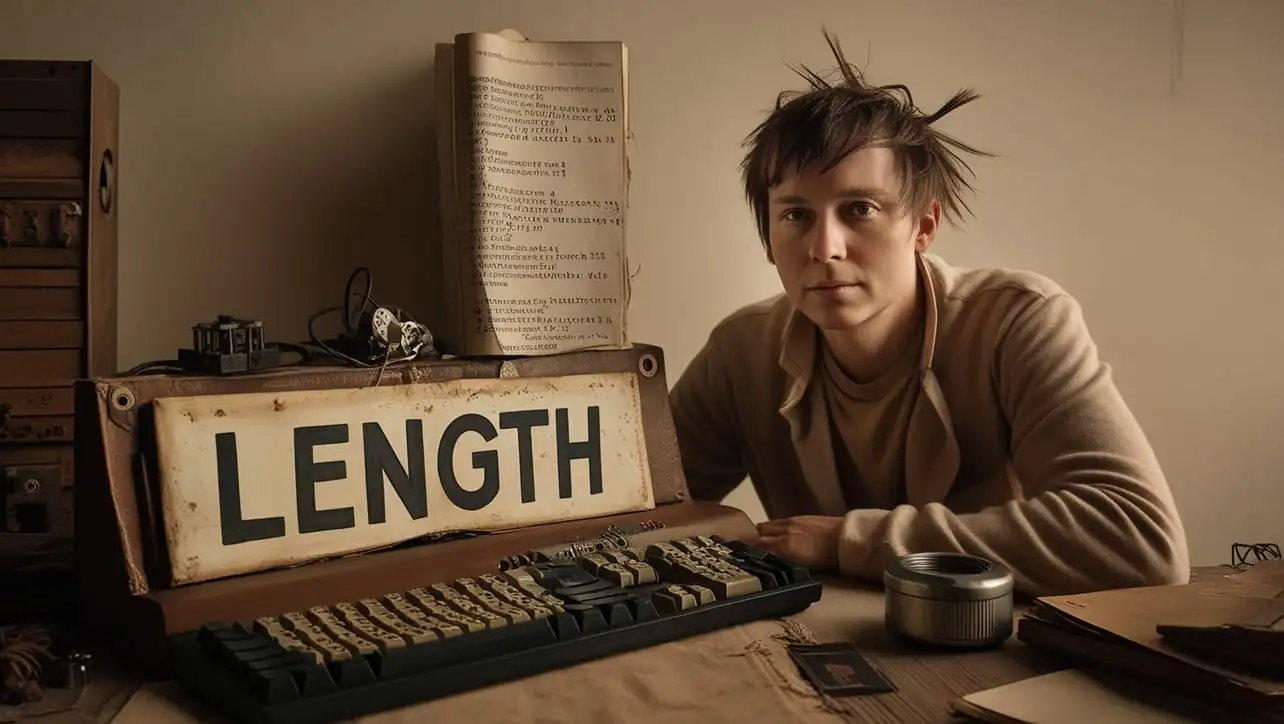
JavaScript Cookies Read
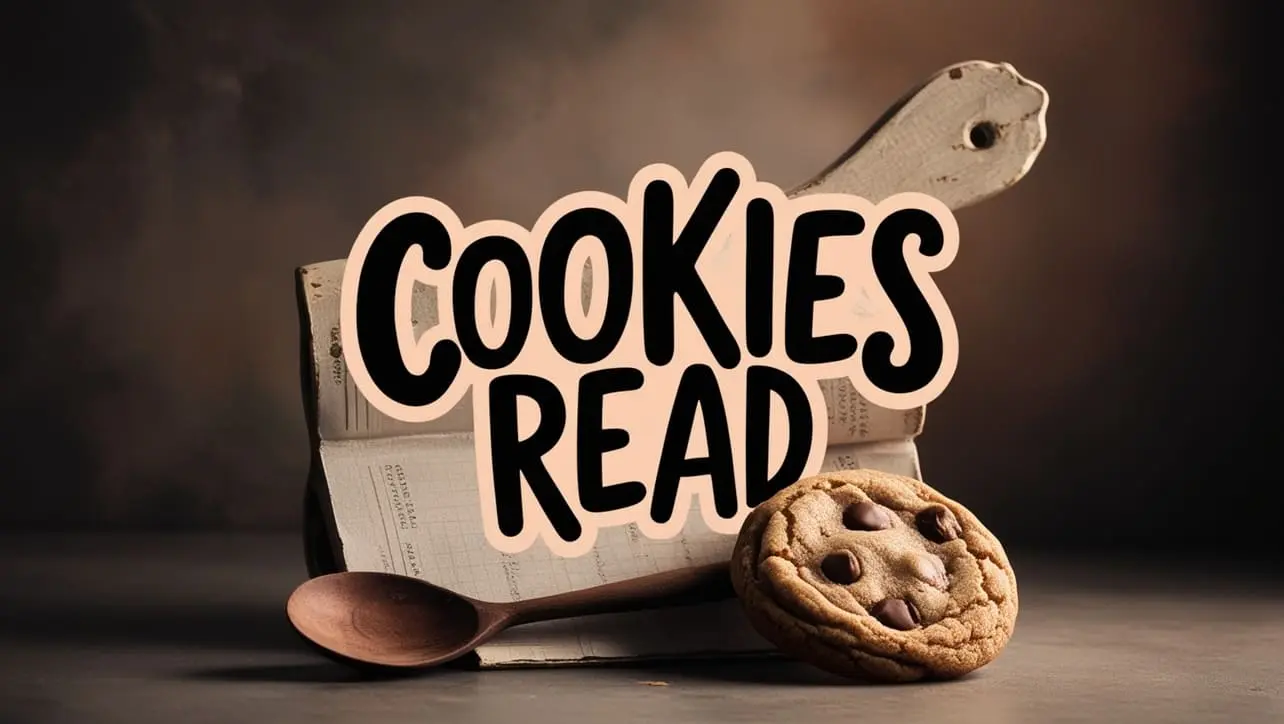
Photo Credit to CodeToFun
Introduction
Cookies are small data files stored on the client side, used to track and store information across different sessions. In JavaScript, you can easily create, read, and delete cookies.
This page focuses on how to read cookies using JavaScript, which is essential for retrieving stored data like user preferences or session details.
What Are Cookies?
Cookies are text files that store small amounts of data on the user's browser. They are sent from the server and stored in the user's browser, allowing the server to recognize returning users and store personalized settings or authentication tokens.
Why Read Cookies?
Reading cookies is crucial for:
- User Authentication: To verify if a user is logged in by checking authentication tokens.
- Personalization: Retrieving user preferences like theme or language.
- Tracking User Activity: Tracking session activity or other analytics.
How to Read Cookies
In JavaScript, cookies are stored in the document.cookie
property, which contains all the cookies for the current domain as a single string. Each cookie is represented as a key-value pair separated by semicolons.
Here’s how you can access cookies:
// Get all cookies as a string
const allCookies = document.cookie;
console.log(allCookies); // Output: "username=JohnDoe; theme=dark"
Accessing Specific Cookie Values
Since document.cookie
returns all cookies in one string, you need to manually parse it to retrieve the value of a specific cookie. A common way is to use a function that searches for the cookie name and extracts its value.
Here’s a simple function to read a specific cookie by name:
function getCookie(name) {
const cookies = document.cookie.split(';');
for (let cookie of cookies) {
let [key, value] = cookie.trim().split('=');
if (key === name) {
return value;
}
}
return null;
}
// Usage
const username = getCookie('username');
console.log(username); // Output: JohnDoe
Handling Special Characters
If your cookies contain special characters, spaces, or non-ASCII characters, they may be encoded. JavaScript uses encodeURIComponent
and decodeURIComponent
to handle these cases. When reading such cookies, you should decode the values:
function getCookie(name) {
const cookies = document.cookie.split(';');
for (let cookie of cookies) {
let [key, value] = cookie.trim().split('=');
if (key === name) {
return decodeURIComponent(value);
}
}
return null;
}
Common Pitfalls
- Case Sensitivity: Cookie names are case-sensitive. Make sure to match the exact name when retrieving them.
- Cookie Expiration: Cookies may expire and become inaccessible. Ensure you check the cookie's validity before trying to read it.
- Security: Cookies can store sensitive data, but they are inherently insecure. Avoid storing sensitive information like passwords directly in cookies.
Example
Here’s an example that reads a user's preferences (theme and username) from cookies and displays them on the page:
<!DOCTYPE html>
<html>
<head>
<title>Read Cookies Example</title>
</head>
<body>
<h1>Cookie Reader</h1>
<p id="welcome"></p>
<p id="theme"></p>
<script>
function getCookie(name) {
const cookies = document.cookie.split(';');
for (let cookie of cookies) {
let [key, value] = cookie.trim().split('=');
if (key === name) {
return decodeURIComponent(value);
}
}
return null;
}
// Display user info
const username = getCookie('username');
const theme = getCookie('theme');
document.getElementById('welcome').textContent = `Welcome, ${username || 'Guest'}!`;
document.getElementById('theme').textContent = `Theme: ${theme || 'default'}`;
</script>
</body>
</html>
Conclusion
Reading cookies in JavaScript is straightforward but requires some handling to parse and extract specific values.
By understanding how to retrieve cookies and handle special cases like encoding, you can effectively manage client-side data storage for various purposes like personalization and session management.
Join our Community:
Author
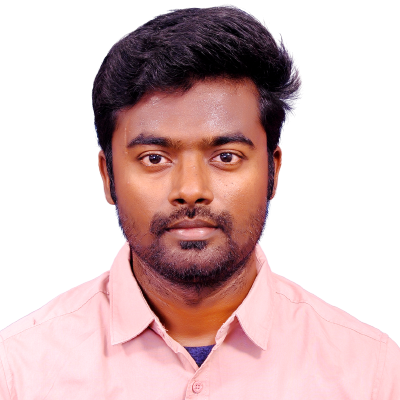
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Cookies Read), please comment here. I will help you immediately.