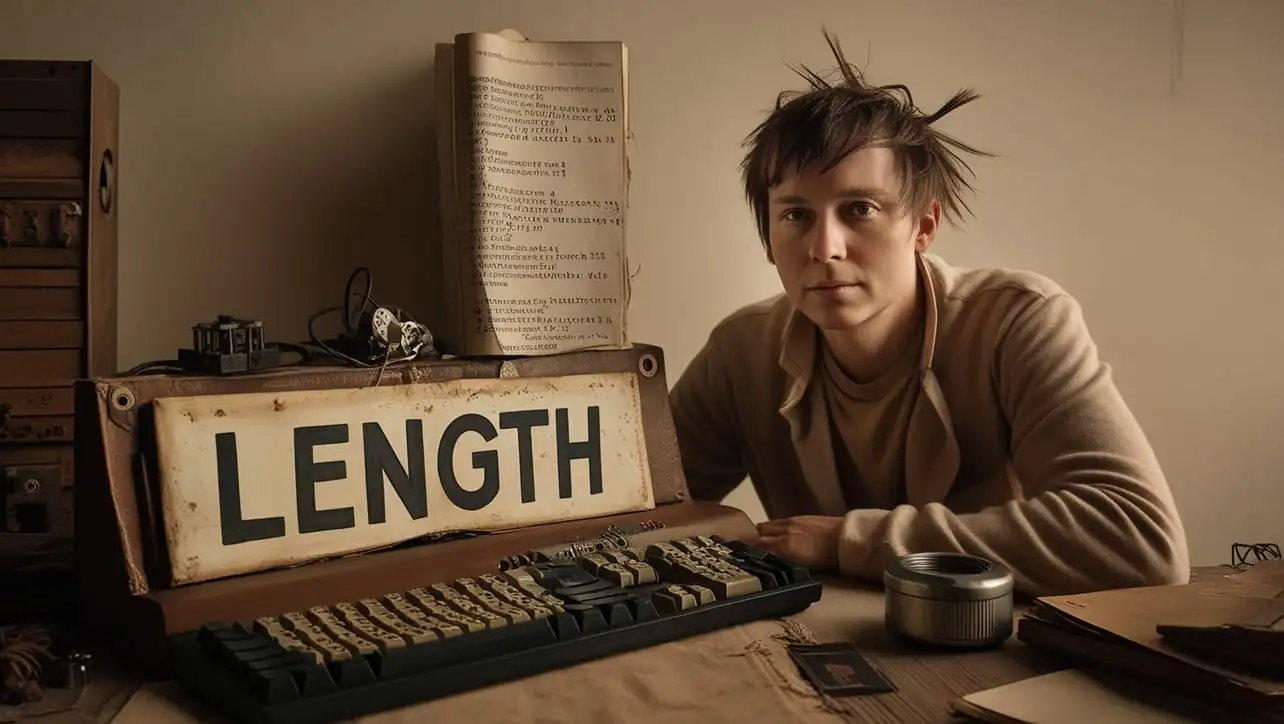
JavaScript Window scrollBy() Method
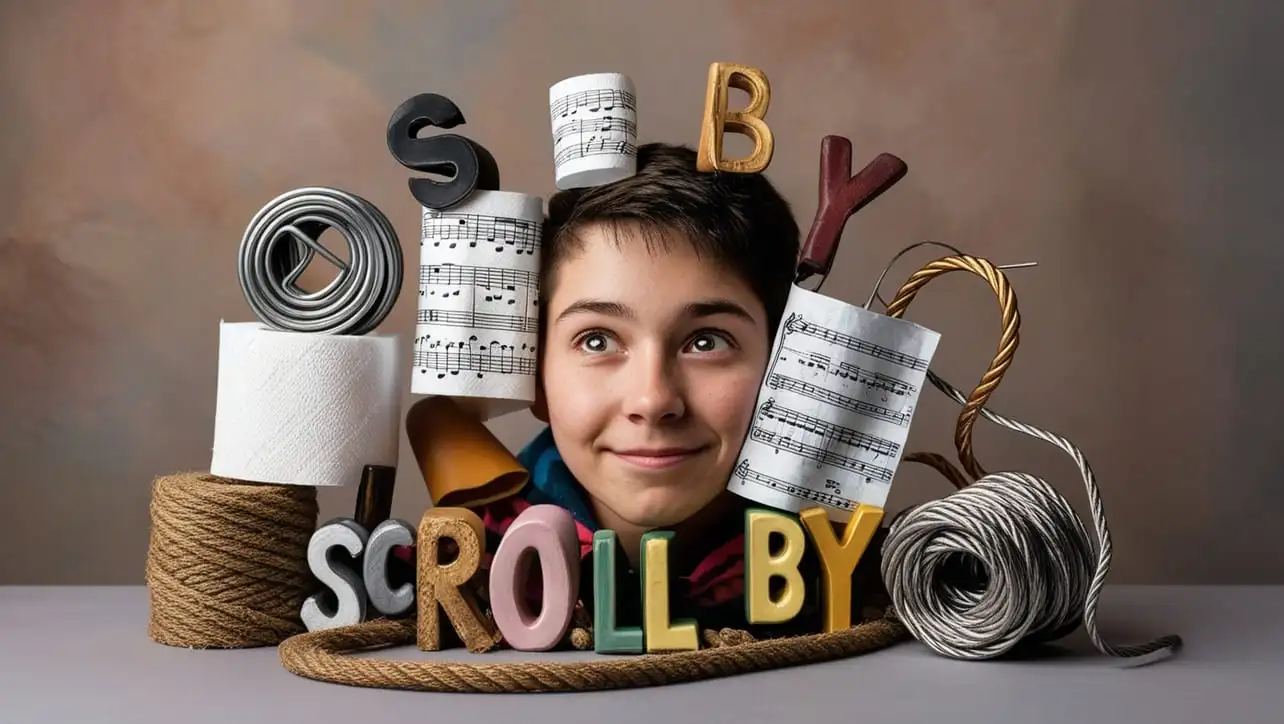
Photo Credit to CodeToFun
🙋 Introduction
When it comes to controlling the scrolling behavior of a webpage, the scrollBy()
method is a valuable tool in a JavaScript developer's toolkit. This method allows you to scroll the content of a window by a specified number of pixels, providing a dynamic and programmatic way to navigate through web pages.
In this guide, we'll explore the scrollBy()
method, its syntax, usage examples, best practices, and practical scenarios.
🧠 Understanding scrollBy() Method
The scrollBy()
method is part of the Window interface in JavaScript and is used to scroll the content of a window by a specified number of pixels horizontally and vertically.
💡 Syntax
The syntax for the scrollBy()
method is straightforward:
window.scrollBy(x, y);
- x: The number of pixels to scroll horizontally. A positive value scrolls to the right, and a negative value scrolls to the left.
- y: The number of pixels to scroll vertically. A positive value scrolls down, and a negative value scrolls up.
📝 Example
Let's dive into a simple example to illustrate the usage of the scrollBy()
method:
// Scroll the window by 200 pixels horizontally and 100 pixels vertically
window.scrollBy(200, 100);
In this example, the scrollBy()
method is used to scroll the window by 200 pixels to the right and 100 pixels down.
🏆 Best Practices
When working with the scrollBy()
method, consider the following best practices:
Smooth Scrolling:
For a smoother scrolling experience, consider using the scrollTo() method with the { behavior: 'smooth' } option.
example.jsCopied// Smoothly scroll the window to the right by 200 pixels window.scrollBy({ left: 200, behavior: 'smooth', });
Boundaries Checking:
Ensure that the scrolling values do not exceed the boundaries of the webpage to avoid unintended behaviors.
example.jsCopied// Check if scrolling to the right won't exceed the document width if (window.scrollX + 200 <= document.documentElement.scrollWidth - window.innerWidth) { window.scrollBy(200, 0); }
📚 Use Cases
Animated Scroll to Section:
The
scrollBy()
method can be used to create smooth animated scrolling to a specific section of a webpage:example.jsCopied// Scroll to the next section with a smooth animation document.getElementById('next-section-button').addEventListener('click', function() { window.scrollBy({ top: window.innerHeight, behavior: 'smooth', }); });
Infinite Scrolling Trigger:
Implementing an infinite scroll feature can be achieved by detecting when the user is close to the bottom of the page and triggering additional content loading:
example.jsCopiedwindow.addEventListener('scroll', function() { // Check if the user is near the bottom of the page if (window.innerHeight + window.scrollY >= document.body.offsetHeight - 100) { // Load more content loadMoreContent(); } });
🎉 Conclusion
The scrollBy()
method is a powerful tool for controlling the scrolling behavior of a webpage dynamically.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the scrollBy()
method in your JavaScript projects.
👨💻 Join our Community:
Author
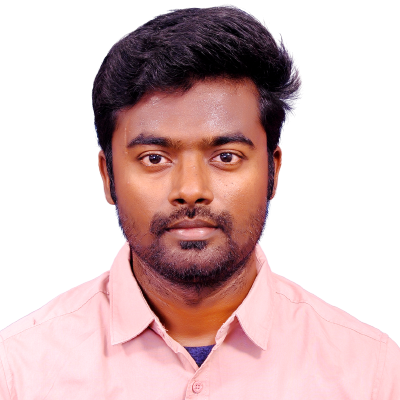
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Window scrollBy() Method), please comment here. I will help you immediately.