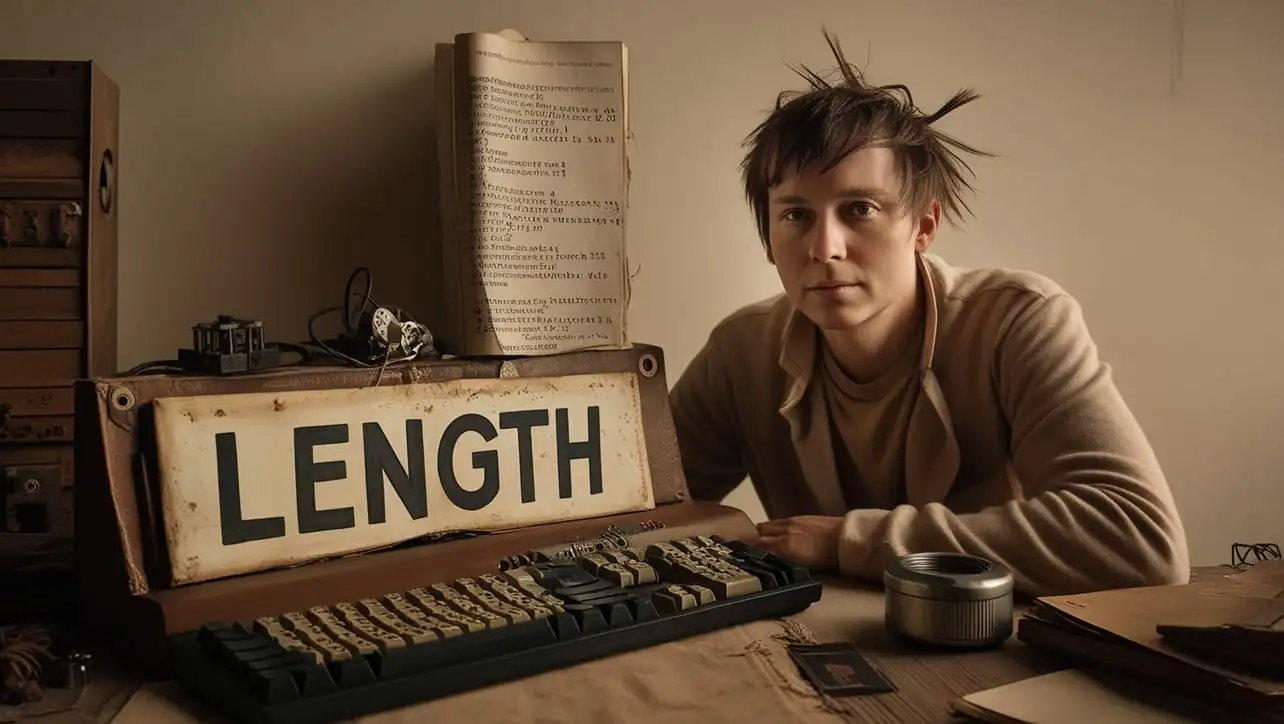
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- JS Console Methods
- JS Date Methods
- getDate()
- getDay()
- getFullYear()
- getHours()
- getMilliseconds()
- getMinutes()
- getMonth()
- getSeconds()
- getTime()
- getTimezoneOffset()
- getUTCDate()
- getUTCDay()
- getUTCFullYear()
- getUTCHours()
- getUTCMilliseconds()
- getUTCMinutes()
- getUTCMonth()
- getUTCSeconds()
- now()
- parse()
- setDate()
- setFullYear()
- setHours()
- setMilliseconds()
- setMinutes()
- setMonth()
- setSeconds()
- setTime()
- setUTCDate()
- setUTCFullYear()
- setUTCHours()
- setUTCMilliseconds()
- setUTCMinutes()
- setUTCMonth()
- setUTCSeconds()
- toDateString()
- toISOString()
- toJSON()
- toLocaleDateString()
- toLocaleString()
- toLocaleTimeString()
- toString()
- toTimeString()
- toUTCString()
- UTC()
- valueOf()
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Date toString() Method
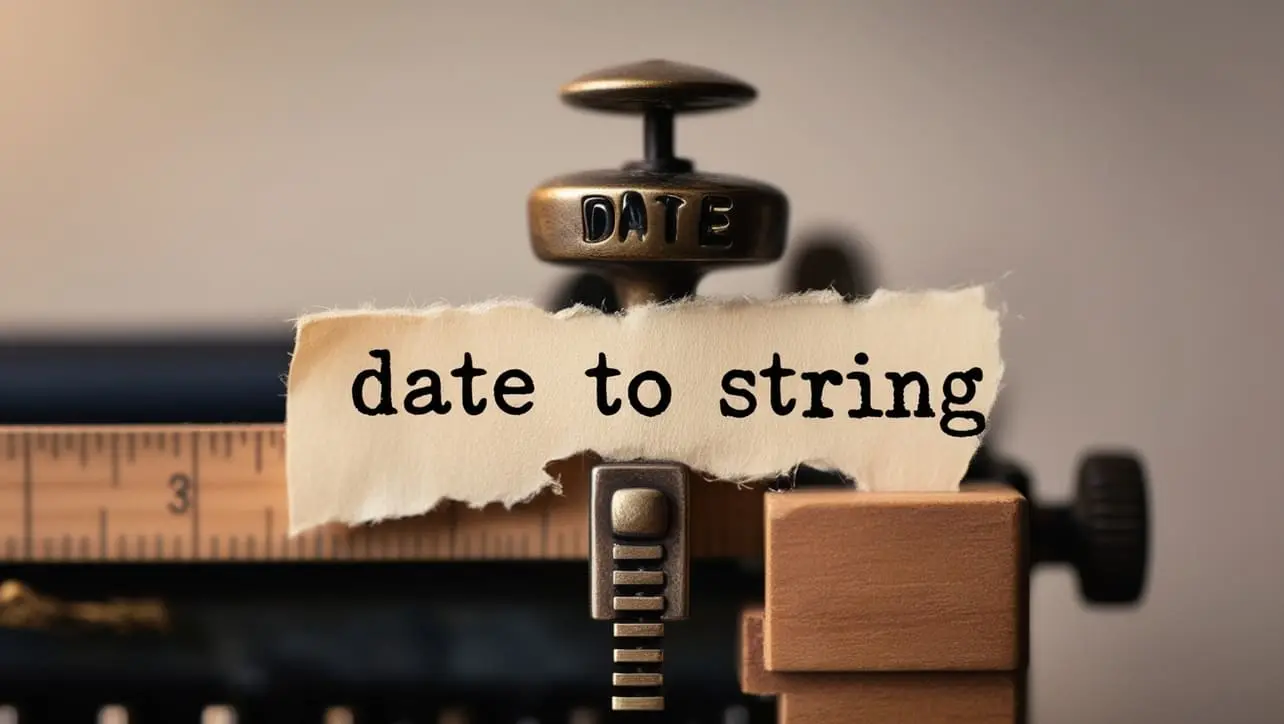
Photo Credit to CodeToFun
Introduction
Working with dates in JavaScript becomes more manageable with the toString()
method, allowing you to obtain a human-readable representation of a Date object.
In this guide, we'll explore the toString()
method, understand its syntax, usage, best practices, and dive into practical examples.
Understanding toString() Method
The toString()
method is a fundamental feature of the Date object in JavaScript. It returns a string representation of the date and time, making it convenient for debugging, logging, or displaying dates to users.
Syntax
The syntax for the toString()
method is straightforward:
date.toString();
- date: The Date object for which you want to obtain the string representation.
Example
Let's look at a simple example to illustrate the usage of the toString()
method:
// Creating a Date object for the current date and time
const currentDate = new Date();
// Using toString() to get a string representation
const dateString = currentDate.toString();
console.log(dateString);
In this example, the toString()
method is applied to a Date object to obtain a string representation of the current date and time.
Best Practices
When working with the toString()
method, consider the following best practices:
Locale Considerations:
Be aware that the string representation returned by
toString()
is implementation-dependent and may vary across different JavaScript engines. For locale-specific representations, consider using the toLocaleString() method.example.jsCopiedconst localizedDateString = currentDate.toLocaleString(); console.log(localizedDateString);
Timezone Awareness:
Keep in mind that
toString()
returns the date and time in the local timezone of the user's browser. If timezone information is crucial, explore options like toISOString() or libraries like moment.js.Formatting Libraries:
For more advanced formatting needs, consider using formatting libraries such as date-fns or Luxon to achieve consistent and customizable date representations.
Use Cases
Logging Current Date:
The
toString()
method is useful for logging the current date and time:example.jsCopiedconst currentDate = new Date(); console.log(currentDate.toString());
Displaying Last Modified Date:
You can utilize the
toString()
method to display the last modified date of a document:example.jsCopiedconst lastModifiedDate = new Date(document.lastModified); console.log(`Last Modified: ${lastModifiedDate.toString()}`);
Conclusion
The toString()
method provides a straightforward way to obtain a human-readable string representation of Date objects in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the toString()
method in your JavaScript projects.
Join our Community:
Author
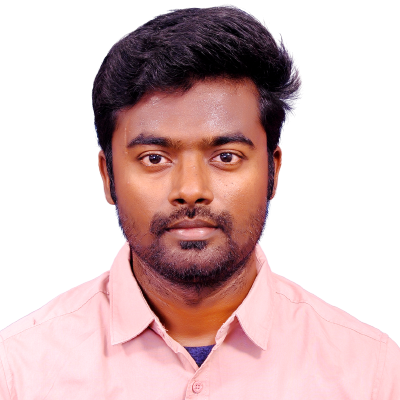
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date toString() Method), please comment here. I will help you immediately.