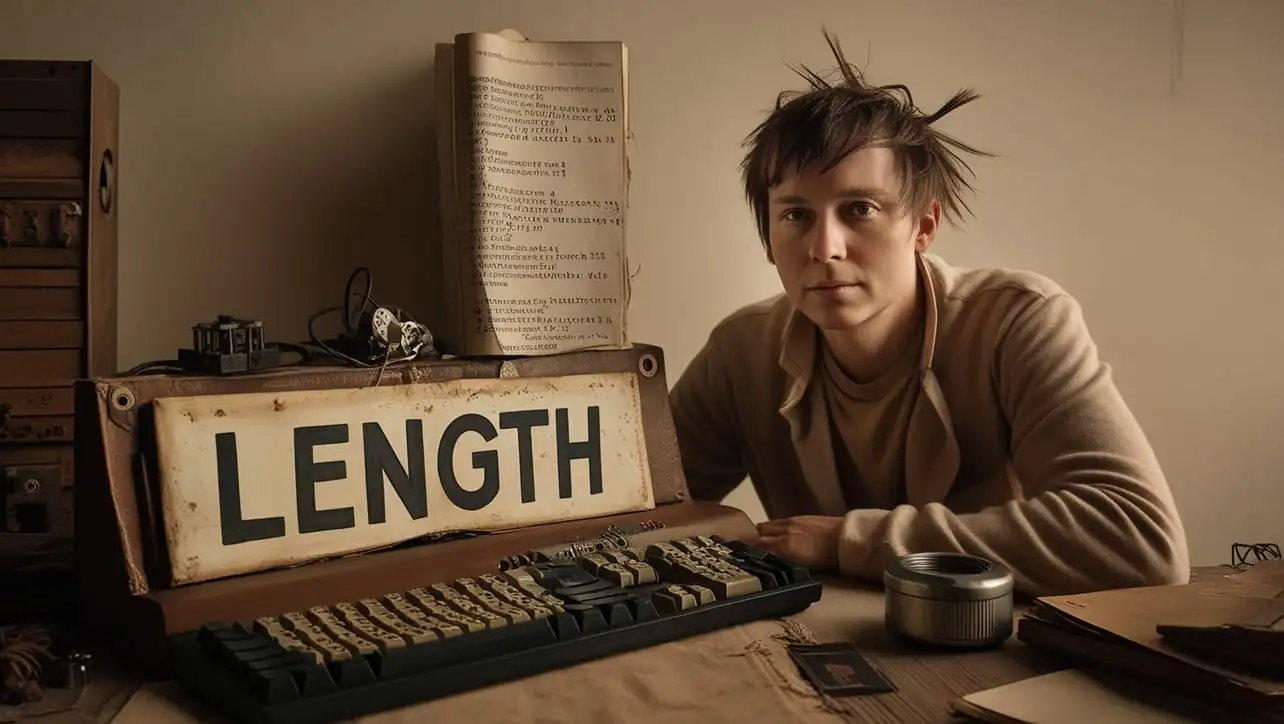
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array keys() Method
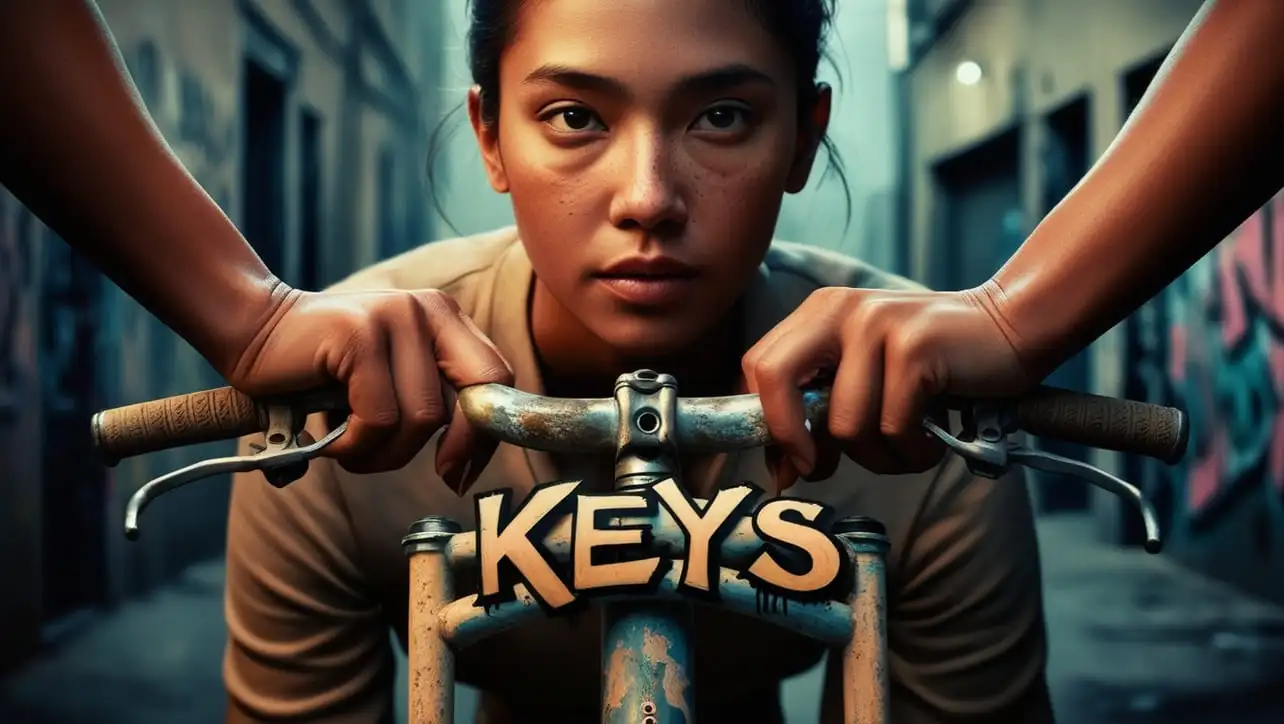
Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays provide a powerful set of methods for manipulating and iterating through their elements. The keys()
method is one such tool, offering a straightforward way to retrieve the keys (indices) of an array.
In this guide, we'll delve into the keys()
method, covering its syntax, usage, best practices, and practical use cases.
🧠 Understanding keys() Method
The keys()
method returns a new Array Iterator object that contains the keys (indices) for each element in the array. This Iterator allows you to iterate through the indices of the array, providing a useful way to access array elements by their positions.
💡 Syntax
The syntax for the keys()
method is straightforward:
array.keys();
- array: The array for which you want to retrieve the keys.
📝 Example
Let's dive into a simple example to illustrate the usage of the keys()
method:
// Sample array
const fruits = ['apple', 'orange', 'banana'];
// Using keys() to iterate through indices
const iterator = fruits.keys();
for (const key of iterator) {
console.log(key);
}
In this example, the keys()
method is used to obtain an iterator, and a for...of loop is employed to iterate through the indices of the fruits array.
🏆 Best Practices
When working with the keys()
method, consider the following best practices:
Array.from() for Iterables:
If you need a standard array containing the keys, you can convert the Iterator to an array using Array.from().
example.jsCopiedconst keysArray = Array.from(fruits.keys()); console.log(keysArray);
Compatibility Checking:
Verify the compatibility of the
keys()
method in your target environments, especially if supporting older browsers.example.jsCopied// Check if the keys() method is supported if (Array.prototype.keys) { // Use the method safely const iterator = fruits.keys(); for (const key of iterator) { console.log(key); } } else { console.error('keys() method not supported in this environment.'); }
📚 Use Cases
Iterating Through Indices:
The primary use case for the
keys()
method is to iterate through the indices of an array:example.jsCopiedfor (const key of fruits.keys()) { console.log(`Index: ${key}, Value: ${fruits[key]}`); }
Finding the First Occurrence:
The
keys()
method can be useful when searching for the index of the first occurrence of a specific value:example.jsCopiedconst targetFruit = 'orange'; for (const key of fruits.keys()) { if (fruits[key] === targetFruit) { console.log(`Found ${targetFruit} at index ${key}`); break; } }
🎉 Conclusion
The keys()
method enhances the capabilities of JavaScript arrays, providing a simple way to iterate through the indices of an array.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the keys()
method in your JavaScript projects.
👨💻 Join our Community:
Author
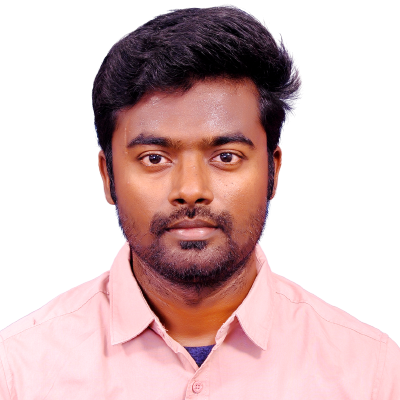
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array keys() Method), please comment here. I will help you immediately.