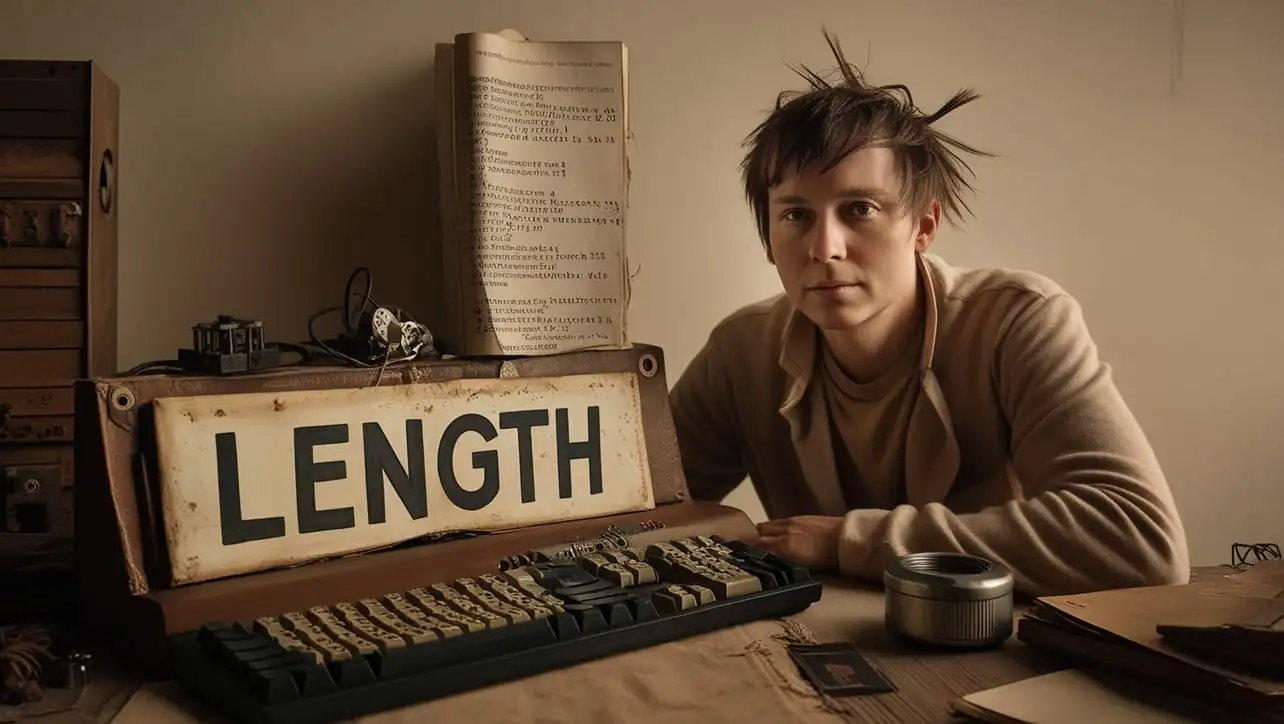
JavaScript Cookies Write
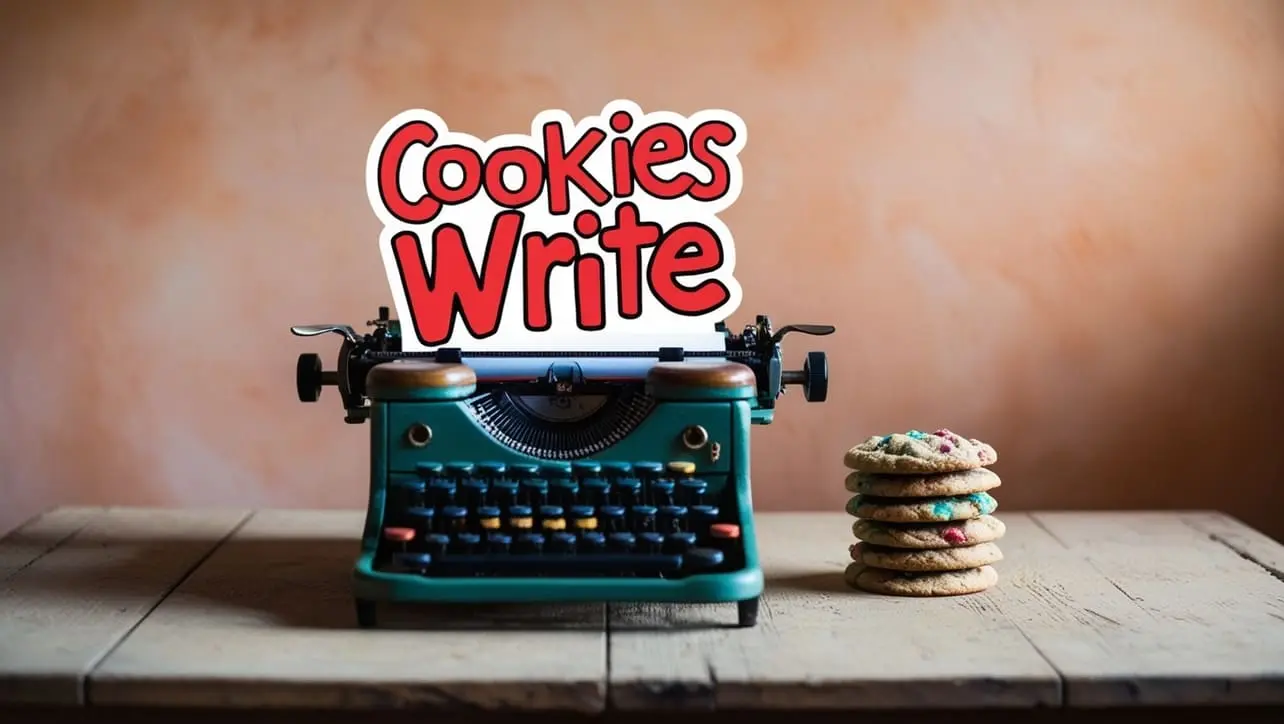
Photo Credit to CodeToFun
Introduction
Cookies are small data files stored by the browser to remember information between sessions. In JavaScript, cookies can be written to store user preferences, session information, and other small data on the client-side. Cookies can be scoped to specific pages or be made available to all pages within a domain. Learning how to manage cookies properly will improve both user experience and application functionality.
What Are Cookies?
Cookies are small key-value pairs stored by the browser, which persist across page reloads and even browser sessions. They can be used for storing session information, preferences, or other small bits of data. Cookies can either be scoped to a specific page or be made available across the entire domain.
How to Write a Cookie in JavaScript
Writing a cookie in JavaScript is as simple as assigning a string to document.cookie
. Here’s the basic syntax for writing a cookie:
document.cookie = "username=JohnDoe";
This creates a cookie with the name username
and value
JohnDoe. However, without additional attributes, this cookie is only valid for the current session and will be removed when the browser is closed.
Setting Cookies for Specific Pages vs All Pages
You can control the scope of a cookie by specifying its path
attribute. This defines which parts of your website can access the cookie:
Specific Page:
By setting the path to a specific page, the cookie will only be accessible on that page.
JavascriptCopieddocument.cookie = "user=JohnDoe; path=/about";
This cookie is only available on the
/about
page of your site.All Pages:
By setting the path to
/
, the cookie will be accessible on all pages within the domain.JavaScriptCopieddocument.cookie = "user=JohnDoe; path=/";
This cookie will be available on every page within the website.
Cookie Attributes
When writing cookies, several important attributes can be specified:
- expires: Sets the expiration date of the cookie. If not set, the cookie will expire when the browser is closed.
- max-age: Specifies the time in seconds after which the cookie will expire.
- path: Defines the URL path where the cookie is accessible (e.g.,
/
,/about
). - domain: Limits the cookie to a specific domain.
- secure: Ensures the cookie is only sent over HTTPS connections.
- SameSite: Prevents the cookie from being sent with cross-site requests, improving security.
Here’s an example of setting a cookie with multiple attributes:
document.cookie = "username=JohnDoe; expires=Fri, 31 Dec 2024 23:59:59 GMT; path=/; secure; SameSite=Lax";
Modifying Cookies
To modify a cookie, simply rewrite it with the same name and a new value or updated attributes:
document.cookie = "username=JaneDoe; expires=Fri, 31 Dec 2024 23:59:59 GMT; path=/";
This will update the username
cookie to JaneDoe
and preserve the expiration date and path.
Common Pitfalls
- Overwriting Cookies: Writing a new cookie does not overwrite existing cookies unless the name matches.
- Path Issues: If you don’t set the
path
attribute properly, cookies might not be accessible across different pages. - Size Limit: Browsers typically limit cookie size to around 4KB, and exceeding this limit will prevent the cookie from being written.
- Security: Always use the
secure
attribute for cookies that contain sensitive data to ensure they are only sent over HTTPS.
Example
Here’s a complete example demonstrating how to write a cookie for all pages, set its expiration date, and scope it to a specific page:
<!DOCTYPE html>
<html>
<head>
<title>Write a Cookie Example</title>
</head>
<body>
<h1>JavaScript Write Cookie Example</h1>
<button id="setCookie">Set Cookie</button>
<script>
document.getElementById('setCookie').addEventListener('click', function() {
// Set a cookie with a name, value, and expiration date
const now = new Date();
now.setTime(now.getTime() + (7 * 24 * 60 * 60 * 1000)); // 7 days
const expires = "expires=" + now.toUTCString();
document.cookie = "user=JohnDoe; " + expires + "; path=/";
alert("Cookie has been set!");
});
</script>
</body>
</html>
Conclusion
Writing cookies in JavaScript is a simple yet powerful way to store client-side data. By understanding how to use cookies effectively with attributes such as expiration dates and secure flags, you can create more robust and secure web applications. Be mindful of the potential limitations and security concerns when working with cookies.
👨💻 Join our Community:
Author
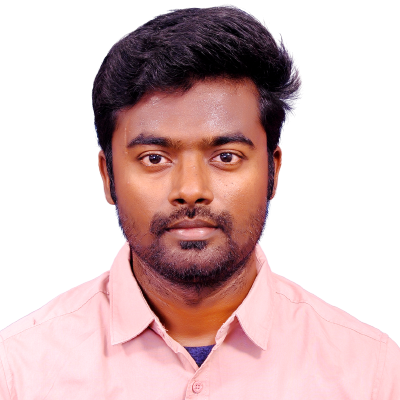
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Cookies Write), please comment here. I will help you immediately.