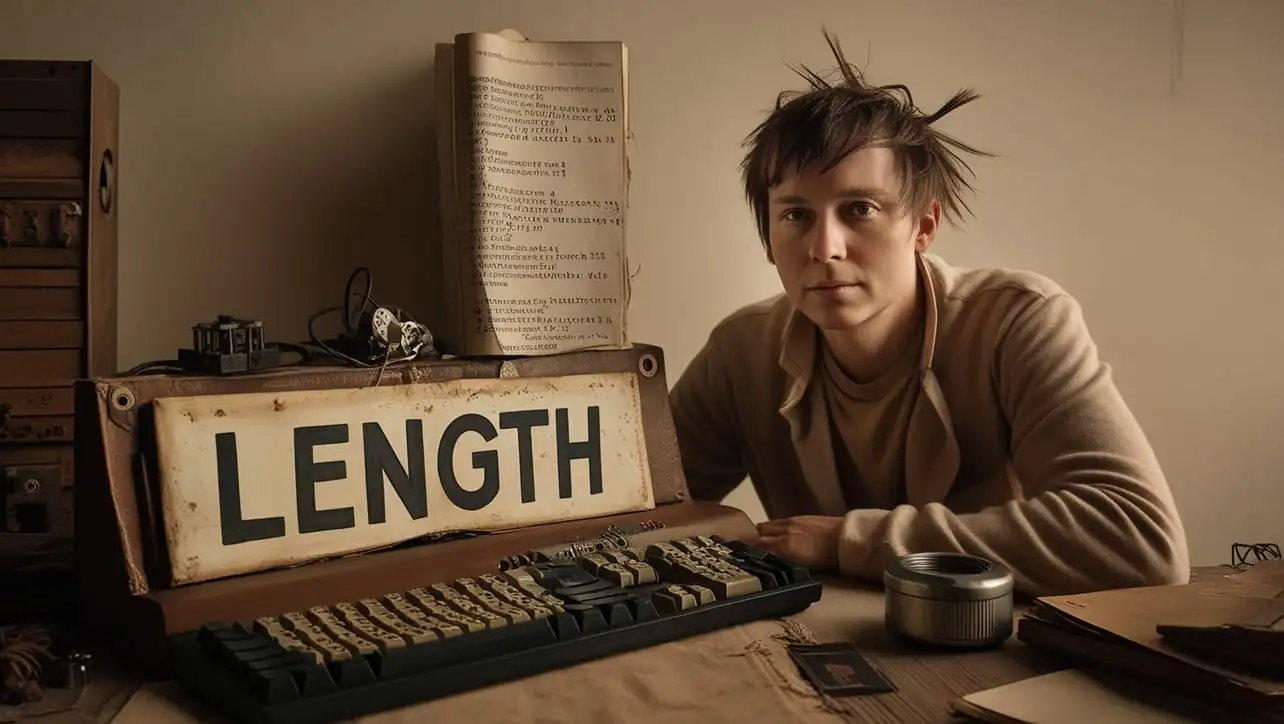
JS Date Methods
JavaScript Date toTimeString() Method
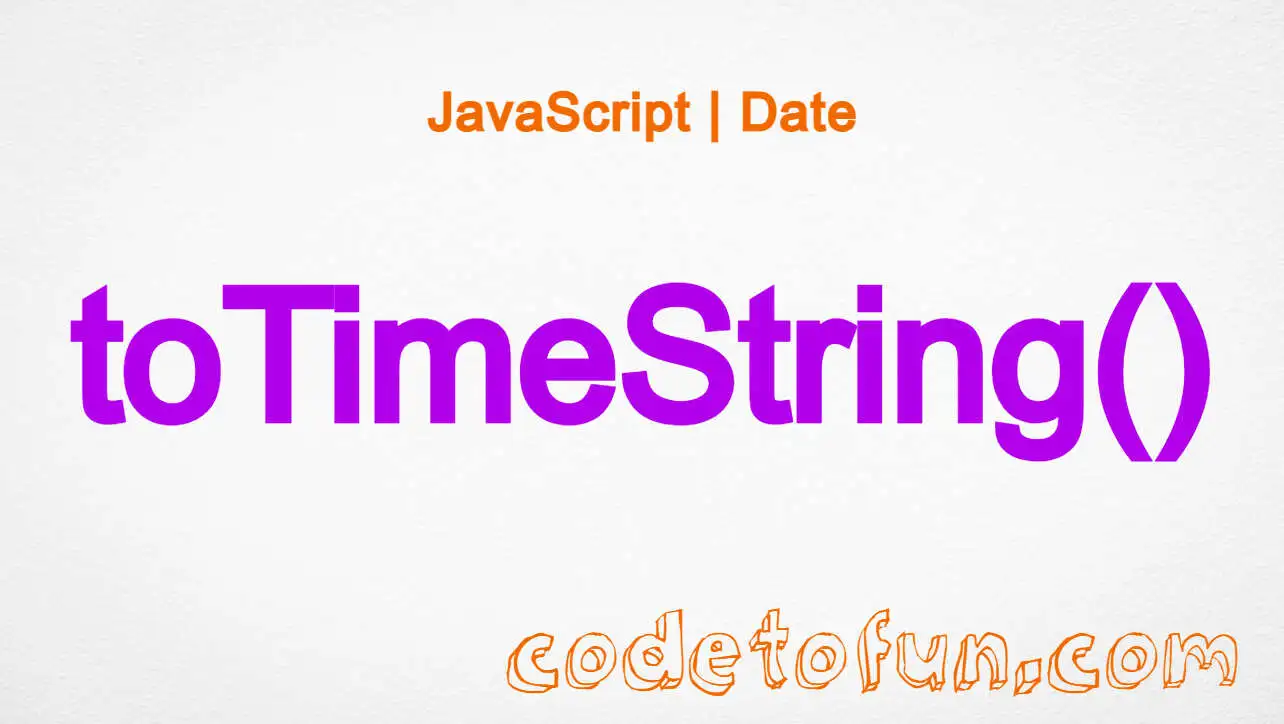
Photo Credit to CodeToFun
🙋 Introduction
Handling dates and times in JavaScript becomes more manageable with various methods available, and the toTimeString()
method is a valuable addition to this toolkit. This method is specifically designed for Date objects, providing a convenient way to extract the time portion in a human-readable format.
In this guide, we'll explore the toTimeString()
method, examining its syntax, example usage, best practices, and practical use cases.
🧠 Understanding toTimeString() Method
The toTimeString()
method is used to convert the time portion of a Date object into a string. This method returns a string representation of the time in the format "hh:mm:ss GMT+xxxx" (the timezone offset may vary).
💡 Syntax
The syntax for the toTimeString()
method is straightforward:
dateObject.toTimeString();
- dateObject: The Date object for which you want to obtain the time string.
📝 Example
Let's dive into a simple example to illustrate the usage of the toTimeString()
method:
// Creating a Date object
const currentDate = new Date();
// Using toTimeString() to get the time string
const timeString = currentDate.toTimeString();
console.log(timeString);
In this example, the toTimeString()
method is applied to a Date object (currentDate), and the resulting time string is then logged to the console.
🏆 Best Practices
When working with the toTimeString()
method, consider the following best practices:
Locale Considerations:
Be aware that
toTimeString()
returns a string representation in the default locale. If you need more control over the formatting, consider using libraries like Intl.DateTimeFormat for localization.example.jsCopiedconst options = { hour: '2-digit', minute: '2-digit', second: '2-digit' }; const formattedTime = new Intl.DateTimeFormat('en-US', options).format(currentDate); console.log(formattedTime);
Timezone Awareness:
Be mindful of the timezone information included in the string. If you require UTC or a specific timezone, consider using methods like toUTCString() or toLocaleTimeString() with appropriate options.
📚 Use Cases
Displaying Current Time:
The
toTimeString()
method is particularly useful when you want to display the current time in a user-friendly format:example.jsCopiedconst currentTimeString = new Date().toTimeString(); console.log(`Current Time: ${currentTimeString}`);
Formatting Time for UI Presentation:
When working with user interfaces, you can utilize the
toTimeString()
method to format time for display:example.jsCopiedconst eventTime = new Date('2024-03-01T18:30:00'); const formattedEventTime = eventTime.toTimeString(); console.log(`Event Time: ${formattedEventTime}`);
🎉 Conclusion
The toTimeString()
method in JavaScript provides a straightforward way to obtain the time portion of a Date object as a string.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the toTimeString()
method in your JavaScript projects.
👨💻 Join our Community:
Author
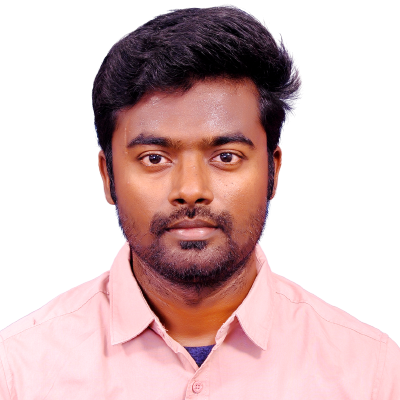
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date toTimeString() Method), please comment here. I will help you immediately.