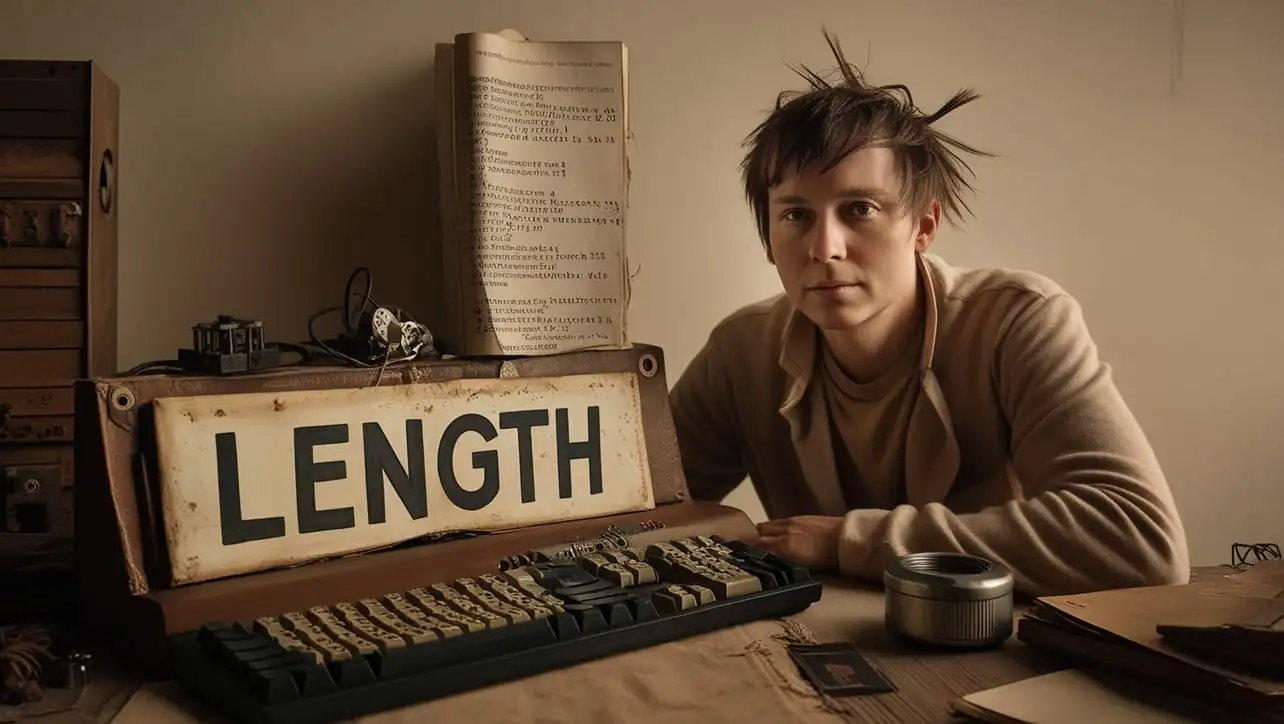
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array forEach() Method
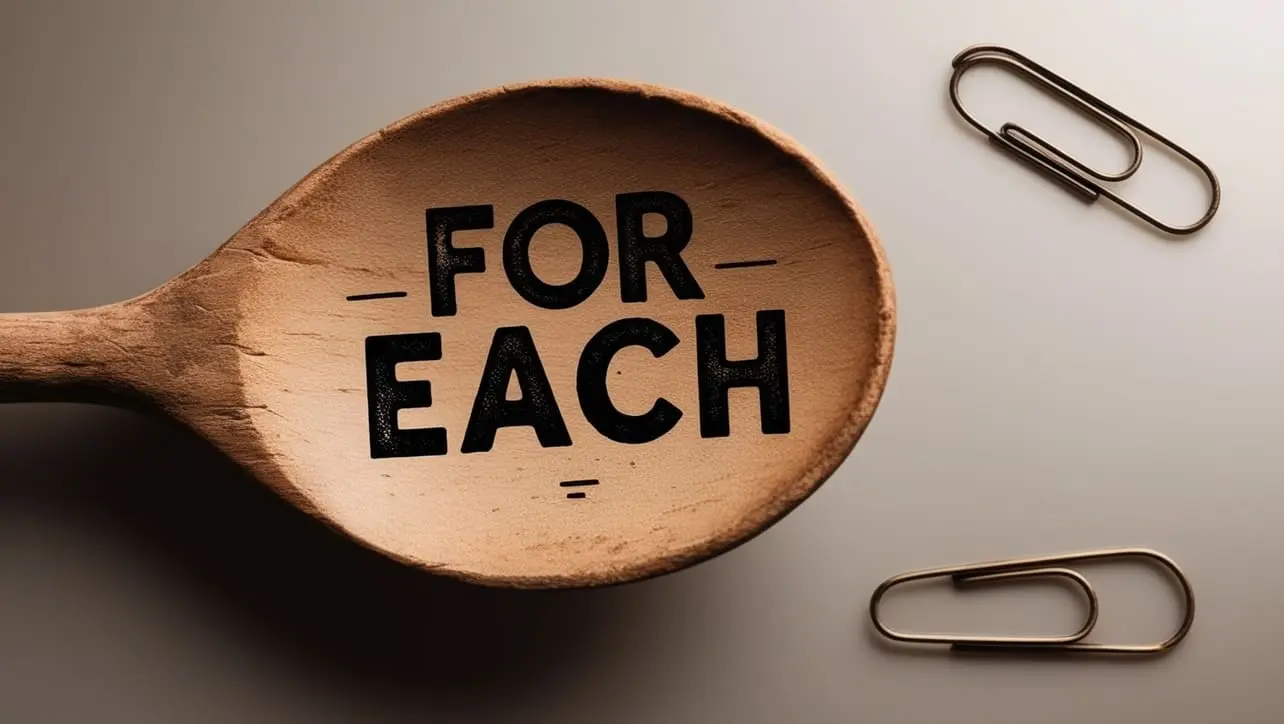
Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays offer powerful features for managing data, and the forEach()
method is a cornerstone for seamless iteration. This method simplifies the process of applying a function to each element in an array, enhancing code readability and maintainability.
In this guide, we'll explore the forEach()
method, covering its syntax, usage, best practices, and practical applications.
🧠 Understanding forEach() Method
The forEach()
method executes a provided function once for each array element, in ascending order. It is a concise and expressive way to iterate through the elements without the need for traditional loop structures.
💡 Syntax
The syntax for the forEach()
method is straightforward:
array.forEach(function(element, index, array) {
// Your code logic here
});
- array: The array to iterate through.
- element: The current element being processed.
- index: The index of the current element.
- array: The array on which
forEach()
was called.
📝 Example
Let's dive into a simple example to illustrate the usage of the forEach()
method:
// Sample array
const numbers = [1, 2, 3, 4, 5];
// Using forEach() to square each element
numbers.forEach(function (number, index, array) {
array[index] = number * number;
});
console.log(numbers); // Output: [1, 4, 9, 16, 25]
In this example, the forEach()
method is employed to square each number in the array.
🏆 Best Practices
When working with the forEach()
method, consider the following best practices:
Callback Function:
Provide a clear and concise callback function, enhancing code readability.
example.jsCopiednumbers.forEach(number => console.log(number));
Breaking out of Loop:
Unlike traditional loops, you cannot break out of a
forEach()
loop. If you need this functionality, consider using a for loop instead.example.jsCopied// Using traditional for loop to break out for (let i = 0; i < numbers.length; i++) { if (numbers[i] === 4) { console.log('Breaking out of loop at index ' + i); break; } }
Avoiding Side Effects:
Be cautious when modifying the array within the
forEach()
loop, as it can lead to unexpected side effects.example.jsCopiedconst doubledNumbers = []; // Avoiding side effects by not modifying the original array numbers.forEach(number => { doubledNumbers.push(number * 2); }); console.log(doubledNumbers); // Output: [2, 8, 18, 32, 50]
📚 Use Cases
Summing Array Elements:
The
forEach()
method is excellent for calculating the sum of array elements:example.jsCopiedlet sum = 0; numbers.forEach(number => { sum += number; }); console.log(`Sum of numbers: ${sum}`); // Output: 55
Filtering Elements:
Filtering elements based on a condition is simplified with the
forEach()
method:example.jsCopiedconst evenNumbers = []; numbers.forEach(number => { if (number % 2 === 0) { evenNumbers.push(number); } }); console.log(evenNumbers); // Output: [4]
🎉 Conclusion
The forEach()
method is a fundamental tool for array manipulation in JavaScript, streamlining the process of iterating through elements.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the forEach()
method in your JavaScript projects.
👨💻 Join our Community:
Author
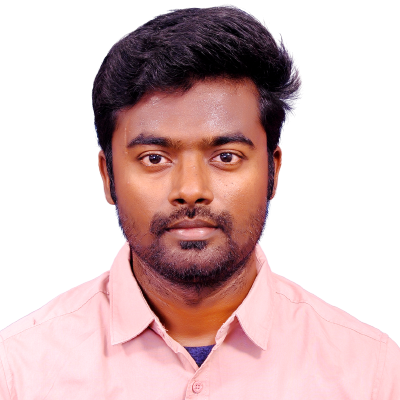
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array forEach() Method), please comment here. I will help you immediately.