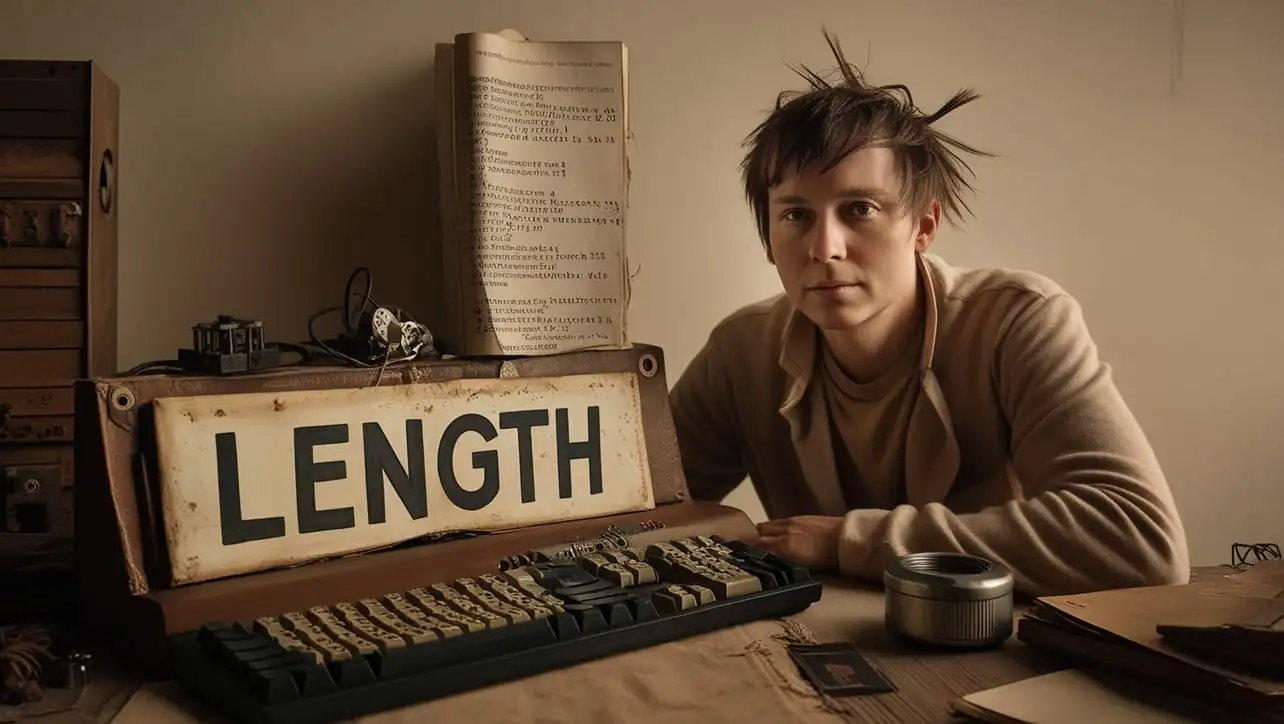
JS Window Methods
JavaScript Window atob() Method

Photo Credit to CodeToFun
🙋 Introduction
In the realm of web development, handling data encoding and decoding is a common task. JavaScript provides the window.atob()
method as a means to decode Base64-encoded strings.
In this guide, we'll delve into the syntax, usage, best practices, and practical scenarios where the window.atob()
method can be a valuable asset.
🧠 Understanding atob() Method
The window.atob()
method is specifically designed to decode a Base64-encoded string. Base64 encoding is a binary-to-text encoding scheme that is commonly used for data exchange in various contexts, such as embedding images in HTML, handling binary data in JSON, and more.
💡 Syntax
The syntax for the atob() method is straightforward:
window.atob(encodedString);
- encodedString: The Base64-encoded string that you want to decode.
📝 Example
Let's explore a simple example to illustrate the usage of the window.atob()
method:
// Base64-encoded string
const encodedData = 'SGVsbG8gd29ybGQh';
// Decoding with atob()
const decodedData = window.atob(encodedData);
console.log(decodedData);
// Output: 'Hello world!'
In this example, the Base64-encoded string is decoded using the window.atob()
method, revealing the original text.
🏆 Best Practices
When working with the atob()
method, consider the following best practices:
Error Handling:
Ensure that the input string is a valid Base64-encoded string to avoid errors during decoding.
example.jsCopiedfunction safeBase64Decode(encodedString) { try { return window.atob(encodedString); } catch (error) { console.error('Invalid Base64-encoded string:', error.message); return null; } }
Cross-Browser Compatibility:
The
window.atob()
method is widely supported in modern browsers, but it's a good practice to check for compatibility in case of specific requirements.example.jsCopiedif (window.atob) { // Safe to use window.atob() in this environment const decodedData = window.atob(encodedData); console.log(decodedData); } else { console.error('window.atob() method not supported in this environment.'); }
📚 Use Cases
Handling Authentication Tokens:
The
window.atob()
method is often employed when dealing with authentication tokens that are Base64-encoded. Decoding such tokens allows developers to extract information like user details or permissions.example.jsCopiedconst authToken = 'eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c'; // Decoding authentication token const decodedToken = window.atob(authToken); console.log(decodedToken);
Image Data Handling:
Base64 encoding is commonly used for embedding images in HTML or CSS. The
window.atob()
method can be used to decode such encoded image data.example.jsCopiedconst base64Image = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAA...'; // Truncated for brevity // Decoding image data const decodedImageData = window.atob(base64Image); console.log(decodedImageData);
🎉 Conclusion
The window.atob()
method is a valuable tool in the JavaScript toolkit for decoding Base64-encoded strings.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the atob()
method in your JavaScript projects.
👨💻 Join our Community:
Author
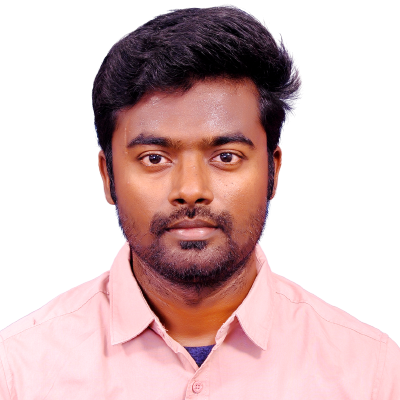
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Window atob() Method), please comment here. I will help you immediately.