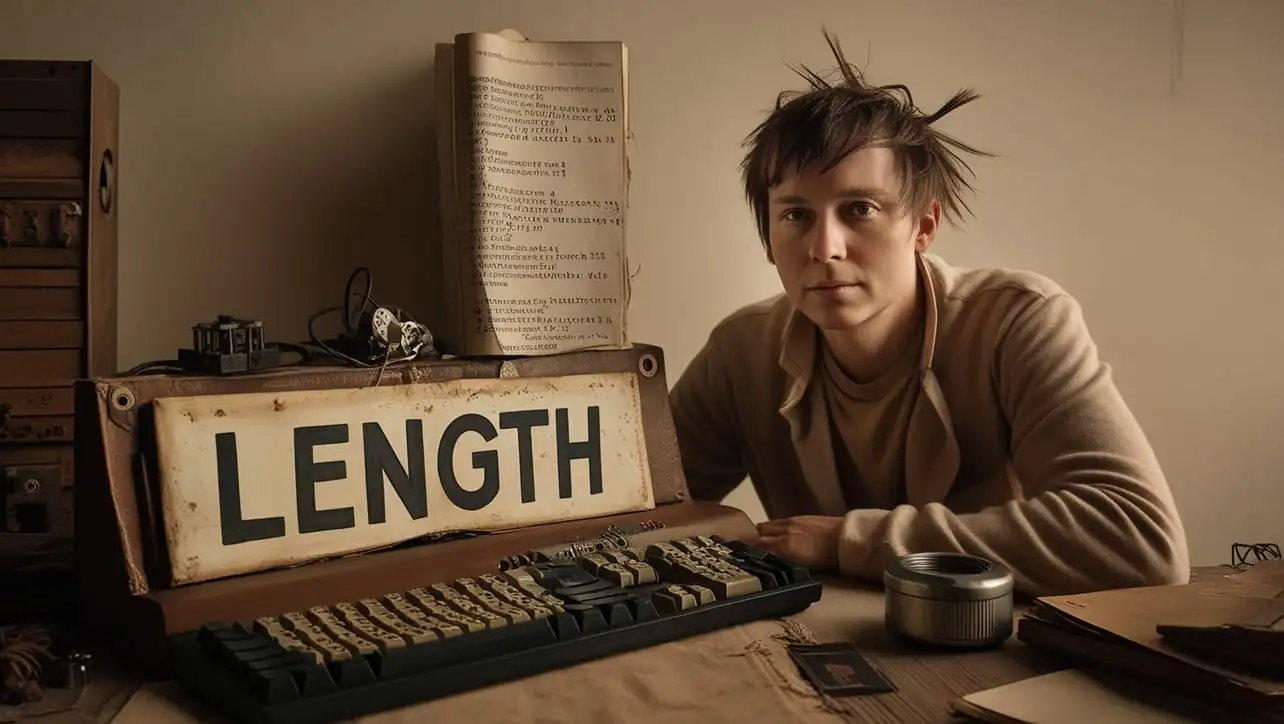
JS Array Methods
JavaScript Array findIndex() Method

Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays offer a wealth of methods for efficient data manipulation, and the findIndex()
method is a powerful addition. This method allows you to locate the index of the first array element that satisfies a given condition.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical applications of the findIndex()
method.
🧠 Understanding findIndex() Method
The findIndex()
method searches through an array and returns the index of the first element that meets the specified condition. If no such element is found, it returns -1. This method is particularly useful when you need to locate an element based on a custom condition.
💡 Syntax
The syntax for the findIndex()
method is straightforward:
array.findIndex(callback(element, index, array), thisArg);
- array: The array to search through.
- callback: A function that accepts up to three arguments:
- element: The current element being processed.
- index: The index of the current element being processed.
- array: The array
findIndex()
was called upon.
- thisArg (Optional): An object to use as this when executing the callback.
📝 Example
Let's illustrate the usage of the findIndex()
method with a practical example:
// Sample array
const numbers = [10, 20, 30, 40, 50];
// Find the index of the first element greater than 25
const index = numbers.findIndex(num => num > 25);
console.log(index); // Output: 2
In this example, the findIndex()
method is used to find the index of the first element greater than 25 in the numbers array.
🏆 Best Practices
When working with the findIndex()
method, consider the following best practices:
Error Handling:
Handle cases where no element satisfies the given condition by checking for a return value of -1.
example.jsCopiedconst index = numbers.findIndex(num => num > 100); if (index === -1) { console.log('No element greater than 100 found.'); }
Compatibility Checking:
Verify the compatibility of the
findIndex()
method in your target environments, especially if supporting older browsers.example.jsCopiedif (!Array.prototype.findIndex) { console.error('findIndex() method not supported in this environment.'); }
📚 Use Cases
Filtering Arrays:
The
findIndex()
method is useful for filtering arrays based on specific conditions:example.jsCopiedconst evenIndex = numbers.findIndex((num, index) => index % 2 === 0); console.log(`Index of the first element with an even index: ${evenIndex}`);
Searching for Objects:
You can use the
findIndex()
method to search for objects within an array based on specific properties:example.jsCopiedconst users = [ { id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' } ]; const index = users.findIndex(user => user.name === 'Bob'); console.log(`Index of Bob: ${index}`);
🎉 Conclusion
The findIndex()
method enriches array manipulation capabilities in JavaScript, offering an efficient means of locating elements based on custom conditions.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the findIndex()
method in your JavaScript projects.
👨💻 Join our Community:
Author
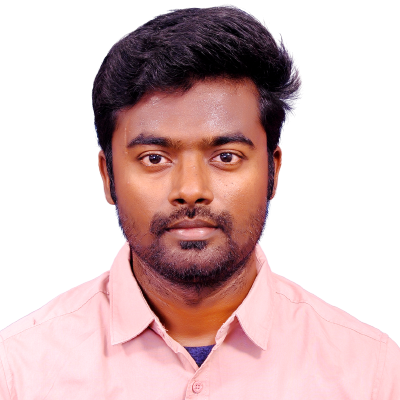
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array findIndex() Method), please comment here. I will help you immediately.