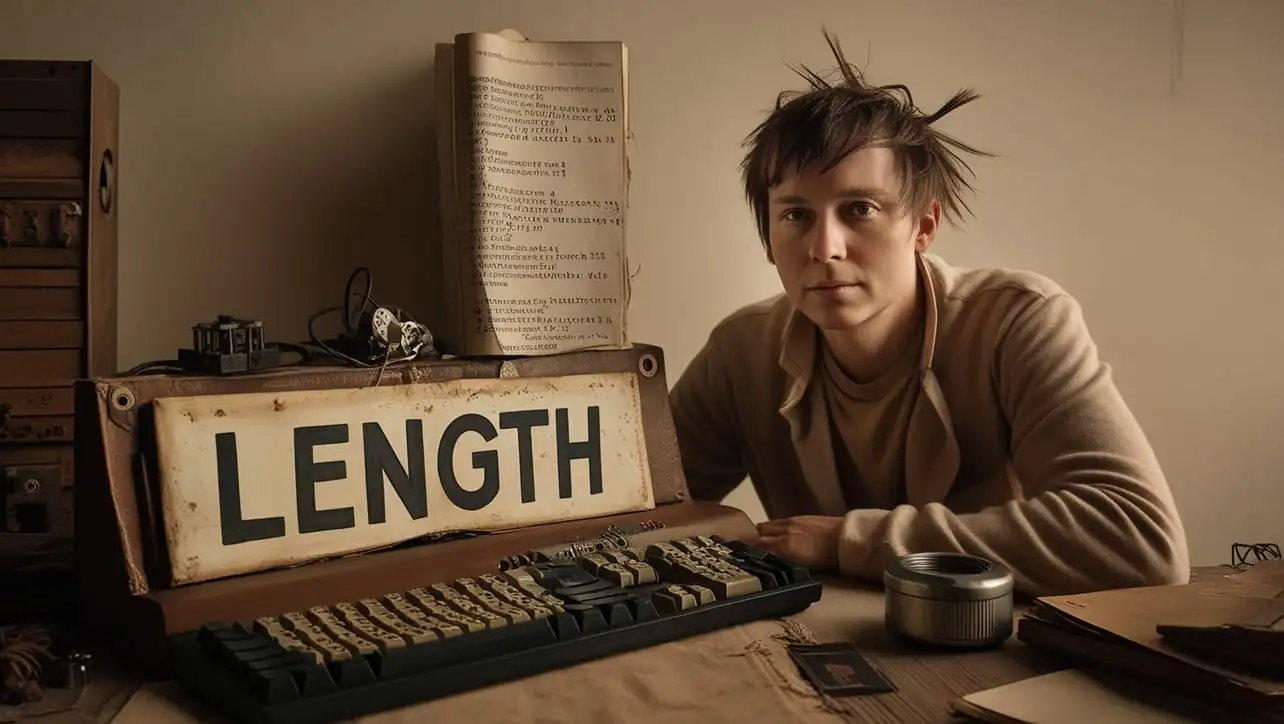
JavaScript Navigator Methods
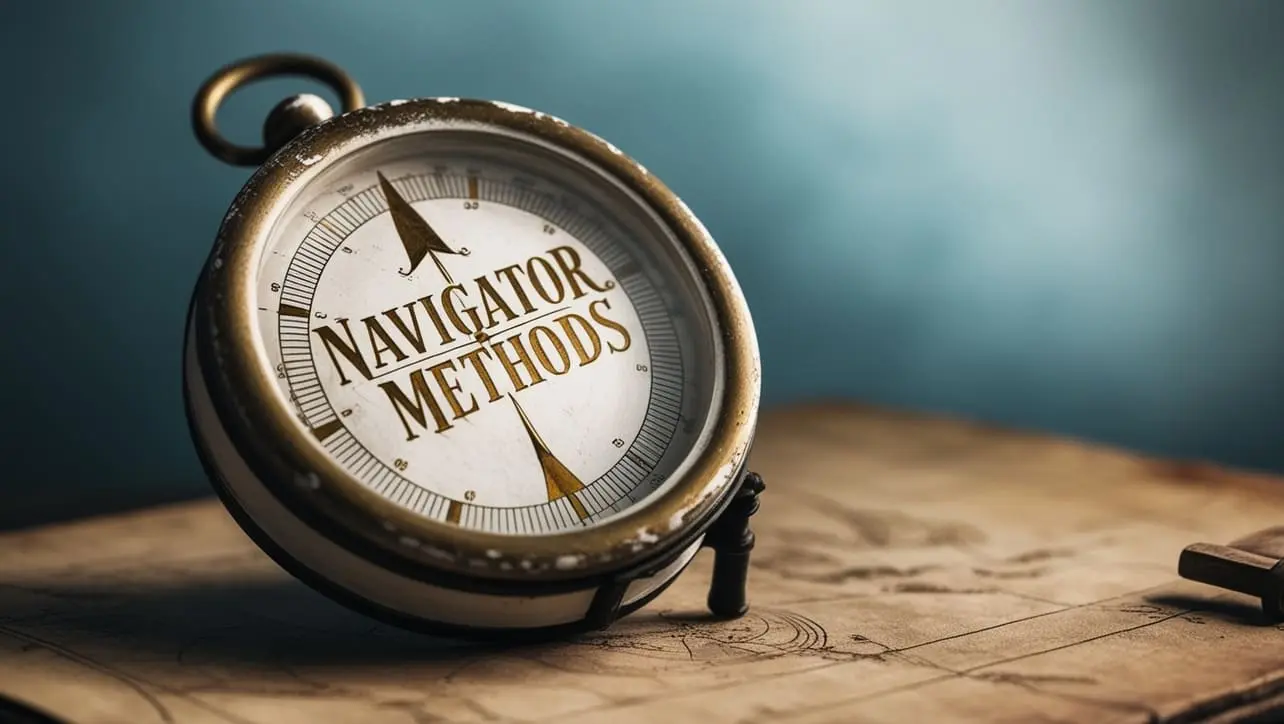
Photo Credit to CodeToFun
Introduction
JavaScript provides various navigator methods that allow developers to access information about the user's browsing environment. These methods offer insights into device capabilities, media access, VR displays, and more.
In this reference guide, we'll briefly explore some essential navigator methods available in JavaScript.
Table of Contents
Some of the commonly used navigator methods in JavaScript includes:
Methods | Explanation |
---|---|
canShare() | Checks if the browser supports the Web Share API, indicating whether sharing functionality is available for a particular data type. |
clearAppBadge() | Used to clear or remove the application badge, typically associated with mobile or browser notifications. |
getAutoplayPolicy() | Retrieves information about the autoplay policy, indicating whether autoplay is allowed or requires user interaction. |
getBattery() | Provides a Battery Status API, allowing developers to retrieve information about the system's battery, including its charge level and charging status. |
getGamepads() | Provides an array of connected gamepad devices, offering a convenient way to access game controller information directly from the browser. |
getInstalledRelatedApps() | Provides information about apps related to the current web app installed on a user's device. |
getUserMedia() | Enables access to a user's camera and microphone, facilitating the capture of audio and video streams in web applications. |
getVRDisplays() | Provides information about connected Virtual Reality (VR) devices, allowing developers to create immersive web experiences. |
javaEnabled() | Determines whether the user's browser has Java support enabled, returning either true or false. |
registerProtocolHandler() | Enables a web application to register itself as a handler for a specific protocol, allowing it to open and handle designated types of URLs. |
requestMediaKeySystemAccess() | Used to request access to a Key System for handling encrypted media, crucial for implementing Digital Rights Management (DRM) in web applications. |
requestMIDIAccess() | Allows web applications to access and interact with MIDI (Musical Instrument Digital Interface) devices, enabling the creation of music and sound-related functionalities on the web. |
sendBeacon() | Allows asynchronous, efficient, and reliable sending of data to a web server in the background, commonly used for analytics or logging purposes. |
setAppBadge() | Allows developers to set a badge on the app icon, providing a visual indicator for notifications or app-specific information. |
share() | Provides a simple way to trigger the native sharing functionality of a device, allowing users to share content seamlessly. |
taintEnabled() | Checks if the taint tracking feature is enabled, allowing developers to determine if tainted canvases can be created. |
unregisterProtocolHandler() | Allows the removal of a registered protocol handler for a specific protocol, providing control over how browsers handle custom protocols. |
vibrate() | Used to initiate device vibration, providing a simple way to offer haptic feedback in web applications. |
Usage Tips
Explore the following tips to make the most out of JavaScript number methods:
Check Browser Compatibility:
Before using any navigator method, ensure it is supported across the browsers you are targeting.
Handle User Permissions:
Methods like getUserMedia require user permission. Implement appropriate handling for user interactions.
Feature Detection:
Use feature detection to gracefully handle scenarios where a method may not be available.
Consult Documentation:
Refer to the official documentation for each method to understand its parameters and behavior.
Example
Here's a basic example demonstrating the use of the navigator.getBattery() method to retrieve battery information:
// Example: Using navigator.getBattery() to get battery information
navigator.getBattery().then(battery => {
console.log(`Battery Level: ${battery.level * 100}%`);
console.log(`Charging Status: ${battery.charging ? 'Charging' : 'Not Charging'}`);
});
Explore similar patterns for other navigator methods to leverage browser-specific functionalities.
Conclusion
This reference guide introduces key navigator methods in JavaScript, offering a glimpse into the capabilities they provide. As you delve deeper into web development, these methods become valuable tools for crafting dynamic and responsive applications.
Join our Community:
Author
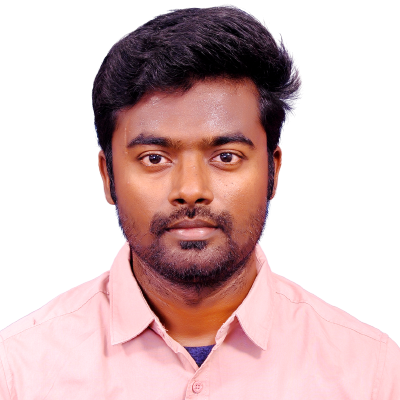
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Navigator Methods), please comment here. I will help you immediately.