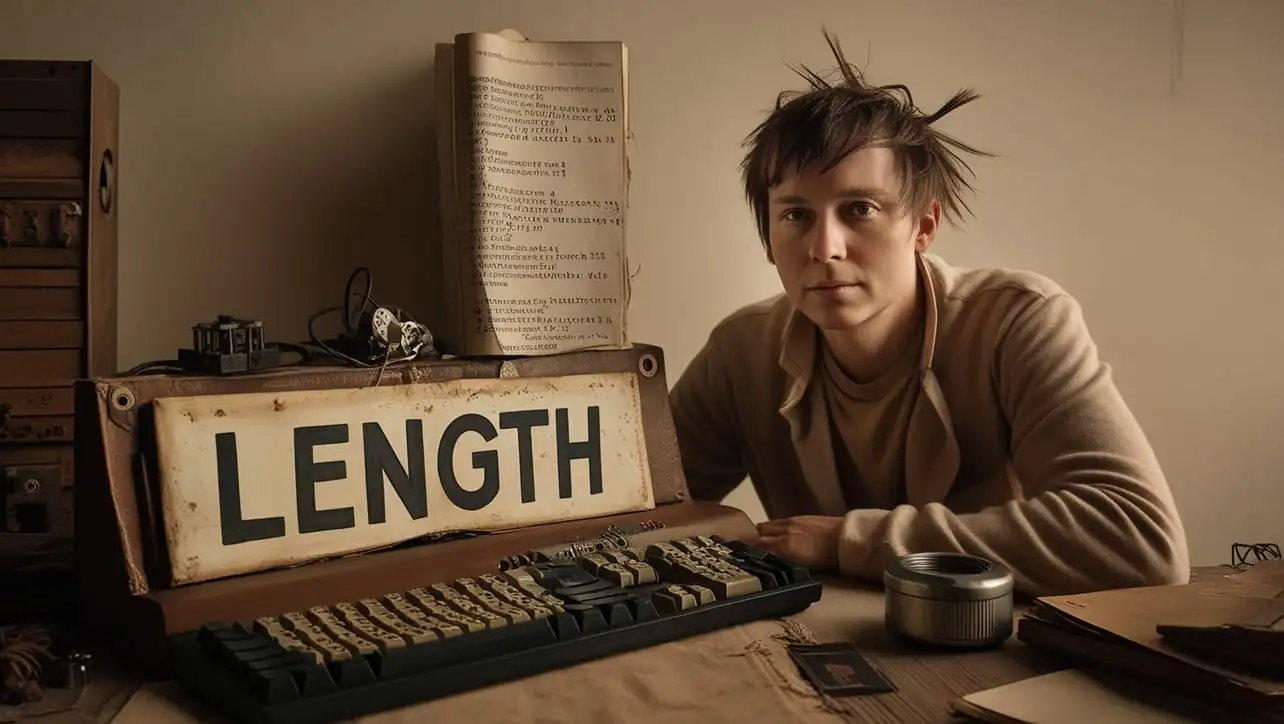
JS Date Methods
JavaScript Date getSeconds() Methods

Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, working with dates and times is made easy by the Date object. The getSeconds()
method is a handy feature that allows you to retrieve the seconds component of a given date.
This guide will walk you through the syntax, usage, best practices, and use cases of the getSeconds()
method.
🧠 Understanding getSeconds() Method
The getSeconds()
method, belonging to the Date object, returns the seconds value of a specified date according to the local time. The returned value is an integer between 0 and 59, representing the seconds.
💡 Syntax
The syntax for the getSeconds()
method is straightforward:
date.getSeconds();
- date: The Date object from which you want to extract the seconds.
📝 Example
Let's explore a simple example to illustrate the usage of the getSeconds()
method:
// Creating a new Date object
const now = new Date();
// Using getSeconds() to retrieve the seconds
const seconds = now.getSeconds();
console.log(`Current seconds: ${seconds}`);
In this example, we create a new Date object representing the current date and time. The getSeconds()
method is then employed to obtain the seconds value.
🏆 Best Practices
When working with the getSeconds()
method, consider the following best practices:
Ensure Valid Date Object:
Before using
getSeconds()
, make sure that the provided Date object is valid to avoid unexpected results.example.jsCopiedconst potentiallyInvalidDate = new Date('invalid date string'); if (!isNaN(potentiallyInvalidDate)) { const seconds = potentiallyInvalidDate.getSeconds(); console.log(`Seconds: ${seconds}`); } else { console.error('Invalid Date object.'); }
Handle Timezone Differences:
Be aware that
getSeconds()
returns the seconds in local time. If working with different timezones, consider using methods like getUTCSeconds() for coordinated universal time (UTC).
📚 Use Cases
Timer Functionality:
The
getSeconds()
method is often used in scenarios where precise timing is crucial, such as implementing a timer or stopwatch feature:example.jsCopiedfunction startTimer() { const startTime = new Date(); // ... other timer logic ... // Display seconds setInterval(() => { const seconds = startTime.getSeconds(); console.log(`Elapsed seconds: ${seconds}`); }, 1000); } startTimer();
Conditional Logic Based on Seconds:
You can leverage
getSeconds()
for conditional logic based on specific seconds of a minute:example.jsCopiedconst currentTime = new Date(); const currentSeconds = currentTime.getSeconds(); if (currentSeconds < 30) { console.log('First half of the minute.'); } else { console.log('Second half of the minute.'); }
🎉 Conclusion
The getSeconds()
method is a valuable tool for working with time-related functionality in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getSeconds()
method in your JavaScript projects.
👨💻 Join our Community:
Author
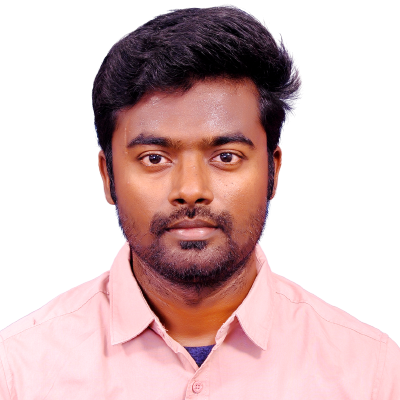
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getSeconds() Methods), please comment here. I will help you immediately.