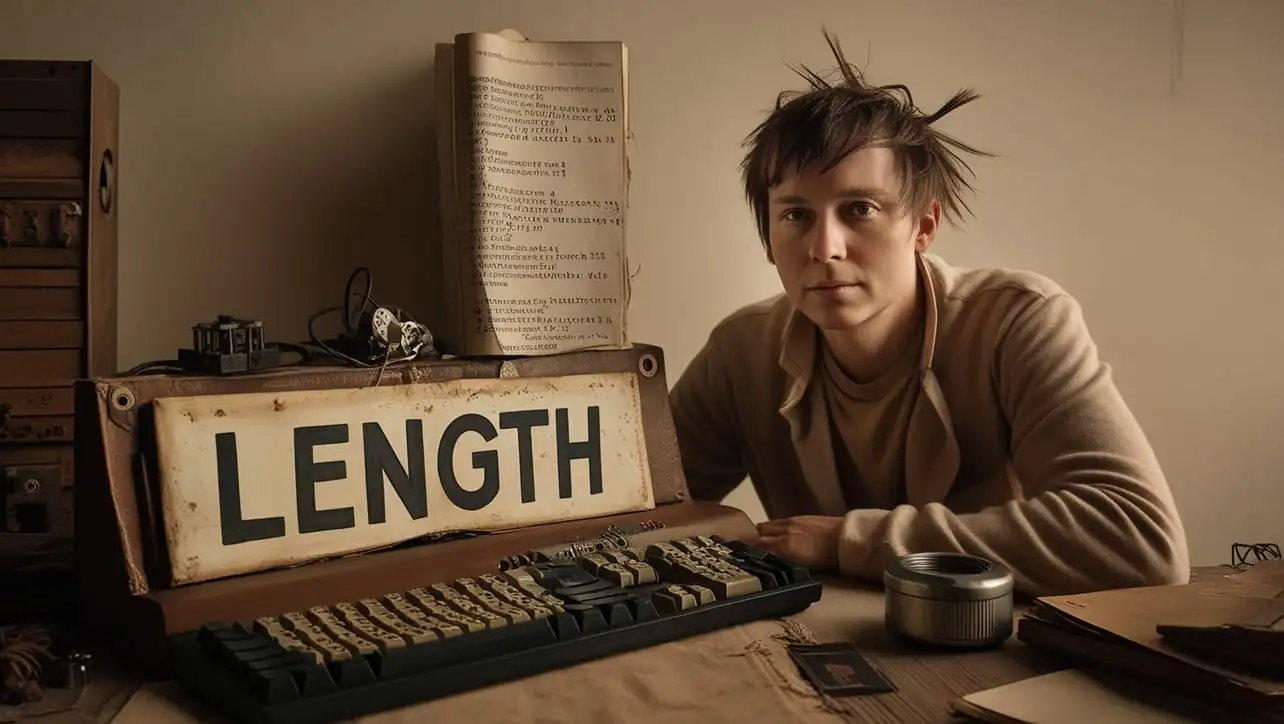
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- JS Console Methods
- JS Date Methods
- getDate()
- getDay()
- getFullYear()
- getHours()
- getMilliseconds()
- getMinutes()
- getMonth()
- getSeconds()
- getTime()
- getTimezoneOffset()
- getUTCDate()
- getUTCDay()
- getUTCFullYear()
- getUTCHours()
- getUTCMilliseconds()
- getUTCMinutes()
- getUTCMonth()
- getUTCSeconds()
- now()
- parse()
- setDate()
- setFullYear()
- setHours()
- setMilliseconds()
- setMinutes()
- setMonth()
- setSeconds()
- setTime()
- setUTCDate()
- setUTCFullYear()
- setUTCHours()
- setUTCMilliseconds()
- setUTCMinutes()
- setUTCMonth()
- setUTCSeconds()
- toDateString()
- toISOString()
- toJSON()
- toLocaleDateString()
- toLocaleString()
- toLocaleTimeString()
- toString()
- toTimeString()
- toUTCString()
- UTC()
- valueOf()
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Date getMonth() Methods
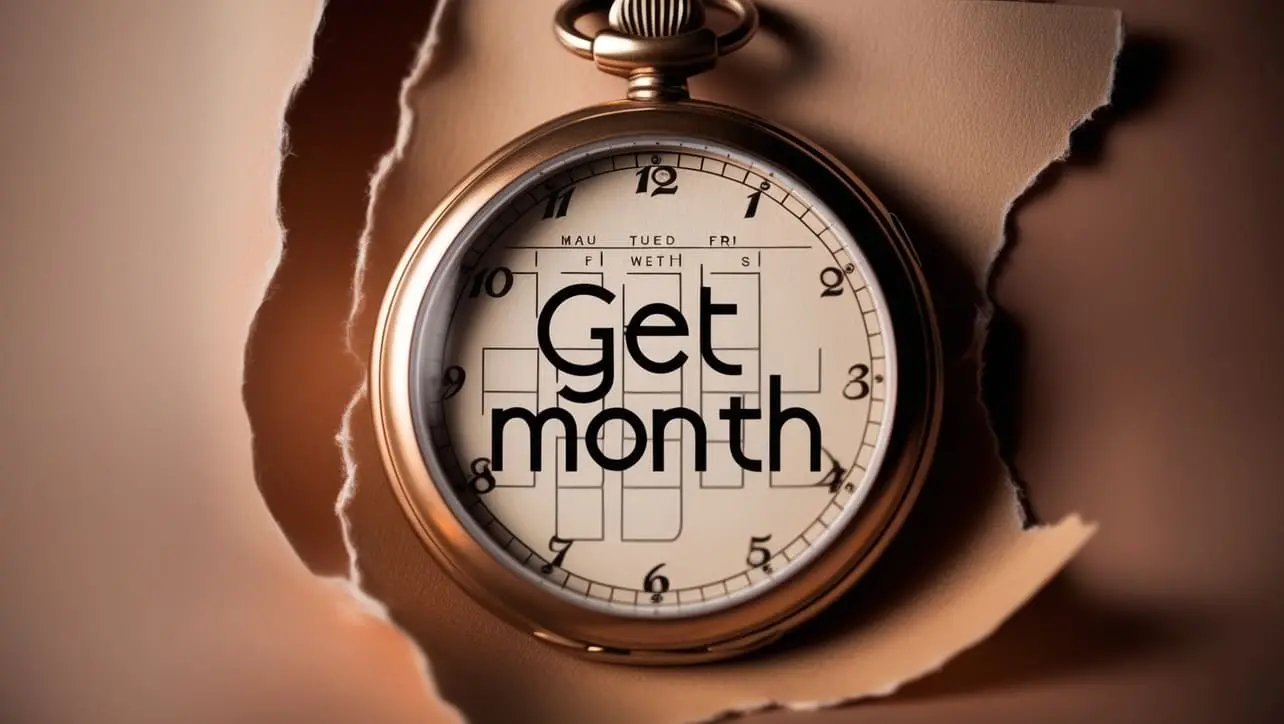
Photo Credit to CodeToFun
🙋 Introduction
Working with dates in JavaScript is a common task, and the getMonth()
method provides a valuable tool for extracting the month component from a Date object.
In this guide, we'll explore the syntax, usage, best practices, and practical examples of the getMonth()
method.
🧠 Understanding getMonth() Method
The getMonth()
method is used to retrieve the month component of a Date object, ranging from 0 (January) to 11 (December). This method simplifies the process of extracting and manipulating the month information.
💡 Syntax
The syntax for the getMonth()
method is straightforward:
date.getMonth();
- date: The Date object from which you want to retrieve the month.
📝 Example
Let's dive into a straightforward example to illustrate the usage of the getMonth()
method:
// Creating a Date object for February 15, 2024
const currentDate = new Date(2024, 1, 15);
// Using getMonth() to retrieve the month
const month = currentDate.getMonth();
console.log(month); // Output: 1 (February is represented by 1)
In this example, the getMonth() method is employed to obtain the month component from the currentDate object.
🏆 Best Practices
When working with the getMonth()
method, consider the following best practices:
Handle Zero-Based Index:
Keep in mind that the
getMonth()
method returns a zero-based index, where January is represented by 0 and December by 11. Adjust your code accordingly.example.jsCopiedconst monthIndex = currentDate.getMonth(); const monthNumber = monthIndex + 1; // Adjust for human-readable month numbers console.log(monthNumber);
Ensure Valid Date Objects:
Before using
getMonth()
, ensure that the Date object you are working with is valid. Invalid dates may lead to unexpected results.example.jsCopiedconst invalidDate = new Date('invalid date'); if (!isNaN(invalidDate.getTime())) { const month = invalidDate.getMonth(); console.log(month); } else { console.error('Invalid date!'); }
📚 Use Cases
Displaying Month Information:
The primary use case for the
getMonth()
method is to display or manipulate the month information in a user-friendly format:example.jsCopiedconst months = [ 'January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December' ]; const currentMonth = months[currentDate.getMonth()]; console.log(`The current month is ${currentMonth}`);
Comparing Dates:
You can use the
getMonth()
method for comparing the months of different Date objects:example.jsCopiedconst date1 = new Date(2024, 5, 10); // June const date2 = new Date(2024, 10, 20); // November if (date1.getMonth() === date2.getMonth()) { console.log('The dates are in the same month.'); } else { console.log('The dates are in different months.'); }
🎉 Conclusion
The getMonth()
method is a valuable tool when working with Date objects in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getMonth()
method in your JavaScript projects.
👨💻 Join our Community:
Author
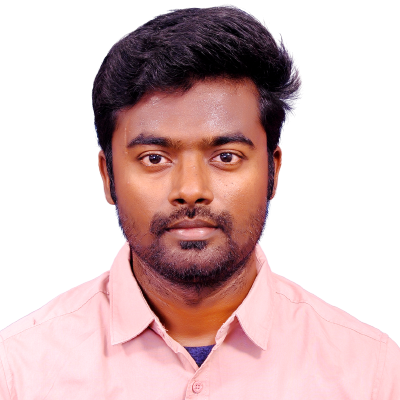
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getMonth() Methods), please comment here. I will help you immediately.