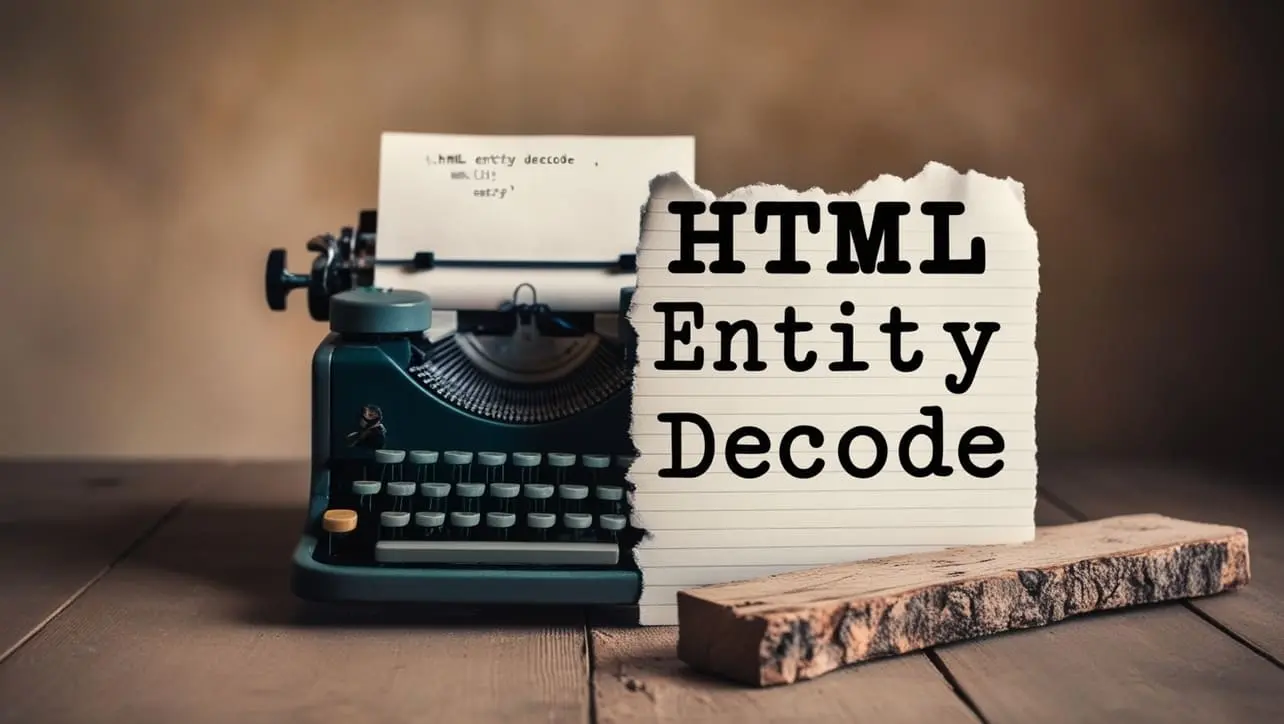
PHP Basic
- PHP Intro
- PHP Star Pattern
- PHP Number Pattern
- PHP Alphabet Pattern
- PHP String Functions
- PHP addcslashes()
- PHP addslashes()
- PHP bin2hex()
- PHP chop()
- PHP chr()
- PHP chunk_split()
- PHP convert_cyr_string()
- PHP convert_uudecode()
- PHP convert_uuencode()
- PHP count_chars()
- PHP crc32()
- PHP crypt()
- PHP explode()
- PHP fprintf()
- PHP get_html_translation_table()
- PHP hebrev()
- PHP hebrevc()
- PHP hex2bin()
- PHP html_entity_decode()
- PHP htmlentities()
- PHP htmlspecialchars_decode()
- PHP htmlspecialchars()
- PHP implode()
- PHP join()
PHP String addslashes() Function
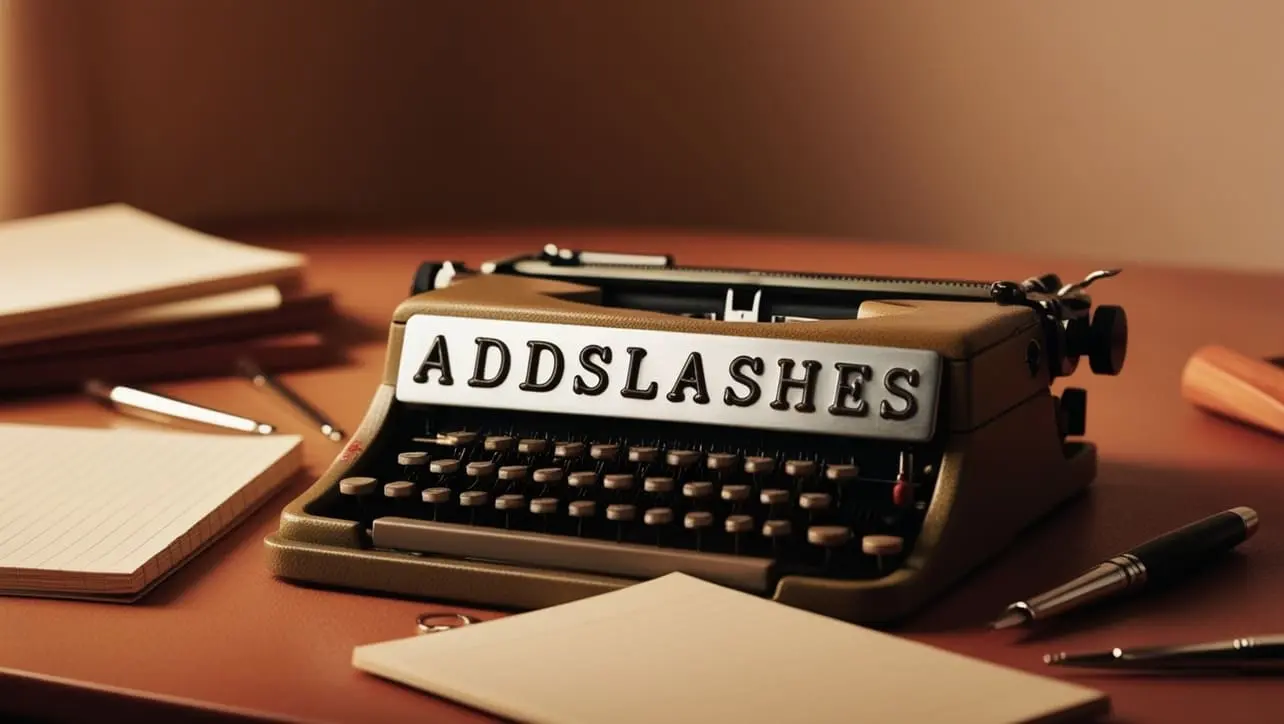
Photo Credit to CodeToFun
đ Introduction
In PHP programming, dealing with strings and escaping special characters is a common task, especially when working with databases or handling user input.
The addslashes()
function is a built-in PHP function that allows you to escape characters in a string.
In this tutorial, we'll explore the usage and functionality of the addslashes()
function.
đĄ Syntax
The signature of the addslashes()
function is as follows:
string addslashes ( string $str )
This function takes a string, str, as an argument and returns the string with certain characters preceded by a backslash.
đ Example
Let's delve into an example to illustrate how the addslashes()
function works.
<?php
// Sample string with special characters
$originalString = "This is a string with a single quote (').";
// Escape special characters
$escapedString = addslashes($originalString);
// Output the result
echo "Original String: $originalString\n";
echo "Escaped String: $escapedString\n";
?>
đģ Output
Original String: This is a string with a single quote ('). Escaped String: This is a string with a single quote (\').
đ§ How the Program Works
In this example, the addslashes()
function escapes the single quote in the original string, and the result is printed.
âŠī¸ Return Value
The addslashes()
function returns a string with certain characters (single quote ('), double quote ("), backslash (\), and NUL (the NULL byte)) preceded by a backslash.
đ Common Use Cases
The addslashes()
function is commonly used when dealing with strings that may contain characters that have special meaning in SQL queries or other contexts. It helps prevent potential security vulnerabilities, such as SQL injection.
đ Notes
- While
addslashes()
can be useful for certain scenarios, it might not be the optimal solution for all situations. For database queries, consider using parameterized statements or prepared statements for increased security.
đĸ Optimization
The addslashes()
function is relatively straightforward and doesn't require specific optimization. However, when dealing with database interactions, it's advisable to use appropriate database-specific functions or prepared statements for enhanced security.
đ Conclusion
The addslashes()
function in PHP is a useful tool for escaping special characters in strings, particularly when working with databases. While it serves its purpose in specific scenarios, it's essential to consider other secure practices, such as parameterized queries, for robust security measures.
Feel free to experiment with different strings and explore the behavior of the addslashes()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
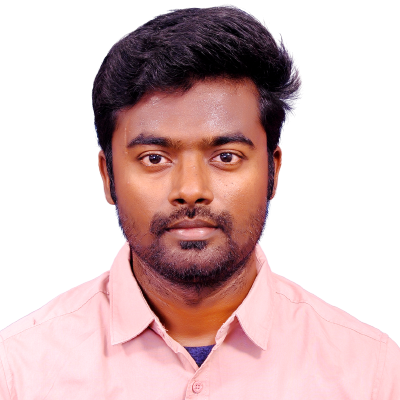
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP String addslashes() Function), please comment here. I will help you immediately.