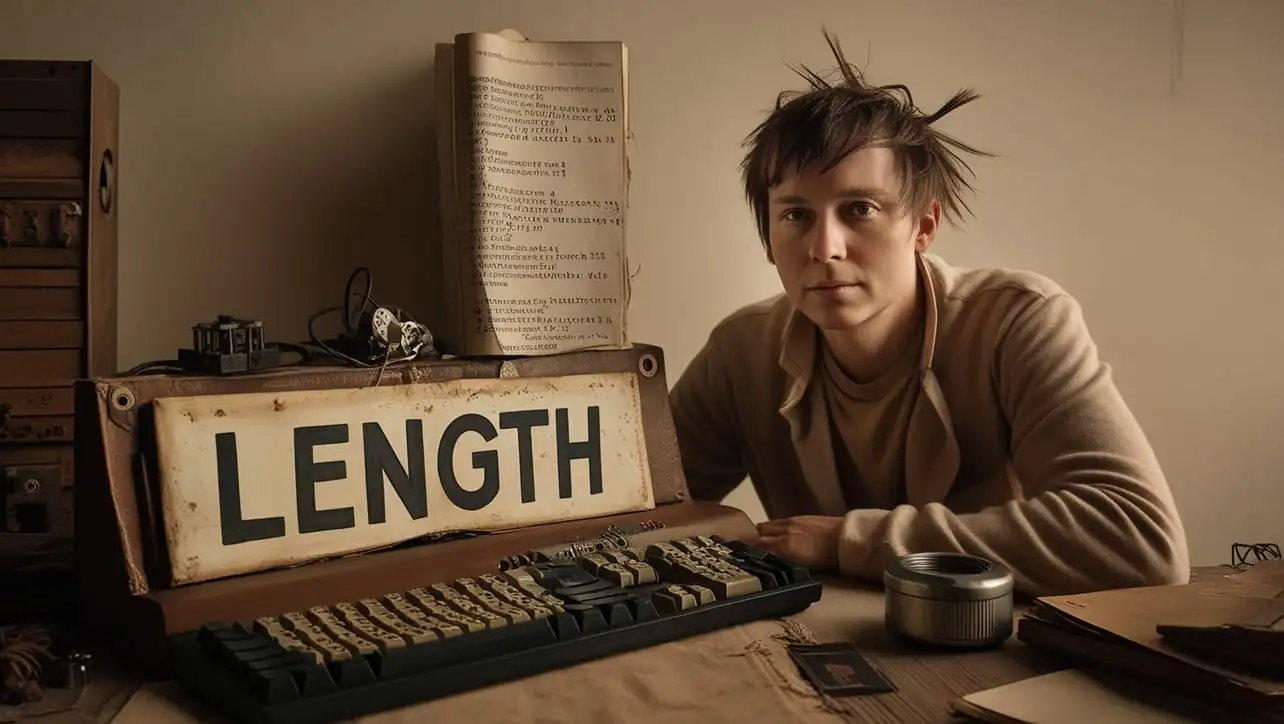
JS Date Methods
JavaScript Date setUTCDate() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript provides a powerful set of tools for working with dates, and the setUTCDate()
method is a valuable addition to this toolkit. This method allows you to set the day of the month for a Date object according to Coordinated Universal Time (UTC).
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical examples of the setUTCDate()
method.
🧠 Understanding setUTCDate() Method
The setUTCDate()
method is used to set the day of the month for a Date object in UTC time. It takes a single parameter representing the day of the month. This method does not directly affect the hours, minutes, seconds, or milliseconds of the Date object.
💡 Syntax
The syntax for the setUTCDate()
method is straightforward:
dateObj.setUTCDate(dayValue);
- dateObj: The Date object for which you want to set the day.
- dayValue: An integer representing the day of the month (1-31).
📝 Example
Let's dive into a practical example to illustrate the usage of the setUTCDate()
method:
// Create a new Date object
const myDate = new Date('2024-02-29T12:00:00Z');
// Set the day to the 15th using setUTCDate()
myDate.setUTCDate(15);
console.log(myDate.toISOString());
In this example, the setUTCDate()
method is used to set the day of the month to the 15th for the myDate object.
🏆 Best Practices
When working with the setUTCDate()
method, consider the following best practices:
Input Validation:
Ensure that the input day value is within the valid range (1-31) to prevent unexpected behavior.
example.jsCopiedconst desiredDay = 25; if (desiredDay >= 1 && desiredDay <= 31) { myDate.setUTCDate(desiredDay); } else { console.error('Invalid day value. Please provide a value between 1 and 31.'); }
Understanding UTC:
Be aware that the
setUTCDate()
method operates in Coordinated Universal Time (UTC), so adjustments may be necessary depending on your application's requirements.
📚 Use Cases
Handling Month-End Scenarios:
The
setUTCDate()
method is particularly useful when dealing with month-end scenarios, such as setting the date to the last day of the month:example.jsCopied// Function to set the date to the last day of the month function setToLastDayOfMonth(dateObj) { dateObj.setUTCDate(0); // Move to the last day of the previous month dateObj.setUTCDate(1); // Move to the first day of the current month } // Example usage const endOfMonthDate = new Date(); setToLastDayOfMonth(endOfMonthDate); console.log(endOfMonthDate.toISOString());
Incrementing the Date:
You can use the
setUTCDate()
method to increment the date by a certain number of days:example.jsCopiedconst currentDate = new Date(); const daysToAdd = 5; currentDate.setUTCDate(currentDate.getUTCDate() + daysToAdd); console.log(currentDate.toISOString());
🎉 Conclusion
The setUTCDate()
method is a valuable tool for manipulating dates in JavaScript, allowing you to precisely set the day of the month in Coordinated Universal Time.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the setUTCDate()
method in your JavaScript projects.
👨💻 Join our Community:
Author
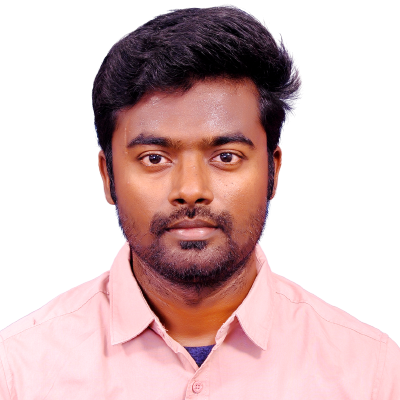
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date setUTCDate() Method), please comment here. I will help you immediately.