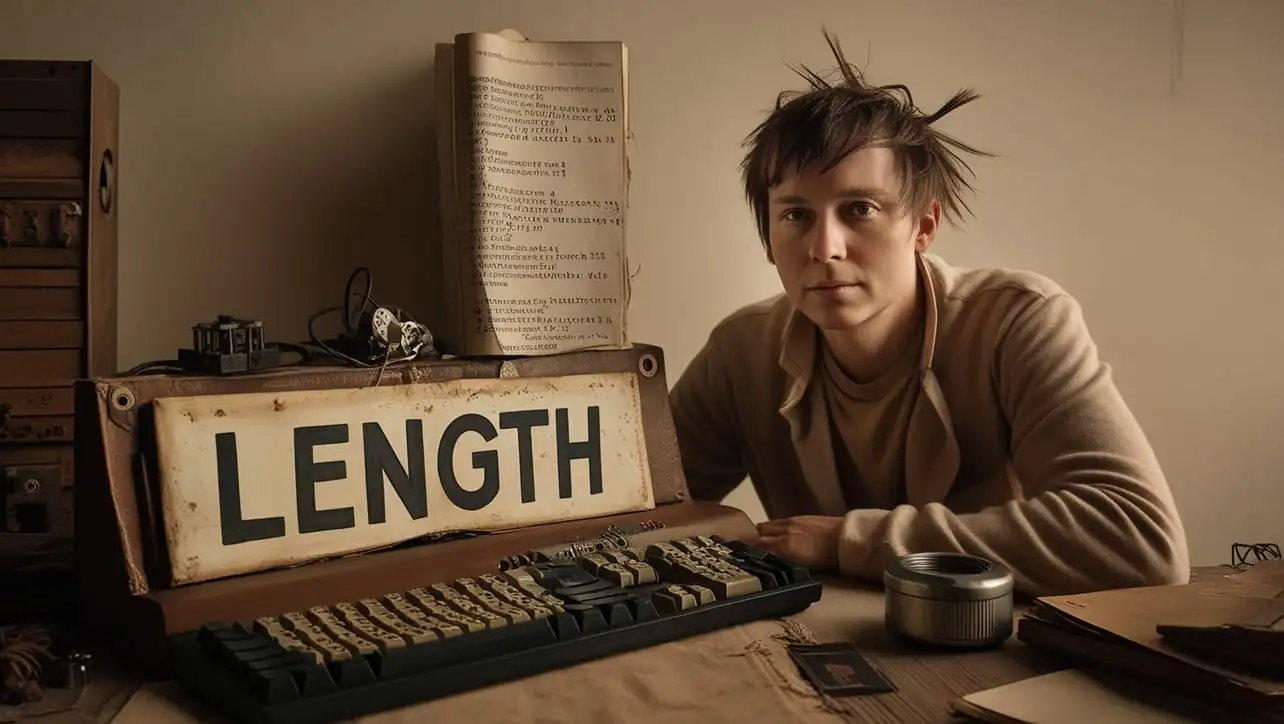
JS Array Methods
JavaScript Array values() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays, known for their versatility, offer various methods to manipulate and traverse their elements. The values()
method is a valuable addition, providing a straightforward way to iterate over the values of an array.
In this guide, we'll explore the syntax, usage, best practices, and practical applications of the values()
method.
🧠 Understanding values() Method
The values()
method returns a new Array Iterator object containing the values for each index in the array. It simplifies the process of accessing and working with the elements directly without concerning yourself with the indices.
💡 Syntax
The syntax for the values()
method is straightforward:
array.values();
- array: The array for which you want to retrieve the values.
📝 Example
Let's jump into an example to illustrate the usage of the values()
method:
// Sample array
const fruits = ['apple', 'orange', 'banana', 'grape', 'kiwi'];
// Using values() to iterate through values
const iterator = fruits.values();
for (const value of iterator) {
console.log(value);
}
In this example, the values()
method is employed to obtain an iterator, and a for...of loop is used to iterate through the values of the fruits array.
🏆 Best Practices
When working with the values()
method, consider the following best practices:
Destructuring:
Use array destructuring to conveniently retrieve values during iteration.
example.jsCopiedfor (const fruit of fruits.values()) { console.log(`Fruit: ${fruit}`); }
Compatibility Checking:
Verify the compatibility of the
values()
method in your target environments, especially if you need to support older browsers.example.jsCopied// Check if the values() method is supported if (Array.prototype.values) { // Use the method safely const iterator = fruits.values(); for (const value of iterator) { console.log(value); } } else { console.error('values() method not supported in this environment.'); }
📚 Use Cases
Iterating and Modifying Elements:
The
values()
method is beneficial when you want to iterate through array elements and modify them directly:example.jsCopiedfor (const value of fruits.values()) { console.log(`Original Fruit: ${value}`); } // Modify the array directly fruits[2] = 'pineapple'; for (const value of fruits.values()) { console.log(`Modified Fruit: ${value}`); }
Checking Array Equality:
You can use
values()
for comparing the values of two arrays:example.jsCopiedconst anotherFruits = ['apple', 'orange', 'banana', 'grape', 'kiwi']; const areArraysEqual = (arr1, arr2) => { const values1 = arr1.values(); const values2 = arr2.values(); for (const value1 of values1) { const value2 = values2.next().value; if (value1 !== value2) { return false; } } return true; }; console.log(areArraysEqual(fruits, anotherFruits)); // Output: true
🎉 Conclusion
The values()
method enhances the array manipulation capabilities in JavaScript, offering a concise way to iterate over array values.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the values()
method in your JavaScript projects.
👨💻 Join our Community:
Author
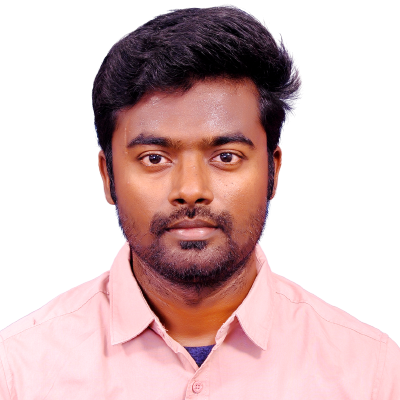
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array values() Method), please comment here. I will help you immediately.