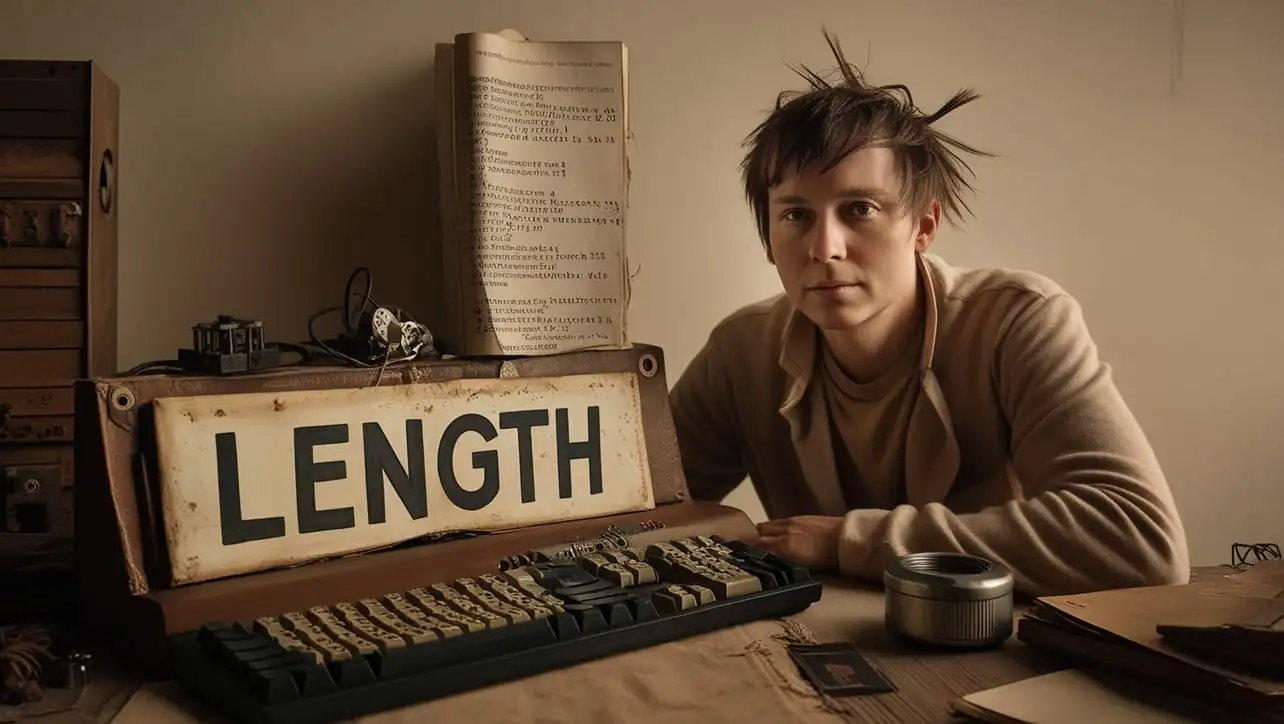
JS Date Methods
JavaScript Date setMilliseconds() Method

Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, the Date object allows you to work with dates and times effectively. The setMilliseconds()
method is a powerful tool that lets you set the milliseconds of a Date object with precision.
In this guide, we'll explore the syntax, usage, best practices, and practical examples of the setMilliseconds()
method.
🧠 Understanding setMilliseconds() Method
The setMilliseconds()
method is part of the Date object's arsenal, providing a straightforward way to manipulate the milliseconds component of a date and time.
💡 Syntax
The syntax for the setMilliseconds()
method is straightforward:
dateObj.setMilliseconds(milliseconds);
- dateObj: The Date object you want to modify.
- milliseconds: An integer representing the milliseconds component (0-999).
📝 Example
Let's dive into a basic example to illustrate the usage of the setMilliseconds()
method:
// Current date and time
const currentDate = new Date();
// Set milliseconds to 500
currentDate.setMilliseconds(500);
console.log(currentDate);
In this example, the setMilliseconds()
method is used to set the milliseconds component of the current date to 500.
🏆 Best Practices
When working with the setMilliseconds()
method, consider the following best practices:
Range Checking:
Ensure that the provided milliseconds value is within the valid range (0-999).
example.jsCopiedconst validMilliseconds = 500; if (validMilliseconds >= 0 && validMilliseconds <= 999) { currentDate.setMilliseconds(validMilliseconds); } else { console.error('Invalid milliseconds value. Must be between 0 and 999.'); }
Chaining:
Take advantage of method chaining for concise and readable code when setting multiple components.
example.jsCopiedcurrentDate .setHours(12) .setMinutes(30) .setSeconds(45) .setMilliseconds(200);
Immutable Operations:
If you prefer immutability, consider creating a new Date object with the modified milliseconds value.
example.jsCopiedconst updatedDate = new Date(currentDate); updatedDate.setMilliseconds(750);
📚 Use Cases
Precise Timing in Animation:
The
setMilliseconds()
method can be beneficial in scenarios where precise timing is crucial, such as in animations:example.jsCopiedfunction animateWithDelay(callback, delayInMilliseconds) { const animationStartTime = new Date().getTime(); const animationEndTime = animationStartTime + delayInMilliseconds; let currentTime; do { currentTime = new Date().getTime(); // Perform animation logic callback(); } while (currentTime < animationEndTime); }
Microbenchmarking in Performance Testing:
When conducting performance tests, the
setMilliseconds()
method can be used to simulate scenarios with specific timing constraints:example.jsCopiedfunction performOperationWithDelay(callback, delayInMilliseconds) { const startTime = new Date().getTime(); const endTime = startTime + delayInMilliseconds; let currentTime; do { currentTime = new Date().getTime(); // Perform operation logic callback(); } while (currentTime < endTime); }
🎉 Conclusion
The setMilliseconds()
method empowers developers to manipulate the milliseconds component of a Date object with precision.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the setMilliseconds()
method in your JavaScript projects.
👨💻 Join our Community:
Author
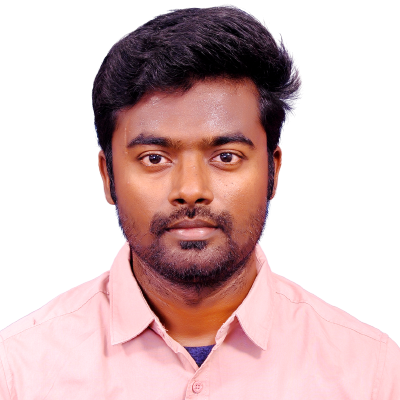
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date setMilliseconds() Method), please comment here. I will help you immediately.