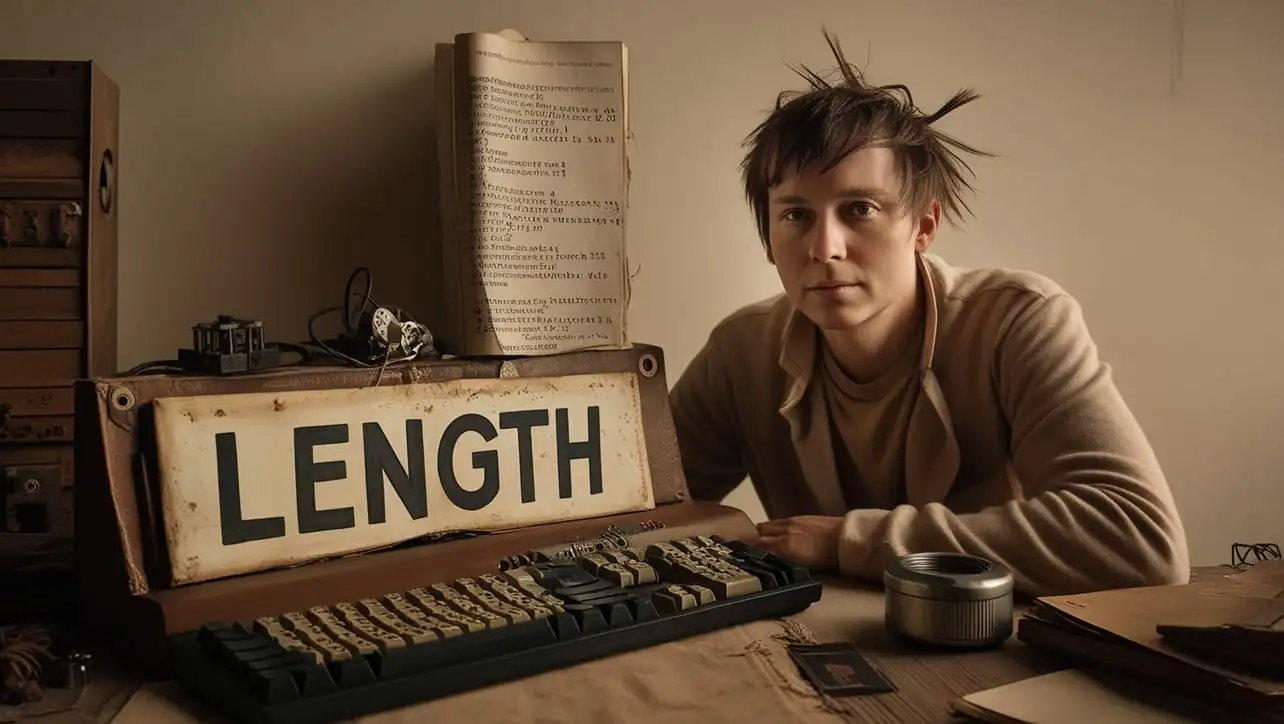
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- JS Console Methods
- JS Date Methods
- getDate()
- getDay()
- getFullYear()
- getHours()
- getMilliseconds()
- getMinutes()
- getMonth()
- getSeconds()
- getTime()
- getTimezoneOffset()
- getUTCDate()
- getUTCDay()
- getUTCFullYear()
- getUTCHours()
- getUTCMilliseconds()
- getUTCMinutes()
- getUTCMonth()
- getUTCSeconds()
- now()
- parse()
- setDate()
- setFullYear()
- setHours()
- setMilliseconds()
- setMinutes()
- setMonth()
- setSeconds()
- setTime()
- setUTCDate()
- setUTCFullYear()
- setUTCHours()
- setUTCMilliseconds()
- setUTCMinutes()
- setUTCMonth()
- setUTCSeconds()
- toDateString()
- toISOString()
- toJSON()
- toLocaleDateString()
- toLocaleString()
- toLocaleTimeString()
- toString()
- toTimeString()
- toUTCString()
- UTC()
- valueOf()
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Date getUTCFullYear() Method
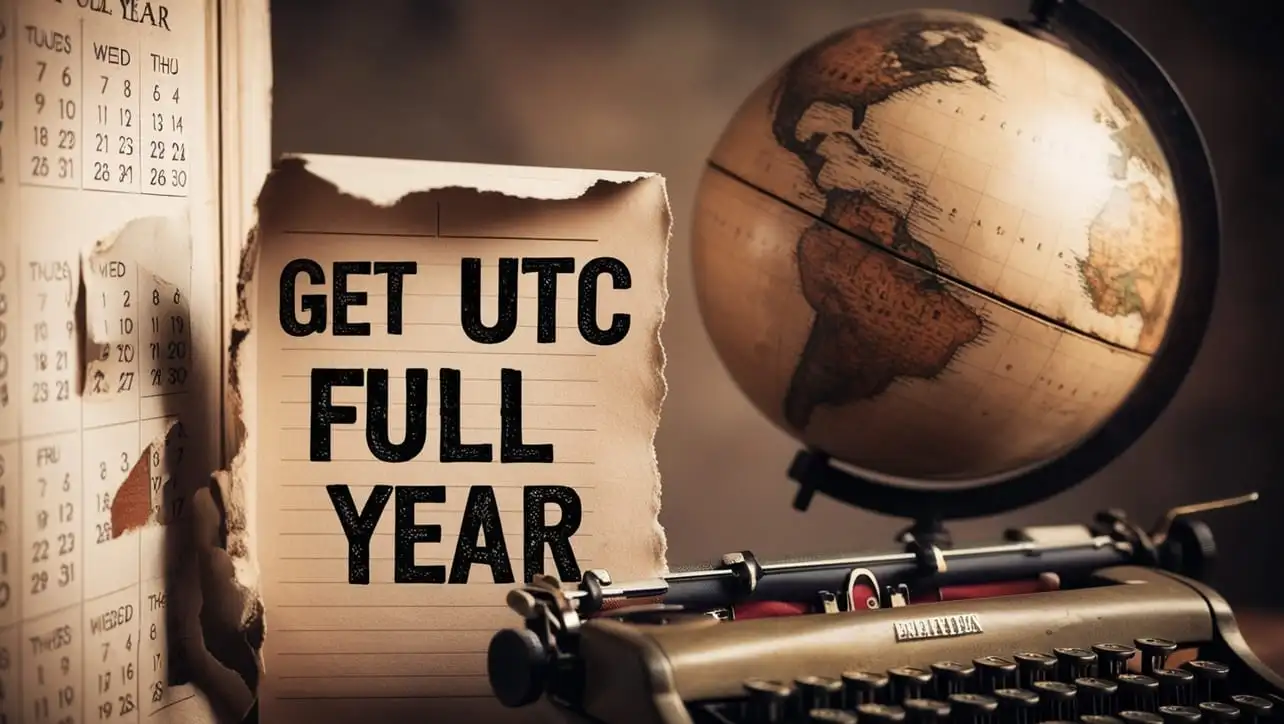
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, the Date object provides various methods for working with dates and times. One such method is getUTCFullYear()
, which allows you to retrieve the year of a date in Coordinated Universal Time (UTC).
In this guide, we'll explore the getUTCFullYear()
method, covering its syntax, usage, best practices, and practical examples.
🧠 Understanding getUTCFullYear() Method
The getUTCFullYear()
method is used to obtain the year of a date according to UTC, regardless of the time zone settings on the local machine.
💡 Syntax
The syntax for the getUTCFullYear()
method is straightforward:
date.getUTCFullYear();
- date: The Date object from which you want to retrieve the year.
📝 Example
Let's dive into a simple example to illustrate the usage of the getUTCFullYear()
method:
// Creating a new Date object
const today = new Date();
// Using getUTCFullYear() to retrieve the year in UTC
const yearInUTC = today.getUTCFullYear();
console.log(`Current year in UTC: ${yearInUTC}`);
In this example, the getUTCFullYear()
method is applied to the Date object today to obtain the current year in Coordinated Universal Time.
🏆 Best Practices
When working with the getUTCFullYear()
method, consider the following best practices:
Understand UTC vs. Local Time:
Be aware that the
getUTCFullYear()
method returns the year in Coordinated Universal Time, which may differ from the local time.example.jsCopiedconst localYear = today.getFullYear(); const utcYear = today.getUTCFullYear(); console.log(`Local year: ${localYear}`); console.log(`UTC year: ${utcYear}`);
Use UTC Methods for Consistency:
If working with UTC dates and times, consider using the corresponding UTC methods (e.g., getUTCFullYear(), getUTCMonth(), etc.) for consistency.
📚 Use Cases
Displaying UTC Year in a Web Application:
In scenarios where it's crucial to display the UTC year in a web application, the
getUTCFullYear()
method becomes essential:example.jsCopied// Assuming you have a date string in UTC format const utcDateString = "2024-02-26T12:00:00Z"; const utcDate = new Date(utcDateString); const utcYear = utcDate.getUTCFullYear(); console.log(`UTC Year: ${utcYear}`);
Calculating Time Differences:
The
getUTCFullYear()
method is valuable when calculating time differences between dates, ensuring consistency across various time zones.example.jsCopiedconst startDate = new Date("2024-01-01T00:00:00Z"); const endDate = new Date(); // Current date const yearsDifference = endDate.getUTCFullYear() - startDate.getUTCFullYear(); console.log(`Years Difference: ${yearsDifference}`);
🎉 Conclusion
The getUTCFullYear()
method in JavaScript's Date object provides a straightforward way to retrieve the year in Coordinated Universal Time.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getUTCFullYear()
method in your JavaScript projects.
👨💻 Join our Community:
Author
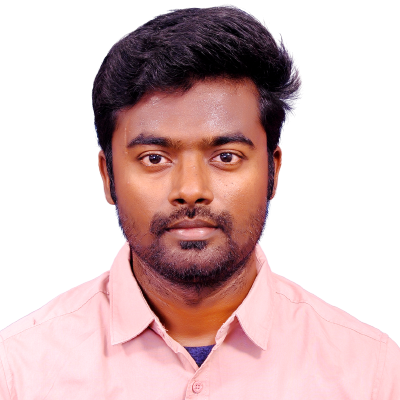
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getUTCFullYear() Method), please comment here. I will help you immediately.