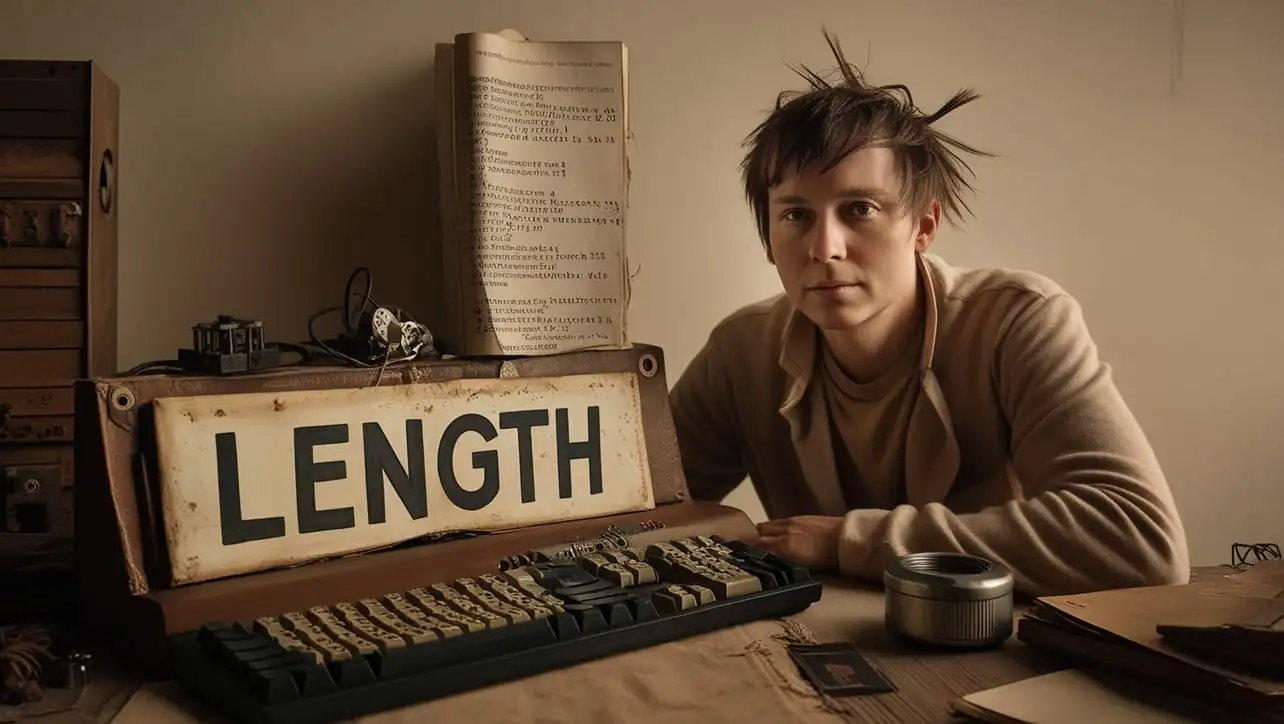
JS Date Methods
JavaScript Date parse() Method

Photo Credit to CodeToFun
🙋 Introduction
Working with dates is a fundamental aspect of programming, and JavaScript provides the parse()
method as a powerful tool for parsing date strings.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical examples of the parse()
method to help you manipulate dates effectively in your JavaScript applications.
🧠 Understanding parse() Method
The parse()
method is a built-in function in JavaScript that allows you to parse a date string and convert it into a timestamp, which is the number of milliseconds since January 1, 1970 (UTC). This method is particularly useful when dealing with date inputs from various sources.
💡 Syntax
The syntax for the parse()
method is straightforward:
Date.parse(dateString);
- dateString: The string representing the date to be parsed.
📝 Example
Let's delve into a practical example to illustrate the usage of the parse()
method:
// Parsing a date string
const dateString = '2024-02-26T12:30:00Z';
const timestamp = Date.parse(dateString);
console.log(timestamp); // Output: 1519664400000 (timestamp in milliseconds)
In this example, the parse()
method is used to convert the ISO 8601 formatted date string into a timestamp.
🏆 Best Practices
When working with the parse()
method, consider the following best practices:
Use ISO 8601 Format:
For consistent results across different browsers, it's recommended to provide date strings in the ISO 8601 format.
example.jsCopiedconst isoDateString = '2024-02-26T12:30:00Z'; const timestamp = Date.parse(isoDateString);
Error Handling:
Always handle potential errors when parsing date strings to avoid unexpected behavior.
example.jsCopiedconst dateString = 'invalid-date-string'; const timestamp = Date.parse(dateString); if (isNaN(timestamp)) { console.error('Invalid date string provided.'); } else { console.log(timestamp); }
📚 Use Cases
Calculating Time Differences:
The
parse()
method can be handy when calculating time differences between two dates:example.jsCopiedconst startTimestamp = Date.parse('2024-02-26T12:00:00Z'); const endTimestamp = Date.parse('2024-02-26T14:30:00Z'); const timeDifferenceInHours = (endTimestamp - startTimestamp) / (1000 * 60 * 60); console.log(`Time difference: ${timeDifferenceInHours} hours`);
Validating User Input:
You can use the
parse()
method to validate date input provided by users:example.jsCopiedconst userInput = '2024-02-26T12:30:00Z'; if (!isNaN(Date.parse(userInput))) { console.log('Valid date input.'); } else { console.error('Invalid date input.'); }
🎉 Conclusion
The parse()
method is a valuable tool for handling date strings in JavaScript, providing a straightforward way to convert them into timestamps.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the parse()
method in your JavaScript projects.
👨💻 Join our Community:
Author
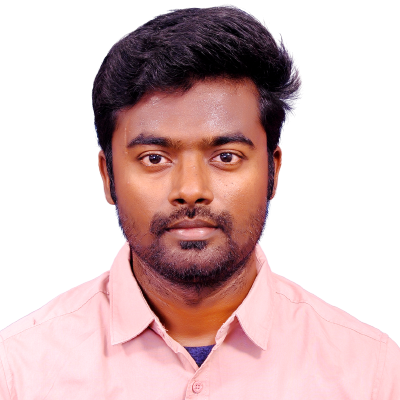
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date parse() Method), please comment here. I will help you immediately.