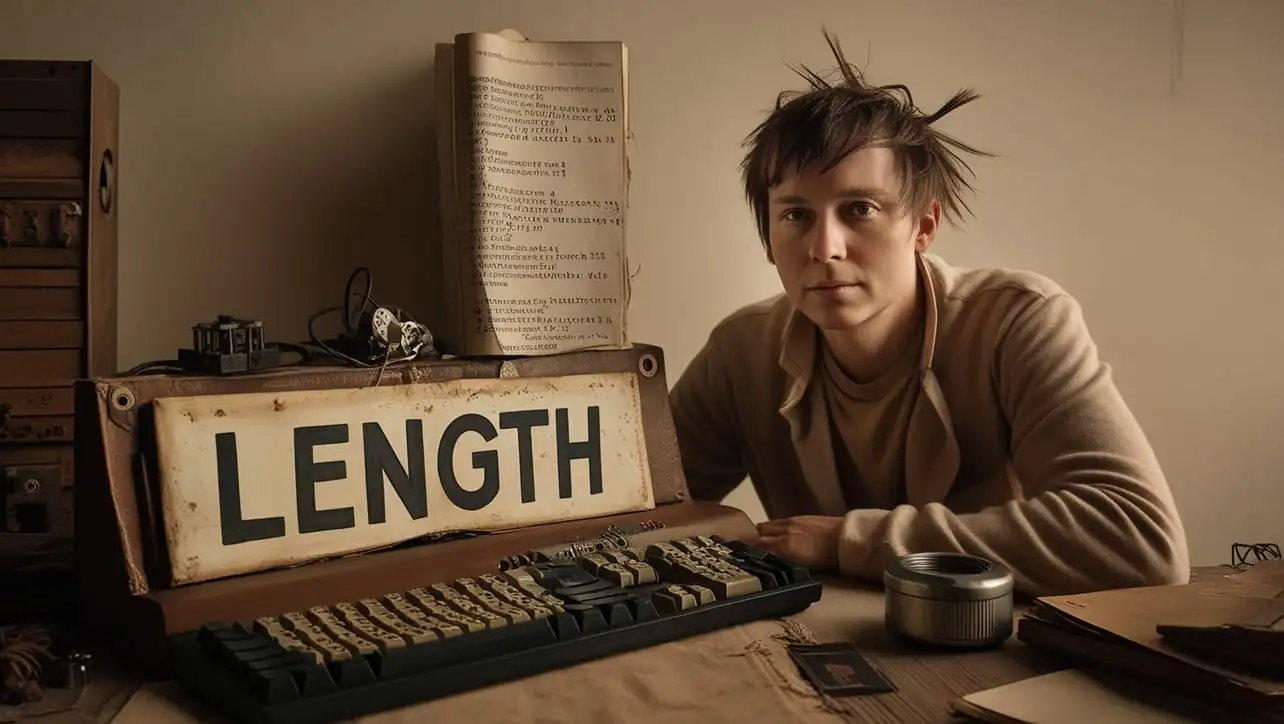
JS Array Methods
JavaScript Array indexOf() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays are fundamental components for storing and managing collections of data. The indexOf()
method is a powerful tool that allows you to search for the first occurrence of a specified element within an array.
In this guide, we'll delve into the indexOf()
method, explore its syntax, and provide examples to help you use it effectively in your JavaScript projects.
🧠 Understanding indexOf() Method
The indexOf()
method is designed to locate the index of the first occurrence of a specified value within an array. If the element is not found, the method returns -1, indicating that the element is not present in the array.
💡 Syntax
The syntax for the indexOf()
method is straightforward:
array.indexOf(searchElement, fromIndex);
- array: The array in which to search for the specified element.
- searchElement: The element to locate within the array.
- fromIndex (optional): The starting index for the search. If omitted, the search starts from the beginning of the array.
📝 Example
Let's explore some practical examples to understand how to use the indexOf()
method:
// Sample array
const colors = ['red', 'blue', 'green', 'yellow', 'blue'];
// Using indexOf() to find the index of 'green'
const indexOfGreen = colors.indexOf('green');
console.log(indexOfGreen); // Output: 2
// Using indexOf() to find the index of 'blue' starting from index 2
const indexOfBlue = colors.indexOf('blue', 2);
console.log(indexOfBlue); // Output: 4
// Using indexOf() to check if 'purple' is in the array
const indexOfPurple = colors.indexOf('purple');
console.log(indexOfPurple); // Output: -1
In these examples, we use the indexOf()
method to find the index of specific elements within the colors array.
🏆 Best Practices
When working with the indexOf()
method, consider the following best practices:
Check for Existence:
Always check the result of
indexOf()
to determine whether the element exists in the array.example.jsCopiedconst elementToFind = 'orange'; const index = colors.indexOf(elementToFind); if (index !== -1) { console.log(`${elementToFind} found at index ${index}`); } else { console.log(`${elementToFind} not found in the array`); }
Strict Equality:
The
indexOf()
method uses strict equality (===) for comparisons, so ensure that the type and value match.example.jsCopiedconst numbers = [1, 2, 3, 4, 5]; const indexOfString = numbers.indexOf('3'); if (indexOfString !== -1) { console.log(`'3' found at index ${indexOfString}`); } else { console.log(`'3' not found in the array`); }
📚 Use Cases
Removing Duplicates:
You can leverage the
indexOf()
method to filter out duplicate elements from an array:example.jsCopiedconst uniqueColors = colors.filter((value, index, array) => { return array.indexOf(value) === index; }); console.log(uniqueColors);
In this example, the filter() method is used in conjunction with
indexOf()
to create a new array containing only unique elements.Checking Array Membership:
Use
indexOf()
to quickly check if an element is present in an array:example.jsCopiedconst isColorPresent = colors.indexOf('red') !== -1; console.log(isColorPresent); // Output: true
🎉 Conclusion
The indexOf()
method is a versatile tool for searching and locating elements within JavaScript arrays.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the indexOf()
method in your JavaScript projects.
👨💻 Join our Community:
Author
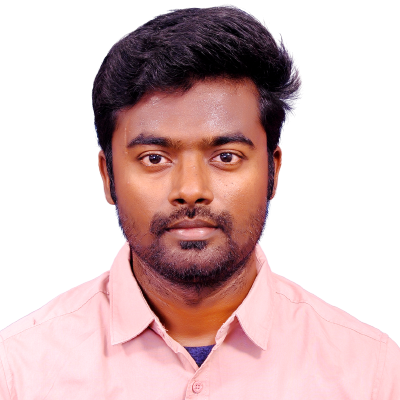
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array indexOf() Method), please comment here. I will help you immediately.