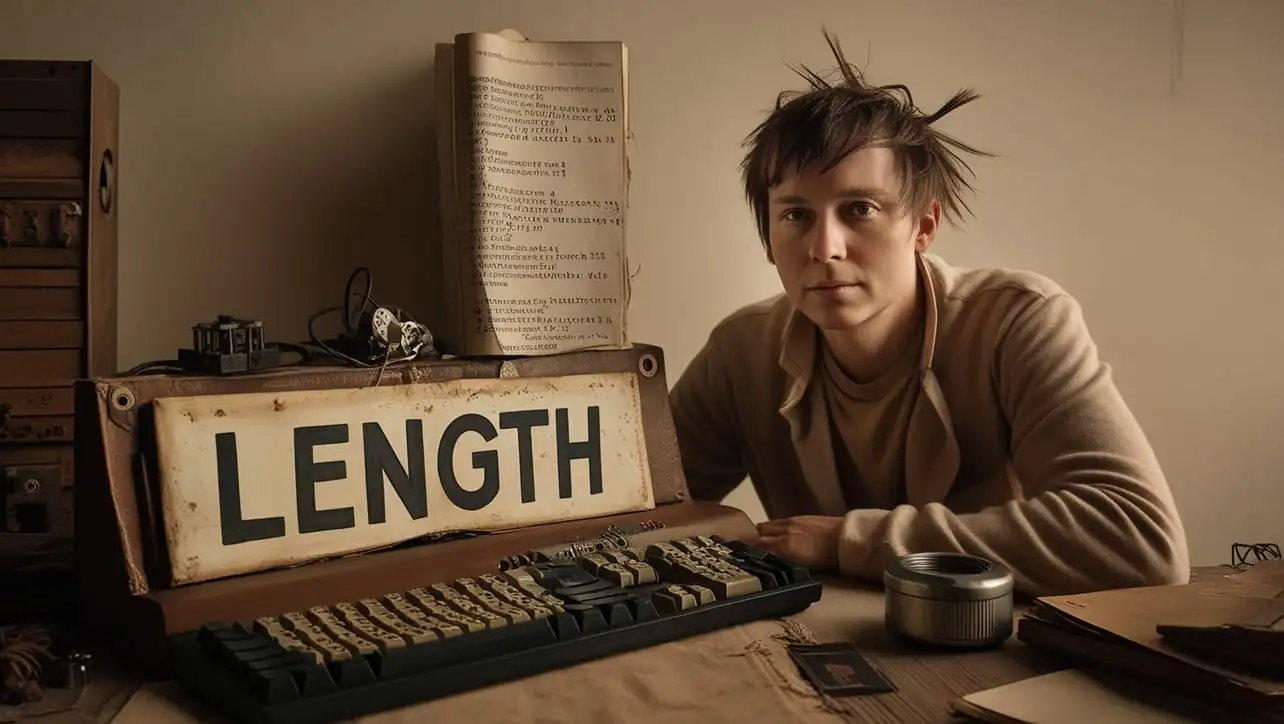
JavaScript Window matchMedia() Method
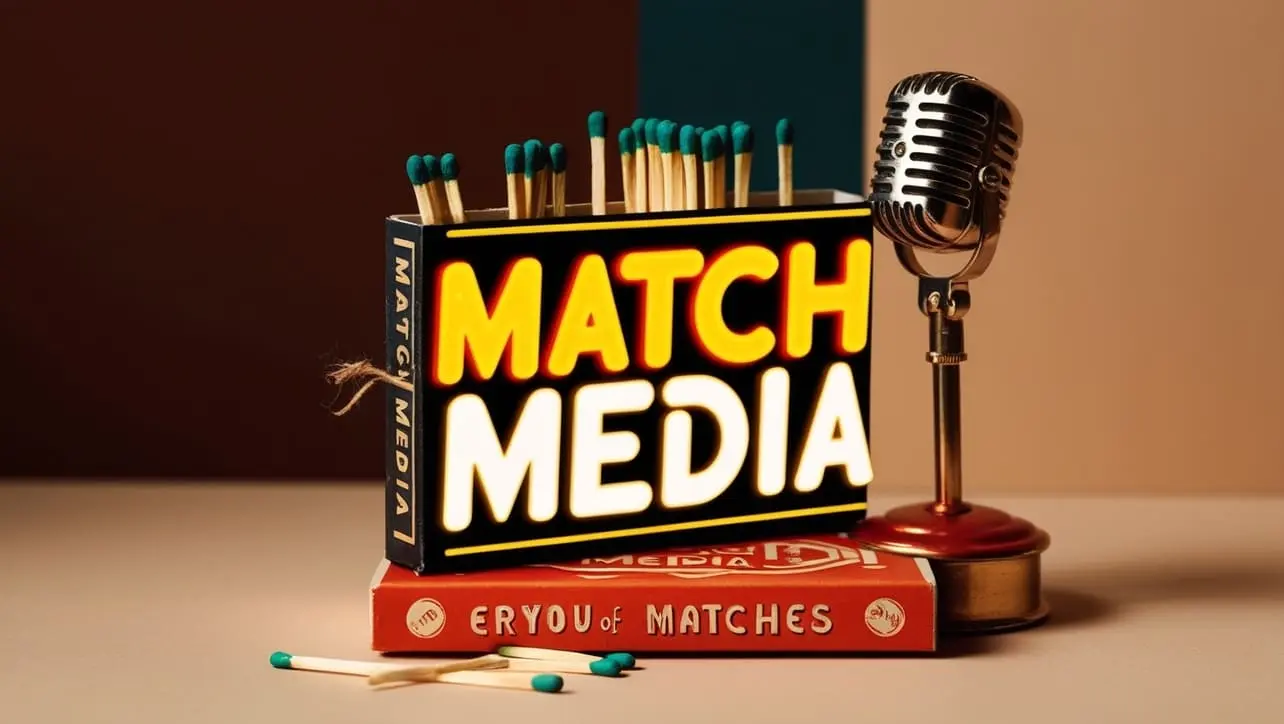
Photo Credit to CodeToFun
🙋 Introduction
The matchMedia()
method is a powerful feature in the Window object of JavaScript that enables responsive web design by providing a way to apply styles or execute scripts based on the result of media queries.
In this guide, we'll dive into the matchMedia()
method, understanding its syntax, usage, best practices, and practical applications.
🧠 Understanding matchMedia() Method
The matchMedia()
method returns a MediaQueryList object representing the results of the specified media query string. This object can be used to check the status of the media query and respond dynamically to changes in the browser's viewport.
💡 Syntax
The syntax for the matchMedia()
method is straightforward:
window.matchMedia(mediaQueryString);
- mediaQueryString: A string representing the media query to be evaluated.
📝 Example
Let's explore a basic example to showcase the usage of the matchMedia()
method:
// Define a media query
const mediaQuery = window.matchMedia('(min-width: 600px)');
// Check if the media query matches
if (mediaQuery.matches) {
console.log('Viewport width is at least 600 pixels.');
} else {
console.log('Viewport width is less than 600 pixels.');
}
In this example, the matchMedia()
method is used to create a MediaQueryList object, and the matches property is checked to determine whether the media query is currently true or false.
🏆 Best Practices
When working with the matchMedia()
method, consider the following best practices:
Use in Conjunction with Event Listeners:
Combine
matchMedia()
with event listeners to respond dynamically to changes in the media query status.example.jsCopiedconst mediaQuery = window.matchMedia('(min-width: 600px)'); // Initial check checkMediaQuery(mediaQuery); // Add a listener for changes mediaQuery.addListener(checkMediaQuery); function checkMediaQuery(query) { if (query.matches) { console.log('Viewport width is at least 600 pixels.'); } else { console.log('Viewport width is less than 600 pixels.'); } }
Support for Complex Media Queries:
Leverage the flexibility of media queries to handle complex conditions and adapt your styles or scripts accordingly.
📚 Use Cases
Responsive Design:
The primary use case for the
matchMedia()
method is creating responsive designs that adapt to different viewport sizes:example.jsCopiedconst mediaQuery = window.matchMedia('(max-width: 768px)'); if (mediaQuery.matches) { // Apply styles or scripts for small screens console.log('Adjusting for small screens.'); } else { // Apply styles or scripts for larger screens console.log('Adjusting for larger screens.'); }
Dark Mode Toggle:
Utilize
matchMedia()
to create a dark mode toggle based on the user's preference:example.jsCopiedconst darkModeQuery = window.matchMedia('(prefers-color-scheme: dark)'); if (darkModeQuery.matches) { // Enable dark mode console.log('Dark mode is enabled.'); } else { // Use default light mode console.log('Using default light mode.'); }
🎉 Conclusion
The matchMedia()
method empowers developers to create responsive and dynamic web designs by programmatically adapting to different viewport conditions.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the matchMedia()
method in your JavaScript projects.
👨💻 Join our Community:
Author
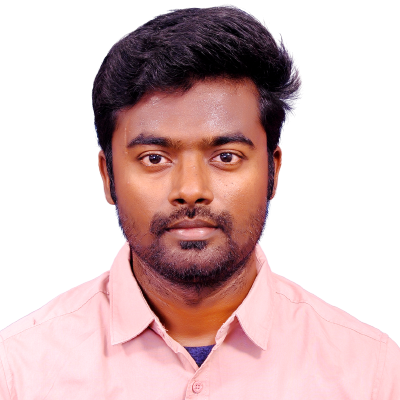
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Window matchMedia() Method), please comment here. I will help you immediately.