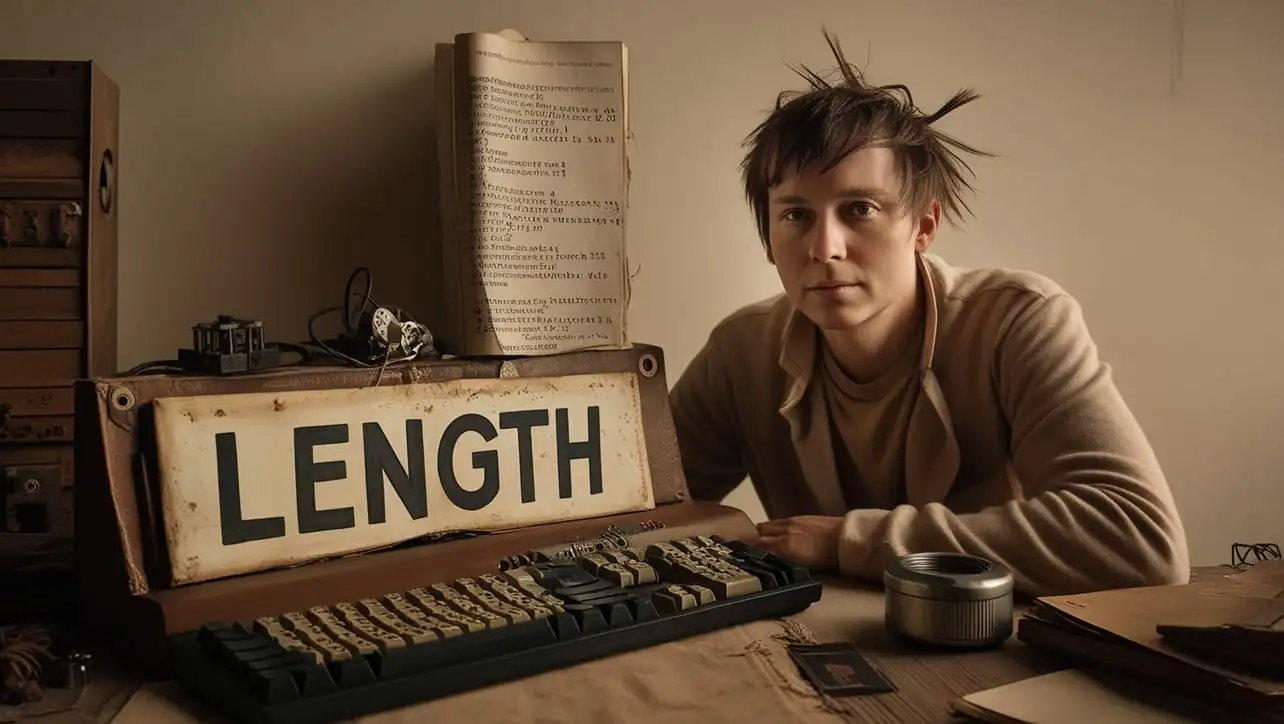
JS Number Methods
JavaScript Number Methods
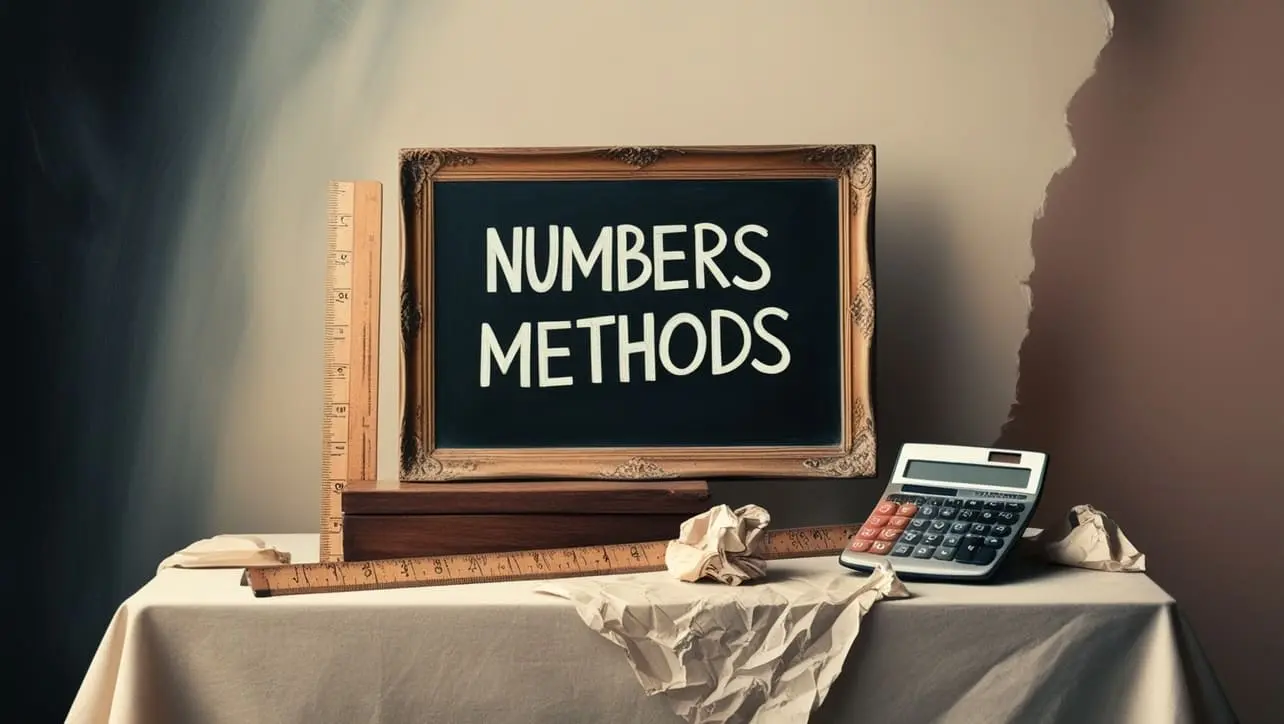
Photo Credit to CodeToFun
🙋 Introduction
JavaScript provides a range of built-in methods for working with numbers. These methods enable you to perform various operations, conversions, and manipulations on numeric values.
In this reference guide, we'll explore some commonly used JavaScript number methods to enhance your proficiency in handling numbers in JavaScript.
📑 Table of Contents
Some of the commonly used number methods in JavaScript includes:
Methods | Explanation |
---|---|
isFinite() | Determines whether a value is a finite number, returning true if it is, and false otherwise. |
isInteger() | Checks if a given value is an integer, returning true if it is and false otherwise. |
isNaN() | Checks whether a value is NaN (Not-a-Number). |
isSafeInteger() | Checks whether a given value is a safe integer, meaning it can be exactly represented as a 53-bit binary number without loss of precision. |
parseFloat() | Parses a string and returns a floating-point number, extracting and converting the numeric portion. |
parseInt() | Converts a string into an integer by parsing it based on a specified radix. |
toExponential() | Converts a number into exponential notation, returning a string representation with a specified number of digits after the decimal point. |
toFixed() | Used to format a number with a specified number of digits after the decimal point. |
toLocaleString() | Used to convert a number into a string using the locale-specific formatting, including the use of commas as thousand separators. |
toPrecision() | Used to format a number to a specified precision, including both the integer and fractional parts. |
toString() | Converts a number to a string, allowing for the representation of numeric values as strings for easier manipulation and display. |
valueOf() | Returns the primitive value of a Number object. |
🚀 Usage Tips
Explore the following tips to make the most out of JavaScript number methods:
Handling NaN:
Use Number.isNaN() to check if a value is NaN, as the standard isNaN() function may produce unexpected results.
Finite Check:
Before performing mathematical operations, use Number.isFinite() to ensure the value is a finite number.
Parsing Numbers:
Use Number.parseInt() and Number.parseFloat() for converting strings to integers and floating-point numbers, respectively.
Number Formatting:
Utilize toExponential(), toFixed(), and toPrecision() for formatting numbers based on your specific requirements.
📝 Example
Let's look at a practical example of using JavaScript number methods to handle and format numeric values:
// Example: Working with JavaScript Number Methods
let exampleNumber = 123.456;
console.log(`Original Number: ${exampleNumber}`);
console.log(`Is NaN? ${Number.isNaN(exampleNumber)}`);
console.log(`Is Finite? ${Number.isFinite(exampleNumber)}`);
console.log(`Parsed Integer: ${Number.parseInt("42")}`);
console.log(`Parsed Float: ${Number.parseFloat("42.5")}`);
console.log(`Exponential Format: ${exampleNumber.toExponential(2)}`);
console.log(`Fixed Decimal Format: ${exampleNumber.toFixed(2)}`);
console.log(`Precision Format: ${exampleNumber.toPrecision(4)}`);
console.log(`Number as String: ${exampleNumber.toString()}`);
console.log(`Value Of: ${exampleNumber.valueOf()}`);
This example demonstrates various JavaScript number methods applied to a numeric value. Experiment with different numbers and methods to enhance your understanding of JavaScript number handling.
🎉 Conclusion
This reference guide provides an overview of essential JavaScript number methods. By incorporating these methods into your code, you can efficiently work with numeric values, perform conversions, and format numbers as needed. Experiment with these methods to discover their versatility and enhance your JavaScript programming skills.
👨💻 Join our Community:
Author
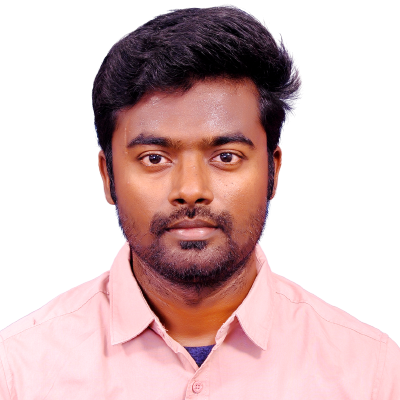
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Number Methods), please comment here. I will help you immediately.