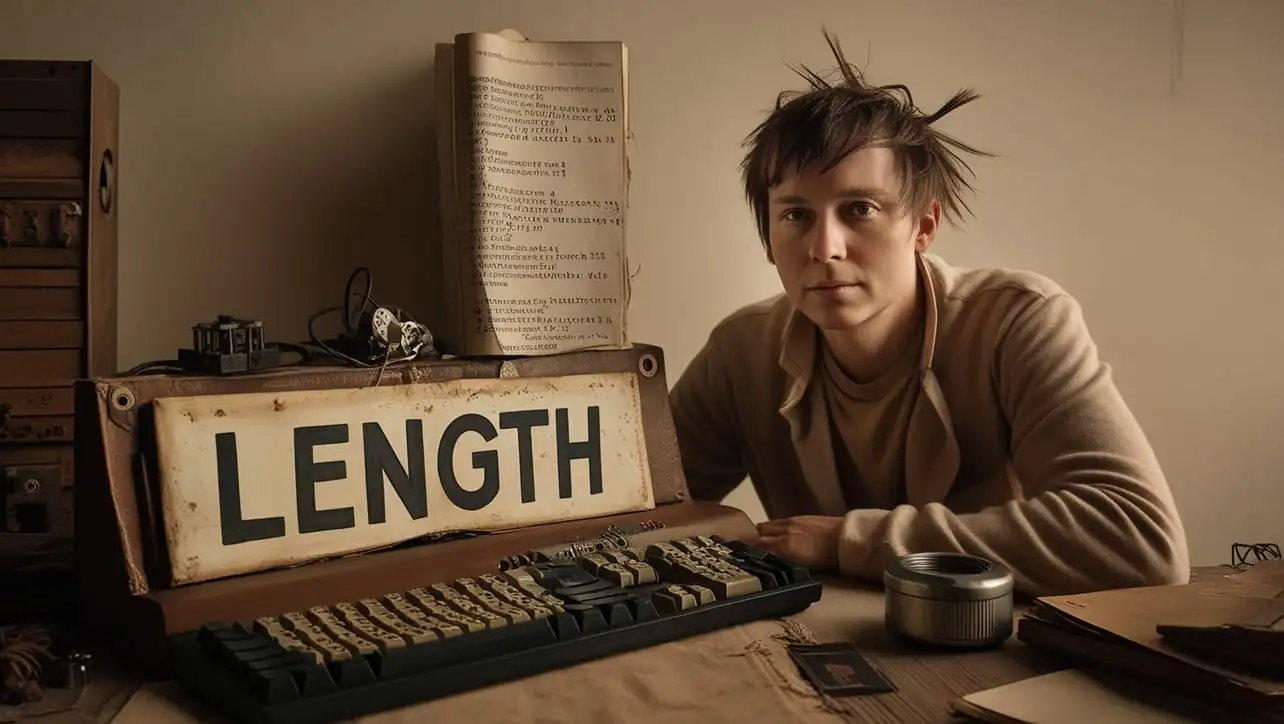
JS Array Methods
JavaScript Array some() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays come equipped with powerful methods, and the some()
method is no exception. This method provides an efficient way to check if at least one element in an array satisfies a given condition.
In this guide, we'll explore the some()
method, understand its syntax, examine usage examples, discuss best practices, and explore practical use cases.
🧠 Understanding some() Method
The some()
method tests whether at least one element in the array passes the provided function's test. It returns true if any element satisfies the condition; otherwise, it returns false. This method is particularly useful when you want to determine if there is at least one "truthy" element in the array.
💡 Syntax
The syntax for the some()
method is straightforward:
array.some(callback(element, index, array));
- array: The array that the
some()
method is being called upon. - callback: A function to test each element in the array. It takes three arguments: element (the current element being processed), index (the index of the current element), and array (the array on which
some()
is called).
📝 Example
Let's explore a basic example to understand the some()
method in action:
// Sample array
const numbers = [10, 20, 30, 40, 50];
// Using some() to check if at least one element is greater than 30
const isGreaterThan30 = numbers.some((element) => element > 30);
console.log(isGreaterThan30); // Output: true
In this example, the some()
method checks if there is at least one element in the numbers array that is greater than 30.
🏆 Best Practices
When working with the some()
method, consider the following best practices:
Callback Function Efficiency:
Ensure that the callback function passed to
some()
is efficient, as it will be executed for each element until a match is found.example.jsCopied// Good practice const hasPositiveNumbers = numbers.some((element) => element > 0); // Avoid unnecessary work in the callback const inefficientCheck = numbers.some((element) => { console.log('Executing unnecessary code'); return element > 0; });
Early Exit:
If you only need to know whether a condition is met and don't require a specific result, you can use the
some()
method with an early exit:example.jsCopiedif (numbers.some((element) => element > 30)) { console.log('At least one element is greater than 30'); }
📚 Use Cases
Validating User Input:
The
some()
method can be beneficial when validating user input in a form. For example, checking if at least one field is filled:example.jsCopiedconst formFields = ['', 'John Doe', '', 'example@email.com']; const isAtLeastOneFieldFilled = formFields.some((field) => field.trim() !== ''); if (isAtLeastOneFieldFilled) { console.log('At least one field is filled. Proceed with form submission.'); } else { console.log('All fields are empty. Please fill in at least one field.'); }
Checking for Specific Element:
Determining if an array contains a specific element is a common use case:
example.jsCopiedconst targetElement = 'apple'; const fruits = ['orange', 'banana', 'apple', 'grape']; const doesArrayContainElement = fruits.some((element) => element === targetElement); console.log(doesArrayContainElement); // Output: true
🎉 Conclusion
The some()
method is a powerful tool in JavaScript arrays, providing an elegant solution for checking if at least one element satisfies a condition.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the some()
method in your JavaScript projects.
👨💻 Join our Community:
Author
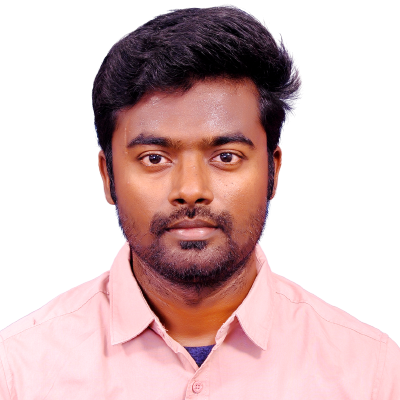
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array some() Method), please comment here. I will help you immediately.