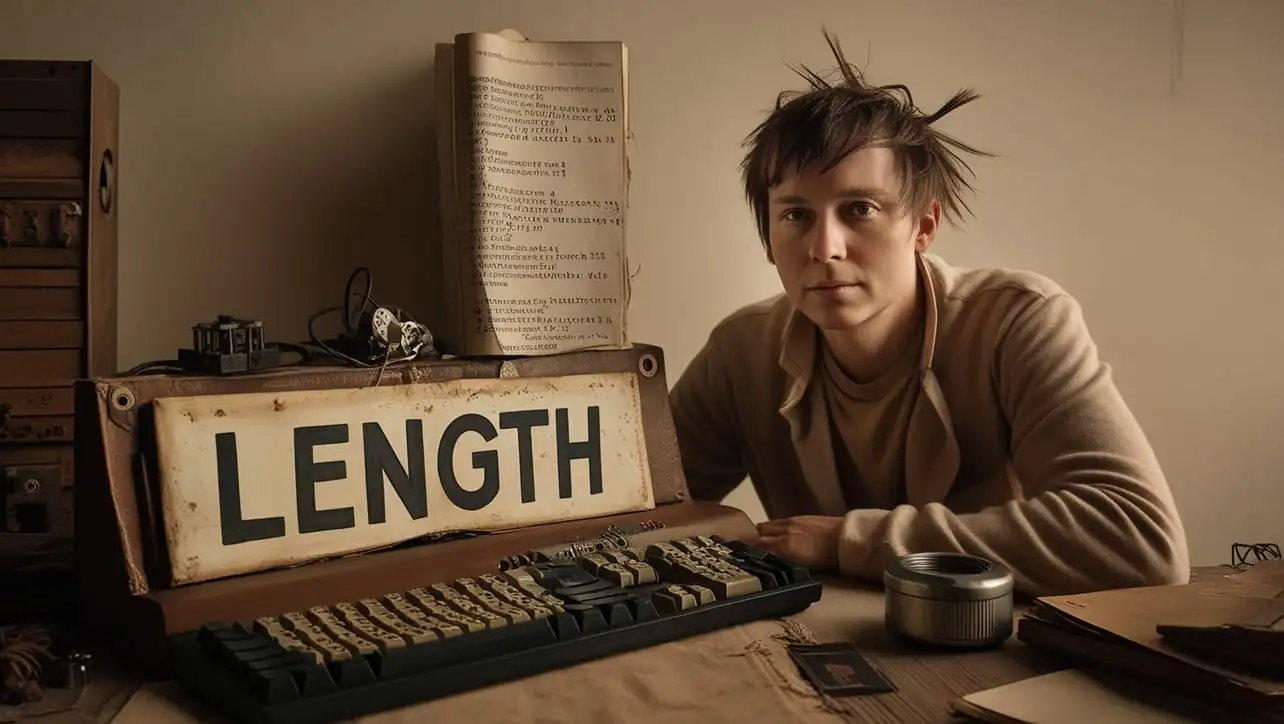
JS Date Methods
JavaScript Date setSeconds() Method

Photo Credit to CodeToFun
🙋 Introduction
Working with dates and times is a common task in JavaScript, and the setSeconds()
method provides a powerful tool for manipulating the seconds component of a Date object.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical examples of the setSeconds()
method to empower you in managing time-related operations in your JavaScript applications.
🧠 Understanding setSeconds() Method
The setSeconds()
method is part of the Date object in JavaScript and is used to set the seconds of a date object, allowing you to modify a specific aspect of time with precision.
💡 Syntax
The syntax for the setSeconds()
method is straightforward:
dateObj.setSeconds(secondsValue[, msValue]);
- dateObj: The Date object you want to modify.
- secondsValue: An integer between 0 and 59, representing the seconds.
- msValue (optional): An integer between 0 and 999, representing the milliseconds.
📝 Example
Let's dive into a practical example to illustrate the usage of the setSeconds()
method:
// Create a new Date object
let currentDate = new Date();
// Set the seconds to 30
currentDate.setSeconds(30);
console.log(currentDate);
In this example, the setSeconds()
method is used to set the seconds of the currentDate object to 30.
🏆 Best Practices
When working with the setSeconds()
method, consider the following best practices:
Immutable Approach:
Since the
setSeconds()
method modifies the existing Date object, consider creating a new Date object with the desired changes to follow an immutable pattern.example.jsCopiedconst originalDate = new Date(); const modifiedDate = new Date(originalDate); // Set the seconds to 45 in the new object modifiedDate.setSeconds(45); console.log(originalDate.getSeconds()); // Original seconds remain unchanged console.log(modifiedDate.getSeconds()); // New seconds value
Error Handling:
Ensure that the provided values are within the valid range to avoid unexpected behavior.
example.jsCopiedfunction setSecondsSafely(dateObj, seconds) { if (seconds >= 0 && seconds <= 59) { dateObj.setSeconds(seconds); } else { console.error('Invalid seconds value.'); } }
📚 Use Cases
Synchronizing with External Data:
The
setSeconds()
method can be valuable when synchronizing your application's time with external data. For instance, updating a countdown timer to align with a server response.example.jsCopiedconst countdownTimer = new Date(); const secondsFromServer = 15; // Synchronize the countdown timer with the seconds from the server countdownTimer.setSeconds(secondsFromServer);
Building Dynamic Time Features:
Create dynamic time-related features, such as updating a clock every second:
example.jsCopiedfunction updateClock() { const currentClockTime = new Date(); const seconds = currentClockTime.getSeconds(); // Update the clock display every second // (Assuming a function displayTime exists for display purposes) displayTime(currentClockTime); // Schedule the next update setTimeout(updateClock, (60 - seconds) * 1000); } // Initial call to start the clock updateClock();
🎉 Conclusion
The setSeconds()
method is a powerful tool for managing time-related aspects in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the setSeconds()
method in your JavaScript projects.
👨💻 Join our Community:
Author
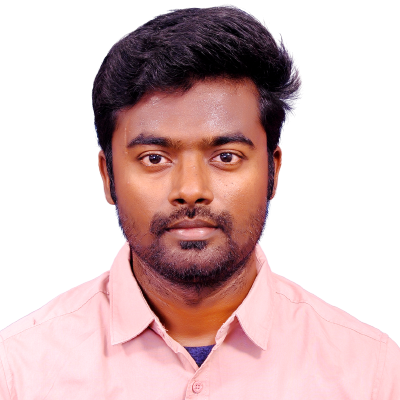
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date setSeconds() Method), please comment here. I will help you immediately.