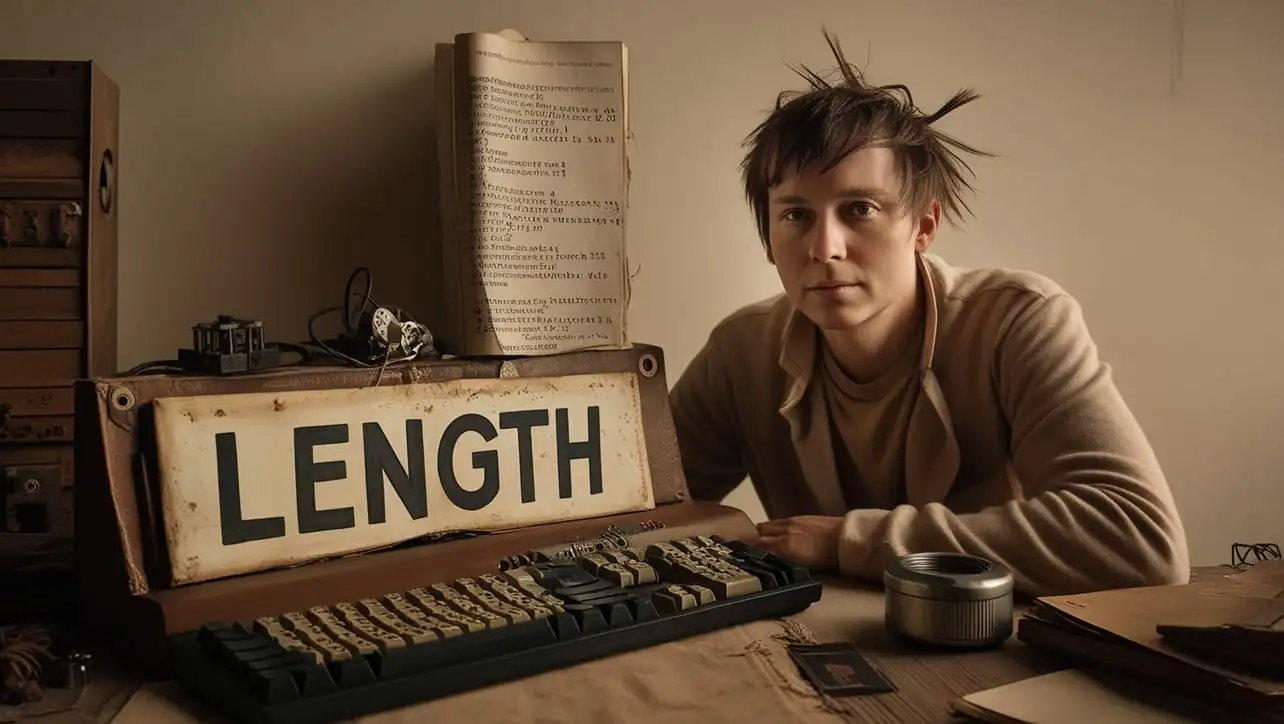
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array of() Method
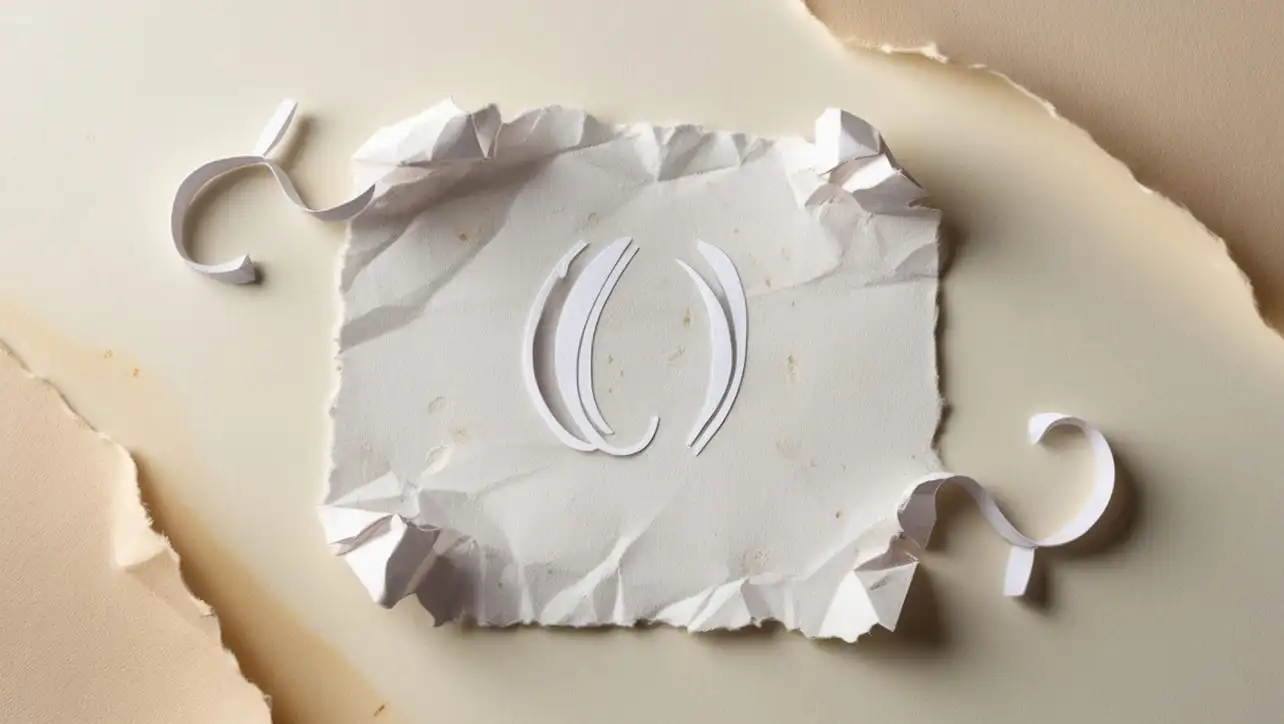
Photo Credit to CodeToFun
Introduction
JavaScript arrays offer a myriad of methods to manipulate and work with data. The Array.of()
method is one such utility that simplifies the creation of arrays, especially when dealing with a variable number of arguments.
In this guide, we'll explore the Array.of()
method, its syntax, usage examples, best practices, and practical applications.
Understanding of() Method
The Array.of()
method creates a new array instance with the provided elements. Unlike the Array constructor, Array.of()
doesn't exhibit the special case behavior when there is only one argument. This ensures consistent array creation, regardless of the number of arguments.
Syntax
The syntax for the Array.of()
method is straightforward:
Array.of(element1, element2, ..., elementN);
- element1, element2, ..., elementN: The elements to include in the newly created array.
Example
Let's explore a simple example to illustrate the use of the Array.of()
method:
// Using Array.of() to create an array
const numbers = Array.of(1, 2, 3, 4, 5);
console.log(numbers); // Output: [1, 2, 3, 4, 5]
In this example, we use Array.of()
to create an array of numbers.
Best Practices
When working with the Array.of()
method, consider the following best practices:
Useful for Single Values:
While
Array.of()
is not the only way to create arrays, it can be particularly useful when dealing with a single value as it ensures that the argument is treated as an individual element.example.jsCopiedconst singleValueArray = Array.of(42); console.log(singleValueArray); // Output: [42]
Consistent Array Creation:
If you want to ensure consistent array creation, especially when dealing with the Array constructor's special case behavior, using
Array.of()
can lead to more predictable results.example.jsCopied// Consistent array creation using Array.of() const consistentArray = Array.of(1, 2, 3); console.log(consistentArray); // Output: [1, 2, 3]
Use Cases
Creating Arrays with Dynamic Values:
Array.of()
is beneficial when creating arrays with dynamic values, especially when the number of elements is not known beforehand.example.jsCopiedconst dynamicArray = Array.of(...someDynamicValues); console.log(dynamicArray);
Avoiding Constructor Quirks:
The
Array.of()
method helps in avoiding potential quirks associated with the Array constructor when dealing with a single value.example.jsCopiedconst singleValueArray = Array.of(42); console.log(singleValueArray); // Output: [42]
Conclusion
The Array.of()
method simplifies the process of creating arrays in JavaScript, offering a consistent and predictable way to handle array initialization.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the Array.of()
method in your JavaScript projects.
Join our Community:
Author
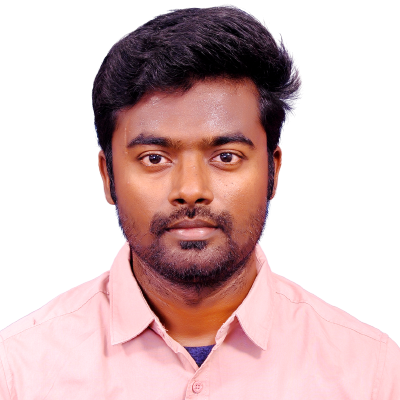
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array of() Method), please comment here. I will help you immediately.