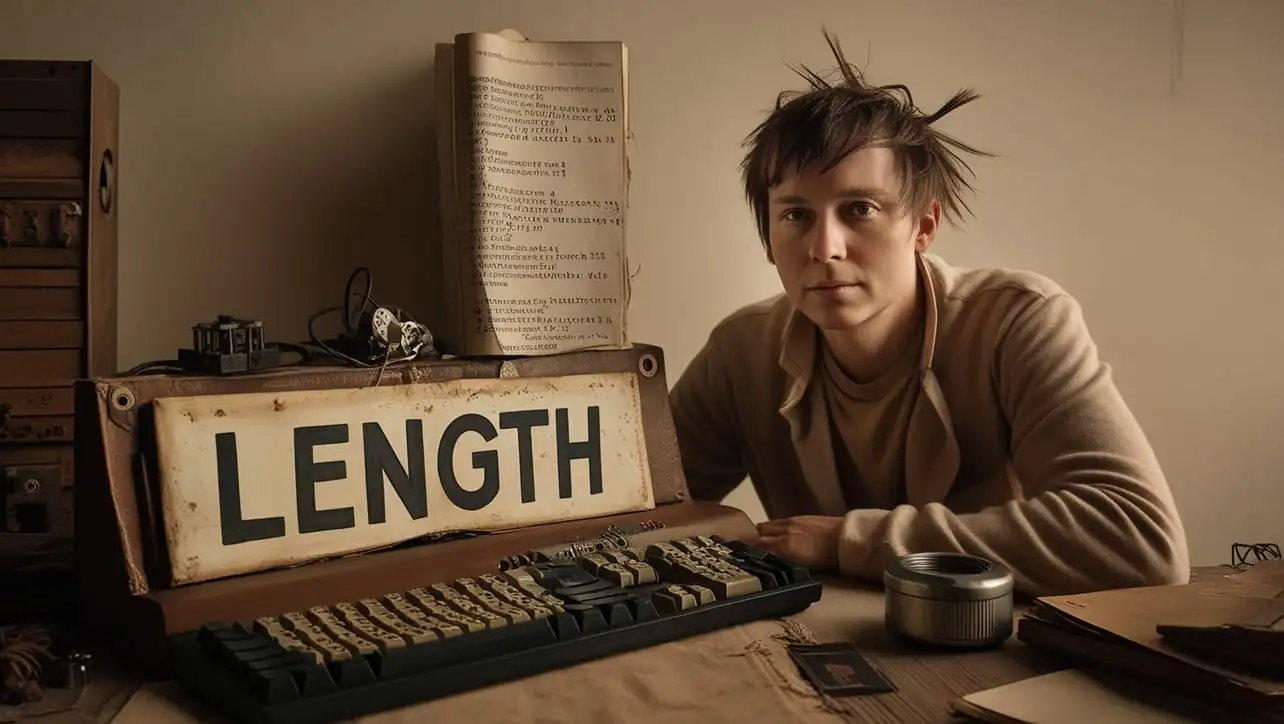
JS Date Methods
JavaScript Date setUTCHours() Method

Photo Credit to CodeToFun
🙋 Introduction
Working with dates and times is a crucial aspect of programming, and JavaScript provides a rich set of methods to manipulate them. The setUTCHours()
method is particularly useful for setting the hours of a Date object in Coordinated Universal Time (UTC).
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical applications of the setUTCHours()
method.
🧠 Understanding setUTCHours() Method
The setUTCHours()
method is part of the Date object in JavaScript, allowing you to set the hours of a date object in UTC time. This method takes up to four parameters: hours, minutes, seconds, and milliseconds. By default, it modifies the date in place but also returns the updated timestamp.
💡 Syntax
The syntax for the setUTCHours()
method is straightforward:
date.setUTCHours(hours, minutes, seconds, milliseconds);
- date: The Date object you want to modify.
- hours: The numeric value representing the hour (0 to 23).
- minutes (Optional): The numeric value representing the minutes (0 to 59).
- seconds (Optional): The numeric value representing the seconds (0 to 59).
- milliseconds (Optional): The numeric value representing the milliseconds (0 to 999).
📝 Example
Let's dive into an example to illustrate the usage of the setUTCHours()
method:
// Create a Date object
const currentDate = new Date();
// Set the hours to 10, minutes to 30, and seconds to 0 in UTC
currentDate.setUTCHours(10, 30, 0);
console.log(currentDate.toISOString());
In this example, the setUTCHours()
method is used to set the hours, minutes, and seconds of the currentDate object to 10, 30, and 0 in UTC time, respectively.
🏆 Best Practices
When working with the setUTCHours()
method, consider the following best practices:
Use toISOString() for Logging:
When logging or displaying the updated date, consider using the toISOString() method to get a standardized string representation in UTC.
example.jsCopiedconsole.log(currentDate.toISOString());
Validate Parameters:
Ensure that the values passed as parameters are within valid ranges to prevent unexpected behavior.
example.jsCopiedfunction setCustomTime(date, hours, minutes) { if (hours >= 0 && hours <= 23 && minutes >= 0 && minutes <= 59) { date.setUTCHours(hours, minutes); } else { console.error('Invalid hours or minutes provided.'); } }
Understand UTC and Local Time:
Be aware that
setUTCHours()
modifies the date in UTC, so take into account the time zone differences if working with local time.
📚 Use Cases
Scheduling Events in Different Time Zones:
The
setUTCHours()
method is invaluable when working with events scheduled across different time zones. By setting the hours in UTC, you ensure consistency regardless of the local time zone.example.jsCopiedconst eventDate = new Date(); eventDate.setUTCHours(15, 0, 0); // Set the event time to 3:00 PM UTC
Time Calculations and Adjustments:
Performing time calculations and adjustments becomes more manageable with the precision offered by the
setUTCHours()
method. For instance, adjusting a date by adding two hours:example.jsCopiedconst now = new Date(); now.setUTCHours(now.getUTCHours() + 2); // Add 2 hours to the current UTC time
🎉 Conclusion
The setUTCHours()
method in JavaScript empowers developers to precisely manipulate hours within Date objects in a UTC context.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the setUTCHours()
method in your JavaScript projects.
👨💻 Join our Community:
Author
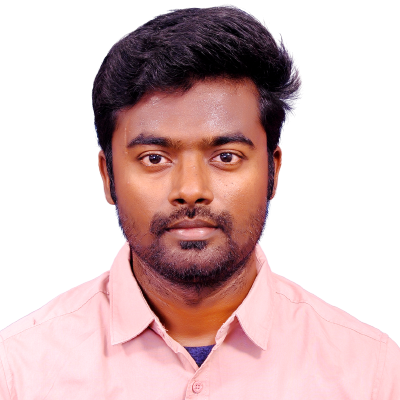
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date setUTCHours() Method), please comment here. I will help you immediately.