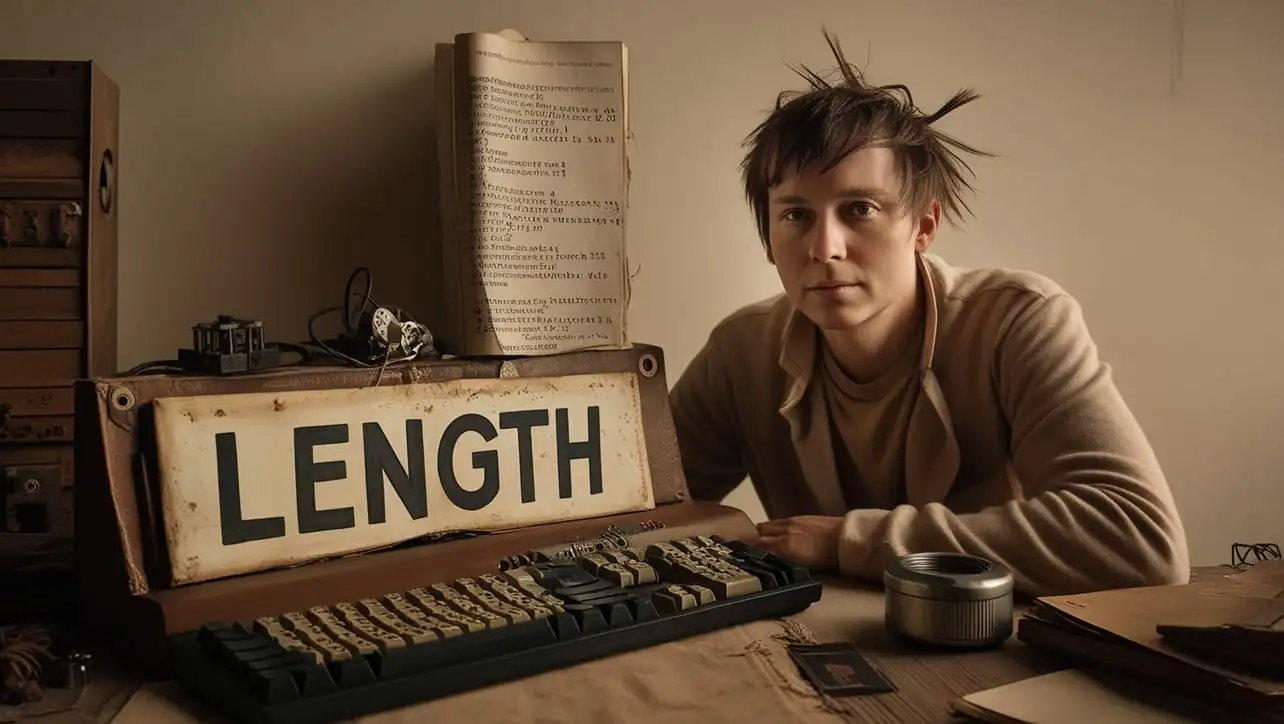
JavaScript Window close() Method
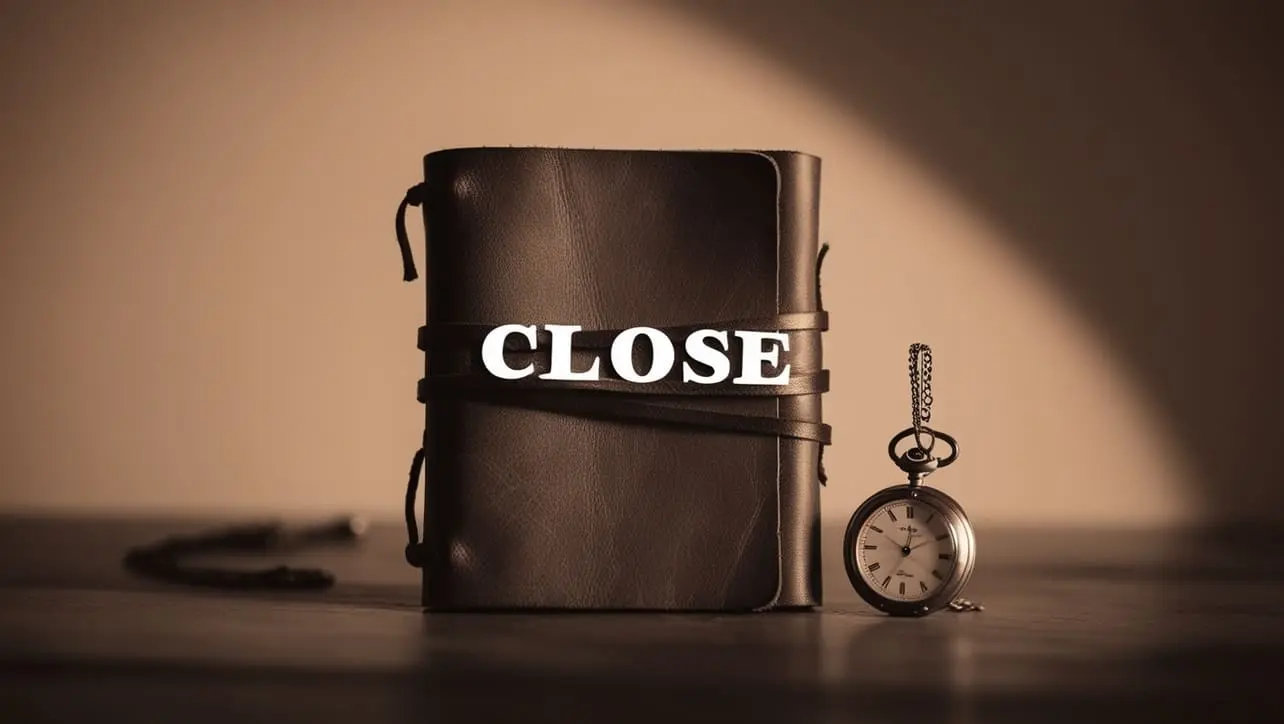
Photo Credit to CodeToFun
Introduction
In the world of web development, effective control over browser windows is crucial. The window.close()
method is a powerful tool that allows you to programmatically close the current browser window or a window that was opened by a script.
This guide will provide an in-depth exploration of the window.close()
method, covering its syntax, usage, best practices, and practical examples.
Understanding close() Method
The window.close()
method is used to close the current browser window or a window that was opened by JavaScript using the window.open() method.
Syntax
The syntax for the close() method is straightforward:
window.close();
This method does not require any parameters.
Example
Let's start with a basic example of using the window.close()
method to close the current window:
function closeCurrentWindow() {
window.close();
}
In this example, calling closeCurrentWindow() will close the current browser window.
Best Practices
When working with the close()
method, consider the following best practices:
User Interaction:
Browsers typically allow the
window.close()
method to be called only as a result of a user action, such as a button click. Attempting to close a window without user interaction might be blocked by browsers to prevent abuse.example.jsCopiedconst closeButton = document.getElementById('closeButton'); closeButton.addEventListener('click', () => { window.close(); });
Popup Windows:
When using window.open() to create popup windows, ensure that the same script that opened the window has permission to close it. Cross-origin policies may prevent closing windows opened by scripts from different origins.
example.jsCopied// Open a popup window const popupWindow = window.open('popup.html', 'Popup', 'width=300,height=200'); // Close the popup window after a delay setTimeout(() => { popupWindow.close(); }, 5000);
In this example, a popup window is opened, and the
window.close()
method is used to close it after a delay of 5 seconds.
Use Cases
Closing a Popup Window:
The most common use case for
window.close()
is closing popup windows:example.jsCopied// Open a popup window const popupWindow = window.open('popup.html', 'Popup', 'width=300,height=200'); // Close the popup window after a delay setTimeout(() => { popupWindow.close(); }, 5000);
In this example, a popup window is opened, and the
window.close()
method is used to close it after a delay of 5 seconds.Close Button in HTML:
Utilizing the
window.close()
method with an HTML button for a clean user interface:example.jsCopied<button id="closeButton">Close Window</button> <script> const closeButton = document.getElementById('closeButton'); closeButton.addEventListener('click', () => { window.close(); }); </script>
This example showcases a close button in an HTML document that, when clicked, triggers the closure of the window.
Conclusion
The window.close()
method is a valuable tool for managing browser windows in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the close()
method in your JavaScript projects.
Join our Community:
Author
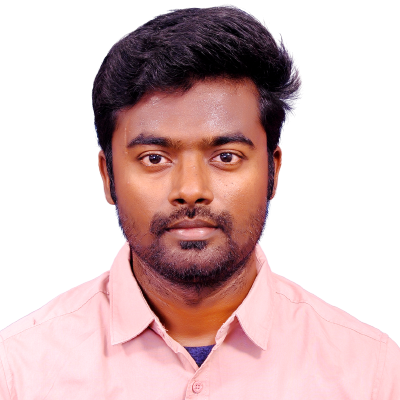
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Window close() Method), please comment here. I will help you immediately.