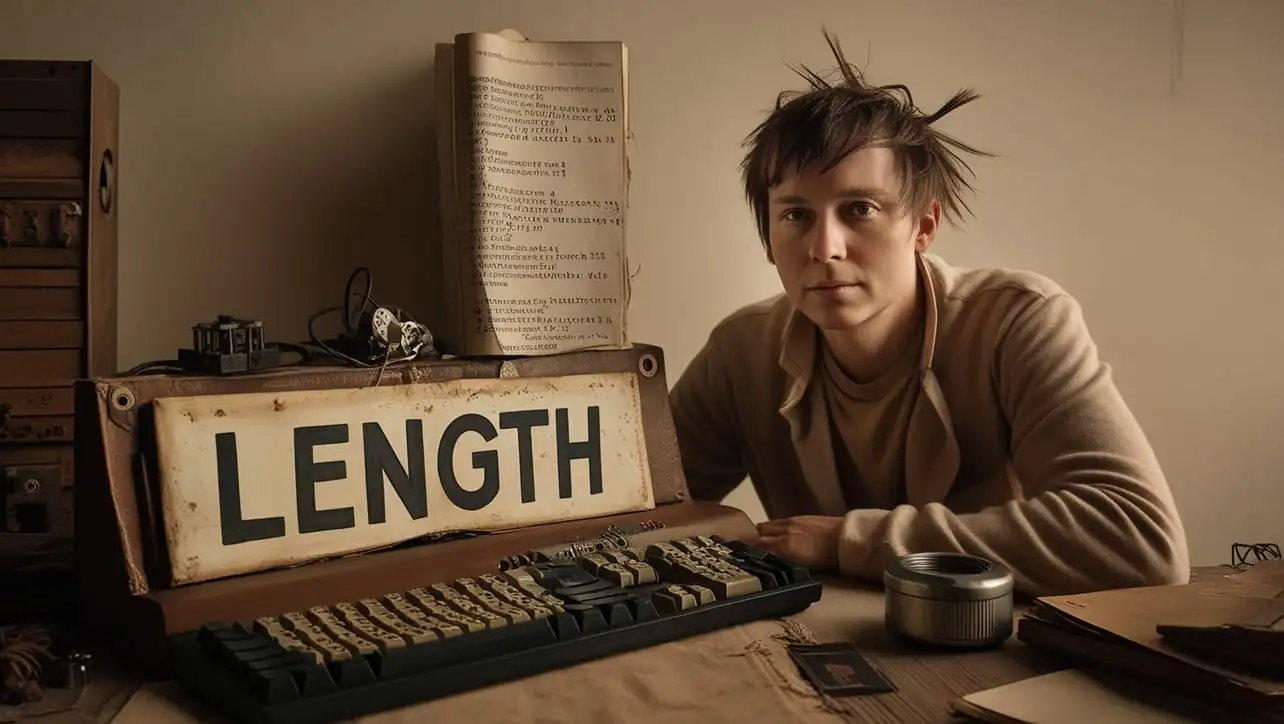
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- JS Console Methods
- JS Date Methods
- getDate()
- getDay()
- getFullYear()
- getHours()
- getMilliseconds()
- getMinutes()
- getMonth()
- getSeconds()
- getTime()
- getTimezoneOffset()
- getUTCDate()
- getUTCDay()
- getUTCFullYear()
- getUTCHours()
- getUTCMilliseconds()
- getUTCMinutes()
- getUTCMonth()
- getUTCSeconds()
- now()
- parse()
- setDate()
- setFullYear()
- setHours()
- setMilliseconds()
- setMinutes()
- setMonth()
- setSeconds()
- setTime()
- setUTCDate()
- setUTCFullYear()
- setUTCHours()
- setUTCMilliseconds()
- setUTCMinutes()
- setUTCMonth()
- setUTCSeconds()
- toDateString()
- toISOString()
- toJSON()
- toLocaleDateString()
- toLocaleString()
- toLocaleTimeString()
- toString()
- toTimeString()
- toUTCString()
- UTC()
- valueOf()
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Date UTC() Method
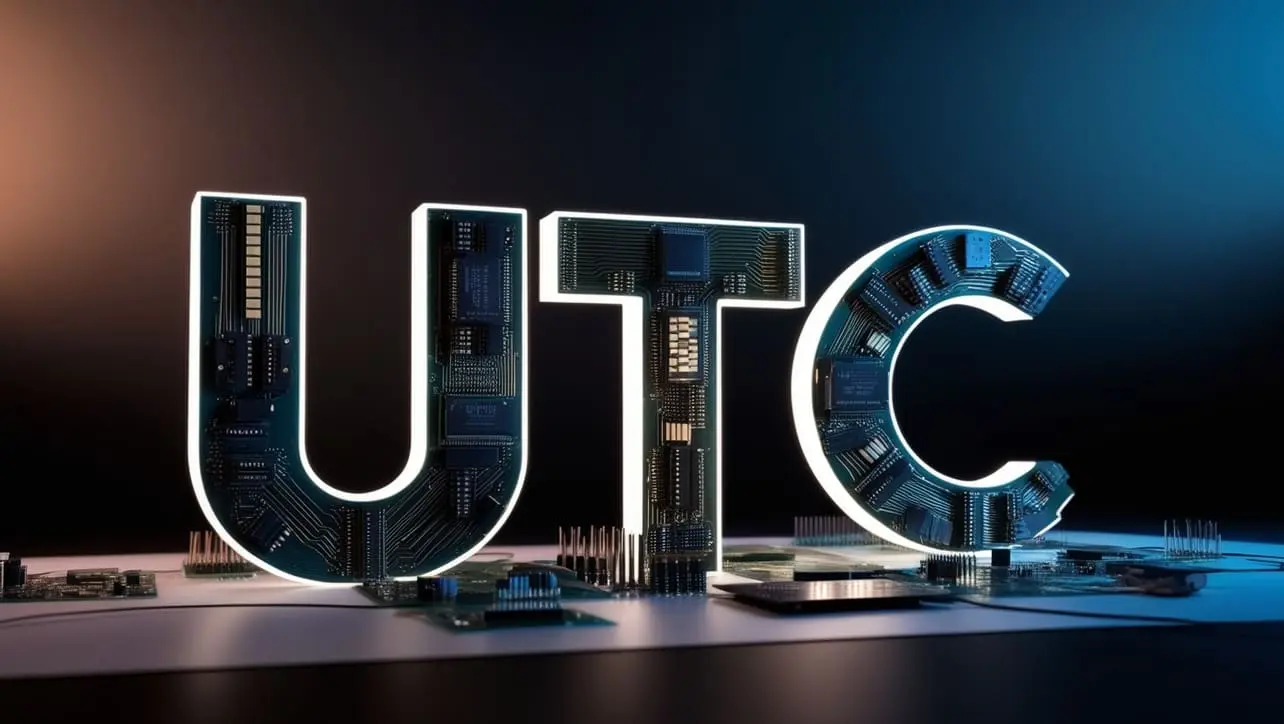
Photo Credit to CodeToFun
🙋 Introduction
Working with dates and times in JavaScript is made seamless with the Date object, and the UTC()
method is a valuable addition for managing Coordinated Universal Time (UTC).
In this guide, we'll explore the Date UTC()
method, understanding its syntax, usage, best practices, and practical applications.
🧠 Understanding UTC() Method
The UTC()
method in the Date object allows you to create a new date by specifying the UTC time. Unlike other Date methods that use the local time zone, UTC()
deals explicitly with Coordinated Universal Time, providing a standardized representation of time.
💡 Syntax
The syntax for the UTC()
method is straightforward:
Date.UTC(year, month[, day[, hour[, minute[, second[, millisecond]]]]]);
- year: The full four-digit year.
- month: An integer representing the month (0 for January, 11 for December).
- day (Optional): An integer representing the day of the month.
- hour (Optional): An integer representing the hour (0 to 23).
- minute (Optional): An integer representing the minute (0 to 59).
- second (Optional): An integer representing the second (0 to 59).
- millisecond (Optional): An integer representing the millisecond (0 to 999).
📝 Example
Let's dive into an example to illustrate the usage of the UTC()
method:
// Creating a date for January 1, 2023, 12:30:45 UTC
const utcDate = new Date(Date.UTC(2023, 0, 1, 12, 30, 45));
console.log(utcDate.toUTCString());
In this example, we use Date.UTC()
to create a Date object representing January 1, 2023, 12:30:45 UTC.
🏆 Best Practices
When working with the UTC()
method, consider the following best practices:
Consistent Input:
Provide consistent input values for accurate representation, and be mindful of the zero-based month index.
example.jsCopied// Correct: March 1, 2023 const correctUTCDate = new Date(Date.UTC(2023, 2, 1)); // Incorrect: March 1, 2023 (month index 3 does not exist) const incorrectUTCDate = new Date(Date.UTC(2023, 3, 1));
Time Component Omission:
If not needed, you can omit the day and time components, and they will default to the earliest possible values.
example.jsCopied// UTC midnight on January 1, 2023 const midnightUTC = new Date(Date.UTC(2023, 0));
📚 Use Cases
Comparing Dates:
The
UTC()
method is useful when comparing dates to ensure a consistent time zone, especially in scenarios where time zone differences may lead to unexpected results.example.jsCopiedconst currentDate = new Date(); const futureUTCDate = new Date(Date.UTC(2025, 5, 1)); if (currentDate < futureUTCDate) { console.log('The future is ahead!'); }
Calculating Time Differences:
When calculating time differences between dates, using the
UTC()
method helps in avoiding discrepancies caused by daylight saving time changes.example.jsCopiedconst startDate = new Date(Date.UTC(2023, 0, 1)); const endDate = new Date(Date.UTC(2023, 6, 1)); const timeDifference = endDate - startDate; console.log(`Time difference: ${timeDifference} milliseconds`);
🎉 Conclusion
The Date UTC()
method proves to be an essential tool for working with dates and times in a standardized and consistent manner.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the UTC()
method in your JavaScript projects.
👨💻 Join our Community:
Author
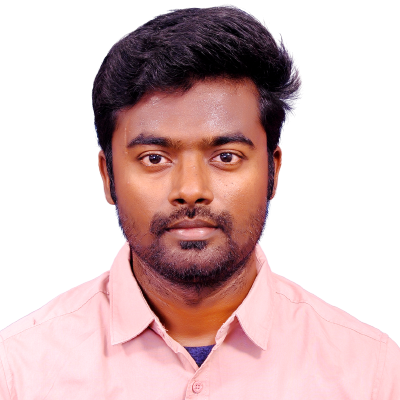
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date UTC() Method), please comment here. I will help you immediately.