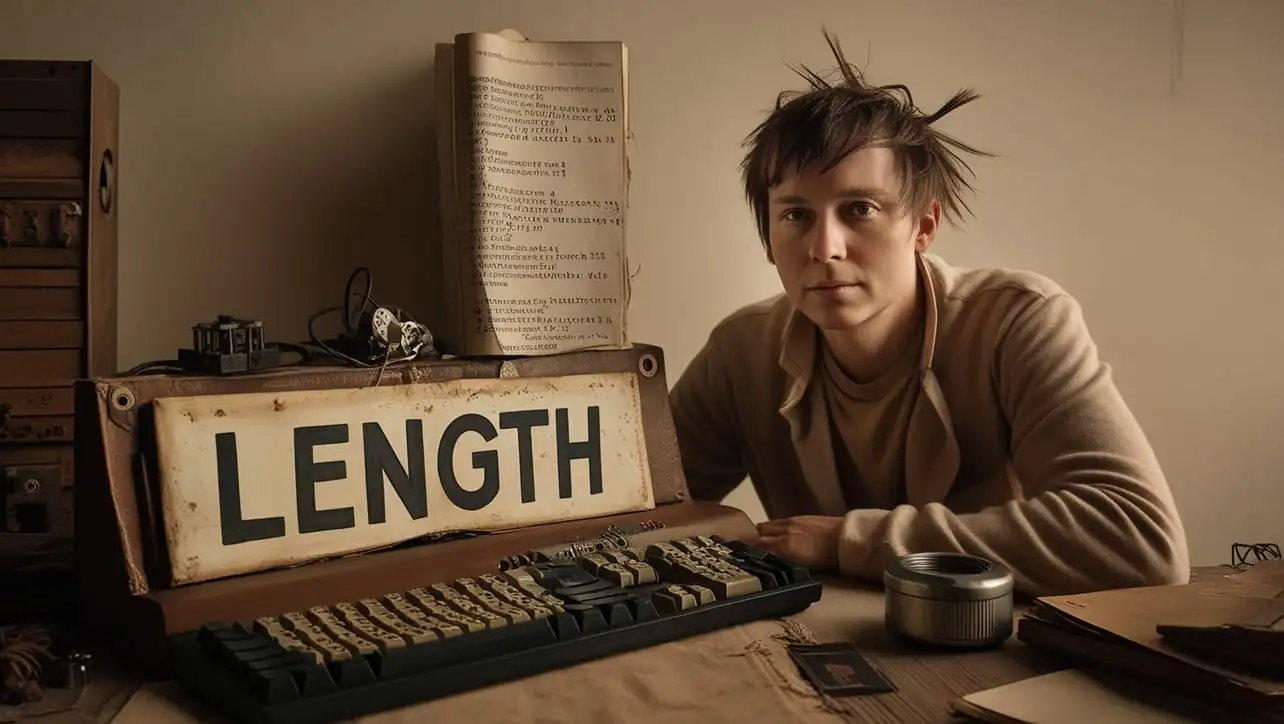
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- JS Console Methods
- JS Date Methods
- getDate()
- getDay()
- getFullYear()
- getHours()
- getMilliseconds()
- getMinutes()
- getMonth()
- getSeconds()
- getTime()
- getTimezoneOffset()
- getUTCDate()
- getUTCDay()
- getUTCFullYear()
- getUTCHours()
- getUTCMilliseconds()
- getUTCMinutes()
- getUTCMonth()
- getUTCSeconds()
- now()
- parse()
- setDate()
- setFullYear()
- setHours()
- setMilliseconds()
- setMinutes()
- setMonth()
- setSeconds()
- setTime()
- setUTCDate()
- setUTCFullYear()
- setUTCHours()
- setUTCMilliseconds()
- setUTCMinutes()
- setUTCMonth()
- setUTCSeconds()
- toDateString()
- toISOString()
- toJSON()
- toLocaleDateString()
- toLocaleString()
- toLocaleTimeString()
- toString()
- toTimeString()
- toUTCString()
- UTC()
- valueOf()
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Date setHours() Method
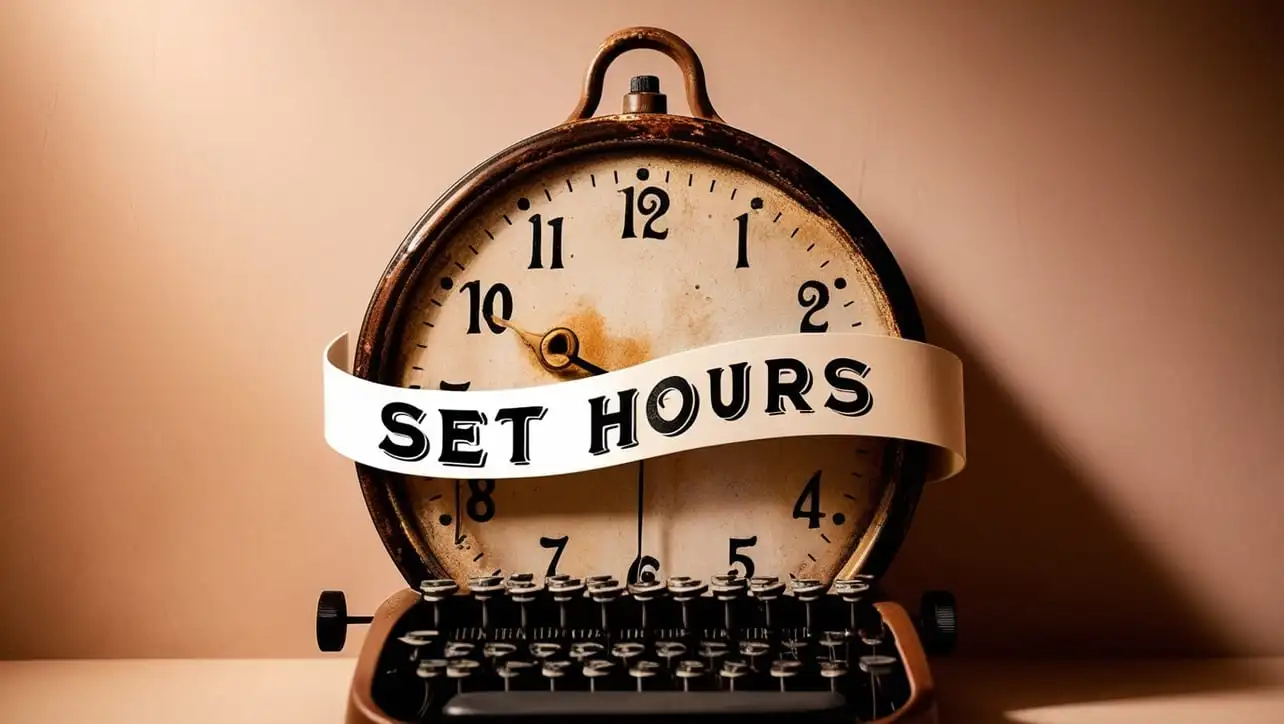
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, working with dates is a common task, and the setHours()
method proves to be a valuable tool when you need to manipulate the hours of a Date object.
This guide will explore the syntax, usage, best practices, and practical examples of the setHours()
method.
🧠 Understanding setHours() Method
The setHours()
method allows you to set the hour value of a Date object. It provides a flexible way to adjust the time component while keeping the other date components unchanged.
💡 Syntax
The syntax for the setHours()
method is straightforward:
dateObject.setHours(hours[, minutes[, seconds[, milliseconds]]]);
- dateObject: The Date object to be modified.
- hours: The numeric value representing the desired hours (0-23).
- minutes (optional): The numeric value representing the desired minutes (0-59).
- seconds (optional): The numeric value representing the desired seconds (0-59).
- milliseconds (optional): The numeric value representing the desired milliseconds (0-999).
📝 Example
Let's explore a practical example of using the setHours()
method:
// Create a Date object for March 1, 2024, 12:30:45
const myDate = new Date(2024, 2, 1, 12, 30, 45);
// Set the hours to 8
myDate.setHours(8);
console.log(myDate);
// Output: Fri Mar 01 2024 08:30:45 GMT+0000 (Coordinated Universal Time)
In this example, the setHours()
method is used to change the hours of the object to 8 while keeping the other components unchanged.
🏆 Best Practices
When working with the setHours()
method, consider the following best practices:
Use Destructuring for Clarity:
If you are only modifying the hours, consider using destructuring for a clearer syntax.
example.jsCopiedconst newHours = 15; myDate.setHours(newHours); // Instead of: // myDate.setHours(15, myDate.getMinutes(), myDate.getSeconds(), myDate.getMilliseconds());
Mind the 24-Hour Format:
Remember that hours are in a 24-hour format, so ensure that the values provided are within the valid range (0-23).
example.jsCopied// Avoid setting hours to 25 const invalidHours = 25; if (invalidHours >= 0 && invalidHours < 24) { myDate.setHours(invalidHours); } else { console.error('Invalid hours value.'); }
📚 Use Cases
Scheduling Events:
The
setHours()
method is particularly useful when dealing with event scheduling. For example, you can easily set a reminder for an event to occur at a specific hour:example.jsCopied// Set a reminder for a meeting at 15:00:00 const meetingTime = new Date(); meetingTime.setHours(15, 0, 0, 0); console.log(`Meeting scheduled for: ${meetingTime}`);
Timezone Adjustments:
When working with timezones, you might need to adjust the hours accordingly. The
setHours()
method simplifies this task:example.jsCopied// Assume the date is in UTC and needs to be adjusted to a different timezone const utcDate = new Date('2024-03-01T12:30:45Z'); utcDate.setHours(utcDate.getHours() + 3); // Adjusting by 3 hours for a new timezone console.log(`Adjusted Date: ${utcDate}`);
🎉 Conclusion
The setHours()
method provides a powerful way to manipulate the hours of a Date object in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the setHours()
method in your JavaScript projects.
👨💻 Join our Community:
Author
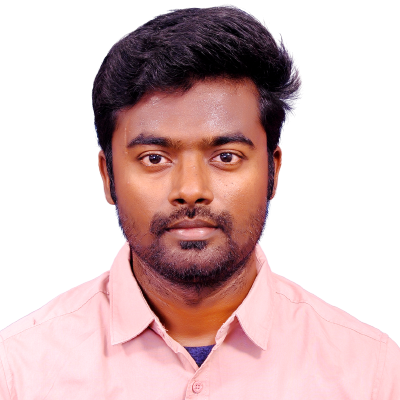
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date setHours() Method), please comment here. I will help you immediately.