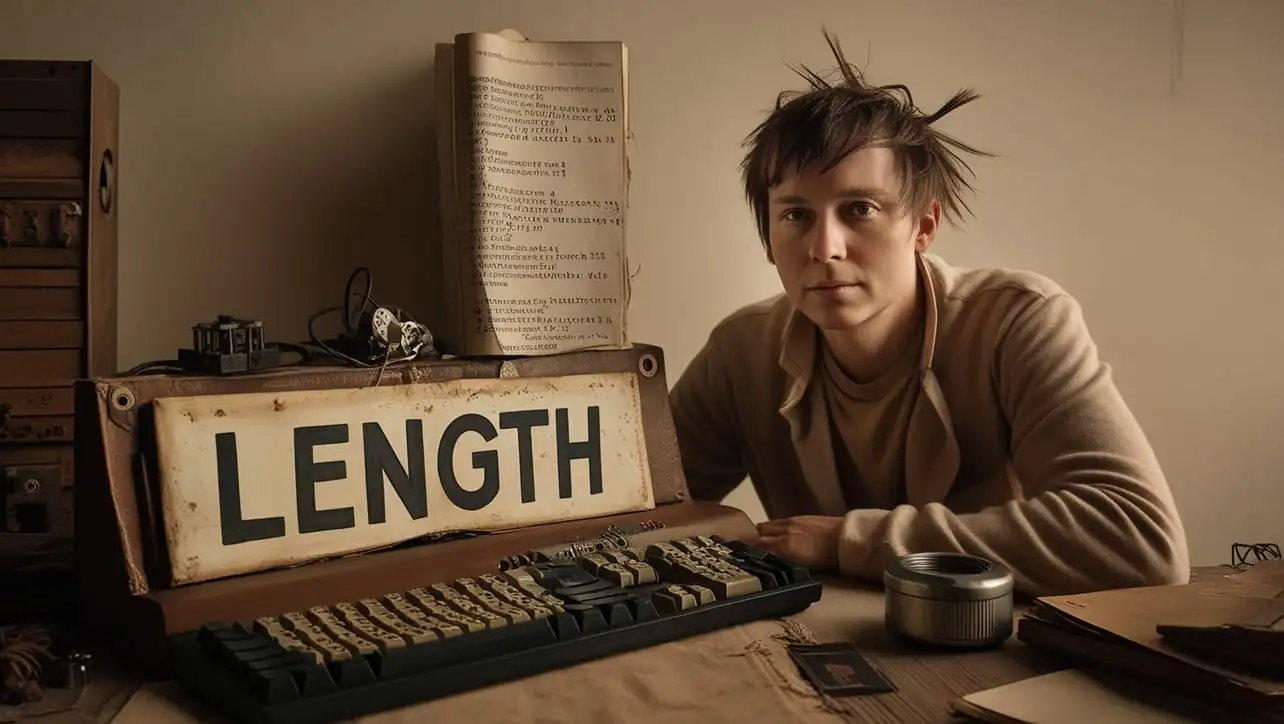
JS Date Methods
JavaScript Date setDate() Method

Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, handling dates is a crucial aspect of many applications, and the setDate()
method plays a pivotal role in manipulating the day of the month within a Date object.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical examples of the setDate()
method to empower you in managing dates effectively.
🧠 Understanding setDate() Method
The setDate()
method is used to set the day of the month for a specified Date object. It allows you to easily update the day component of a date without affecting other parts, such as the month or year.
💡 Syntax
The syntax for the setDate()
method is straightforward:
dateObj.setDate(day);
- dateObj: The Date object you want to modify.
- day: The numeric value representing the day of the month (1-31).
📝 Example
Let's jump into a practical example to illustrate the usage of the setDate()
method:
// Create a new Date object
let myDate = new Date('2024-02-26');
// Using setDate() to set the day to the 10th
myDate.setDate(10);
console.log(myDate);
In this example, the setDate()
method is applied to update the day of the month to the 10th.
🏆 Best Practices
When working with the setDate()
method, consider the following best practices:
Avoid Month Overflow:
Be cautious when setting the day to a value greater than the number of days in the current month. The
setDate()
method automatically adjusts the month and year accordingly, potentially leading to unexpected results.example.jsCopied// Avoiding month overflow const currentDate = new Date(); const desiredDay = 31; // Check if the desired day is valid for the current month if (desiredDay <= new Date(currentDate.getFullYear(), currentDate.getMonth() + 1, 0).getDate()) { currentDate.setDate(desiredDay); } else { console.error('Invalid day for the current month.'); }
Consider Using Date Methods:
For more complex date manipulations, consider using other Date methods in combination with
setDate()
. For instance, updating both the month and day:example.jsCopiedmyDate.setMonth(5); // June myDate.setDate(15);
Handle Invalid Input:
Validate input values to ensure they are within a reasonable range for days (1-31).
example.jsCopied// Handling invalid input function setSafeDate(dateObj, day) { if (day >= 1 && day <= 31) { dateObj.setDate(day); } else { console.error('Invalid day input. Please provide a value between 1 and 31.'); } } // Example usage const invalidDate = new Date(); setSafeDate(invalidDate, 40); // Outputs: 'Invalid day input. Please provide a value between 1 and 31.'
📚 Use Cases
Dynamic Date Generation:
The
setDate()
method is commonly used to dynamically generate dates. For example, setting the date to the last day of the current month:example.jsCopiedconst lastDayOfMonth = new Date(); lastDayOfMonth.setMonth(lastDayOfMonth.getMonth() + 1, 0);
Countdown Timers:
Countdown timers often involve updating the days remaining. The
setDate()
method is useful for decrementing the day value:example.jsCopiedconst endDate = new Date('2024-03-15'); const daysRemaining = Math.ceil((endDate - new Date()) / (1000 * 60 * 60 * 24)); // Update a countdown display document.getElementById('countdown').innerText = `${daysRemaining} days left!`;
🎉 Conclusion
Mastering the setDate()
method empowers you to effortlessly manage the day component of your Date objects in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the setDate()
method in your JavaScript projects.
👨💻 Join our Community:
Author
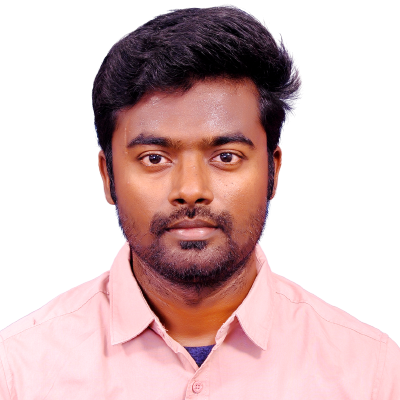
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date setDate() Method), please comment here. I will help you immediately.