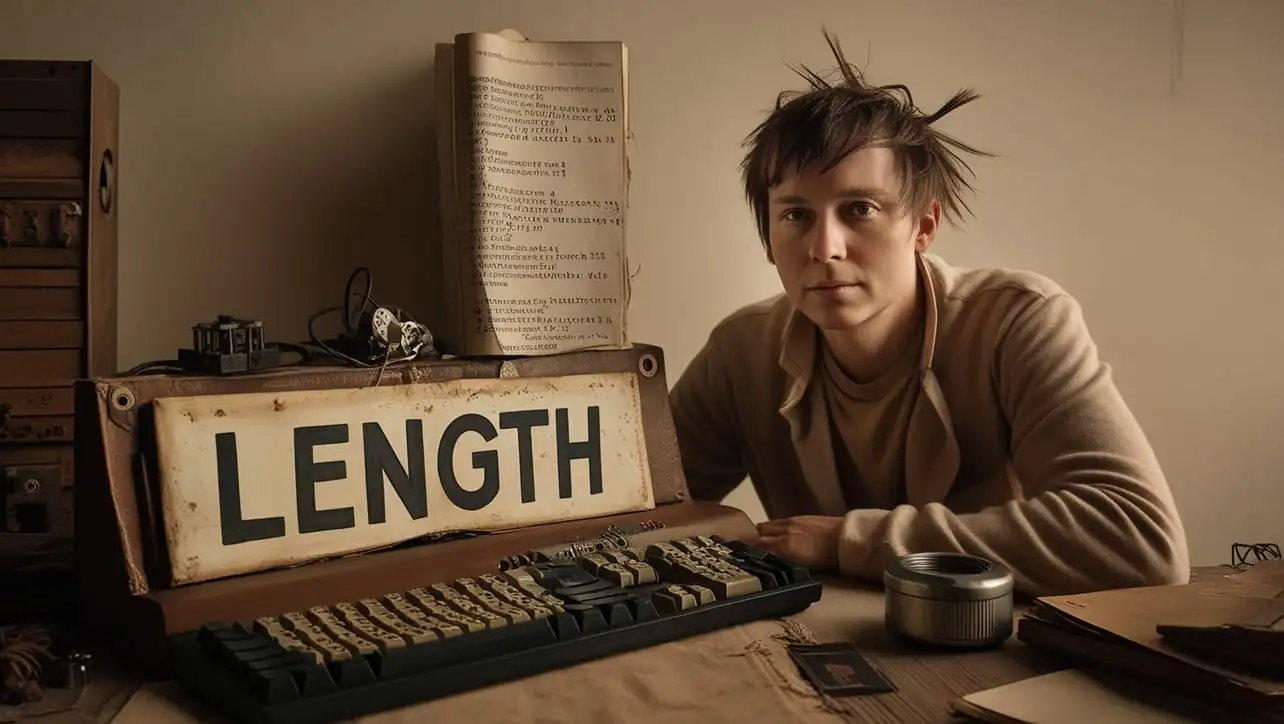
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array sort() Method
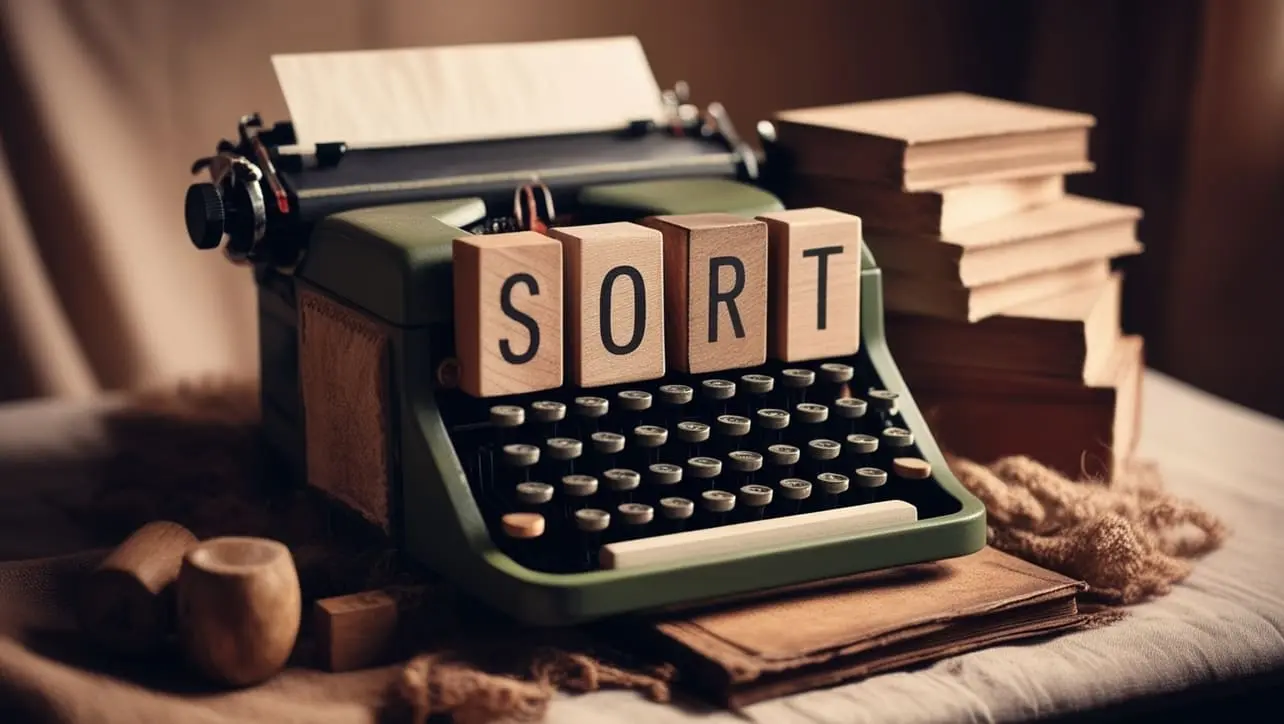
Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays offer a rich set of methods for manipulating and organizing data, and the sort()
method is a powerful tool for arranging elements within an array.
In this comprehensive guide, we will explore the intricacies of the sort()
method, its syntax, and provide practical examples to enhance your understanding.
🧠 Understanding sort() Method
The sort()
method is used to arrange the elements of an array either in ascending or descending order. By default, it sorts elements as strings, which may lead to unexpected results when working with numbers. However, a custom sorting function can be applied to address this.
💡 Syntax
The syntax for the sort()
method is straightforward:
array.sort(compareFunction);
- array: The array to be sorted.
- compareFunction (optional): An optional function that defines the sort order. If omitted, the array elements are converted to strings, and the default sorting order is applied.
📝 Example
Let's delve into a basic example to illustrate the default behavior of the sort()
method:
// Sample array of numbers
const numbers = [8, 3, 6, 2, 9];
// Using sort() without a compare function
numbers.sort();
console.log(numbers); // Output: [2, 3, 6, 8, 9]
In this example, the sort()
method arranges the numbers in ascending order by converting them to strings. To properly sort numeric values, a custom compare function can be utilized.
🏆 Best Practices
When working with the sort()
method, consider the following best practices:
Numeric Sorting:
To sort numeric values correctly, always provide a custom compare function.
example.jsCopiedconst numericSort = (a, b) => a - b; numbers.sort(numericSort);
Immutable Sorting:
Be cautious when using
sort()
directly on the original array, as it modifies the array in place. If immutability is crucial, consider creating a copy before sorting.example.jsCopiedconst sortedNumbers = [...numbers].sort();
📚 Use Cases
Sorting Objects by a Property:
The
sort()
method is versatile and can be applied to arrays of objects. For instance, sorting an array of objects by a specific property:example.jsCopiedconst products = [ { name: 'Laptop', price: 1200 }, { name: 'Smartphone', price: 800 }, { name: 'Tablet', price: 500 } ]; // Sorting products by price in ascending order products.sort((a, b) => a.price - b.price);
Reversing the Sort Order:
To sort an array in descending order, simply reverse the order of subtraction in the compare function:
example.jsCopiednumbers.sort((a, b) => b - a);
🎉 Conclusion
Mastering the sort()
method empowers you to efficiently organize array elements based on your specific requirements.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the sort()
method in your JavaScript projects.
👨💻 Join our Community:
Author
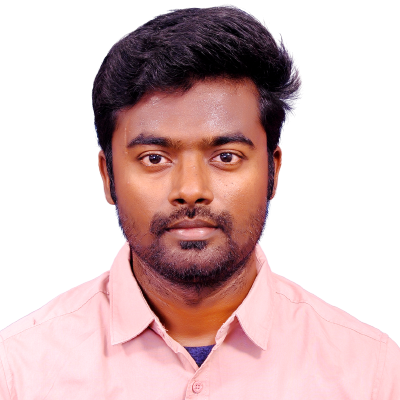
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array sort() Method), please comment here. I will help you immediately.