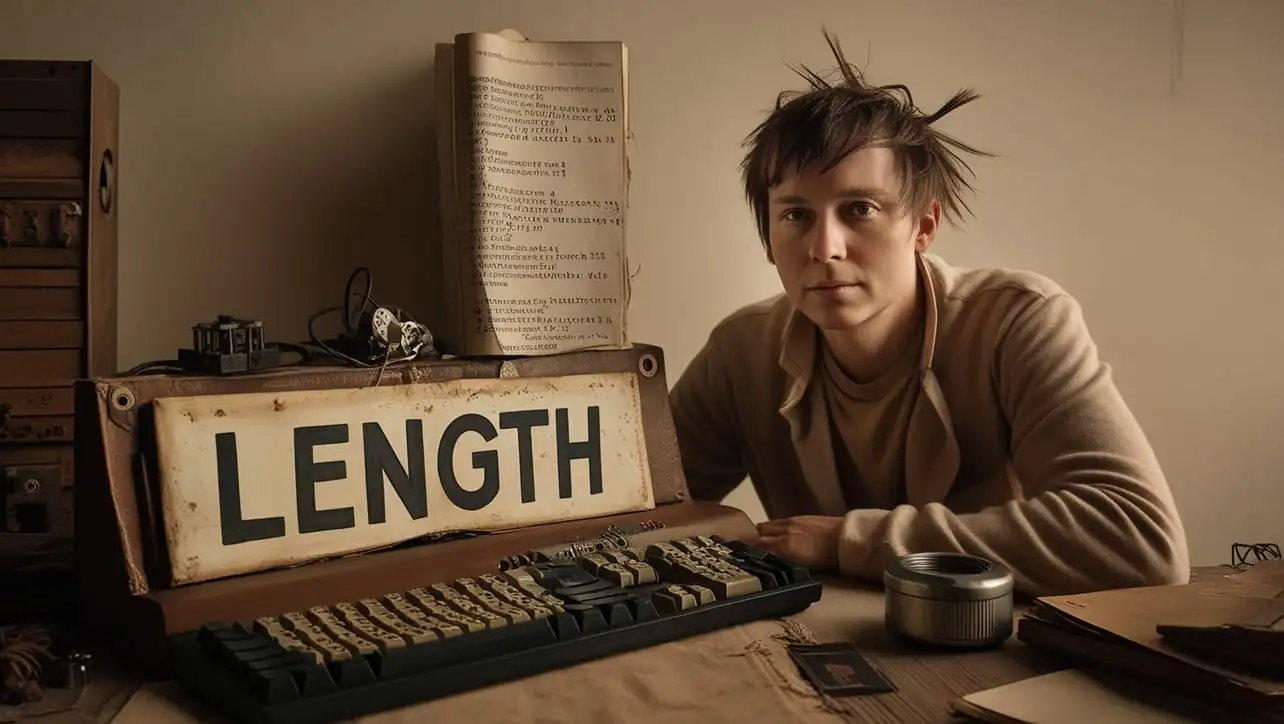
JS Window Methods
JavaScript Window confirm() Method
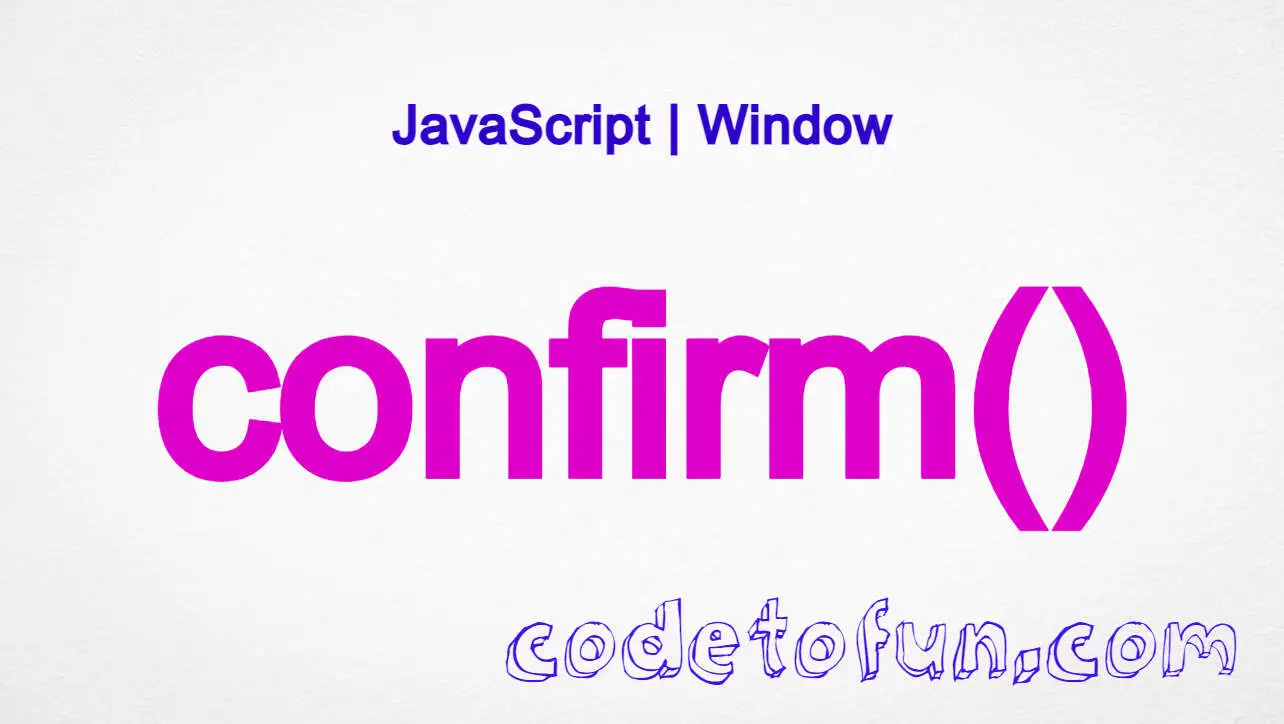
Photo Credit to CodeToFun
🙋 Introduction
The confirm()
method is a built-in function in JavaScript that creates a dialog box with a specified message and two buttons: OK and Cancel. This method is often used to obtain user confirmation before proceeding with a particular action on a webpage.
In this guide, we'll explore the syntax, usage, best practices, and practical examples of the confirm()
method.
🧠 Understanding confirm() Method
The confirm()
method is a part of the window object in the browser's JavaScript environment. It displays a modal dialog box with a message, an OK button, and a Cancel button. The dialog is a simple way to interact with users and prompt them to confirm or cancel an action.
💡 Syntax
The syntax for the confirm()
method is straightforward:
confirm(message);
- message: A string that will be displayed as the message in the dialog box.
The method returns a boolean value: true if the user clicks OK and false if the user clicks Cancel.
📝 Example
Let's consider a basic example of using the confirm()
method to confirm a user's intention to delete an item:
const userConfirmed = confirm("Are you sure you want to delete this item?");
if (userConfirmed) {
// Code to delete the item
console.log("Item deleted successfully.");
} else {
console.log("Deletion canceled by the user.");
}
In this example, the confirm()
method prompts the user with the specified message, and the result (true or false) is stored in the userConfirmed variable.
🏆 Best Practices
When working with the confirm()
method, consider the following best practices:
Provide Clear Messages:
Ensure that the message in the dialog box clearly conveys the action the user is confirming or canceling.
example.jsCopiedconst userConfirmed = confirm("Do you want to submit the form?");
Use the Result Carefully:
Be mindful of how you use the boolean result returned by
confirm()
to avoid unintended consequences.example.jsCopiedif (confirm("Do you want to proceed?")) { // Code to execute if the user confirms } else { // Code to execute if the user cancels }
Consider Accessibility:
Dialog boxes may not be accessible to all users, so ensure there are alternative ways to perform critical actions.
📚 Use Cases
Confirming Form Submission:
example.jsCopiedconst submitForm = confirm("Do you want to submit the form?"); if (submitForm) { document.getElementById("myForm").submit(); } else { console.log("Form submission canceled by the user."); }
Confirming Navigation:
example.jsCopiedconst leavePage = confirm("Do you want to leave this page?"); if (leavePage) { window.location.href = "new-page.html"; } else { console.log("Navigation canceled by the user."); }
🎉 Conclusion
The confirm()
method provides a simple yet effective way to interact with users and obtain their confirmation for specific actions on a webpage.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the confirm()
method in your JavaScript projects.
👨💻 Join our Community:
Author
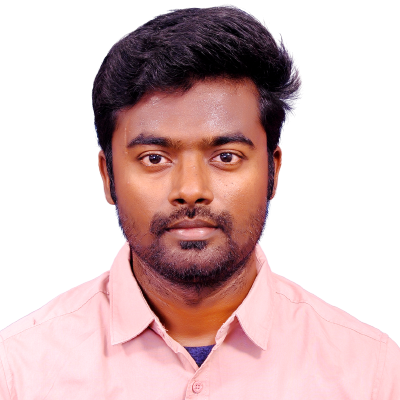
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Window confirm() Method), please comment here. I will help you immediately.