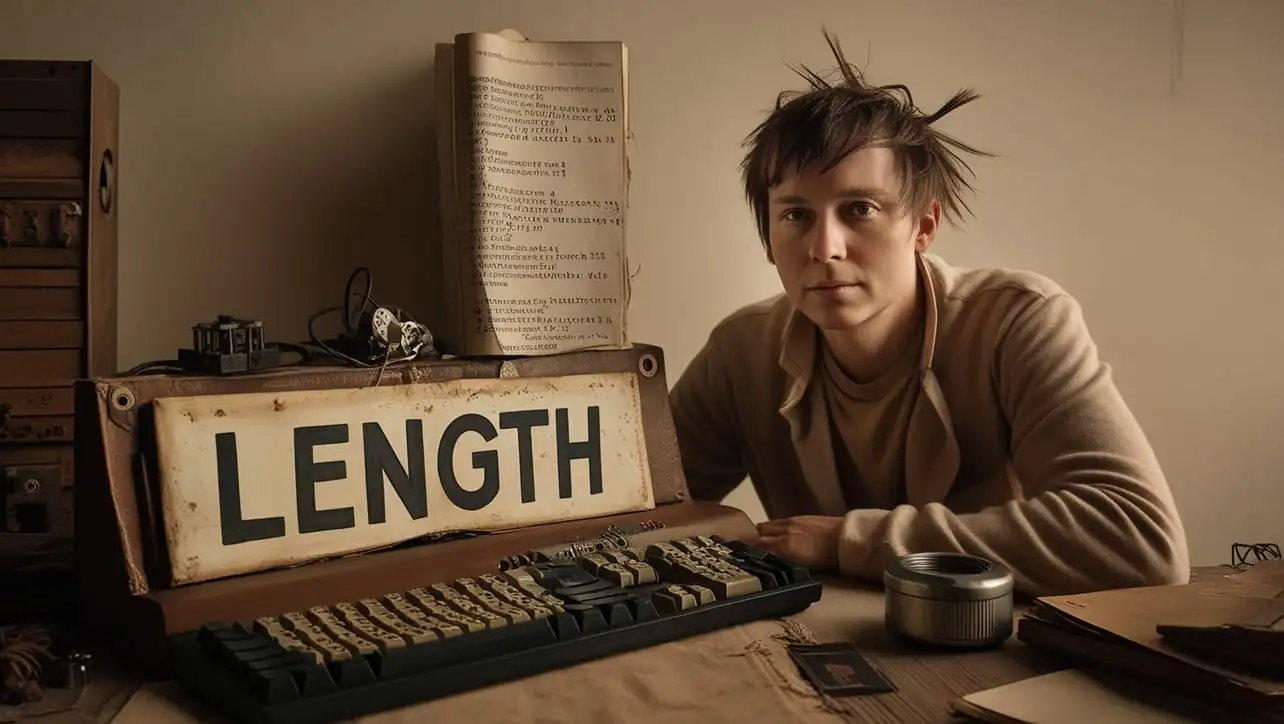
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array map() Method
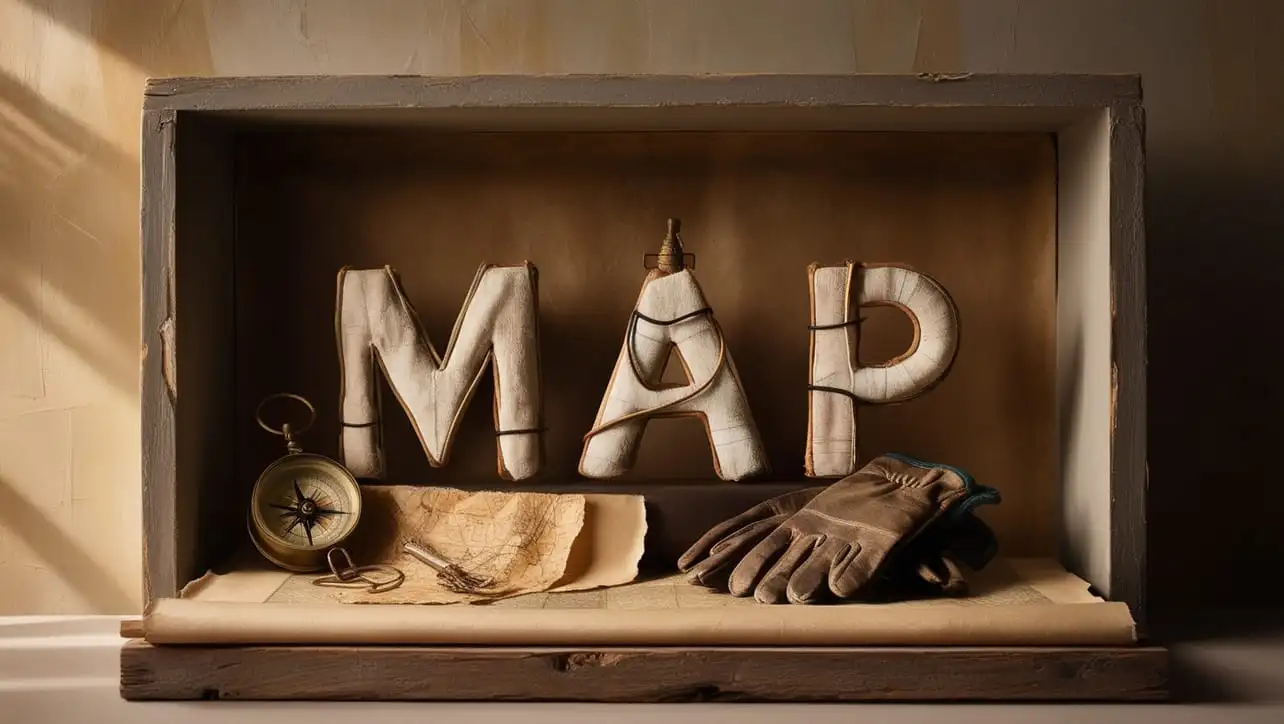
Photo Credit to CodeToFun
Introduction
Arrays in JavaScript provide a powerful foundation for managing and manipulating collections of data. The map()
method is a key player in this domain, offering an elegant way to transform each element of an array according to a provided function.
In this comprehensive guide, we'll delve into the map()
method, exploring its syntax, practical examples, best practices, and various use cases.
Understanding map() Method
The map()
method is designed to iterate through each element of an array, allowing you to apply a specified function to each item and create a new array of the results. This functional programming approach provides a concise and expressive means of transforming data within an array.
Syntax
The syntax for the map()
method is straightforward:
const newArray = array.map(callback(currentValue, index, array));
- array: The array to be mapped.
- callback: A function to execute on each element in the array, taking three arguments:
- currentValue: The current element being processed in the array.
- index: The index of the current element.
- array: The array on which
map()
was called.
Example
Let's dive into a simple example to illustrate the usage of the map()
method:
// Sample array
const numbers = [1, 2, 3, 4, 5];
// Using map() to square each number
const squaredNumbers = numbers.map((num) => num ** 2);
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
In this example, the map()
method is used to square each number in the numbers array, resulting in a new array of squared values.
Best Practices
When working with the map()
method, consider the following best practices:
Immutability:
Since
map()
returns a new array, it's a good practice to avoid modifying the original array directly. Instead, assign the result to a new variable.example.jsCopiedconst doubledNumbers = numbers.map((num) => num * 2);
Function Reusability:
Define reusable functions to use as the callback, promoting code clarity and maintainability.
example.jsCopiedconst square = (num) => num ** 2; const squaredNumbers = numbers.map(square);
Use Cases
Transforming Object Properties:
The
map()
method is excellent for transforming properties of objects within an array. Consider the following example where we extract names from an array of user objects:example.jsCopiedconst users = [ { id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' }, ]; const userNames = users.map((user) => user.name); console.log(userNames); // Output: ['Alice', 'Bob', 'Charlie']
Converting Data Types:
You can use
map()
to convert data types within an array, such as parsing strings to integers:example.jsCopiedconst stringNumbers = ['1', '2', '3']; const integerNumbers = stringNumbers.map(Number); console.log(integerNumbers); // Output: [1, 2, 3]
Conclusion
The map()
method is a versatile tool for transforming and manipulating arrays in JavaScript. Its expressive syntax and functional programming paradigm contribute to writing clean and concise code.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the map()
method in your JavaScript projects.
Join our Community:
Author
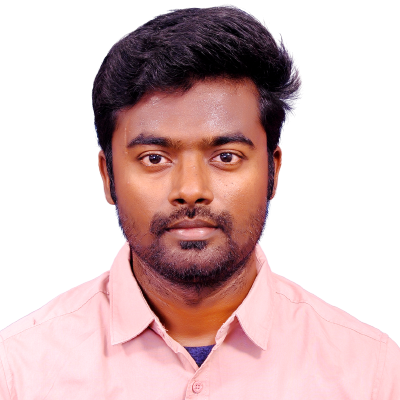
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array map() Method), please comment here. I will help you immediately.