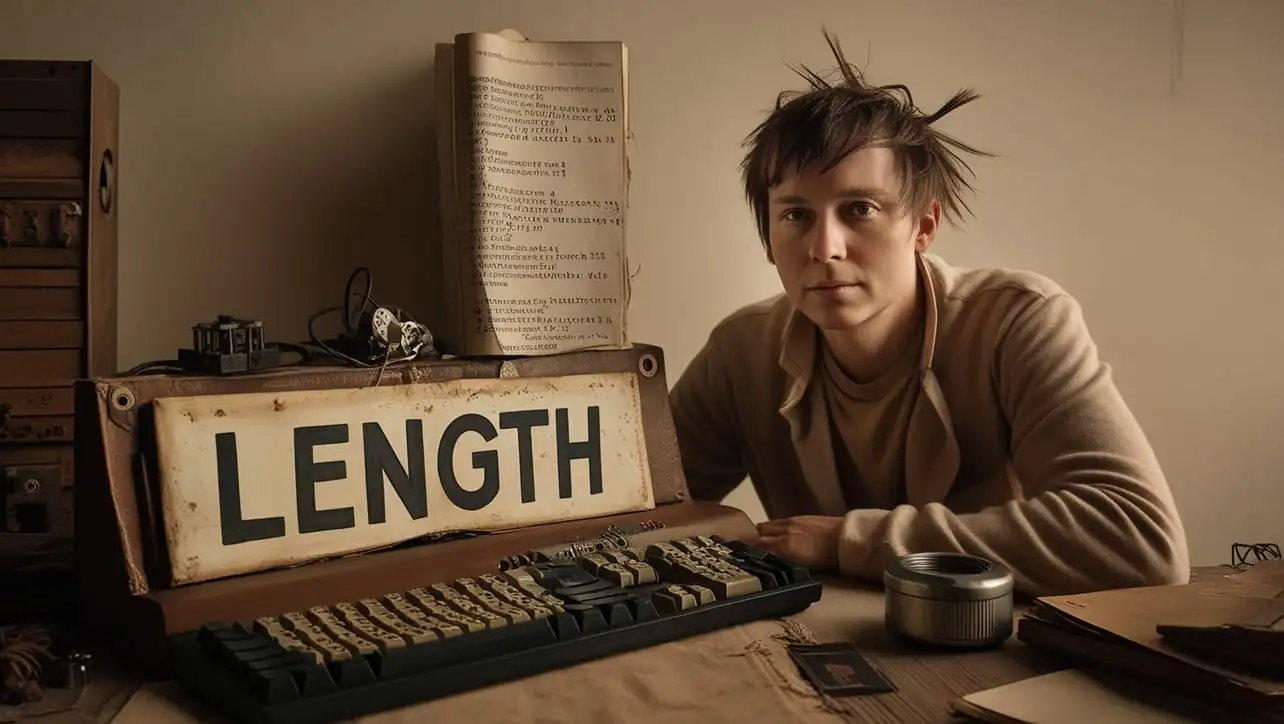
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- JS Console Methods
- JS Date Methods
- getDate()
- getDay()
- getFullYear()
- getHours()
- getMilliseconds()
- getMinutes()
- getMonth()
- getSeconds()
- getTime()
- getTimezoneOffset()
- getUTCDate()
- getUTCDay()
- getUTCFullYear()
- getUTCHours()
- getUTCMilliseconds()
- getUTCMinutes()
- getUTCMonth()
- getUTCSeconds()
- now()
- parse()
- setDate()
- setFullYear()
- setHours()
- setMilliseconds()
- setMinutes()
- setMonth()
- setSeconds()
- setTime()
- setUTCDate()
- setUTCFullYear()
- setUTCHours()
- setUTCMilliseconds()
- setUTCMinutes()
- setUTCMonth()
- setUTCSeconds()
- toDateString()
- toISOString()
- toJSON()
- toLocaleDateString()
- toLocaleString()
- toLocaleTimeString()
- toString()
- toTimeString()
- toUTCString()
- UTC()
- valueOf()
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Date getUTCDate() Methods
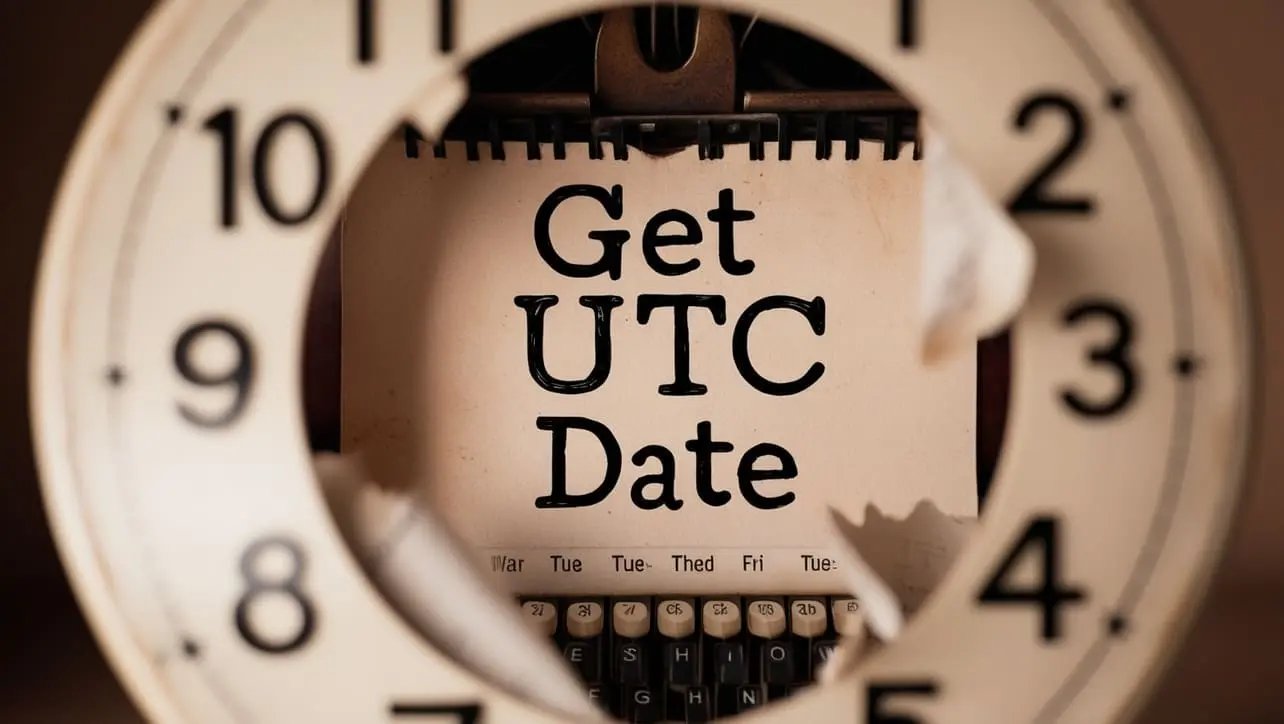
Photo Credit to CodeToFun
🙋 Introduction
JavaScript provides powerful features for working with dates, and the getUTCDate()
method is a key component of this functionality. This method, belonging to the Date object, allows you to retrieve the day of the month in Coordinated Universal Time (UTC).
In this comprehensive guide, we will explore the syntax, usage, best practices, and practical examples of the getUTCDate()
method.
🧠 Understanding getUTCDate() Method
The getUTCDate()
method is used to obtain the day of the month from a Date object in UTC, ranging from 1 to 31.
💡 Syntax
The syntax for the getUTCDate()
method is straightforward:
dateObj.getUTCDate();
- dateObj: The Date object from which you want to retrieve the day of the month.
📝 Example
Let's delve into a straightforward example to illustrate the usage of the getUTCDate()
method:
// Creating a Date object for the current date and time
const currentDate = new Date();
// Using getUTCDate() to retrieve the day of the month in UTC
const dayOfMonthUTC = currentDate.getUTCDate();
console.log(`Day of the month in UTC: ${dayOfMonthUTC}`);
In this example, we create a Date object representing the current date and time, and then use getUTCDate()
to extract the day of the month in UTC.
🏆 Best Practices
When working with the getUTCDate()
method, consider the following best practices:
Timezone Awareness:
Be aware that
getUTCDate()
retrieves the day in UTC, regardless of the local timezone. If you need the day in the local timezone, consider using getDate().example.jsCopied// Using getDate() for day in local timezone const dayOfMonthLocal = currentDate.getDate(); console.log(`Day of the month in local timezone: ${dayOfMonthLocal}`);
Error Handling:
Ensure that the Date object is valid before calling
getUTCDate()
to avoid unexpected results.example.jsCopied// Check if the Date object is valid if (!isNaN(currentDate)) { const dayOfMonthUTC = currentDate.getUTCDate(); console.log(`Day of the month in UTC: ${dayOfMonthUTC}`); } else { console.error('Invalid Date object.'); }
📚 Use Cases
Displaying Current Day:
The
getUTCDate()
method is useful when you need to display the current day of the month in UTC.example.jsCopiedconst dayOfMonthUTC = currentDate.getUTCDate(); console.log(`Today's date in UTC: ${dayOfMonthUTC}`);
Building Date Strings:
When constructing date strings, you might use the
getUTCDate()
method to include the day in a standardized format.example.jsCopiedconst year = currentDate.getUTCFullYear(); const month = currentDate.getUTCMonth() + 1; // Month is zero-indexed const dayOfMonth = currentDate.getUTCDate(); const formattedDate = `${year}-${month < 10 ? '0' : ''}${month}-${dayOfMonth < 10 ? '0' : ''}${dayOfMonth}`; console.log(`Formatted Date in UTC: ${formattedDate}`);
🎉 Conclusion
The getUTCDate()
method is a valuable tool for handling date-related operations in JavaScript, providing a straightforward way to obtain the day of the month in Coordinated Universal Time.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getUTCDate()
method in your JavaScript projects.
👨💻 Join our Community:
Author
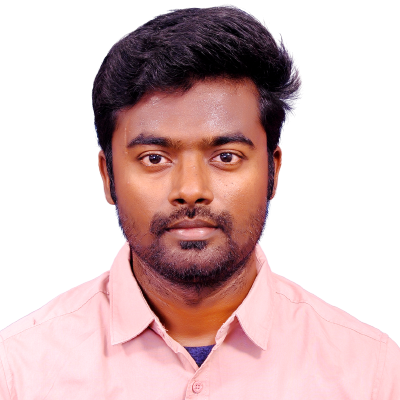
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getUTCDate() Methods), please comment here. I will help you immediately.