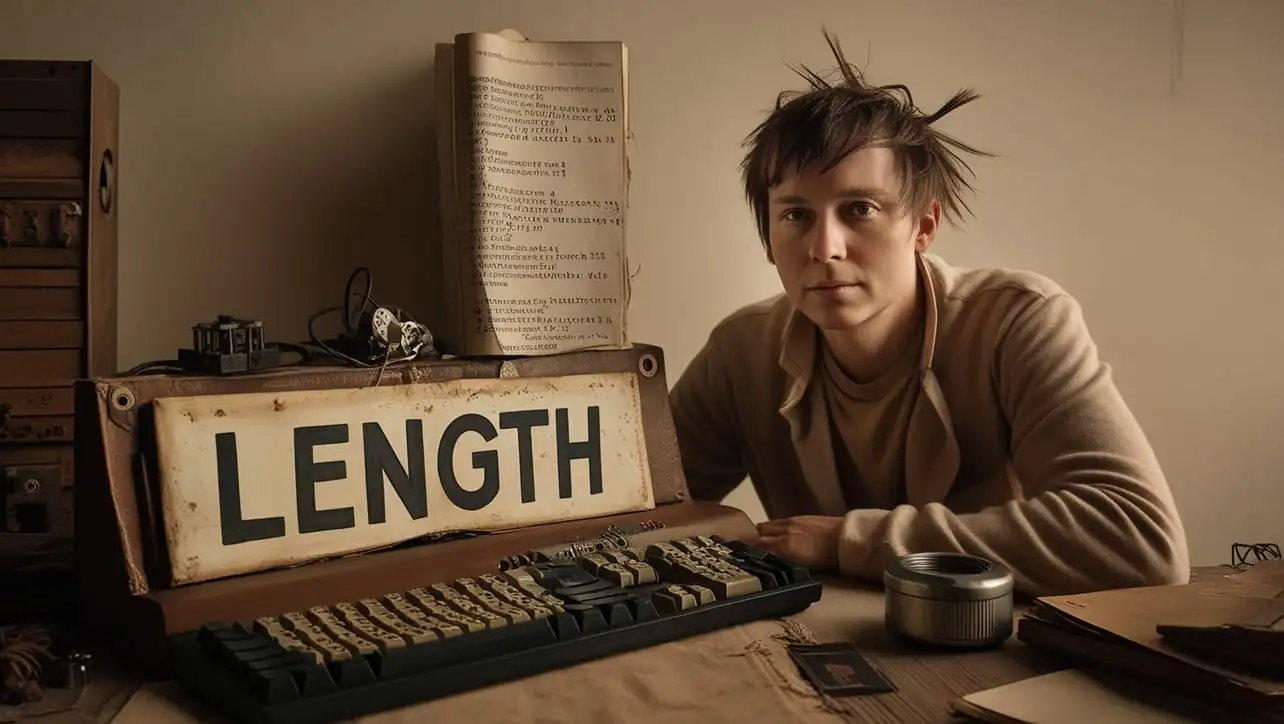
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- JS Console Methods
- JS Date Methods
- getDate()
- getDay()
- getFullYear()
- getHours()
- getMilliseconds()
- getMinutes()
- getMonth()
- getSeconds()
- getTime()
- getTimezoneOffset()
- getUTCDate()
- getUTCDay()
- getUTCFullYear()
- getUTCHours()
- getUTCMilliseconds()
- getUTCMinutes()
- getUTCMonth()
- getUTCSeconds()
- now()
- parse()
- setDate()
- setFullYear()
- setHours()
- setMilliseconds()
- setMinutes()
- setMonth()
- setSeconds()
- setTime()
- setUTCDate()
- setUTCFullYear()
- setUTCHours()
- setUTCMilliseconds()
- setUTCMinutes()
- setUTCMonth()
- setUTCSeconds()
- toDateString()
- toISOString()
- toJSON()
- toLocaleDateString()
- toLocaleString()
- toLocaleTimeString()
- toString()
- toTimeString()
- toUTCString()
- UTC()
- valueOf()
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Date getDate() Methods
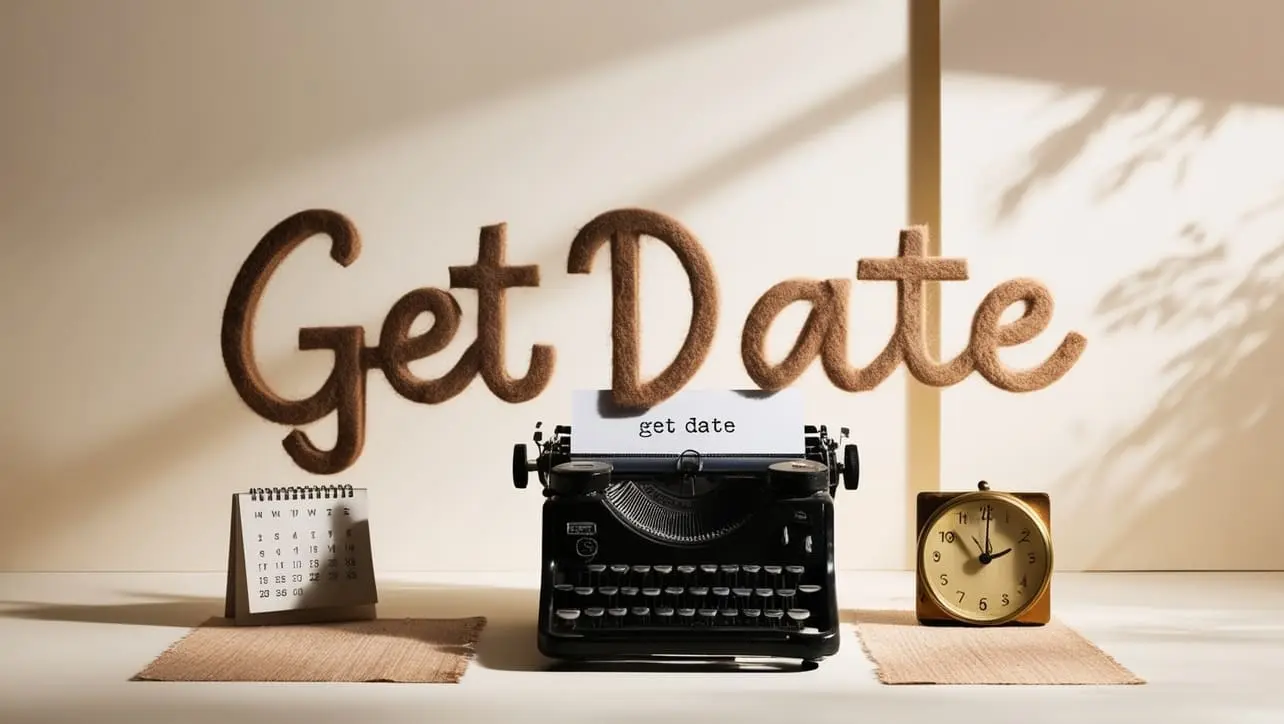
Photo Credit to CodeToFun
🙋 Introduction
Working with dates is a common requirement in JavaScript applications, and the getDate()
method provides a convenient way to retrieve the day of the month from a Date object.
In this comprehensive guide, we'll explore the getDate()
method, understand its syntax, and discover how to use it effectively in various scenarios.
🧠 Understanding getDate() Method
The getDate()
method is a part of the JavaScript Date object, allowing you to extract the day of the month as a numeric value. The day is represented as an integer between 1 and 31.
💡 Syntax
The syntax for the getDate()
method is straightforward:
dateObj.getDate();
- dateObj: The Date object from which you want to retrieve the day of the month.
📝 Example
Let's dive into a practical example to illustrate the usage of the getDate()
method:
// Create a new Date object
const today = new Date();
// Get the current day of the month
const dayOfMonth = today.getDate();
console.log(`Today is the ${dayOfMonth}th day of the month.`);
In this example, we use the getDate()
method to obtain the day of the month from the current date.
🏆 Best Practices
When working with the getDate()
method, consider the following best practices:
Check for Valid Date Objects:
Before using
getDate()
, ensure that the object is a valid Date instance to prevent unexpected errors.example.jsCopiedif (today instanceof Date && !isNaN(today)) { const dayOfMonth = today.getDate(); console.log(`Today is the ${dayOfMonth}th day of the month.`); } else { console.error('Invalid Date object.'); }
Locale Considerations:
Be mindful of potential issues related to time zones and locales, especially when working with user-specific date displays.
📚 Use Cases
Building a Date Display Function:
The
getDate()
method can be incorporated into a function to create a user-friendly date display:example.jsCopiedfunction displayDateInfo(date) { const day = date.getDate(); const month = date.toLocaleString('default', { month: 'long' }); const year = date.getFullYear(); console.log(`Today is ${month} ${day}, ${year}.`); } // Example usage const currentDate = new Date(); displayDateInfo(currentDate);
Calculating Future Dates:
You can use the
getDate()
method along with other date manipulation methods to calculate future dates:example.jsCopiedconst daysToAdd = 7; const futureDate = new Date(); futureDate.setDate(futureDate.getDate() + daysToAdd); console.log(`The date ${daysToAdd} days from now will be: ${futureDate}`);
🎉 Conclusion
The getDate()
method is a valuable tool in handling date-related operations in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the getDate()
method in your JavaScript projects.
👨💻 Join our Community:
Author
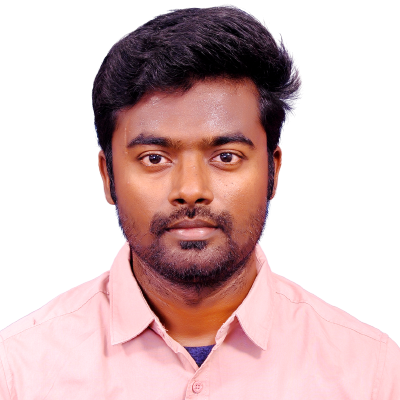
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date getDate() Methods), please comment here. I will help you immediately.