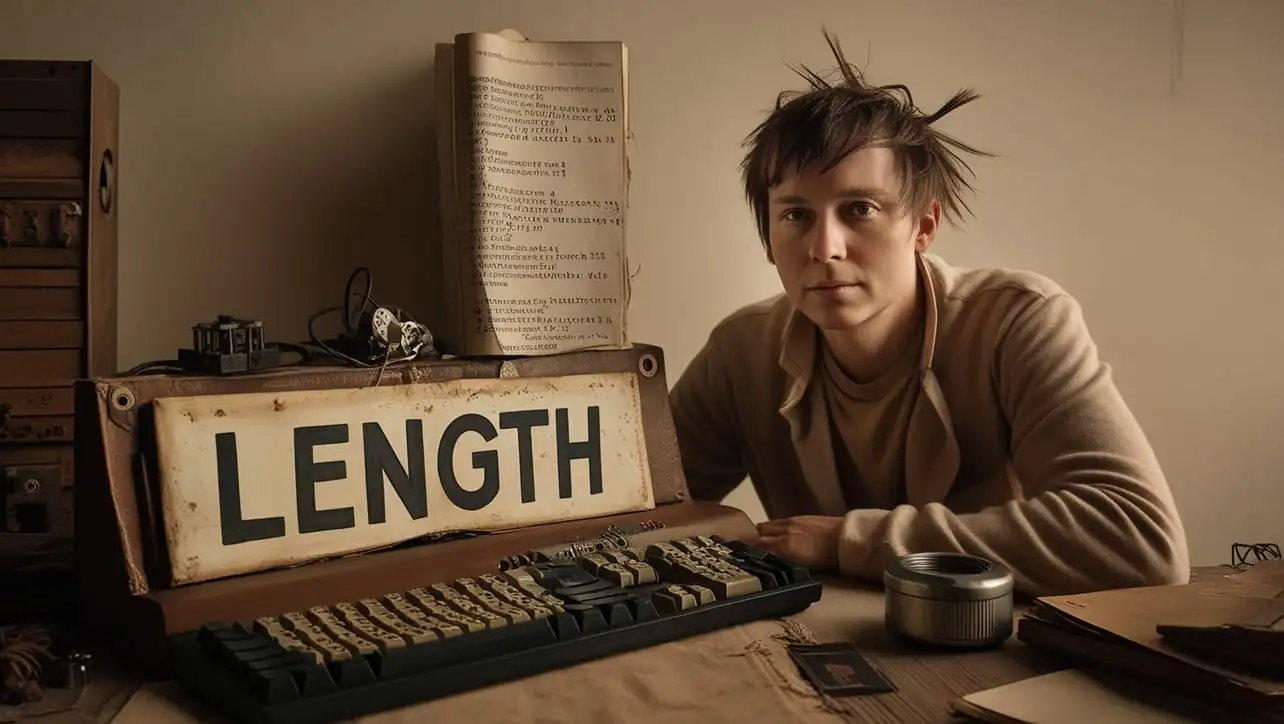
JavaScript Cookies Expires
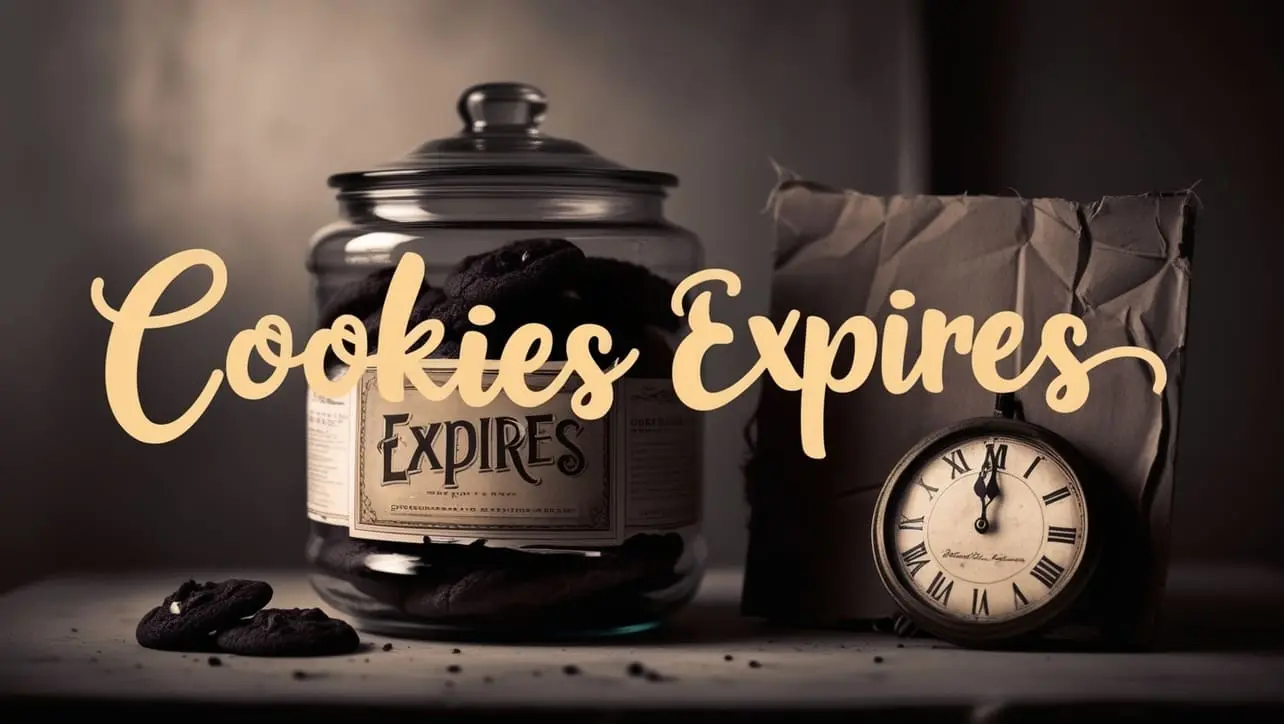
Photo Credit to CodeToFun
Introduction
Cookies are small data files stored on the user's computer, and they play a vital role in web development, especially for maintaining user sessions and preferences. One important feature of cookies is the ability to set an expiration date, which controls how long the cookie is retained by the browser.
What Are Cookies in JavaScript?
Cookies in JavaScript are used to store small amounts of data on the user's computer. They can store information such as user preferences, authentication tokens, or shopping cart data. Cookies can have an expiration date or last only for the current browser session (session cookies).
Setting Cookies with Expiration
To set a cookie in JavaScript with an expiration date, you use the document.cookie
property, appending an expiration date in UTC format. Here’s how you can set a cookie with an expiration date:
// Set a cookie that expires in 7 days
let date = new Date();
date.setTime(date.getTime() + (7 * 24 * 60 * 60 * 1000)); // 7 days in milliseconds
let expires = "expires=" + date.toUTCString();
document.cookie = "username=JohnDoe; " + expires + "; path=/";
In this example:
- The cookie
username=JohnDoe
is set. - The cookie is set to expire in 7 days.
path=/
means the cookie is accessible from any page on the site.
How Cookie Expiration Works
The expiration date of a cookie determines how long the browser retains it. If a cookie does not have an expiration date, it is considered a session cookie and will be deleted once the browser is closed. If an expiration date is set, the cookie is stored until the specified date and time.
Common Pitfalls
- Incorrect Date Format: The expiration date must be in UTC string format (e.g.,
expires=Thu, 18 Dec 2024 12:00:00 UTC
). Any other format will be ignored by the browser. - Time Zone Issues: Always use UTC time to avoid problems with different time zones.
- Cookies Not Being Set: If the path is incorrectly defined or missing, the cookie may not be available across all pages, especially when working with subdomains or different directory levels.
Reading Expiring Cookies
Reading cookies in JavaScript is simple, but you don’t get the expiration information when retrieving them. You can read a cookie using the document.cookie
property:
let cookies = document.cookie;
console.log(cookies); // Output: "username=JohnDoe"
To retrieve a specific cookie:
function getCookie(name) {
let cookieArr = document.cookie.split(';');
for(let i = 0; i < cookieArr.length; i++) {
let cookie = cookieArr[i].trim();
if (cookie.indexOf(name) == 0) {
return cookie.substring(name.length + 1);
}
}
return null;
}
This function will allow you to retrieve the value of the username
cookie if it exists.
Example
Here’s an example of setting a cookie with an expiration and retrieving it:
<!DOCTYPE html>
<html>
<head>
<title>Cookie Expiration Example</title>
</head>
<body>
<h1>JavaScript Cookies Expiration Example</h1>
<button onclick="setCookie('username', 'JohnDoe', 7)">Set Cookie</button>
<button onclick="alert(getCookie('username'))">Get Cookie</button>
<script>
function setCookie(name, value, days) {
let date = new Date();
date.setTime(date.getTime() + (days * 24 * 60 * 60 * 1000));
let expires = "expires=" + date.toUTCString();
document.cookie = name + "=" + value + "; " + expires + "; path=/";
}
function getCookie(name) {
let nameEQ = name + "=";
let cookieArray = document.cookie.split(';');
for(let i = 0; i < cookieArray.length; i++) {
let cookie = cookieArray[i].trim();
if (cookie.indexOf(nameEQ) == 0) {
return cookie.substring(nameEQ.length, cookie.length);
}
}
return null;
}
</script>
</body>
</html>
In this example:
- A cookie named
username
is set with a value ofJohnDoe
and expires in 7 days. - You can retrieve and display the value of the cookie using the second button.
Conclusion
Setting cookie expiration dates in JavaScript is a fundamental practice for maintaining user data across sessions. By understanding how to properly manage expiration times, you can control how long the cookie is stored in the user's browser, enabling you to enhance user experience and persist user data effectively.
👨💻 Join our Community:
Author
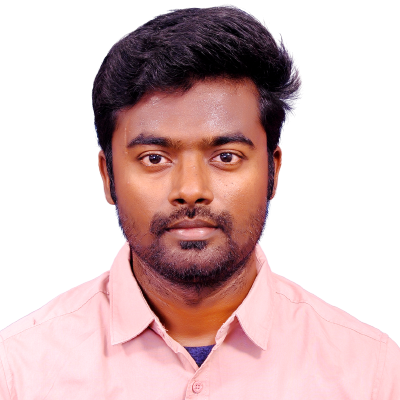
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Cookies Expires), please comment here. I will help you immediately.