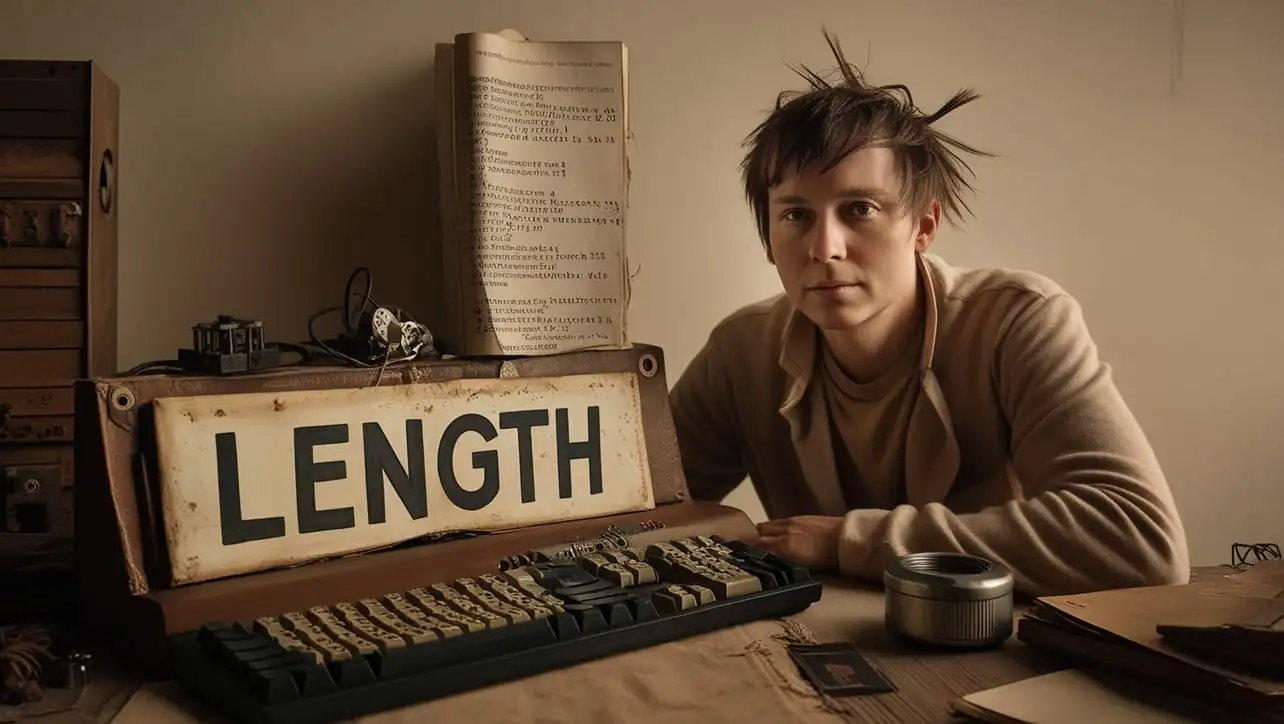
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array flatMap() Method
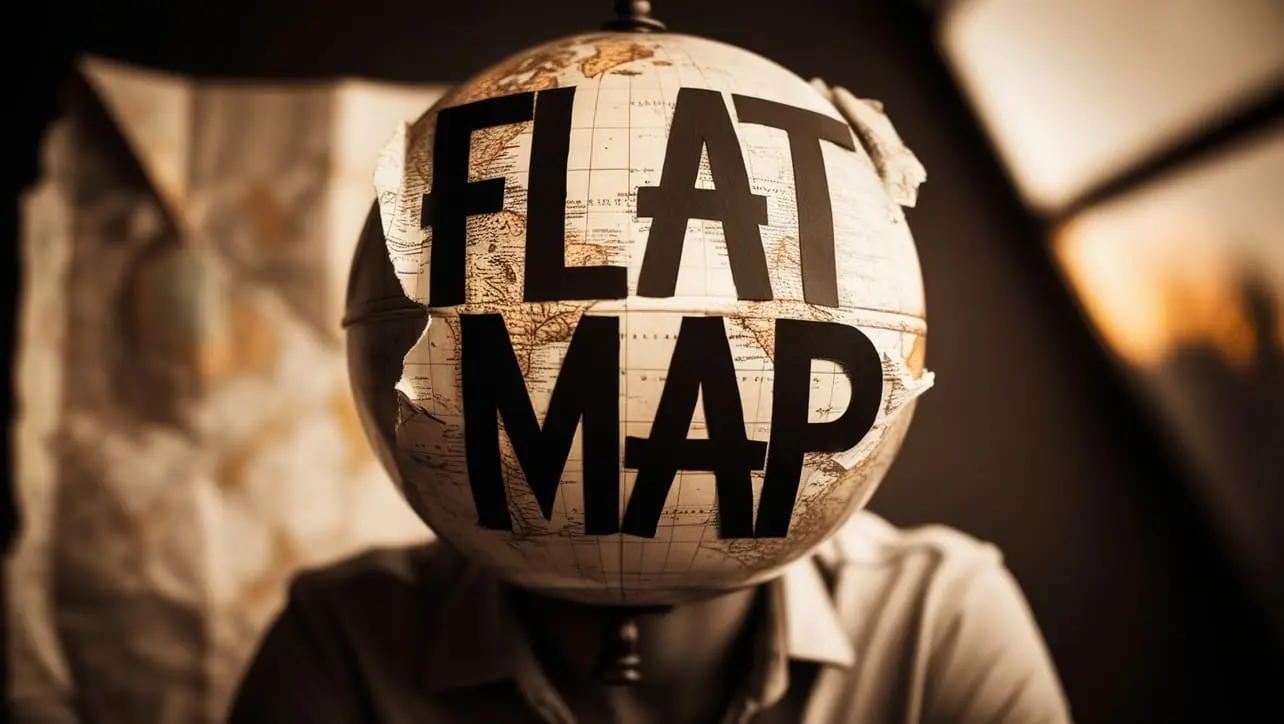
Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays are powerful tools for managing and manipulating data, and the flatMap()
method brings an extra layer of versatility to array operations. This method, introduced in ECMAScript 2019, is designed to both flatten and map arrays in a concise manner.
In this comprehensive guide, we'll delve into the syntax, usage, best practices, and practical applications of the flatMap()
method.
🧠 Understanding flatMap() Method
The flatMap()
method is a combination of the map() and flat() methods. It allows you to both map each element of an array to a new element and flatten the result into a single array.
💡 Syntax
The syntax for the flatMap()
method is straightforward:
array.flatMap(callback(currentValue, index, array), thisArg);
- array: The array that you want to map and flatten.
- callback: A function that is called for each element of the array. It should return either a new array or a single value.
- thisArg (Optional): The value to use as this when executing the callback.
📝 Example
Let's illustrate the usage of the flatMap()
method with a simple example:
// Sample array
const numbers = [1, 2, 3, 4];
// Using flatMap() to double each number and flatten the result
const doubledAndFlattened = numbers.flatMap((num) => [num * 2]);
console.log(doubledAndFlattened); // Output: [2, 4, 6, 8]
In this example, the flatMap()
method is used to double each element of the numbers array and flatten the resulting nested arrays into a single array.
🏆 Best Practices
When working with the flatMap()
method, consider the following best practices:
Error Handling:
Ensure that the callback function provided to
flatMap()
returns an array for each element. This helps prevent unexpected behavior and ensures that the result is properly flattened.example.jsCopiedconst nestedArray = [[1, 2], [3, 4], [5, 6]]; const flattened = nestedArray.flatMap(arr => { if (Array.isArray(arr)) { return arr; } else { // Handle non-array elements return [arr]; } }); console.log(flattened);
Compatibility Checking:
Verify the compatibility of the
flatMap()
method in your target environments, especially if you need to support older browsers.example.jsCopied// Check if the flatMap() method is supported if (Array.prototype.flatMap) { // Use the method safely const result = numbers.flatMap(num => [num, num * 2]); console.log(result); } else { console.error('flatMap() method not supported in this environment.'); }
📚 Use Cases
Flattening and Mapping Arrays:
The primary use case for the
flatMap()
method is to both map and flatten arrays simultaneously:example.jsCopiedconst words = ['Hello', 'World']; const mappedAndFlattened = words.flatMap(word => word.split('')); console.log(mappedAndFlattened); // Output: ['H', 'e', 'l', 'l', 'o', 'W', 'o', 'r', 'l', 'd']
Handling Optional Values:
The
flatMap()
method is also handy when dealing with arrays containing optional values:example.jsCopiedconst numbers = [1, 2, , 3, , 4]; const filteredAndMapped = numbers.flatMap(num => (num % 2 === 0 ? [num] : [])); console.log(filteredAndMapped); // Output: [2, 4]
🎉 Conclusion
The flatMap()
method is a versatile addition to the array manipulation toolbox in JavaScript, offering a streamlined approach to both mapping and flattening arrays.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the flatMap()
method in your JavaScript projects.
👨💻 Join our Community:
Author
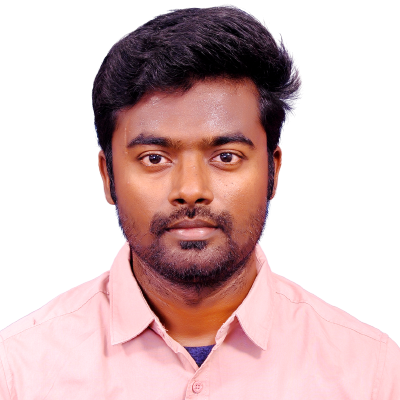
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array flatMap() Method), please comment here. I will help you immediately.