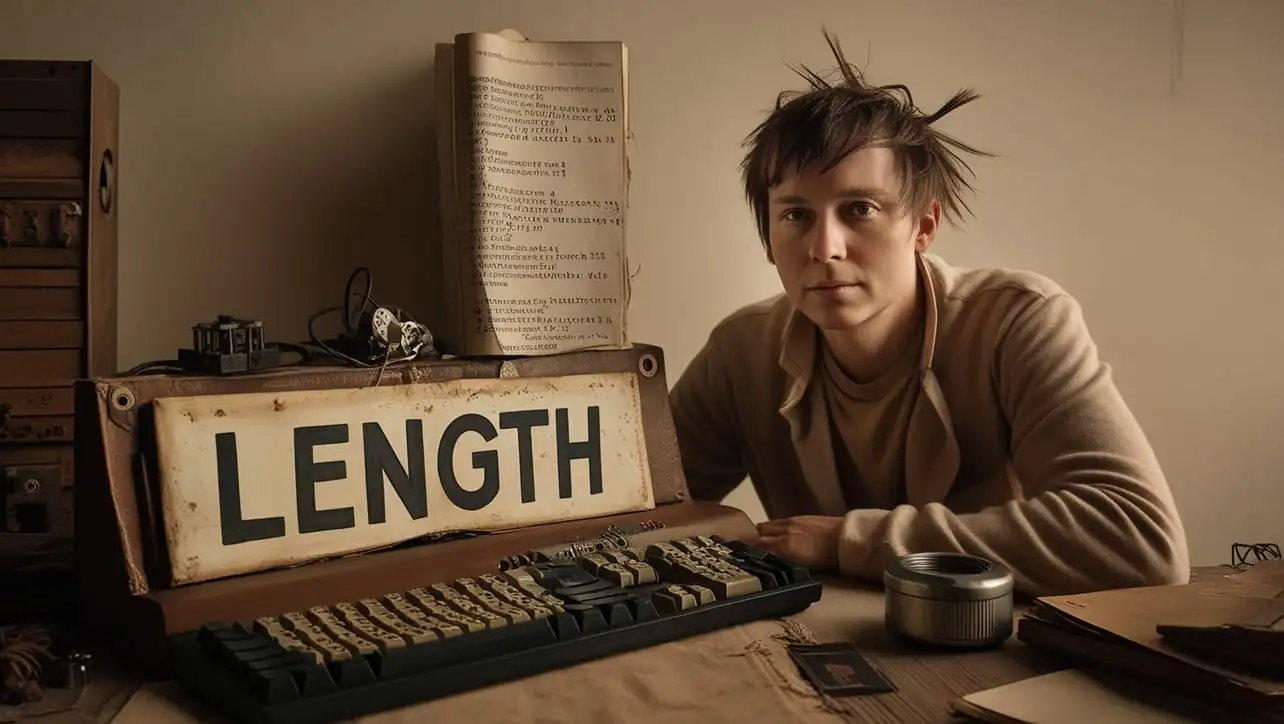
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- JS Console Methods
- JS Date Methods
- getDate()
- getDay()
- getFullYear()
- getHours()
- getMilliseconds()
- getMinutes()
- getMonth()
- getSeconds()
- getTime()
- getTimezoneOffset()
- getUTCDate()
- getUTCDay()
- getUTCFullYear()
- getUTCHours()
- getUTCMilliseconds()
- getUTCMinutes()
- getUTCMonth()
- getUTCSeconds()
- now()
- parse()
- setDate()
- setFullYear()
- setHours()
- setMilliseconds()
- setMinutes()
- setMonth()
- setSeconds()
- setTime()
- setUTCDate()
- setUTCFullYear()
- setUTCHours()
- setUTCMilliseconds()
- setUTCMinutes()
- setUTCMonth()
- setUTCSeconds()
- toDateString()
- toISOString()
- toJSON()
- toLocaleDateString()
- toLocaleString()
- toLocaleTimeString()
- toString()
- toTimeString()
- toUTCString()
- UTC()
- valueOf()
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Date setMonth() Method
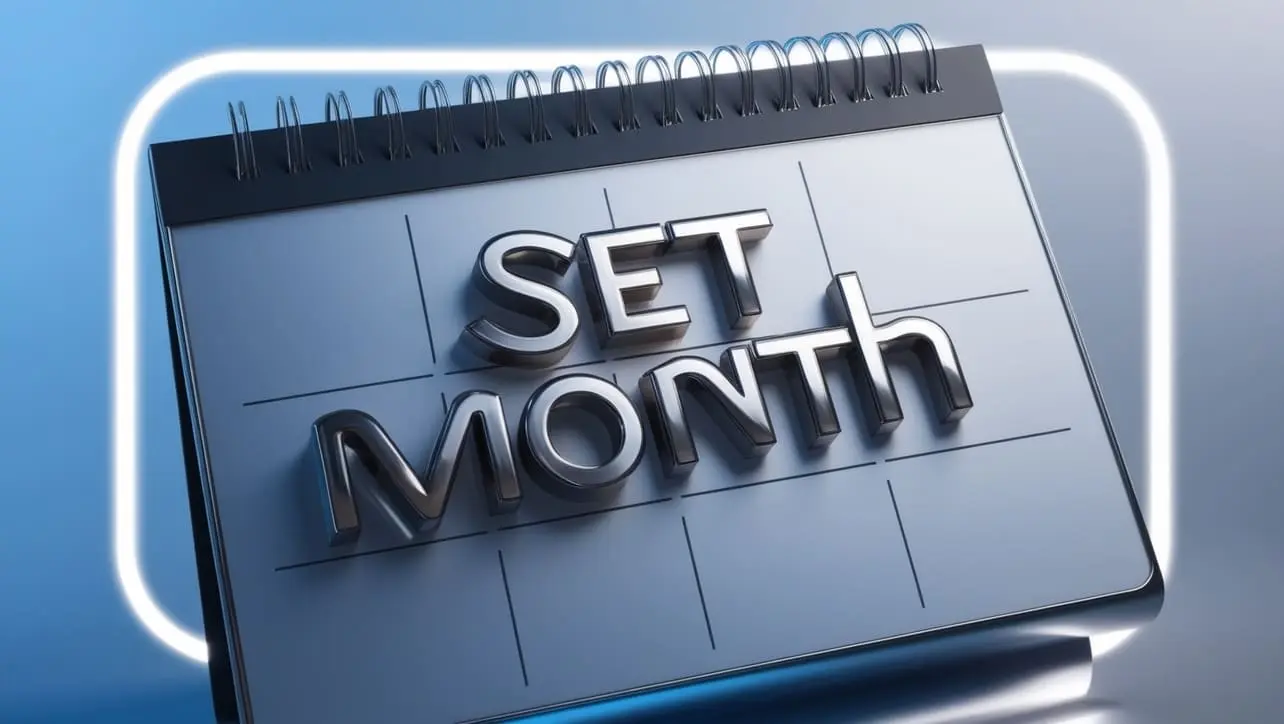
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, the Date object empowers developers to handle date and time-related operations effectively. Among its versatile set of methods, the setMonth()
method stands out, allowing you to modify the month component of a date instance.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical examples of the setMonth()
method.
🧠 Understanding setMonth() Method
The setMonth()
method is part of the Date object, enabling you to set the month of a date according to either a specified numeric value or a date string.
💡 Syntax
The syntax for the setMonth()
method is straightforward:
dateObj.setMonth(month, day);
- dateObj: The Date object you want to modify.
- month: The numeric value representing the month (0-indexed, where 0 is January and 11 is December).
- day (optional): The day of the month. If not provided, the day will be set to the 1st by default.
📝 Example
Let's dive into a simple example to illustrate the usage of the setMonth()
method:
// Create a Date object
let myDate = new Date('2022-02-15');
// Set the month to April (3 is the numeric value for April)
myDate.setMonth(3);
console.log(myDate);
In this example, the setMonth()
method is used to change the month of the myDate object to April.
🏆 Best Practices
When working with the setMonth()
method, consider the following best practices:
Handling Overflow:
Be aware that the
setMonth()
method automatically handles overflow and underflow of months, adjusting the year accordingly.example.jsCopiedlet dateWithOverflow = new Date('2022-01-31'); dateWithOverflow.setMonth(1); // Setting the month to February console.log(dateWithOverflow); // Output: March 3, 2022 (adjusted for overflow)
Use Constants for Months:
To enhance code readability, consider using constants for months (e.g., const JANUARY = 0, FEBRUARY = 1, ...) instead of hardcoded numeric values.
example.jsCopiedconst APRIL = 3; myDate.setMonth(APRIL);
📚 Use Cases
Generating Future Dates:
The
setMonth()
method is handy for generating future dates by incrementing the current month:example.jsCopiedlet currentDate = new Date(); let futureDate = new Date(currentDate); futureDate.setMonth(currentDate.getMonth() + 3); // Add 3 months to the current date console.log(futureDate);
Setting Specific Dates:
You can use the
setMonth()
method to set specific dates while keeping other components unchanged:example.jsCopiedlet specificDate = new Date('2022-06-15'); specificDate.setMonth(11); // Set the month to December console.log(specificDate);
🎉 Conclusion
The setMonth()
method empowers you to manipulate the month component of a Date object efficiently.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the setMonth()
method in your JavaScript projects.
👨💻 Join our Community:
Author
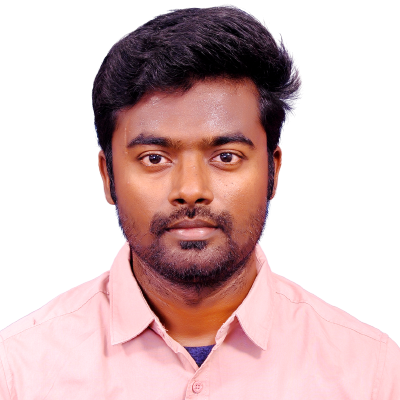
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date setMonth() Method), please comment here. I will help you immediately.