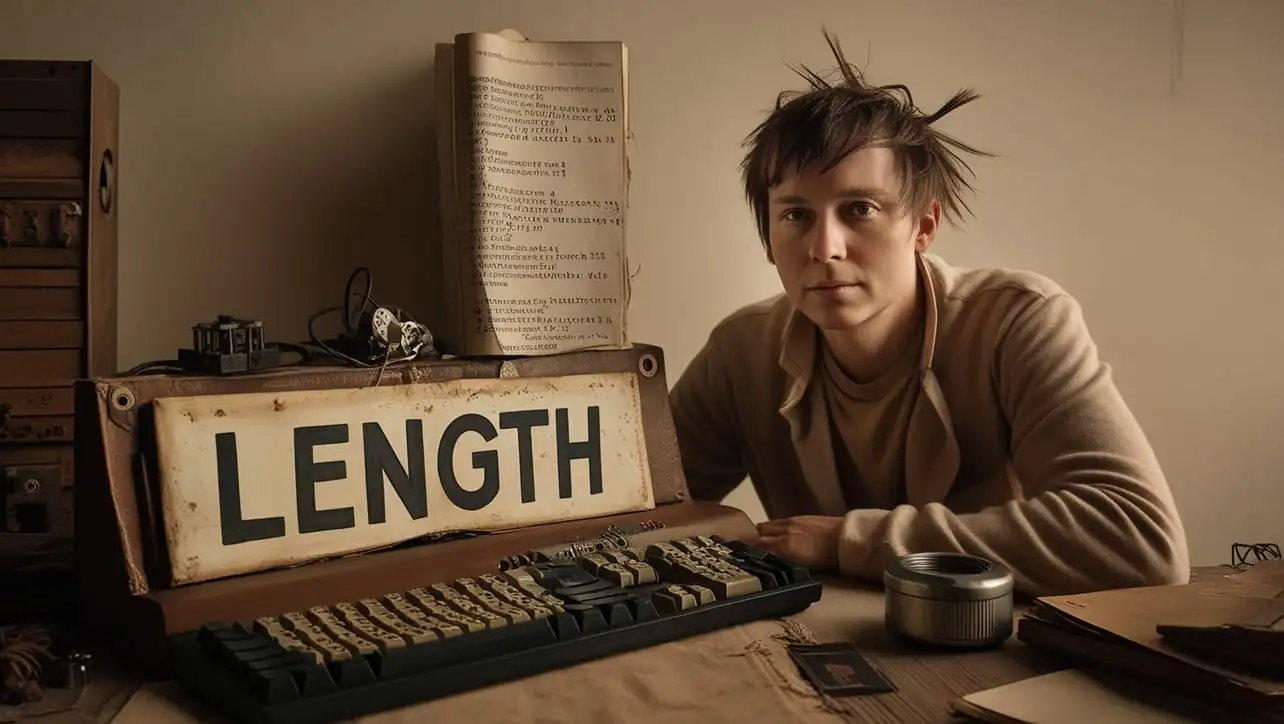
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array flat() Method
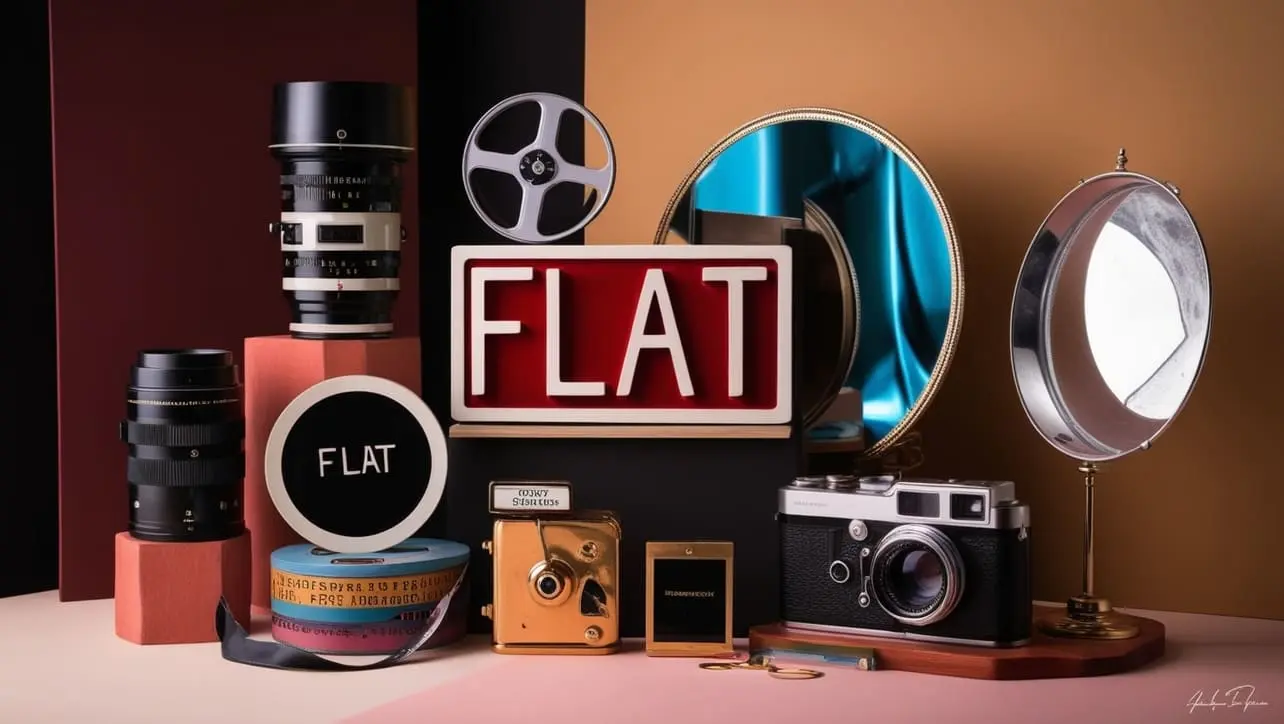
Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays are fundamental data structures, and the flat()
method provides a concise solution for handling nested arrays. This method simplifies the process of flattening nested arrays, making your code more readable and efficient.
In this guide, we'll explore the flat()
method, understand its syntax, delve into example usage, discuss best practices, and explore practical use cases.
🧠 Understanding flat() Method
The flat()
method is designed to flatten nested arrays by a specified depth. This depth determines how deeply nested arrays should be recursively flattened. By default, the method flattens the array by one level.
💡 Syntax
The syntax for the flat()
method is straightforward:
array.flat([depth]);
- array: The array to be flattened.
- depth (optional): The depth to which the array should be flattened. Default is 1.
📝 Example
Let's explore a basic example to illustrate the usage of the flat()
method:
// Nested array
const nestedArray = [1, [2, [3, 4, [5]]]];
// Using flat() to flatten the array
const flattenedArray = nestedArray.flat();
console.log(flattenedArray);
// Output: [1, 2, [3, 4, [5]]]
In this example, the flat()
method is applied to nestedArray, resulting in a flattened array by one level.
🏆 Best Practices
When working with the flat()
method, consider the following best practices:
Specify Depth Carefully:
Be mindful of the depth parameter to avoid over-flattening or under-flattening your array.
example.jsCopied// Correct usage - flattening by two levels const doubleFlattened = nestedArray.flat(2); // Incorrect usage - flattening by one level (default) const incorrectFlattened = nestedArray.flat();
Error Handling for Non-Numeric Depths:
Ensure that the specified depth is a numeric value. Non-numeric values will result in the default flattening of one level.
example.jsCopiedconst nonNumericDepth = nestedArray.flat('invalid');
📚 Use Cases
Simplifying Arrays with Irregular Nesting:
The
flat()
method is particularly useful when dealing with arrays containing irregular nesting levels:example.jsCopiedconst irregularArray = [1, [2, [3, [4, [5]]]]]; const simplifiedArray = irregularArray.flat(Infinity); console.log(simplifiedArray); // Output: [1, 2, 3, 4, 5]
Flattening Arrays in a Recursive Structure:
In scenarios where arrays are nested within objects or other complex structures, the
flat()
method helps simplify the array for further processing:example.jsCopiedconst recursiveStructure = [1, { data: [2, [3, 4]], meta: { info: [5] } }]; const flattenedRecursive = recursiveStructure.flat(Infinity); console.log(flattenedRecursive); // Output: [1, { data: [2, [3, 4]], meta: { info: [5] } }]
🎉 Conclusion
The flat()
method is a valuable addition to JavaScript arrays, streamlining the process of handling nested structures.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the flat()
method in your JavaScript projects.
👨💻 Join our Community:
Author
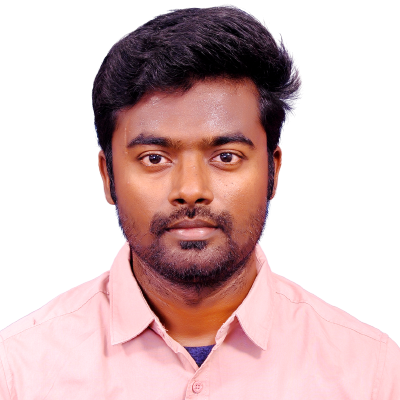
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array flat() Method), please comment here. I will help you immediately.