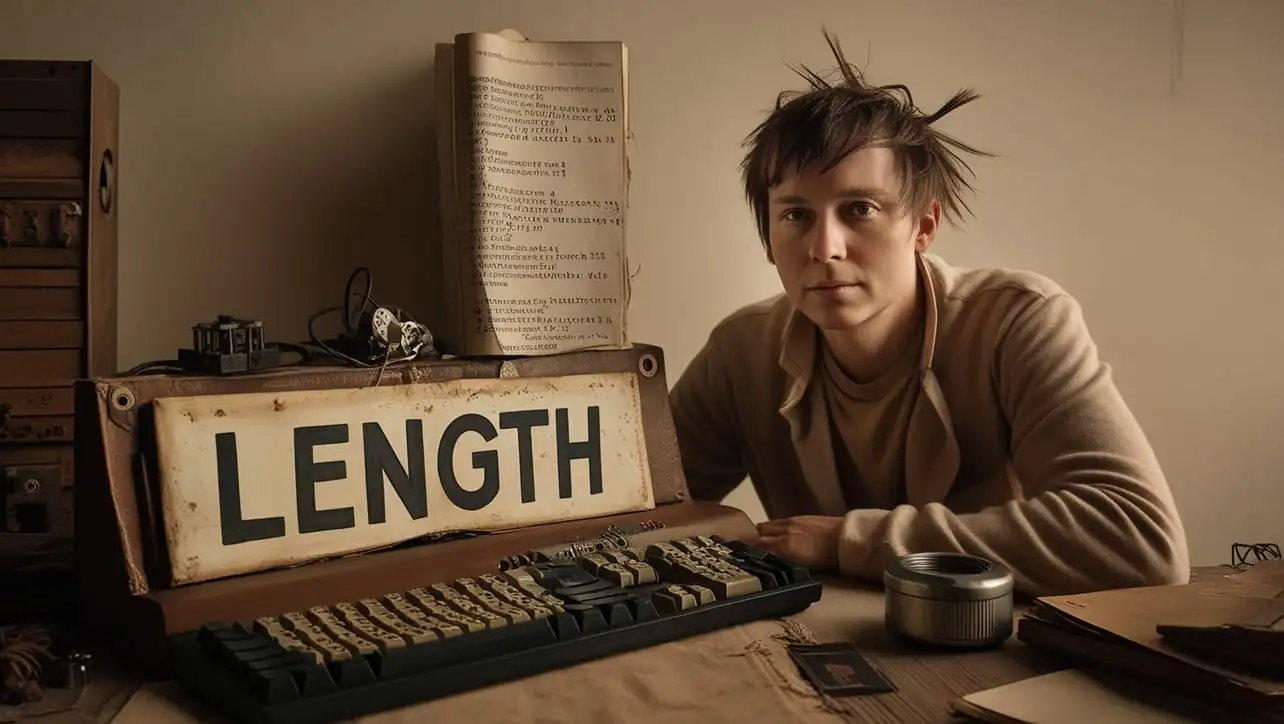
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- JS Console Methods
- JS Date Methods
- getDate()
- getDay()
- getFullYear()
- getHours()
- getMilliseconds()
- getMinutes()
- getMonth()
- getSeconds()
- getTime()
- getTimezoneOffset()
- getUTCDate()
- getUTCDay()
- getUTCFullYear()
- getUTCHours()
- getUTCMilliseconds()
- getUTCMinutes()
- getUTCMonth()
- getUTCSeconds()
- now()
- parse()
- setDate()
- setFullYear()
- setHours()
- setMilliseconds()
- setMinutes()
- setMonth()
- setSeconds()
- setTime()
- setUTCDate()
- setUTCFullYear()
- setUTCHours()
- setUTCMilliseconds()
- setUTCMinutes()
- setUTCMonth()
- setUTCSeconds()
- toDateString()
- toISOString()
- toJSON()
- toLocaleDateString()
- toLocaleString()
- toLocaleTimeString()
- toString()
- toTimeString()
- toUTCString()
- UTC()
- valueOf()
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Date now() Method
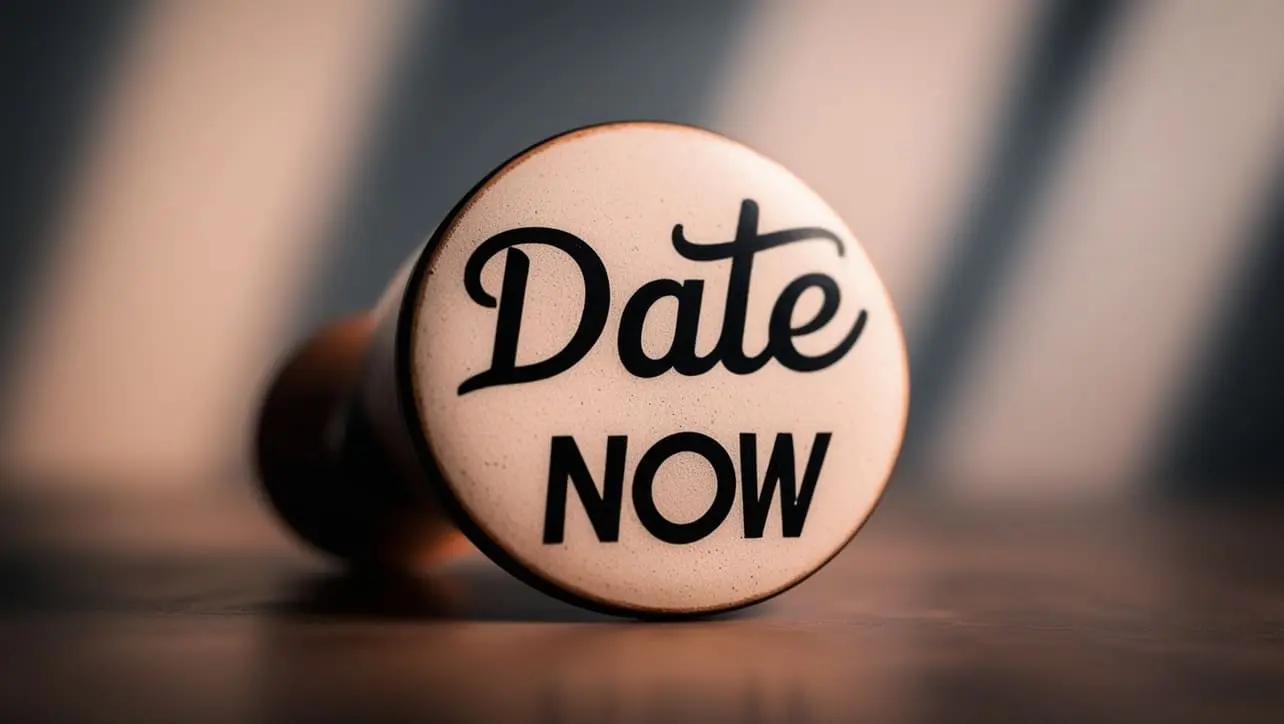
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, the Date object provides various methods for working with dates and times. The now()
method is a simple yet powerful feature that allows you to obtain the current timestamp. This timestamp represents the number of milliseconds elapsed since the Unix Epoch.
In this guide, we'll explore the now()
method, understand its syntax, and examine how to effectively use it in your JavaScript applications.
🧠 Understanding now() Method
The now()
method of the Date object returns the current timestamp in milliseconds. This timestamp is a numerical value representing the time elapsed since January 1, 1970, 00:00:00 UTC (known as the Unix Epoch).
💡 Syntax
The syntax for the now()
method is straightforward:
const currentTime = Date.now();
- currentTime: A variable that stores the current timestamp.
📝 Example
Let's dive into a simple example to illustrate the usage of the now()
method:
// Using now() to obtain the current timestamp
const currentTime = Date.now();
console.log(currentTime);
In this example, the now()
method is called on the Date object, and the resulting timestamp is logged to the console.
🏆 Best Practices
When working with the now()
method, consider the following best practices:
Avoid Creating Unnecessary Date Objects:
Since
now()
is a static method, there's no need to create a Date object before calling it. UseDate.now()
directly for efficiency.example.jsCopied// Avoid unnecessary Date object creation const currentTime = Date.now();
Understanding the Timestamp Format:
Be aware that the timestamp returned by
now()
is in milliseconds. If you need seconds, consider dividing the result by 1000.example.jsCopiedconst currentSeconds = Math.floor(Date.now() / 1000);
📚 Use Cases
Measuring Execution Time:
The
now()
method is commonly used to measure the execution time of a piece of code. By recording the timestamp before and after code execution, you can calculate the elapsed time.example.jsCopied// Recording the start time const startTime = Date.now(); // Your code to measure execution time // Recording the end time const endTime = Date.now(); // Calculating elapsed time const elapsedTime = endTime - startTime; console.log(`Code execution time: ${elapsedTime} milliseconds`);
Generating Timestamps for Data:
When working with data that requires timestamps, such as logging or tracking events,
Date.now()
is a convenient way to obtain a unique identifier.example.jsCopiedconst logEvent = (message) => { const timestamp = Date.now(); console.log(`[${timestamp}] ${message}`); }; logEvent('User logged in');
🎉 Conclusion
The Date.now()
method provides a straightforward way to obtain the current timestamp in JavaScript. Whether measuring execution time or generating unique identifiers, incorporating this method into your code can enhance your handling of dates and times.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the now()
method in your JavaScript projects.
👨💻 Join our Community:
Author
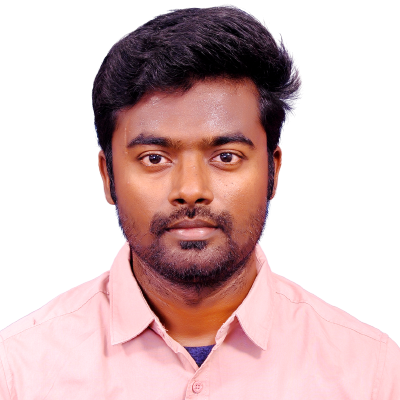
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date now() Method), please comment here. I will help you immediately.