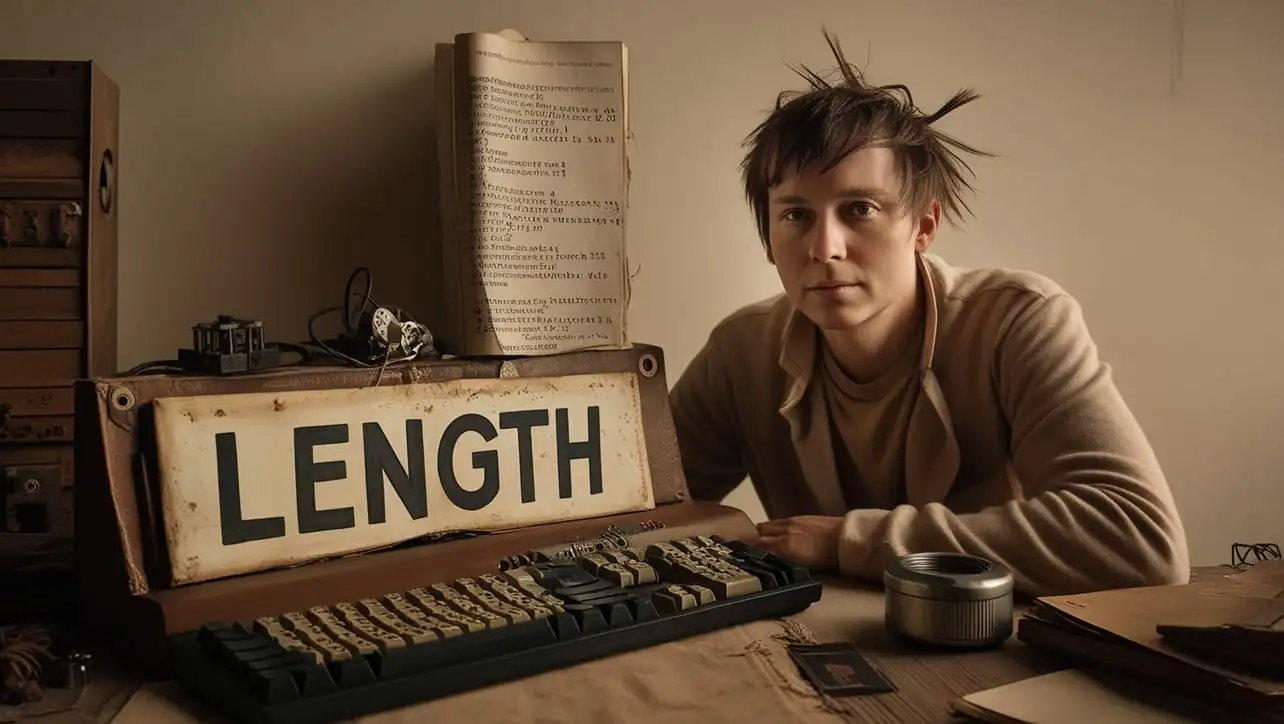
JS Date Methods
JavaScript Date getUTCMilliseconds() Method

Photo Credit to CodeToFun
🙋 Introduction
Working with dates and times is a crucial aspect of many applications, and JavaScript's Date object provides various methods to facilitate these operations. One such method is getUTCMilliseconds()
, which allows you to retrieve the milliseconds component of a date in Coordinated Universal Time (UTC).
In this guide, we'll delve into the syntax, usage, best practices, and practical examples of the getUTCMilliseconds()
method.
🧠 Understanding getUTCMilliseconds() Method
The getUTCMilliseconds()
method is a part of the Date object in JavaScript. It returns the milliseconds component of a date, according to the UTC time zone.
💡 Syntax
The syntax for the getUTCMilliseconds()
method is straightforward:
date.getUTCMilliseconds();
- date: The Date object from which you want to retrieve the milliseconds.
📝 Example
Let's explore a basic example to illustrate the usage of the getUTCMilliseconds()
method:
// Creating a Date object with the current date and time
const currentDate = new Date();
// Using getUTCMilliseconds() to obtain the milliseconds
const milliseconds = currentDate.getUTCMilliseconds();
console.log(`Milliseconds: ${milliseconds}`);
In this example, getUTCMilliseconds()
is applied to the current date, and the result is logged to the console.
🏆 Best Practices
When working with the getUTCMilliseconds()
method, consider the following best practices:
Ensure Proper Date Object:
Confirm that the object on which you are calling
getUTCMilliseconds()
is indeed a Date object to avoid errors.example.jsCopiedconst potentialDate = new Date(); if (potentialDate instanceof Date) { const milliseconds = potentialDate.getUTCMilliseconds(); console.log(`Milliseconds: ${milliseconds}`); } else { console.error('Invalid date object.'); }
Handle Edge Cases:
Be aware that
getUTCMilliseconds()
returns a value between 0 and 999. Handle any potential edge cases in your application logic.example.jsCopiedfunction getMillisecondsWithFallback(date) { if (date instanceof Date) { const milliseconds = date.getUTCMilliseconds(); // Handle edge case where milliseconds are outside the expected range return Math.min(Math.max(milliseconds, 0), 999); } else { console.error('Invalid date object.'); return null; } } const potentialDate = new Date(); const sanitizedMilliseconds = getMillisecondsWithFallback(potentialDate); if (sanitizedMilliseconds !== null) { console.log(`Sanitized Milliseconds: ${sanitizedMilliseconds}`); }
In this example, the getMillisecondsWithFallback function ensures that the retrieved milliseconds are within the expected range (0 to 999).
📚 Use Cases
Timing Operations:
The
getUTCMilliseconds()
method is useful when precise timing is required in applications, such as measuring the duration of specific operations.example.jsCopiedconst start = new Date(); // Perform some time-consuming operation const end = new Date(); const durationMilliseconds = end.getUTCMilliseconds() - start.getUTCMilliseconds(); console.log(`Operation took ${durationMilliseconds} milliseconds.`);
🎉 Conclusion
The getUTCMilliseconds()
method is a valuable tool when dealing with dates and times in a UTC context. By incorporating it into your JavaScript applications, you can efficiently extract the milliseconds component of a Date object.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getUTCMilliseconds()
method in your JavaScript projects.
👨💻 Join our Community:
Author
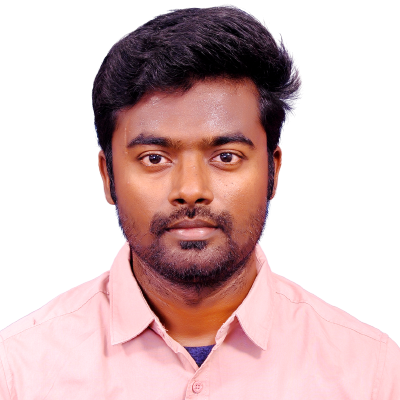
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getUTCMilliseconds() Method), please comment here. I will help you immediately.