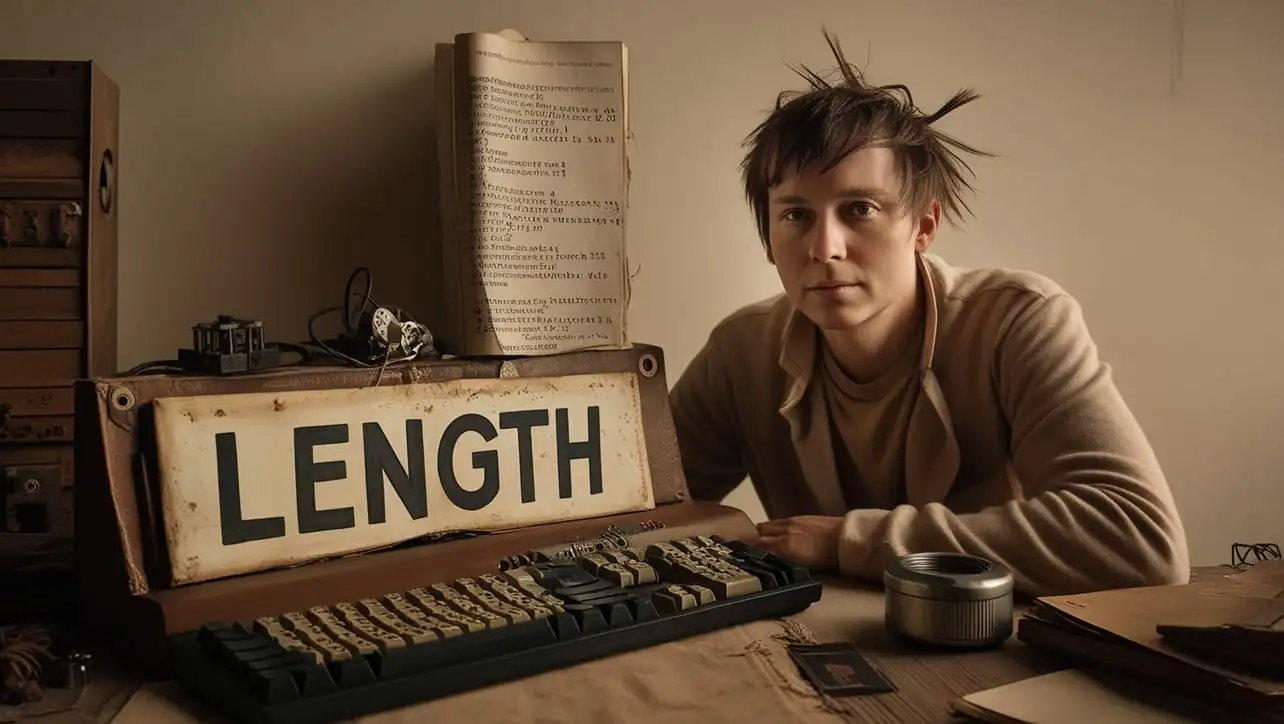
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- JS Console Methods
- JS Date Methods
- getDate()
- getDay()
- getFullYear()
- getHours()
- getMilliseconds()
- getMinutes()
- getMonth()
- getSeconds()
- getTime()
- getTimezoneOffset()
- getUTCDate()
- getUTCDay()
- getUTCFullYear()
- getUTCHours()
- getUTCMilliseconds()
- getUTCMinutes()
- getUTCMonth()
- getUTCSeconds()
- now()
- parse()
- setDate()
- setFullYear()
- setHours()
- setMilliseconds()
- setMinutes()
- setMonth()
- setSeconds()
- setTime()
- setUTCDate()
- setUTCFullYear()
- setUTCHours()
- setUTCMilliseconds()
- setUTCMinutes()
- setUTCMonth()
- setUTCSeconds()
- toDateString()
- toISOString()
- toJSON()
- toLocaleDateString()
- toLocaleString()
- toLocaleTimeString()
- toString()
- toTimeString()
- toUTCString()
- UTC()
- valueOf()
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Date toISOString() Method
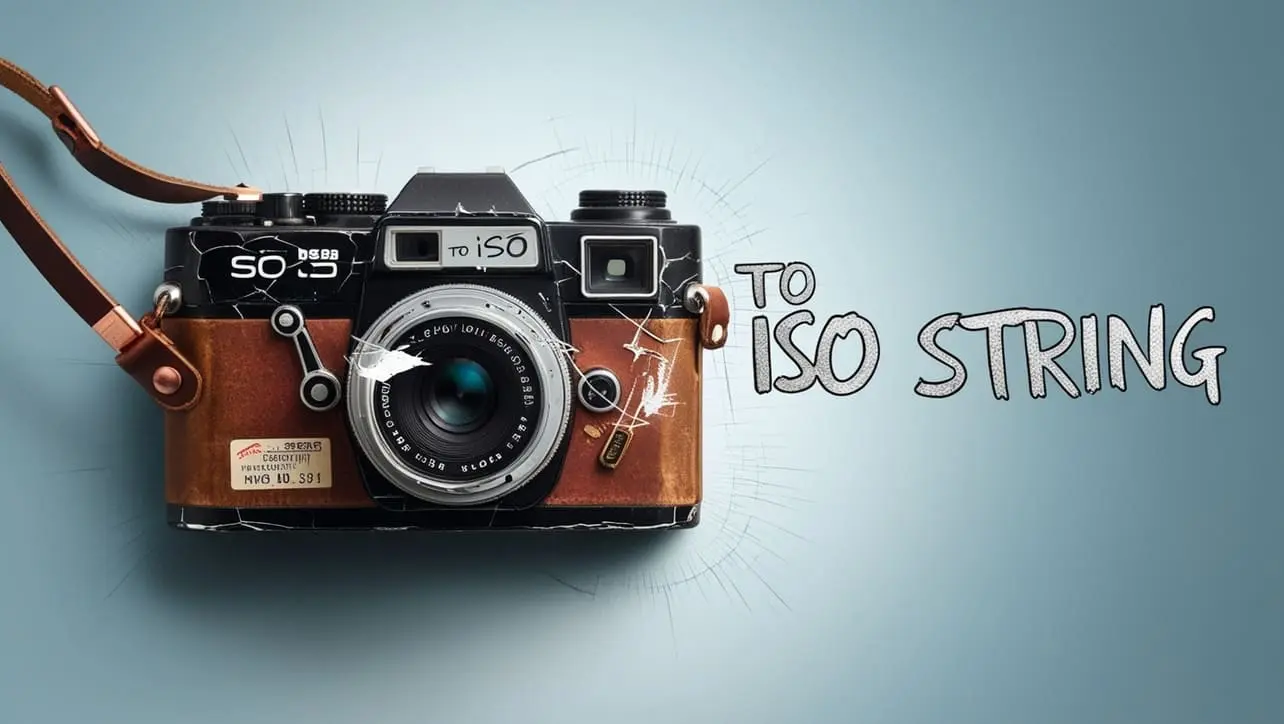
Photo Credit to CodeToFun
Introduction
Handling dates in JavaScript is a common requirement, and the toISOString()
method for Date objects provides a powerful tool for converting date values to a standardized string representation.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical applications of the toISOString()
method.
Understanding toISOString() Method
The toISOString()
method is a built-in function in JavaScript that belongs to the Date object prototype. Its primary purpose is to convert a Date object into a string following the ISO 8601 format, which is widely accepted and used for representing dates and times.
Syntax
The syntax for the toISOString()
method is straightforward:
date.toISOString();
- date: The Date object you want to convert to a string.
Example
Let's look at a basic example to understand how to use the toISOString()
method:
// Create a Date object
const currentDate = new Date();
// Convert the Date object to ISO string
const isoString = currentDate.toISOString();
console.log(isoString);
In this example, toISOString()
is called on a Date object, resulting in a string representing the current date and time in the ISO 8601 format.
Best Practices
When working with the toISOString()
method, consider the following best practices:
Timezone Awareness:
Be aware that the
toISOString()
method returns a string in Coordinated Universal Time (UTC). If you need to work with local time, additional steps may be required.example.jsCopiedconst localISOString = currentDate.toLocaleString(); console.log(localISOString);
Error Handling:
Always handle potential errors when working with Date objects to ensure your code is robust.
example.jsCopiedif (isNaN(currentDate)) { console.error('Invalid date!'); } else { const isoString = currentDate.toISOString(); console.log(isoString); }
Compatibility Checking:
Confirm that the
toISOString()
method is supported in your target environments, especially if you need to cater to older browsers.example.jsCopied// Check if toISOString() method is supported if (Date.prototype.toISOString) { const isoString = currentDate.toISOString(); console.log(isoString); } else { console.error('toISOString() method not supported in this environment.'); }
Use Cases
Sending Dates in API Requests:
The
toISOString()
method is particularly useful when dealing with APIs that expect date values in a standardized format. For example:example.jsCopiedconst payload = { eventName: 'Meeting', eventDate: currentDate.toISOString(), }; // Send payload to the API
Logging Timestamps:
When logging timestamps in your application, the
toISOString()
method ensures a consistent and easily readable format:example.jsCopiedconsole.log(`[${currentDate.toISOString()}] Application started.`);
Conclusion
The toISOString()
method for Date objects in JavaScript is a valuable asset for working with dates and times.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the toISOString()
method in your JavaScript projects.
Join our Community:
Author
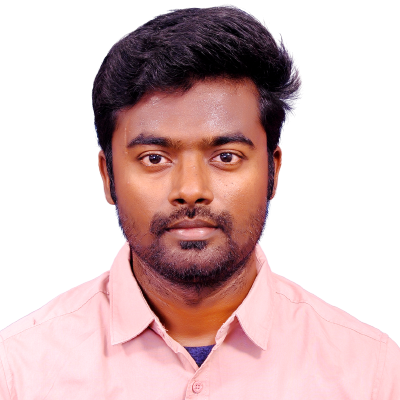
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date toISOString() Method), please comment here. I will help you immediately.