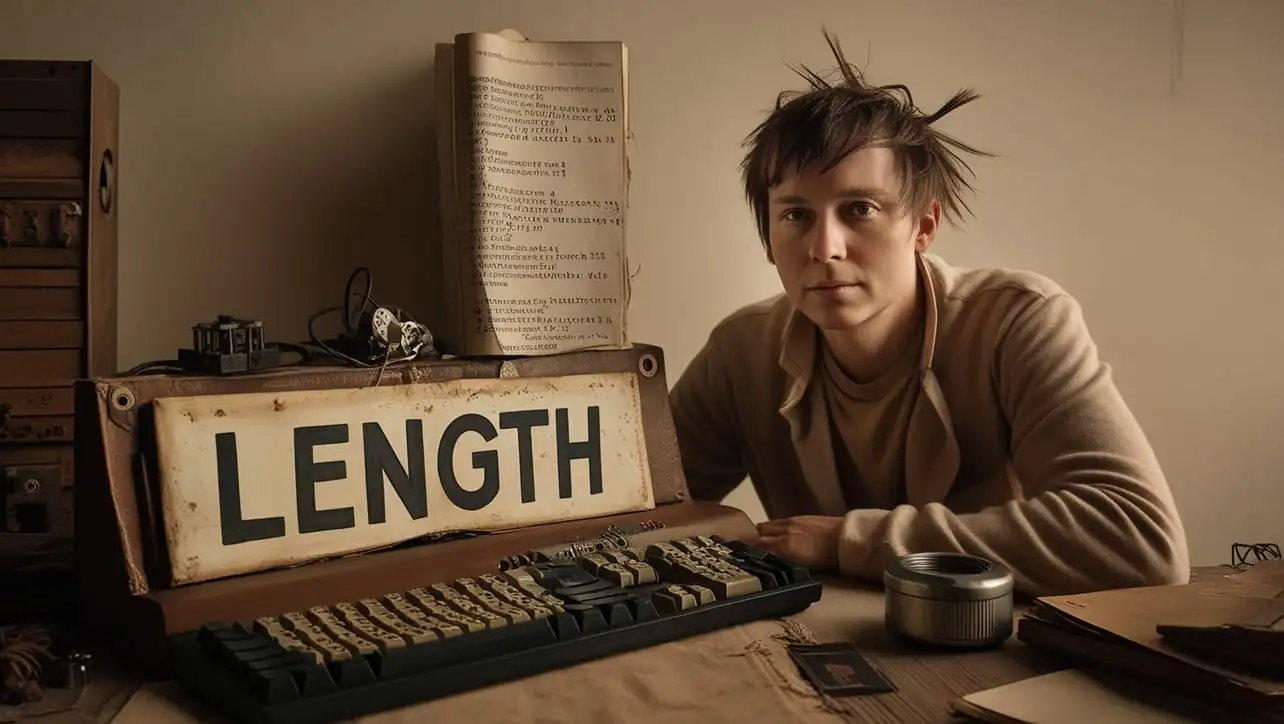
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array reduceRight() Method
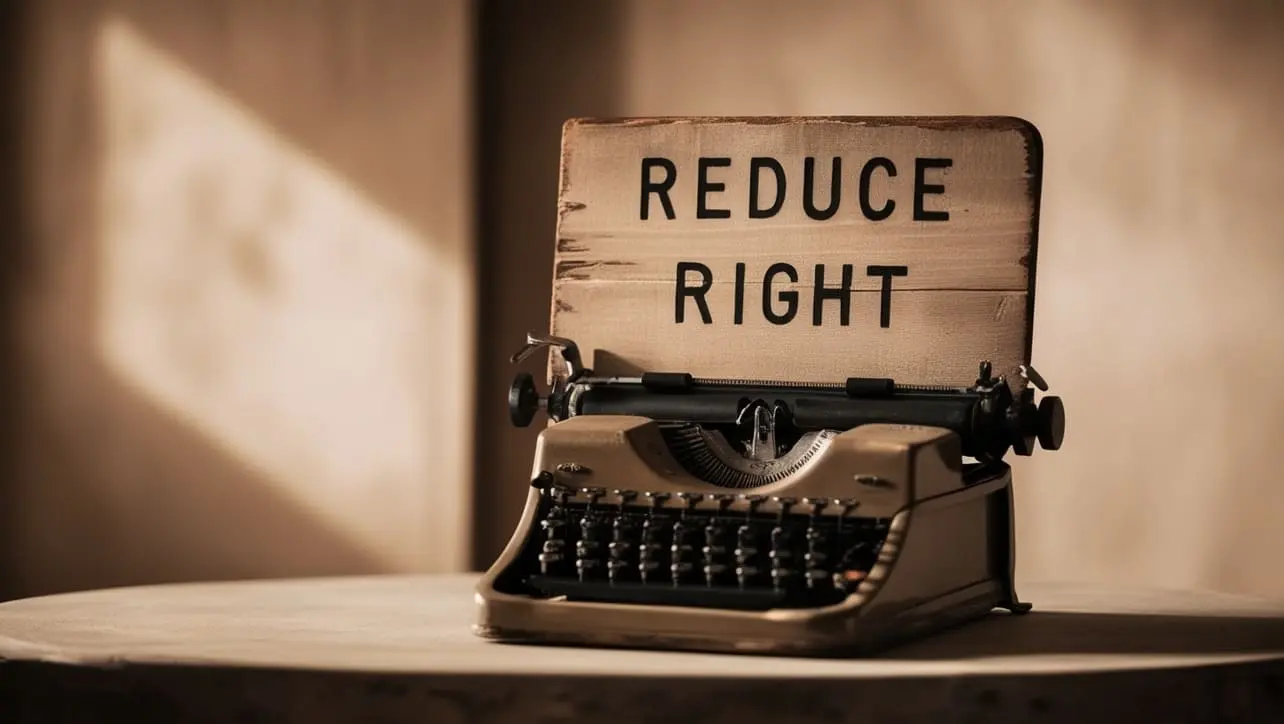
Photo Credit to CodeToFun
Introduction
JavaScript arrays come equipped with a multitude of powerful methods, and the reduceRight()
method is no exception. This method empowers you to perform a right-to-left reduction on an array, accumulating the elements into a single value.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical examples of the reduceRight()
method.
Understanding reduceRight() Method
The reduceRight()
method applies a provided function against an accumulator and each element of the array (from right to left) to reduce it to a single value.
Syntax
The syntax for the reduceRight()
method is straightforward:
array.reduceRight(callback[, initialValue]);
- array: The array to be reduced.
- callback: A function that is called for each element in the array, taking four arguments: accumulator, current value, current index, and the array itself.
- initialValue (optional): A value to use as the initial accumulator.
Example
Let's delve into an example to illustrate the usage of the reduceRight()
method:
// Sample array
const numbers = [1, 2, 3, 4, 5];
// Using reduceRight() to calculate the product of elements
const product = numbers.reduceRight((accumulator, currentValue) => {
return accumulator * currentValue;
}, 1);
console.log(product); // Output: 120
In this example, the reduceRight()
method is employed to calculate the product of all elements in the array.
Best Practices
When working with the reduceRight()
method, consider the following best practices:
Provide an Initial Value:
While the initialValue parameter is optional, providing it can help avoid unexpected behavior and ensure consistency in the reduction process.
example.jsCopiedconst sum = numbers.reduceRight((accumulator, currentValue) => { return accumulator + currentValue; }, 0);
Use Arrow Functions Sparingly:
Be cautious when using arrow functions for the callback, especially if the reduction logic becomes complex. Using a separate named function can improve readability.
example.jsCopiedconst sum = numbers.reduceRight(calculateSum, 0); function calculateSum(accumulator, currentValue) { return accumulator + currentValue; }
Error Handling:
Handle potential errors or edge cases within the callback function to ensure a robust reduction process.
example.jsCopiedconst safeProduct = numbers.reduceRight((accumulator, currentValue) => { if (currentValue === 0) { throw new Error('Cannot calculate product with zero.'); } return accumulator * currentValue; }, 1); console.log(safeProduct); // Output: Uncaught Error: Cannot calculate product with zero.
Use Cases
Flattening Nested Arrays:
The
reduceRight()
method is useful for flattening nested arrays:example.jsCopiedconst nestedArray = [[1, 2], [3, 4], [5, 6]]; const flattened = nestedArray.reduceRight((accumulator, currentValue) => { return accumulator.concat(currentValue); }, []); console.log(flattened); // Output: [5, 6, 3, 4, 1, 2]
Reversing an Array:
You can leverage
reduceRight()
to reverse the elements of an array:example.jsCopiedconst reversed = numbers.reduceRight((accumulator, currentValue) => { accumulator.push(currentValue); return accumulator; }, []); console.log(reversed); // Output: [5, 4, 3, 2, 1]
Conclusion
The reduceRight()
method is a powerful tool for array manipulation in JavaScript, enabling you to perform complex operations efficiently.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the reduceRight()
method in your JavaScript projects.
Join our Community:
Author
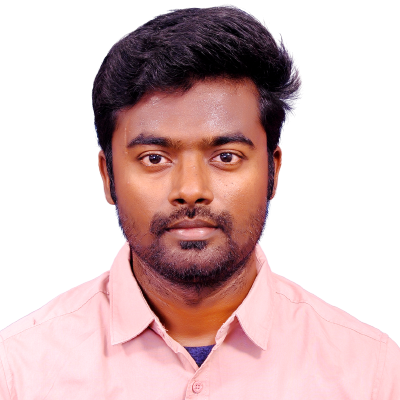
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array reduceRight() Method), please comment here. I will help you immediately.