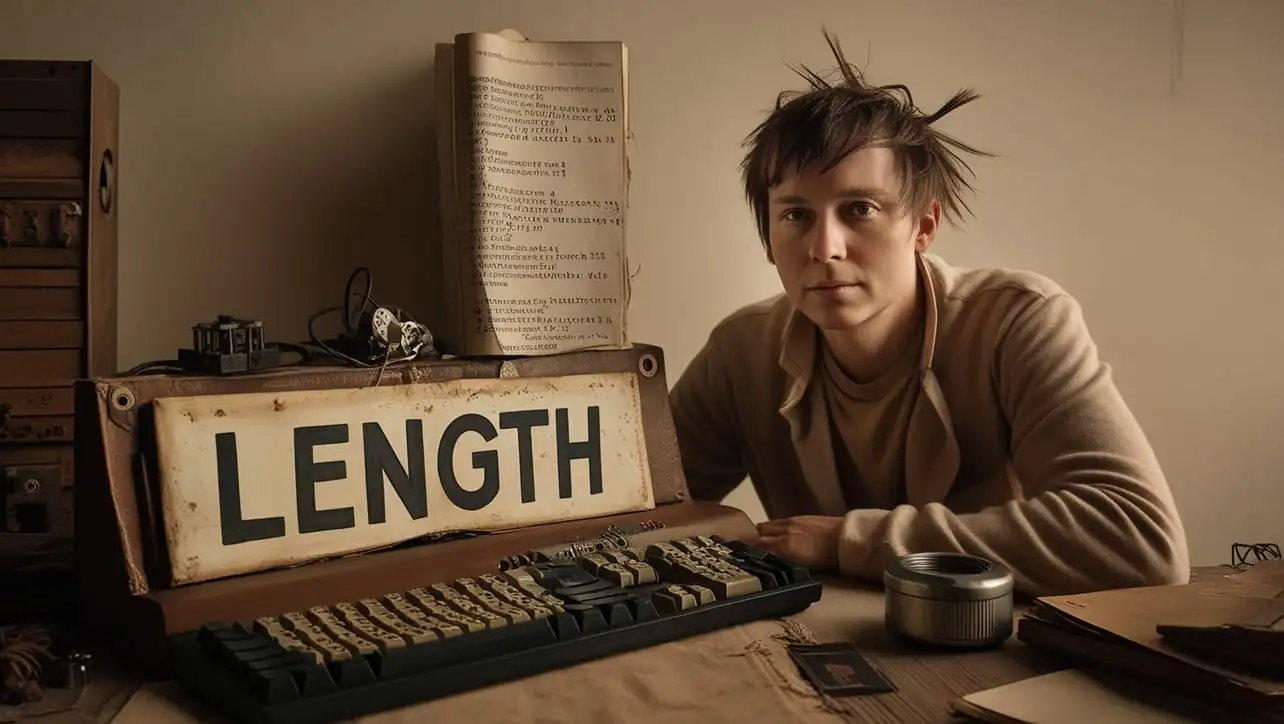
JS Date Methods
JavaScript Date getDay() Methods

Photo Credit to CodeToFun
🙋 Introduction
Working with dates in JavaScript becomes more efficient and intuitive with methods like getDay()
. This method, belonging to the Date object, provides an easy way to retrieve the day of the week.
In this guide, we'll explore the getDay()
method, covering its syntax, usage, best practices, and practical applications.
🧠 Understanding getDay() Method
The getDay()
method is used to extract the day of the week from a Date object. It returns a value between 0 and 6, where 0 represents Sunday, 1 represents Monday, and so forth. This method simplifies working with date-related logic and enables you to tailor your applications based on the day of the week.
💡 Syntax
The syntax for the getDay()
method is straightforward:
date.getDay();
- date: The Date object from which you want to extract the day of the week.
📝 Example
Let's delve into a basic example to illustrate the usage of the getDay()
method:
// Create a Date object for February 26, 2024 (a Tuesday)
const today = new Date('2024-02-26');
// Using getDay() to retrieve the day of the week
const dayOfWeek = today.getDay();
console.log(`Today is a ${getDayName(dayOfWeek)}.`);
In this example, the getDay()
method is applied to a Date object, and a custom function getDayName() is used to convert the numeric result into the corresponding day name.
function getDayName(dayIndex) {
const days = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'];
return days[dayIndex];
}
🏆 Best Practices
When working with the getDay()
method, consider the following best practices:
Check Validity:
Ensure that the Date object is valid before using
getDay()
to avoid unexpected results.example.jsCopiedconst invalidDate = new Date('invalid-date-string'); if (!isNaN(invalidDate.getTime())) { const dayOfWeek = invalidDate.getDay(); console.log(`Day of the week: ${getDayName(dayOfWeek)}`); } else { console.error('Invalid Date!'); }
Use a Helper Function:
Consider creating a helper function, like getDayName() in the example, to enhance code readability.
📚 Use Cases
Dynamic Content Based on Day:
Adjusting content dynamically based on the day of the week is a common use case:
example.jsCopiedconst welcomeMessage = () => { const today = new Date(); const dayOfWeek = today.getDay(); if (dayOfWeek === 1 || dayOfWeek === 5) { return 'Happy coding day!'; } else { return 'Enjoy your day!'; } }; console.log(welcomeMessage());
Scheduling and Notifications:
In applications involving scheduling or notifications, the
getDay()
method can be used to determine specific actions on particular days.example.jsCopiedconst scheduleMeeting = () => { const today = new Date(); const dayOfWeek = today.getDay(); if (dayOfWeek === 2 || dayOfWeek === 4) { console.log('Meeting scheduled for today!'); } else { console.log('No meeting today.'); } }; scheduleMeeting();
🎉 Conclusion
The getDay()
method proves to be a valuable tool for handling date-related operations in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getDay()
method in your JavaScript projects.
👨💻 Join our Community:
Author
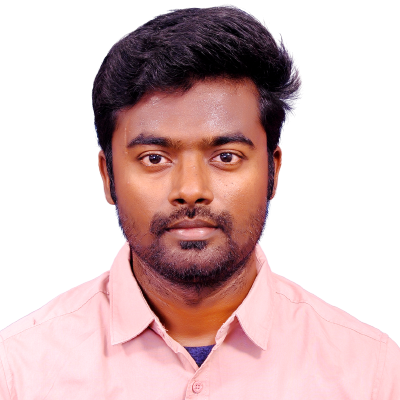
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getDay() Methods), please comment here. I will help you immediately.