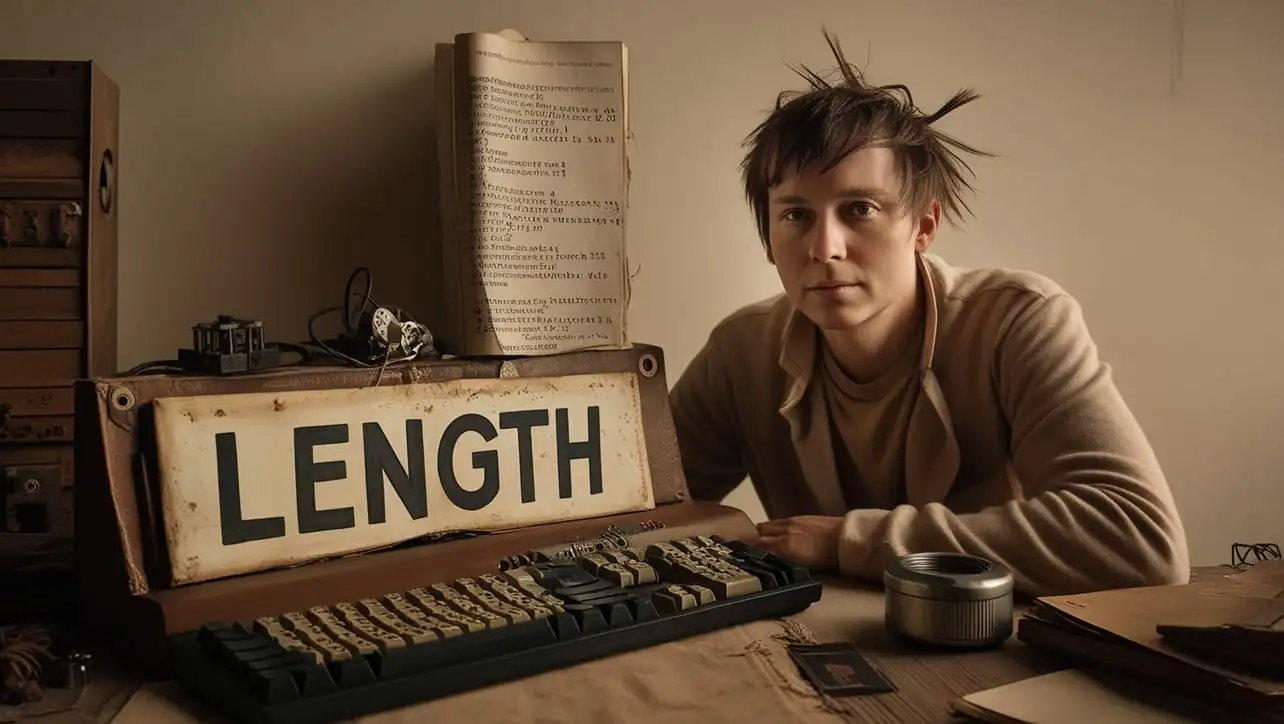
JS Array Methods
JavaScript Array splice() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays offer a myriad of methods to manipulate their contents, and the splice()
method stands out as a powerful tool for adding, removing, and replacing elements at specified positions.
In this comprehensive guide, we will delve into the splice()
method, exploring its syntax, practical examples, best practices, and use cases.
🧠 Understanding splice() Method
The splice()
method is a versatile tool that allows you to modify an array by adding or removing elements at a specified index. It can also be used to replace existing elements with new ones.
💡 Syntax
The syntax for the splice()
method is straightforward:
array.splice(start, deleteCount, item1, item2, ...);
- array: The array on which to perform the splice operation.
- start: The index at which to start changing the array.
- deleteCount: The number of elements to remove from the array.
- item1, item2, ...: The elements to add to the array, starting at the start index.
📝 Example
Let's dive into a basic example to illustrate the usage of the splice()
method:
// Sample array
const fruits = ['apple', 'banana', 'orange', 'kiwi'];
// Using splice() to remove and add elements
fruits.splice(1, 2, 'grape', 'melon');
console.log(fruits);
// Output: ['apple', 'grape', 'melon', 'kiwi']
In this example, the splice()
method removes two elements starting from index 1 and adds grape and melon in their place.
🏆 Best Practices
When working with the splice()
method, consider the following best practices:
Original Array Preservation:
If you want to keep the original array intact, consider creating a copy before using
splice()
.example.jsCopiedconst originalArray = [...fruits];
Avoid Negative Indices:
Be cautious with negative start indices, as they count from the end of the array. Ensure that the calculated index is within a valid range.
example.jsCopiedconst startIndex = -2; const adjustedIndex = startIndex < 0 ? fruits.length + startIndex : startIndex; fruits.splice(adjustedIndex, 1);
📚 Use Cases
Removing Elements:
The primary use case for
splice()
is removing elements from an array:example.jsCopiedconst removedElements = fruits.splice(1, 2); console.log(removedElements); // Output: ['grape', 'melon'] // fruits array: ['apple', 'kiwi']
Inserting Elements:
You can use
splice()
to insert elements at a specific index:example.jsCopiedfruits.splice(1, 0, 'pineapple', 'peach'); console.log(fruits); // Output: ['apple', 'pineapple', 'peach', 'kiwi']
Replacing Elements:
To replace elements at a particular index, use
splice()
with a non-zero deleteCount:example.jsCopiedfruits.splice(1, 2, 'pear', 'cherry'); console.log(fruits); // Output: ['apple', 'pear', 'cherry', 'kiwi']
🎉 Conclusion
The splice()
method empowers you to dynamically modify the contents of JavaScript arrays, offering flexibility in adding, removing, and replacing elements.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the splice()
method in your JavaScript projects.
👨💻 Join our Community:
Author
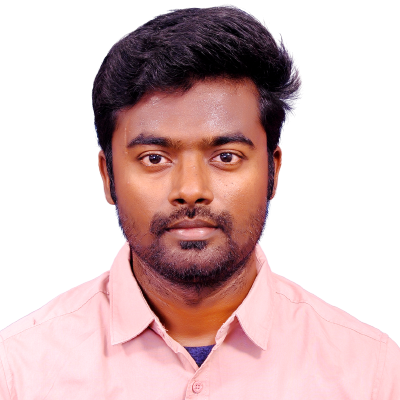
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array splice() Method), please comment here. I will help you immediately.