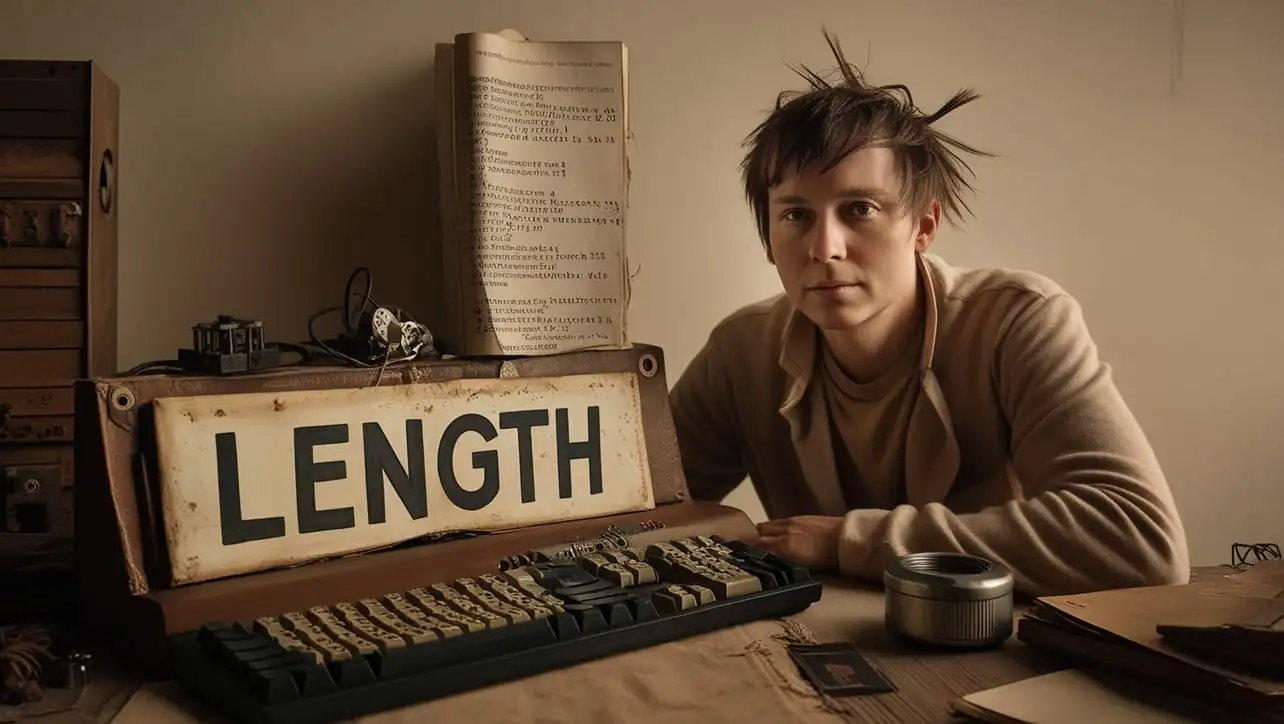
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array isArray() Method
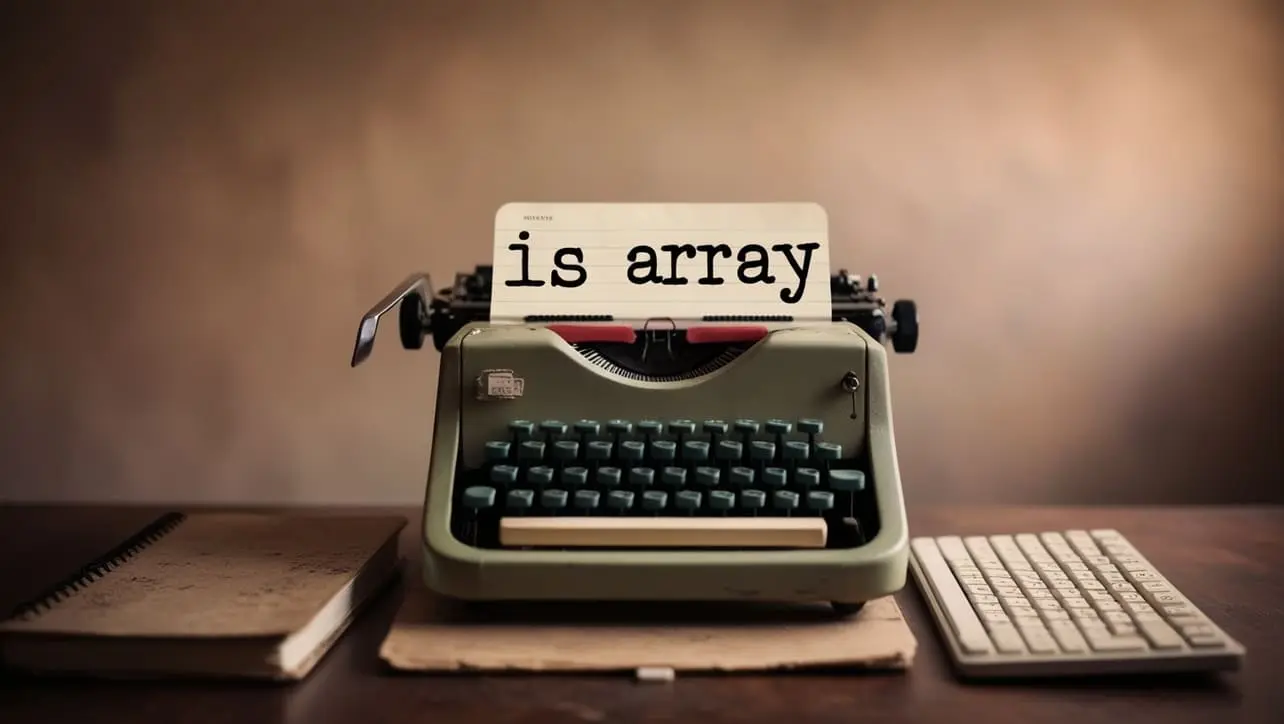
Photo Credit to CodeToFun
🙋 Introduction
Arrays are fundamental in JavaScript, and the isArray()
method is a powerful tool to determine whether an object is an array or not.
In this guide, we'll explore the isArray()
method, understand its syntax, delve into practical examples, and discuss best practices to effectively use it in your JavaScript projects.
🧠 Understanding isArray() Method
The isArray()
method is a built-in function in JavaScript that allows you to check if a given value is an array. It returns true if the value is an array and false otherwise.
💡 Syntax
The syntax for the isArray()
method is straightforward:
Array.isArray(value);
- value: The value to be checked for being an array.
📝 Example
Let's start with a basic example to illustrate the usage of the isArray()
method:
// Sample arrays
const numbers = [1, 2, 3];
const text = 'Hello, world!';
// Using isArray() to check if values are arrays
const isNumbersArray = Array.isArray(numbers);
const isTextArray = Array.isArray(text);
console.log(isNumbersArray); // Output: true
console.log(isTextArray); // Output: false
In this example, isArray()
is used to check whether numbers and text are arrays.
🏆 Best Practices
When working with the isArray()
method, consider the following best practices:
Array-Like Objects:
Be aware that
isArray()
returns false for array-like objects. If you need to check if an object is iterable like an array, consider alternative methods.example.jsCopiedconst arrayLike = { 0: 'a', 1: 'b', length: 2 }; // isArray() returns false for array-like objects console.log(Array.isArray(arrayLike)); // Output: false // Check for array-like using alternative methods const isIterable = typeof arrayLike.length === 'number' && arrayLike.length >= 0; console.log(isIterable); // Output: true
Nested Arrays:
isArray()
checks only one level deep. If you have nested arrays, consider recursive checks if necessary.example.jsCopiedconst nestedArray = [1, [2, 3], 4]; // isArray() returns true for the outer array console.log(Array.isArray(nestedArray)); // Output: true // Recursive check for nested arrays const isNestedArray = nestedArray.every(item => Array.isArray(item)); console.log(isNestedArray); // Output: true
📚 Use Cases
Dynamic Functionality Based on Array Presence:
The
isArray()
method can be useful in scenarios where you want to apply specific functionality based on whether a variable is an array:example.jsCopiedfunction processArrayOrValue(input) { if (Array.isArray(input)) { input.forEach(item => console.log(`Array item: ${item}`)); } else { console.log(`Single value: ${input}`); } } processArrayOrValue('Hello'); // Output: Single value: Hello processArrayOrValue(['a', 'b', 'c']); // Output: Array item: a, Array item: b, Array item: c
Validating Function Arguments:
You can use
isArray()
for argument validation, ensuring that a parameter passed to a function is an array:example.jsCopiedfunction sumArray(numbers) { if (!Array.isArray(numbers)) { throw new Error('Input must be an array'); } return numbers.reduce((sum, num) => sum + num, 0); } console.log(sumArray([1, 2, 3])); // Output: 6
🎉 Conclusion
The isArray()
method is a crucial tool for handling arrays in JavaScript. By understanding its syntax, following best practices, and exploring diverse use cases, you can confidently integrate isArray()
into your codebase.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the isArray()
method in your JavaScript projects.
👨💻 Join our Community:
Author
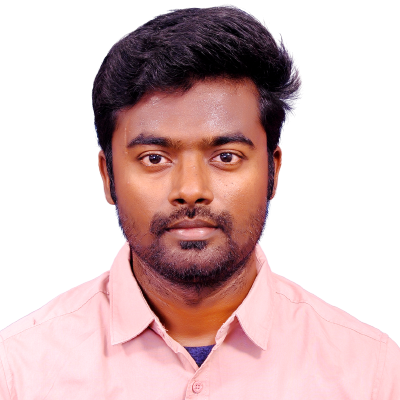
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array isArray() Method), please comment here. I will help you immediately.