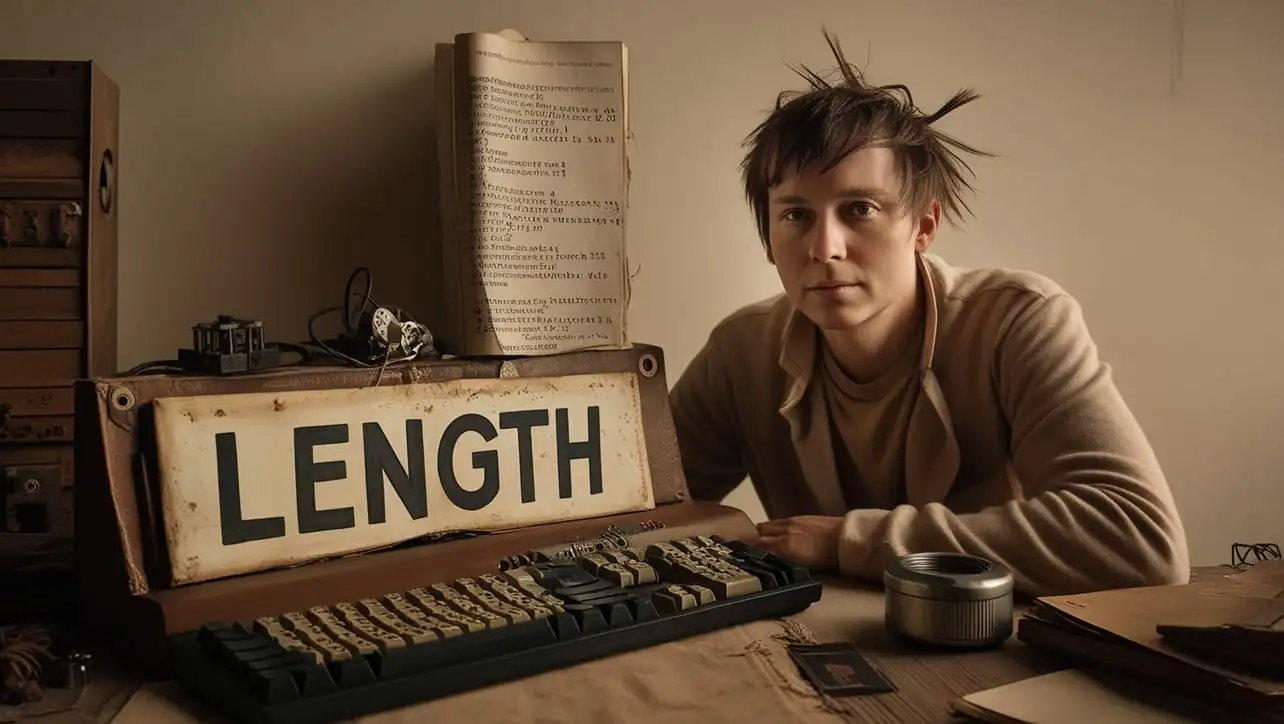
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- JS Console Methods
- JS Date Methods
- getDate()
- getDay()
- getFullYear()
- getHours()
- getMilliseconds()
- getMinutes()
- getMonth()
- getSeconds()
- getTime()
- getTimezoneOffset()
- getUTCDate()
- getUTCDay()
- getUTCFullYear()
- getUTCHours()
- getUTCMilliseconds()
- getUTCMinutes()
- getUTCMonth()
- getUTCSeconds()
- now()
- parse()
- setDate()
- setFullYear()
- setHours()
- setMilliseconds()
- setMinutes()
- setMonth()
- setSeconds()
- setTime()
- setUTCDate()
- setUTCFullYear()
- setUTCHours()
- setUTCMilliseconds()
- setUTCMinutes()
- setUTCMonth()
- setUTCSeconds()
- toDateString()
- toISOString()
- toJSON()
- toLocaleDateString()
- toLocaleString()
- toLocaleTimeString()
- toString()
- toTimeString()
- toUTCString()
- UTC()
- valueOf()
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Date getUTCSeconds() Method
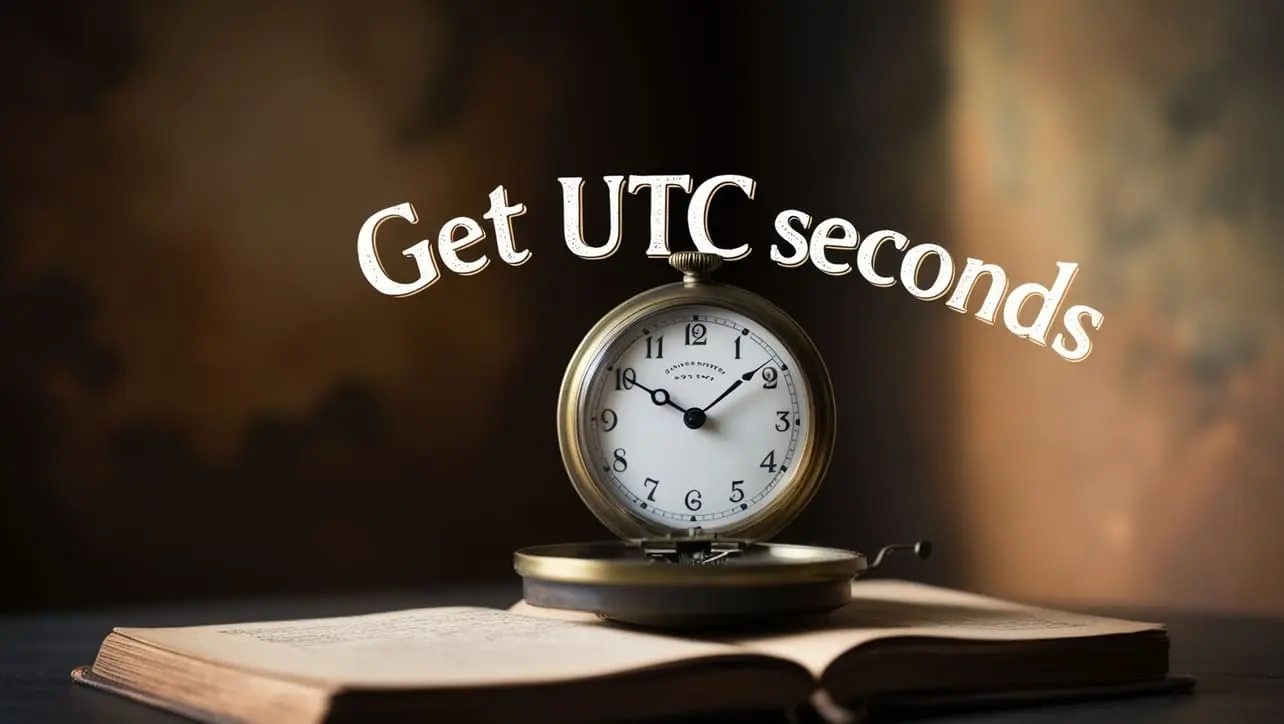
Photo Credit to CodeToFun
🙋 Introduction
Working with dates and times in JavaScript is a common requirement, and the getUTCSeconds()
method is a valuable tool when dealing with UTC (Coordinated Universal Time) in the context of Date objects.
In this guide, we'll explore the getUTCSeconds()
method, understand its syntax, and see how it can be effectively used in JavaScript applications.
🧠 Understanding getUTCSeconds() Method
The getUTCSeconds()
method is a part of the Date object in JavaScript, and it allows you to retrieve the seconds component of a date in UTC. This method returns an integer between 0 and 59, representing the seconds.
💡 Syntax
The syntax for the getUTCSeconds()
method is straightforward:
dateObj.getUTCSeconds();
- dateObj: The Date object for which you want to obtain the UTC seconds.
📝 Example
Let's dive into a practical example to illustrate the usage of the getUTCSeconds()
method:
// Creating a Date object for the current date and time
const currentDate = new Date();
// Getting the UTC seconds
const utcSeconds = currentDate.getUTCSeconds();
console.log(`UTC Seconds: ${utcSeconds}`);
In this example, the getUTCSeconds()
method is used to retrieve the seconds component of the current date in UTC.
🏆 Best Practices
When working with the getUTCSeconds()
method, consider the following best practices:
Local vs. UTC:
Be mindful of whether your application requires UTC or local time. Use
getUTCSeconds()
for UTC-specific calculations.example.jsCopiedconst localSeconds = currentDate.getSeconds(); const utcSeconds = currentDate.getUTCSeconds(); console.log(`Local Seconds: ${localSeconds}, UTC Seconds: ${utcSeconds}`);
Validation:
Ensure that the Date object you're working with is valid to prevent unexpected results.
example.jsCopiedif (!isNaN(currentDate)) { const utcSeconds = currentDate.getUTCSeconds(); console.log(`UTC Seconds: ${utcSeconds}`); } else { console.error('Invalid Date object.'); }
📚 Use Cases
Countdown Timer:
The
getUTCSeconds()
method is often useful in scenarios like building a countdown timer. Here's a simplified example:example.jsCopiedconst targetDate = new Date('2024-12-31T23:59:59Z'); function updateCountdown() { const now = new Date(); const secondsRemaining = targetDate.getUTCSeconds() - now.getUTCSeconds(); console.log(`Seconds remaining: ${secondsRemaining}`); } // Call the updateCountdown function at regular intervals setInterval(updateCountdown, 1000);
Timezone-Agnostic Comparisons:
When comparing timestamps or durations across different timezones, using UTC components like
getUTCSeconds()
ensures consistency.example.jsCopiedconst timestamp1 = new Date('2024-02-28T12:30:00Z'); const timestamp2 = new Date('2024-02-28T18:30:00+06:00'); const secondsDifference = timestamp1.getUTCSeconds() - timestamp2.getUTCSeconds(); console.log(`Time difference in seconds: ${secondsDifference}`);
🎉 Conclusion
The getUTCSeconds()
method provides a straightforward way to retrieve the seconds component of a Date object in UTC.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getUTCSeconds()
method in your JavaScript projects.
👨💻 Join our Community:
Author
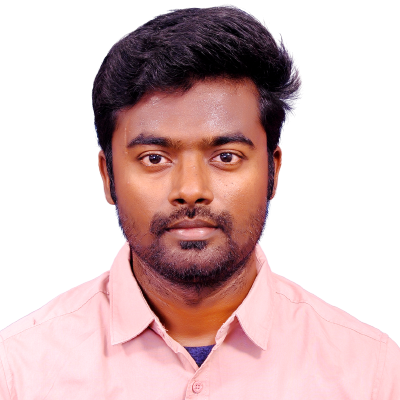
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getUTCSeconds() Method), please comment here. I will help you immediately.