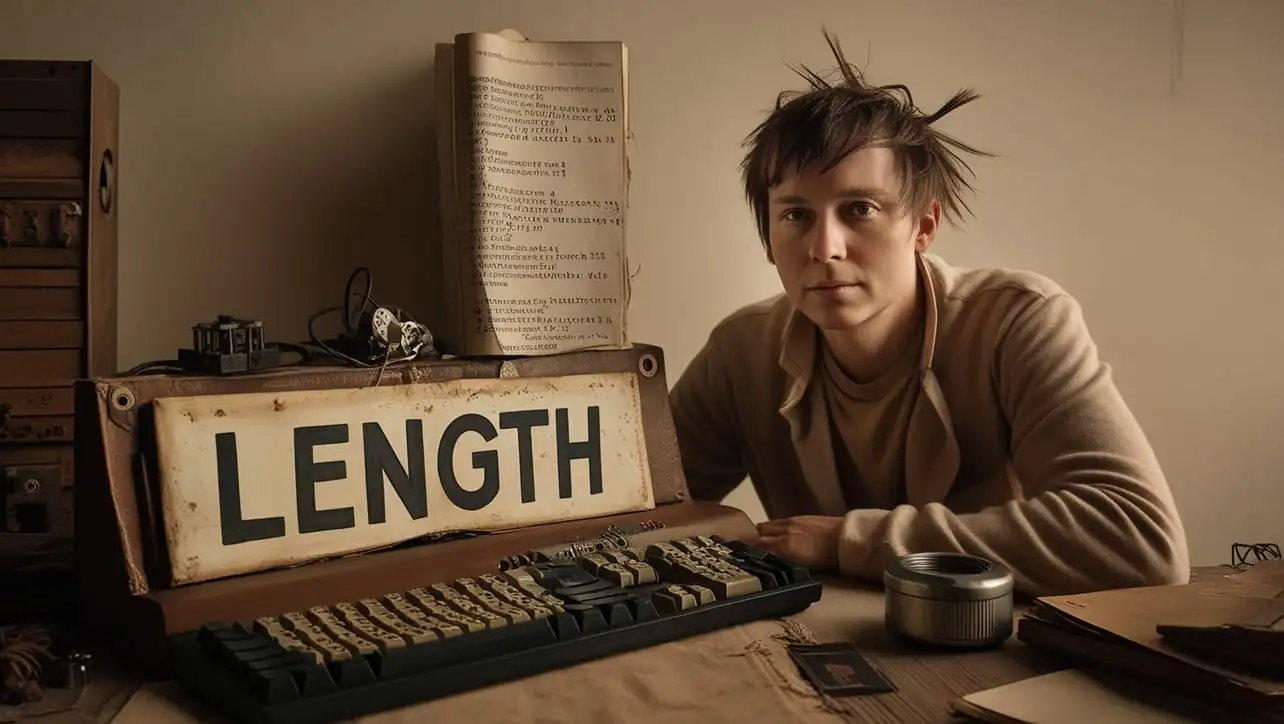
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array from() Method

Photo Credit to CodeToFun
🙋 Introduction
The JavaScript from()
method is a versatile tool that allows you to create arrays from array-like objects, iterables, or even map functions to transform and map elements.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical use cases of the from()
method to harness its full potential in your JavaScript projects.
🧠 Understanding from() Method
The from()
method is used to create a new, shallow-copied array instance from an array-like or iterable object. It also accepts an optional mapping function, providing flexibility in transforming elements during array creation.
💡 Syntax
The syntax for the from()
method is straightforward:
Array.from(arrayLike[, mapFunction[, thisArg]]);
- arrayLike: The array-like or iterable object to convert to an array.
- mapFunction (optional): A function to call on each element of the array.
- thisArg (optional): An object to use as this when executing the mapFunction.
📝 Example
Let's delve into a basic example to illustrate the usage of the from()
method:
// Convert a string to an array
const greeting = 'Hello, World!';
const charArray = Array.from(greeting);
console.log(charArray); // Output: ['H', 'e', 'l', 'l', 'o', ',', ' ', 'W', 'o', 'r', 'l', 'd', '!']
Here, the from()
method is used to transform the characters of the string greeting into an array.
🏆 Best Practices
When working with the from()
method, consider the following best practices:
Handling Non-Array Iterables:
Ensure compatibility with non-array iterables by using the map function to convert elements appropriately.
example.jsCopiedconst set = new Set(['apple', 'orange', 'banana']); const arrayFromSet = Array.from(set, fruit => fruit.toUpperCase()); console.log(arrayFromSet); // Output: ['APPLE', 'ORANGE', 'BANANA']
Avoiding Pitfalls with Objects:
Be cautious when using
from()
with objects, as it might not behave as expected. If you need to convert object values into an array, consider using Object.values(obj).example.jsCopiedconst obj = { a: 1, b: 2, c: 3 }; const arrayFromObj = Array.from(obj); // Not recommended for objects console.log(arrayFromObj); // Output: [] const valuesArray = Object.values(obj); console.log(valuesArray); // Output: [1, 2, 3]
📚 Use Cases
Mapping Elements during Array Creation:
The
from()
method allows you to apply a mapping function during array creation, providing a concise way to transform elements:example.jsCopiedconst numbers = [1, 2, 3, 4, 5]; const squaredArray = Array.from(numbers, num => num * num); console.log(squaredArray); // Output: [1, 4, 9, 16, 25]
Creating Arrays from DOM Collections:
Convert a DOM NodeList into an array using
from()
:example.jsCopiedconst nodeList = document.querySelectorAll('.list-item'); const arrayFromNodeList = Array.from(nodeList); console.log(arrayFromNodeList); // Array containing elements from the NodeList
🎉 Conclusion
The from()
method proves to be a valuable asset in creating arrays from a variety of sources in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the from()
method in your JavaScript projects.
👨💻 Join our Community:
Author
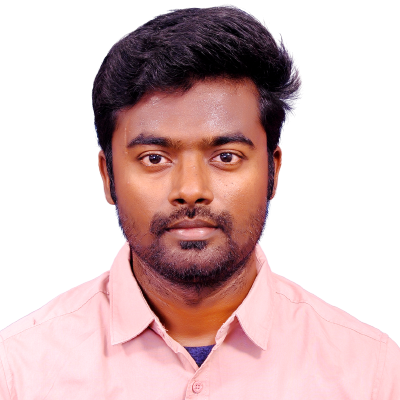
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array from() Method), please comment here. I will help you immediately.