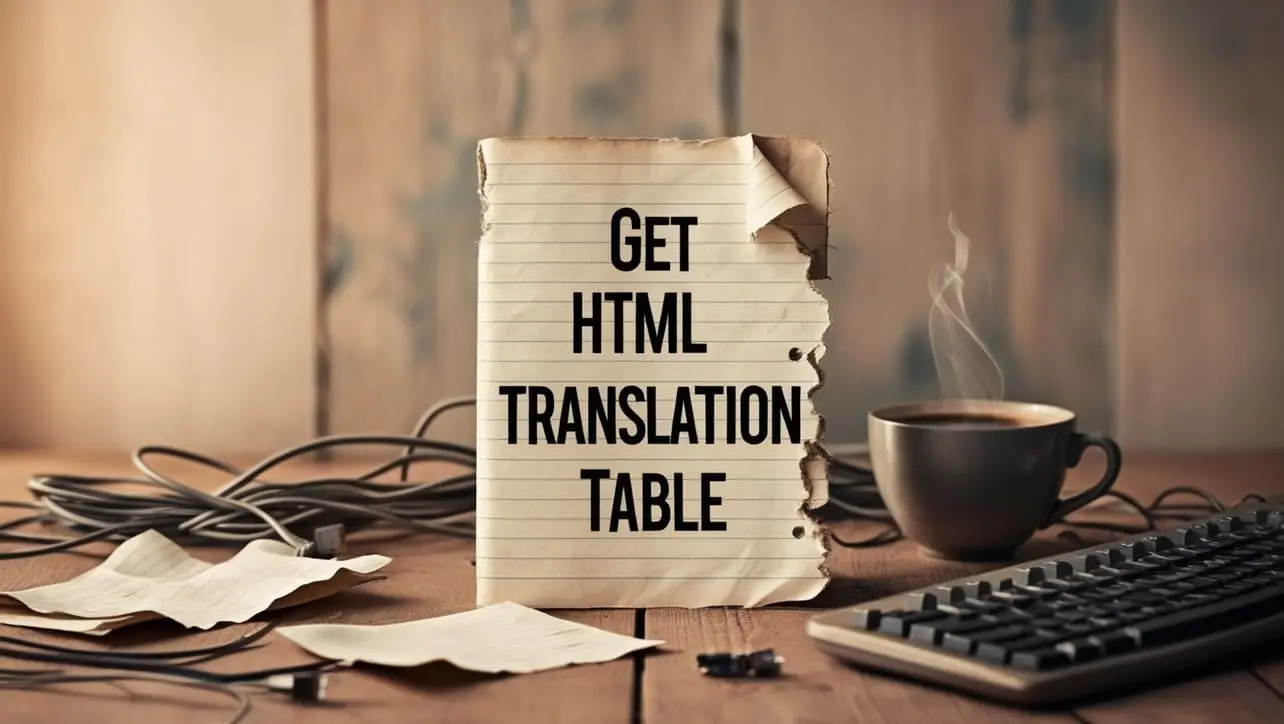
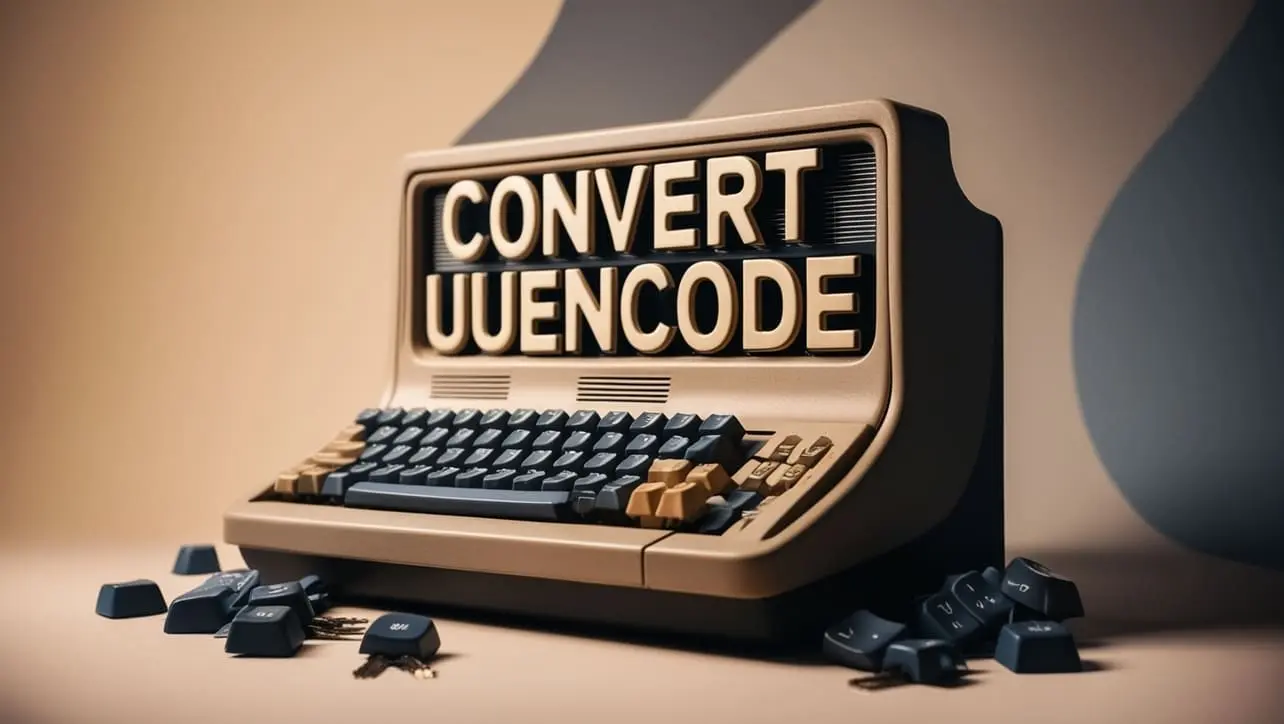
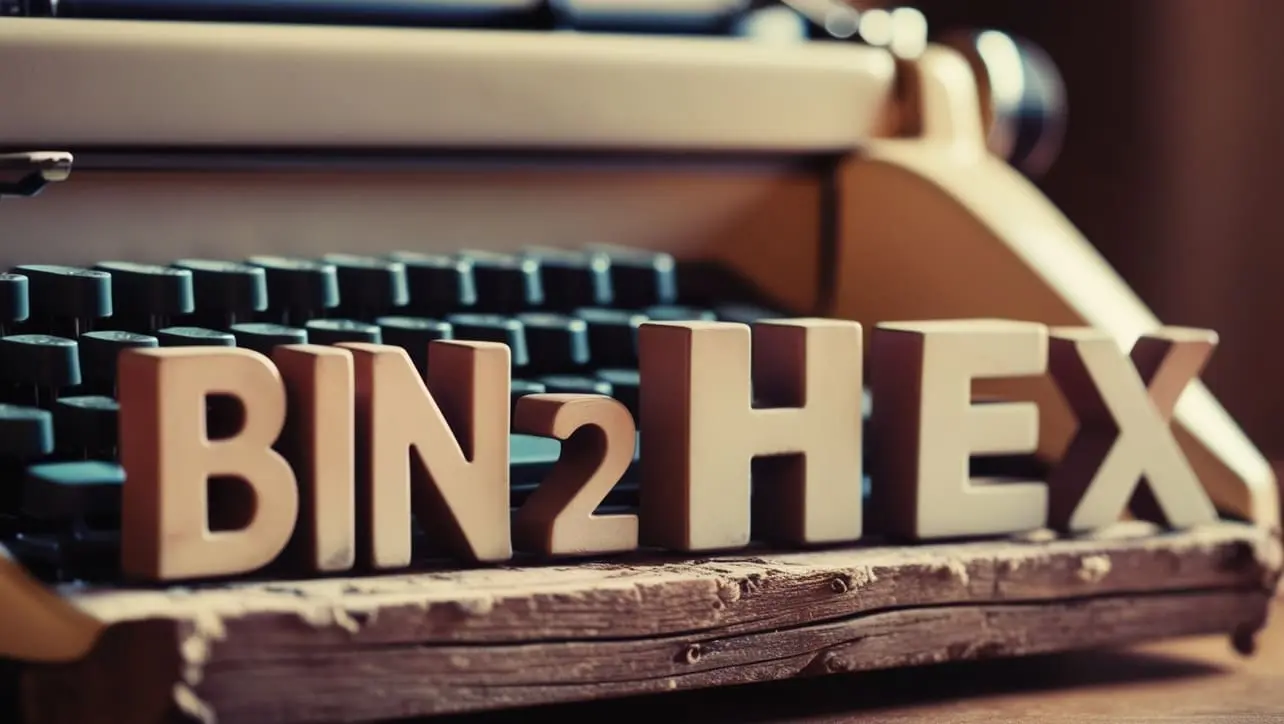
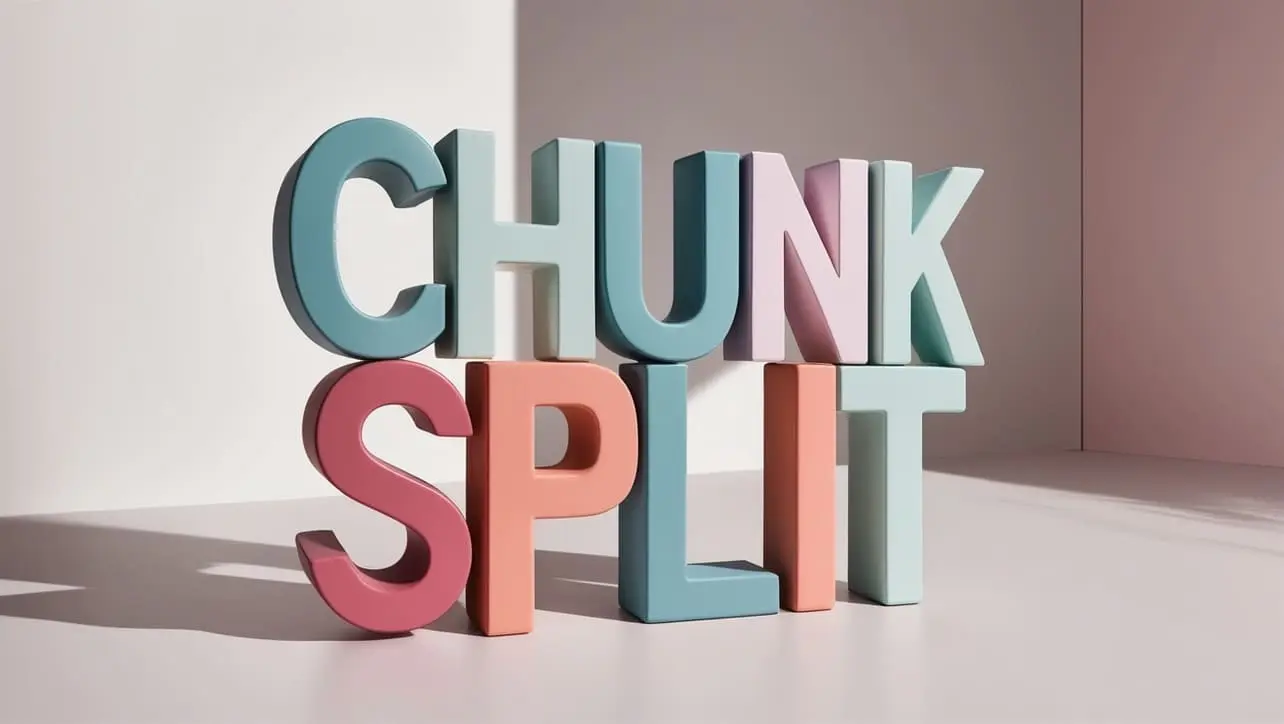

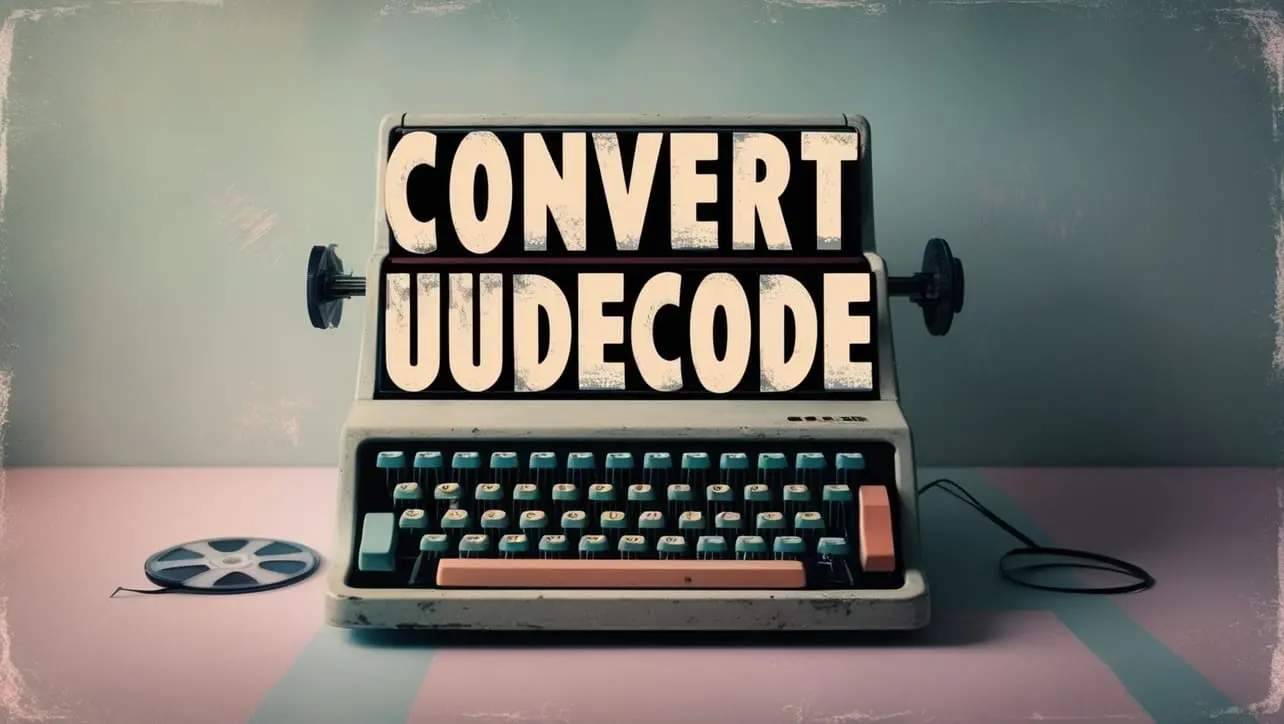
If you have any doubts regarding this article (PHP String chop() Function), please comment here. I will help you immediately.